Friendly Machine Learning for the Web: Kickstart Your AI/ML Journey with JavaScript Using ML5 🚀
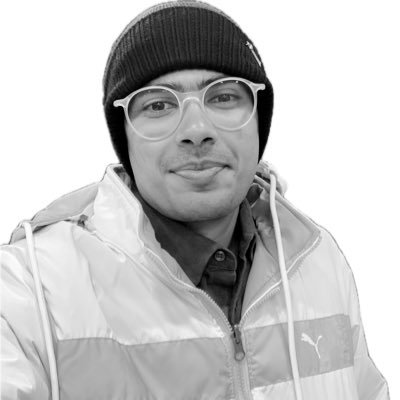
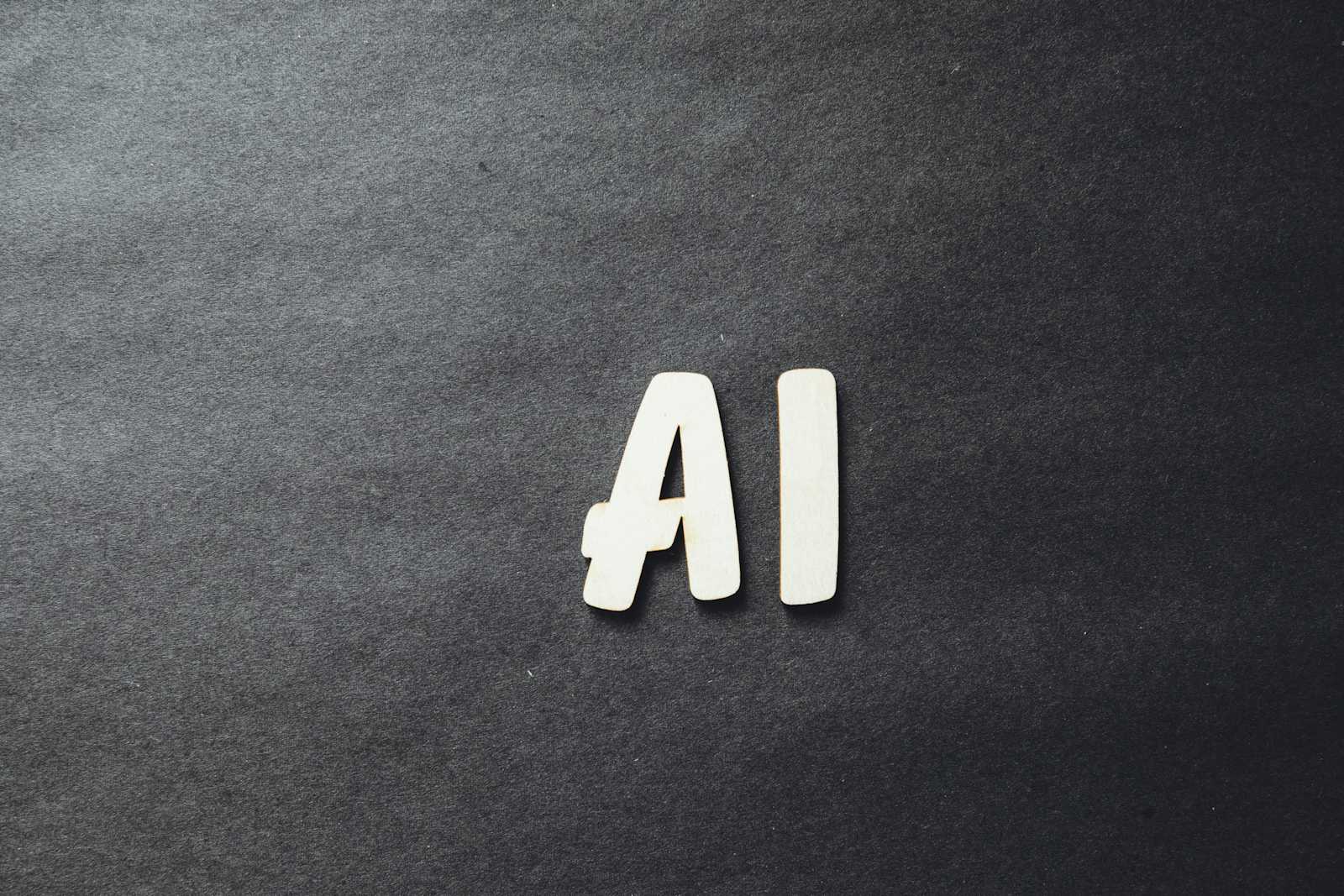
Machine learning (ML) and artificial intelligence (AI) have become essential tools in today's technology landscape. However, diving into the world of ML can seem daunting, especially if you're not familiar with the complex mathematics and algorithms involved. But what if you could start your AI/ML journey right from your web browser using JavaScript? That’s where ML5 comes in—a friendly, easy-to-use library that brings machine learning to the web, making it accessible to developers of all skill levels.
In this blog post, we'll explore how you can get started with ML5, build your first machine learning model, and integrate it into your web projects. 🌐
What is ML5? 🤔
ML5 is an open-source JavaScript library that provides a simple interface for working with machine learning in the browser. It’s built on top of TensorFlow.js, offering pre-trained models and easy-to-use functions that allow you to create interactive, intelligent web applications without needing a deep understanding of machine learning concepts.
🌟 Key Features of ML5
Pre-trained Models: Use pre-trained models for tasks like image classification, pose detection, and text generation.
Ease of Use: A simple API that abstracts away the complexities of machine learning, allowing you to focus on building cool stuff.
Client-Side Execution: All models run directly in the browser, ensuring privacy and reducing latency.
Interactive Learning: Experiment and see results in real-time, making ML5 a great tool for learning and prototyping.
Getting Started with ML5 🛠️
Step 1: Setting Up Your Project
To get started, you'll need to set up a basic HTML file and include the ML5 library. Here’s how you can do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ML5 Example</title>
<!-- Include the ML5 library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/ml5/0.6.0/ml5.min.js"></script>
</head>
<body>
<h1>Machine Learning with ML5</h1>
<p id="result">Loading model...</p>
<script src="app.js"></script>
</body>
</html>
Step 2: Implementing Your First Model
Next, let’s add some JavaScript to classify an image using a pre-trained model. In this example, we’ll use the Image Classification model:
// app.js
// Initialize the Image Classifier method with MobileNet
const classifier = ml5.imageClassifier('MobileNet', modelLoaded);
// When the model is loaded
function modelLoaded() {
console.log('Model Loaded!');
document.getElementById('result').innerText = 'Model Loaded!';
}
// Classify an image
classifier.classify(document.getElementById('image'), (err, results) => {
if (err) {
console.error(err);
} else {
console.log(results);
document.getElementById('result').innerText = `Label: ${results[0].label}`;
}
});
Step 3: Displaying Results 🎉
Now, add an image to your HTML file, and the model will classify it as soon as the page loads:
<img id="image" src="path_to_your_image.jpg" alt="Test Image" />
When you open the page, ML5 will load the MobileNet model, classify the image, and display the result on the screen. It’s that simple!
Exploring More with ML5 🚀
ML5 offers a wide range of pre-trained models that you can experiment with, from PoseNet (for detecting human poses) to CharRNN (for text generation). Here are a few ideas to get your creative juices flowing:
🤖 1. Build an Image Classifier
Create a web app that can classify images uploaded by users. This could be anything from identifying objects in photos to distinguishing between different types of plants.
💬 2. Text Generation with CharRNN
Generate creative writing, poetry, or even code by training a model on your own dataset using CharRNN.
🎨 3. Real-Time Pose Detection
Use PoseNet to build an interactive app that reacts to the user’s movements, opening up possibilities for games, fitness applications, or digital art installations.
Why Choose ML5 for Your Projects? 🌟
ML5 is designed to make machine learning accessible to everyone, regardless of their technical background. Here are a few reasons why you should consider using it:
Accessibility: No need for specialized hardware or software—everything runs in the browser.
Community and Support: A vibrant community of developers and educators who contribute to the library and provide help through forums and social media.
Flexibility: Whether you’re building a prototype or a full-fledged web application, ML5 scales to meet your needs.
🦾
ML5 brings the power of machine learning to the web in a way that’s friendly and accessible to developers of all levels. By simplifying the process of integrating AI/ML into your web projects, ML5 allows you to focus on creativity and innovation, rather than getting bogged down by the complexities of machine learning algorithms.
Whether you're looking to build a fun side project or start your journey into AI/ML, ML5 is a fantastic place to begin. So why not give it a try? 🚀
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
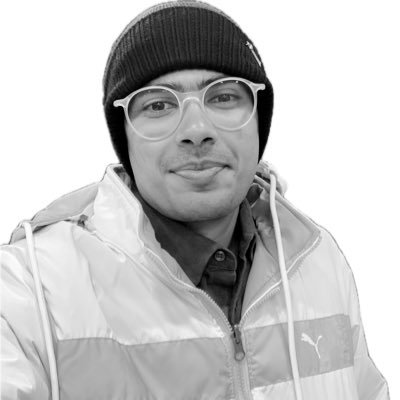
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on ⌨️ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix 👨🏻💻