Tanstack Table : How and When to use
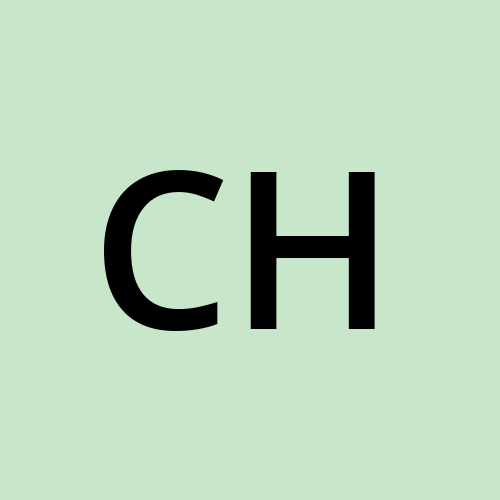
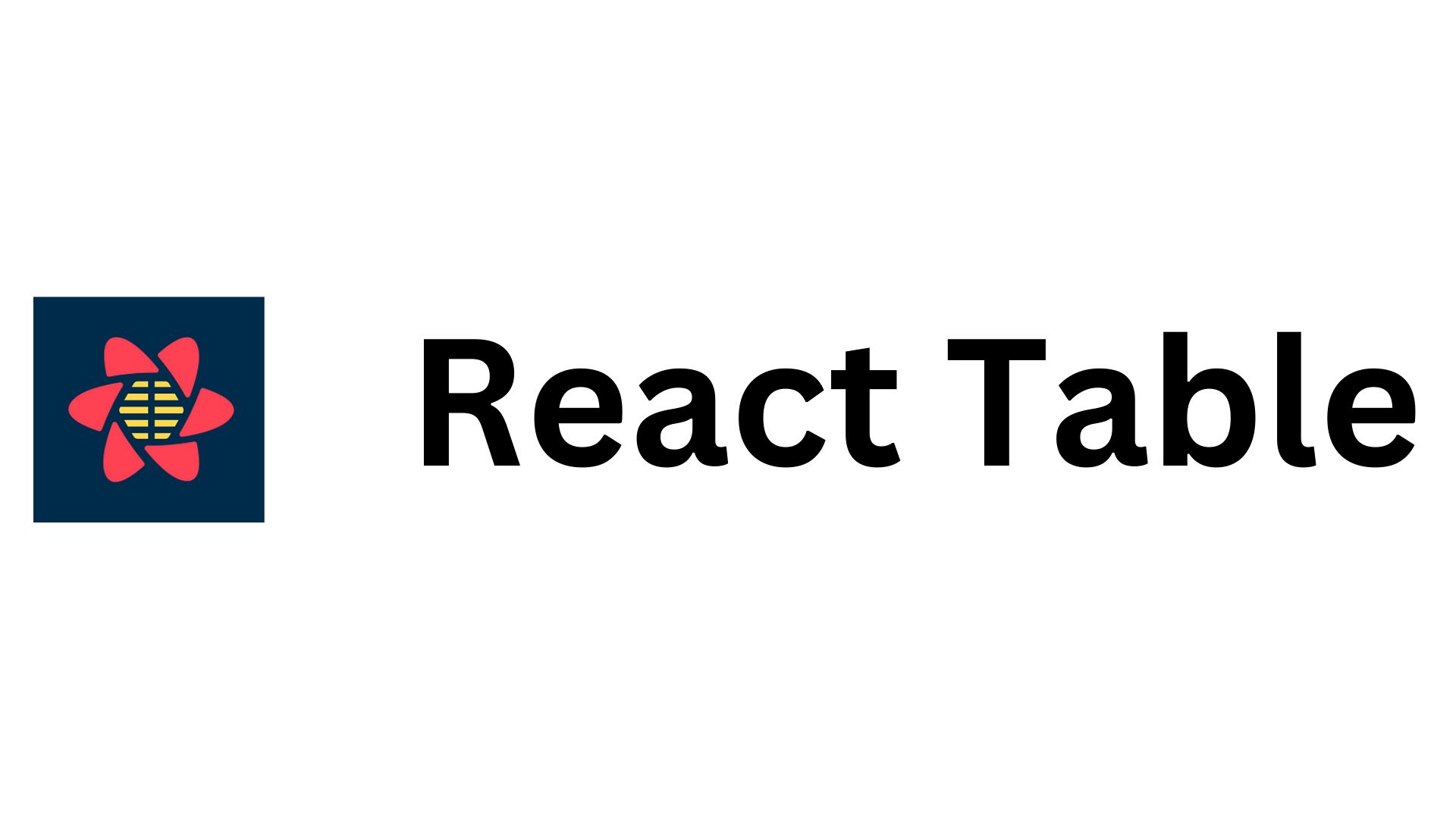
TanStack Table, previously known as React Table, is a powerful data grid library for building highly customizable and performant tables in modern web applications. It offers a wide range of features, including sorting, filtering, pagination, and grouping, while maintaining a lightweight footprint and providing excellent performance.
Why Use TanStack Table?
TanStack Table is an ideal choice when you need:
Complex Data Presentation: If your application requires the display of complex datasets with features like sorting, filtering, and grouping, TanStack Table provides a robust solution that can handle these needs efficiently.
Customizable UI: Unlike other table libraries that come with predefined styles, TanStack Table allows you to fully customize the appearance of your tables, making it easier to match your application's design language.
Scalability: Whether you're working with a small dataset or millions of rows, TanStack Table is built to handle large-scale data efficiently, thanks to its virtualization capabilities.
When to Use TanStack Table
Enterprise Applications: In applications where data presentation is critical, such as dashboards, reporting tools, or data management systems, TanStack Table's advanced features and customization options make it a perfect fit.
Data-Heavy Applications: If your application needs to display large datasets with performance in mind, TanStack Table's virtualization and efficient rendering ensure smooth interactions even with extensive data.
Custom Requirements: When you need to implement unique table features not provided out-of-the-box by other libraries, TanStack Table's flexible API allows you to build custom solutions without compromising performance.
Understanding TanStack Table Hooks: useTable
, useQuery
, and useMutation
TanStack Table provides several powerful hooks to manage and manipulate table data in a React application. Let's explore some of these hooks, focusing on their parameters, return values, and practical use cases.
useTable
The useTable
hook is the core of TanStack Table. It manages the state and logic of your table, providing methods and properties to build custom table components.
Parameters:
options
: An object containing the configuration for your table, including:columns
: An array defining the structure of your table's columns.data
: The dataset to be displayed in the table.initialState
: An object to define the initial state of the table (e.g., sorting, pagination).
plugins
: Optional array of plugins to enhance table functionality (e.g.,useSortBy
,usePagination
).
Returns:
An object containing methods and properties to manage table behavior, such as:
getTableProps
: Props for the table element.getTableBodyProps
: Props for the table body element.headerGroups
: An array of header groups for rendering column headers.rows
: An array of row objects representing the data.prepareRow
: A function to prepare a row before rendering.
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({ columns, data });
useQuery
useQuery
is not a direct TanStack Table hook but is often used alongside it when working with data-fetching libraries like React Query.
Parameters:
key
: A unique key for the query, typically an array (e.g.,['users']
).queryFn
: A function that returns a promise, fetching the data.options
: An optional object for configuring query behavior (e.g.,staleTime
,cacheTime
,enabled
).
Returns:
An object containing the query state, including:
data
: The fetched data, orundefined
if not yet loaded.error
: Any error encountered during fetching.isLoading
: A boolean indicating if the query is in the loading state.isSuccess
: A boolean indicating if the query succeeded.const { data, error, isLoading } = useQuery(['users'], fetchUsers);
useMutation
useMutation
is used for creating, updating, or deleting data, again often used with React Query or TanStack Query.
Parameters:
mutationFn
: A function that performs the mutation (e.g., creating or updating data).options
: An optional object for configuring mutation behavior, including:onSuccess
: A callback function to execute when the mutation succeeds.onError
: A callback function to execute when the mutation fails.onSettled
: A callback to execute when the mutation is either successful or fails.
Returns:
An object with methods and state properties, including:
mutate
: A function to trigger the mutation.mutateAsync
: A promise-based version ofmutate
.isLoading
: A boolean indicating if the mutation is in progress.isError
: A boolean indicating if the mutation failed.isSuccess
: A boolean indicating if the mutation succeeded.
const { mutate, isLoading, isError } = useMutation(createUser, {
onSuccess: () => {
console.log('User created successfully');
},
});
Conclusion
The hooks provided by TanStack Table, along with complementary hooks like useQuery
and useMutation
from React Query, offer powerful tools for managing data and state in your React applications. These hooks, such as useTable
, handle core table functionality, while others facilitate data fetching and mutation, enabling you to build interactive and responsive tables tailored to your needs.
In addition to these, there are other hooks available within the TanStack ecosystem, such as useSortBy
, useFilters
, and usePagination
, which further extend the capabilities of your tables, allowing for advanced sorting, filtering, and pagination.
Subscribe to my newsletter
Read articles from Chandni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
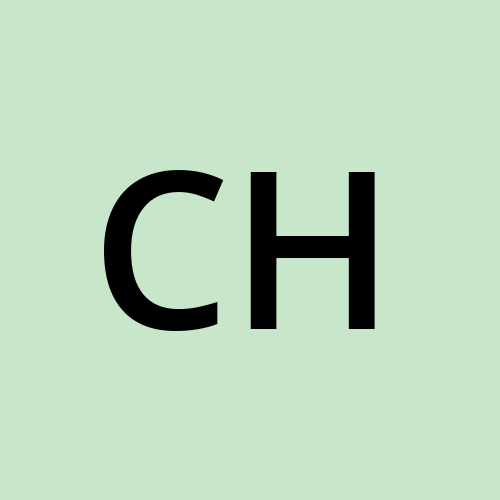