Boosting Web Performance with Debouncing and Throttling Techniques
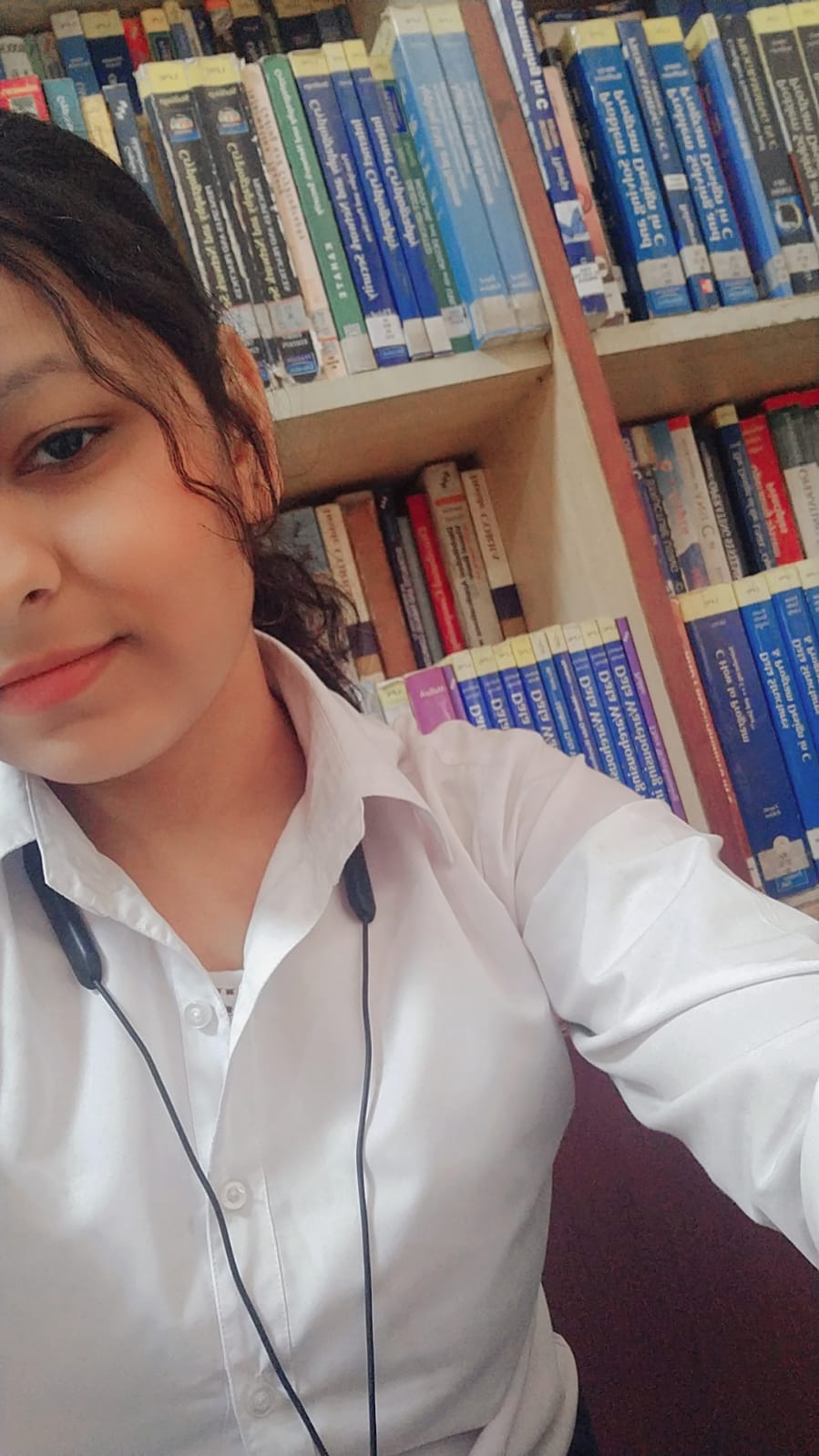
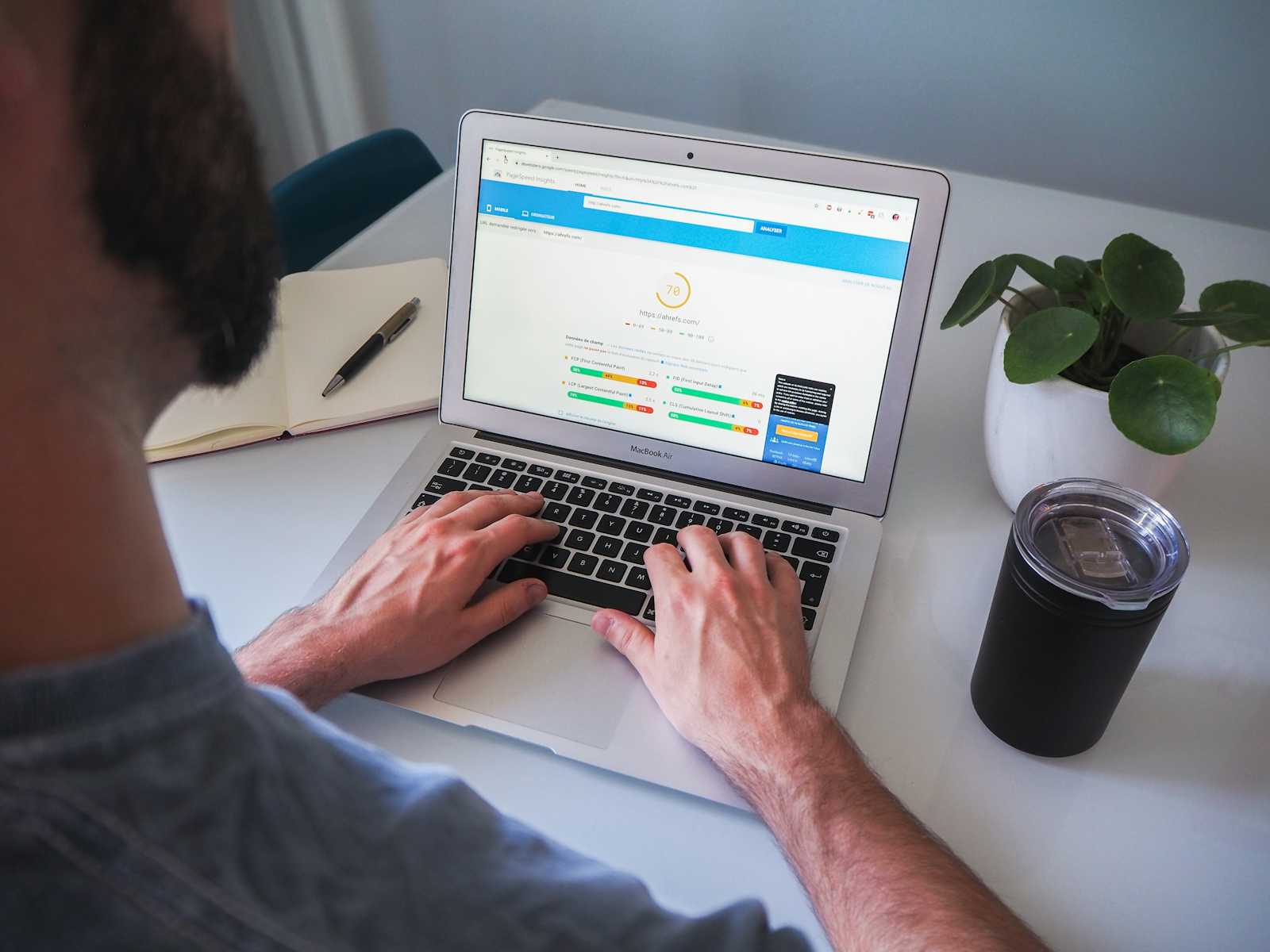
When you are developing a web app you need to be very performant and you can achieve great performance using debouncing and throttling techniques.
So in this article, we will learn about the concept of debouncing and throttling, how they differ, and when to use each.
Understanding the Need for Debouncing
Have you ever experienced a laggy search bar that triggers an API call on every keystroke, causing a choppy user experience? Debouncing comes to the rescue! It's a technique that optimizes web page performance by reducing the frequency of function calls, especially during continuous user interactions. In essence, it allows us to wait for a slight pause in user input before triggering the associated action. This is particularly valuable in scenarios like search bars, where we can hold off making API calls until the user has finished typing.
Example: Suppose there is a scenario where we need to check is username available so if we make an API call on every keystroke that would not be optimised. It also reduces the performance. But by using debouncing we can reduce the number of API calls and load on the server. We will only make an API call when the user pauses writing certain words.
How debouncing works
The Conventional Way:
The idea behind debouncing is simple: you set a timer to wait for a specified period (e.g. 300ms). If the event is triggered again before the timer expires, the timer is reset. The function is only executed if the timer completes without interruption.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" onkeyup="betterfunction()"/>
<script src="index.js"></script>
</body>
</html>
let count =0;
const getData = () =>{
// calls api and gets data
console.log('fetching data from api', count++);
}
const debounce = function(fn, delay){
let timer;
return function(){
let context = this;
args = arguments;
clearTimeout(timer);
timer = setTimeout(()=>{
fn.apply(context, arguments);
},delay);
}
}
const betterfunction = debounce(getData,300);
apart from this conventional method, we can also use the useDebounceValue or useDebounceCallback from usehooks-ts https://usehooks-ts.com/
Common use cases:
Form Validation: Running validation only after the user has stopped making changes.
Resizing: Adjusting the layout only after the window resize event has stopped.
Searching: Triggering a search function only after the user has stopped typing.
Text Editor Autosave: Implementing autosave in a text editor? Debouncing ensures that saving only happens after the user stops typing.
As we see in Leetcode and other common platforms
Throttling
Rather than debouncing throttling is more about control. Throttling is a technique that ensures a function is called at most once within a specified time interval. Unlike debouncing, which waits until there’s a pause in event firing, throttling ensures the function is executed at regular intervals, regardless of how many times the event is triggered.
Use cases:
Resizing: Continuously adjusting the layout at regular intervals while the window is being resized.
Mouse Movements: Tracking the mouse position at a consistent rate.
Scrolling: Updating the UI at regular intervals while the user scrolls.
Button Clicks: Prevent issues like double-clicking by throttling button clicks to ensure a single action at a time.
Thank You for Reading!
Subscribe to my newsletter
Read articles from Radha Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
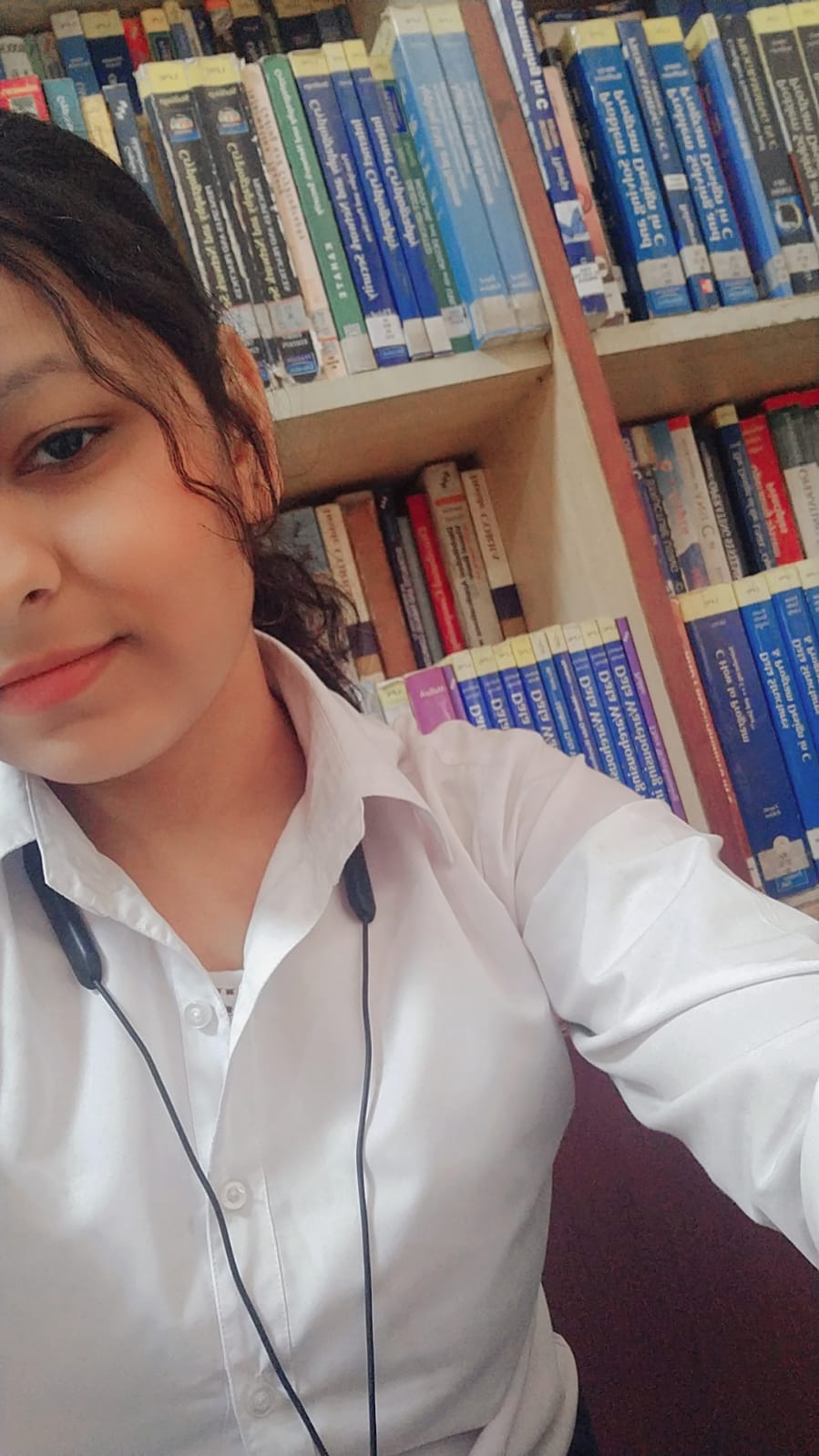
Radha Sharma
Radha Sharma
Web Developer