Understanding Terraform: A Comprehensive Guide to Infrastructure as Code (IaC)
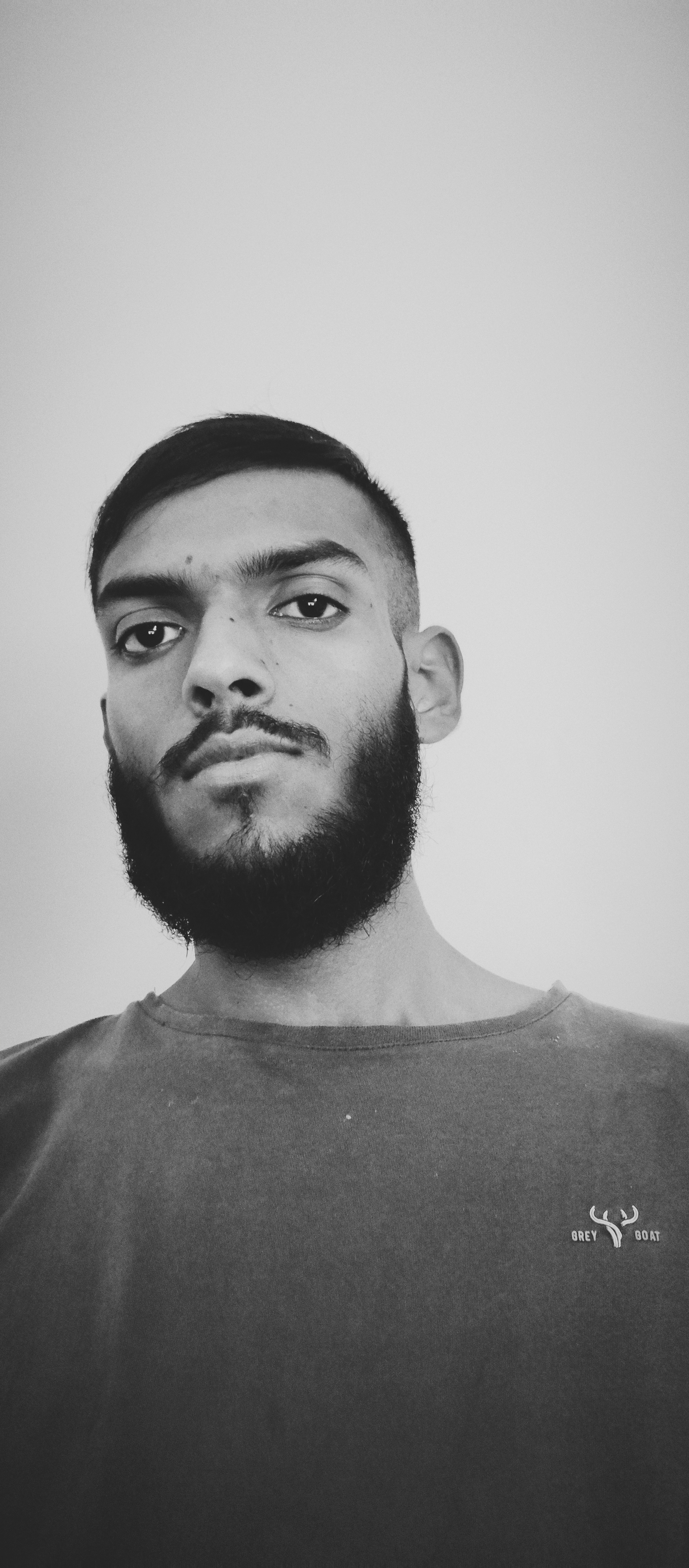
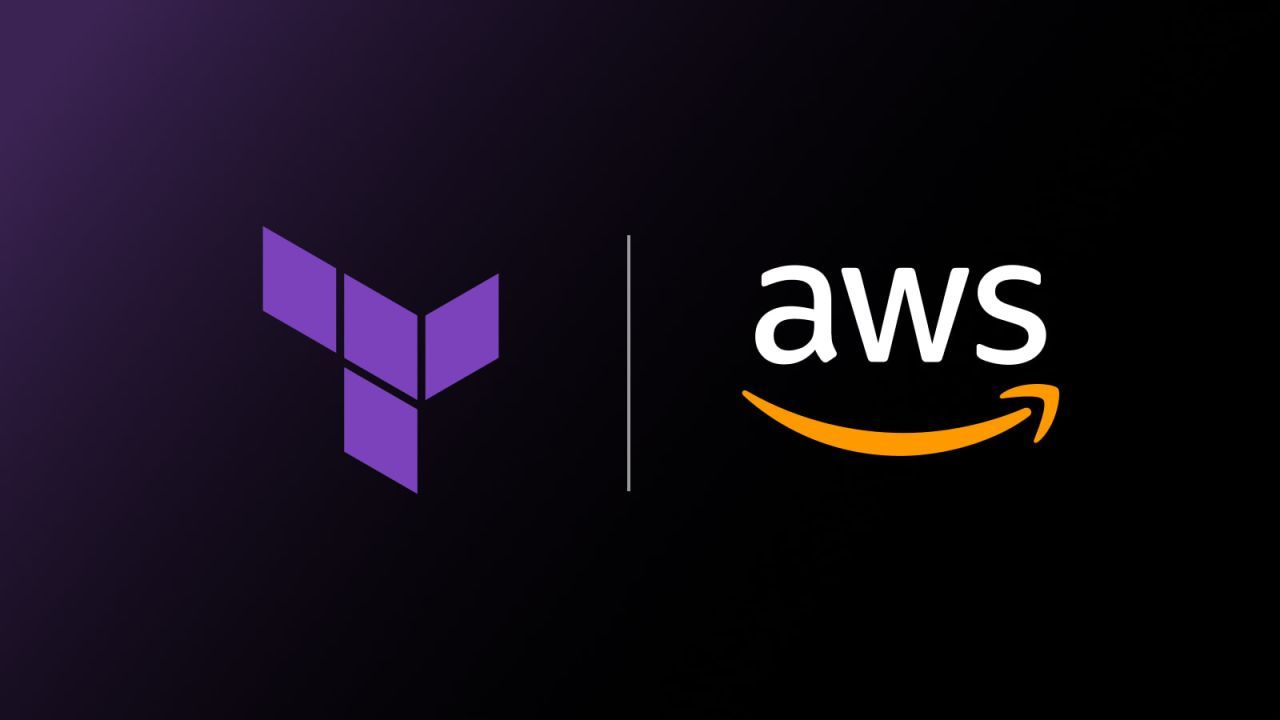
In today's world of cloud computing, managing infrastructure efficiently is crucial. Terraform, an open-source tool by HashiCorp, provides a robust way to define and manage your infrastructure as code (IaC). In this blog, I’ll break down the core concepts of Terraform, how it interacts with cloud providers, and best practices for effective infrastructure management.
What is Terraform?
Terraform is an IaC tool that allows you to define and provision infrastructure using a high-level configuration language. With Terraform, you can describe your infrastructure needs in configuration files, and Terraform will take care of provisioning and managing the resources.
Key Terraform Commands
terraform init
:Purpose: Initializes your Terraform working directory. This command downloads necessary provider plugins and sets up the environment.
Usage: Run this command after writing or modifying your Terraform configuration files.
terraform plan
:Purpose: Generates an execution plan. It shows what Terraform intends to do to reach the desired state defined in your configuration files.
Usage: Use this command to review the changes Terraform will make before applying them.
terraform apply
:Purpose: Applies the changes required to reach the desired state. It makes the necessary API calls to the cloud provider to create, update, or delete resources.
Usage: Run this command to execute the changes outlined in the plan.
terraform destroy
:Purpose: Destroys all the resources defined in your configuration files. This is useful for cleaning up resources when they are no longer needed.
Usage: Use this command to remove resources and clean up your environment.
Writing Configuration Files
Configuration files are the heart of Terraform. They are written in HashiCorp Configuration Language (HCL) or JSON and typically have a .tf
extension. Here’s a basic example:
provider "aws" {
region = "us-west-2" }
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro" }
provider "aws": Specifies the cloud provider (AWS in this case).
resource "aws_instance" "example": Defines an EC2 instance resource.
This "example" is not the actual name that will be given to the instance in aws.
It is for referencing within the configuration files.
For actual name we can use the following :
tags = {
Name = "Terraform_Demo"
}
This name would be visible in the EC2 instance dashboard in the AWS management console .
State Management
Terraform uses a state file (terraform.tfstate
) to keep track of resources. This file is crucial because it maps your configuration to actual resources in the cloud. Here’s a detailed look at state management:
Local State:
Description: The state file is stored on your local machine in a file named
terraform.tfstate
.Pros: Simple setup for single-user scenarios or small projects.
Cons: Not suitable for team collaboration or production environments as it lacks features like state locking and versioning.
Remote State:
Description: The state file is stored in a remote location, such as AWS S3 or Terraform Cloud.
Pros: Ideal for team collaboration and production environments. Remote state provides state locking, versioning, and centralized management.
Cons: Requires setup and configuration of remote storage solutions.
Best Practices for State Management:
Use Remote Backends: Store your state files in remote backends like AWS S3 for better management and collaboration.
State File Security: Avoid pushing state files to version control systems as they contain sensitive information. Restrict access to the state files to prevent unauthorized changes.
State File Locking: Implement state file locking (e.g., using DynamoDB with S3) to prevent concurrent modifications that could lead to inconsistencies.
A Good Setup for Terraform
Store Configuration Files in Version Control: Keep your
.tf
files in a version control system like GitHub. This allows for tracking changes, collaboration, and maintaining a history of your infrastructure configurations.Integrate with CI/CD Tools: Use Continuous Integration/Continuous Deployment (CI/CD) tools to automate the execution of Terraform commands. This ensures that changes to your infrastructure are applied consistently and automatically.
Use Remote Backend Storage: Store your state files in a remote backend storage solution, such as AWS S3. This centralizes the state file, making it accessible across different environments and users.
Implement State File Locking: Integrate your remote state storage with a state locking mechanism, such as DynamoDB for AWS . This prevents simultaneous execution of Terraform scripts by locking the state file, avoiding inconsistencies and conflicts in the infrastructure.
Using Modules
Modules are a powerful feature in Terraform that help organize and reuse infrastructure configurations. They are containers for a set of resources and configurations. Here’s a closer look at modules:
Defining a Module:
Description: A module is defined as a directory containing configuration files. Each module should encapsulate a specific set of resources or functionality.
Example: Create a directory named
my_module
with a filemain.tf
defining your resources.
resource "aws_security_group" "my_sg" {
name = "my-security-group" }
Using a Module:
Description: Reference the module in your main configuration file to reuse its resources.
Example: Use the module defined in
my_module
in your main configuration file.
module "network" {
source = "./my_module" }
Benefits of Using Modules:
Reusability: Modules allow you to reuse code across different parts of your infrastructure.
Organization: Helps keep your configurations organized and modular.
Maintainability: Simplifies updates and maintenance by encapsulating functionality in separate modules.
Conclusion
Terraform is a powerful tool for managing cloud infrastructure through code. By understanding its core concepts, including state management and module usage, you can effectively provision and manage your infrastructure. Using best practices for state management and leveraging modules will help ensure your infrastructure remains organized, secure, and scalable.
Subscribe to my newsletter
Read articles from Frepin Gonsalvese directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
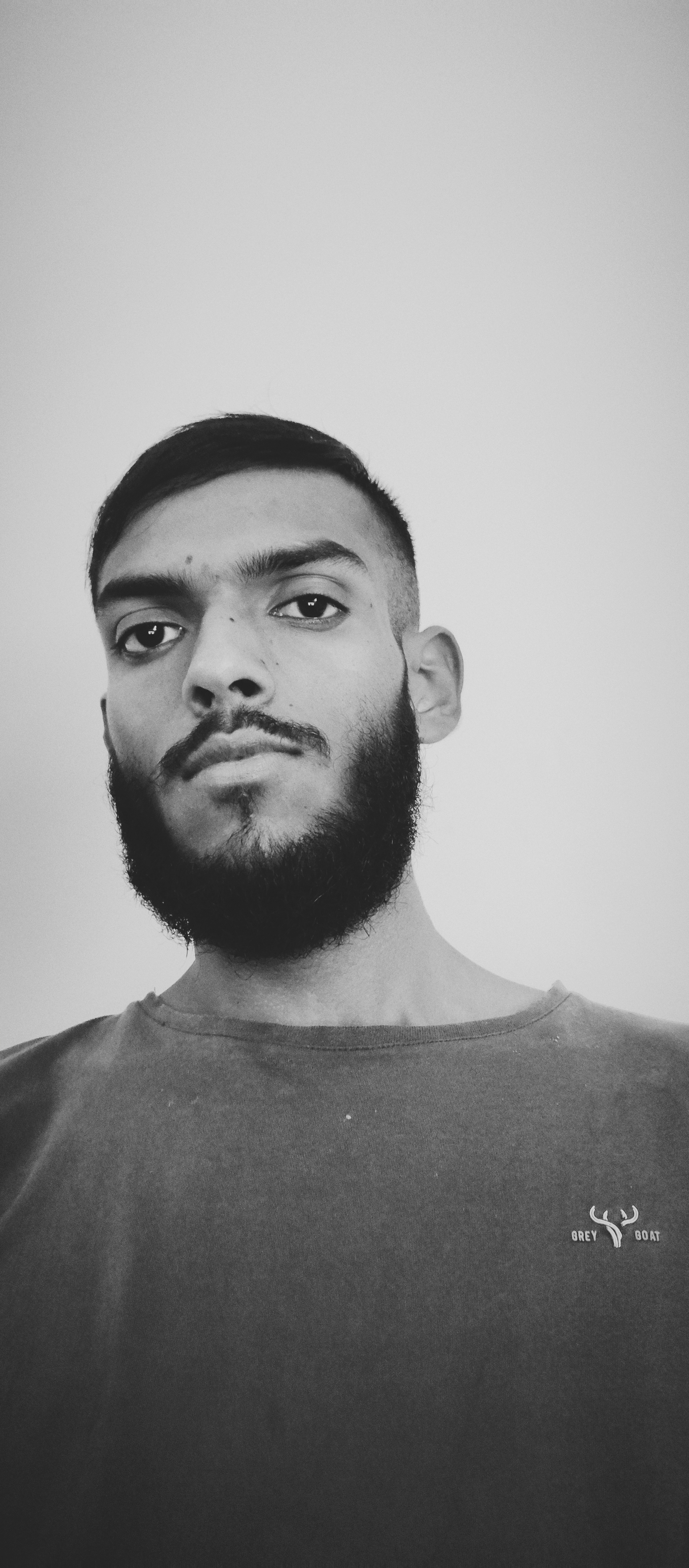