Introduction to Docker file, Image & Container

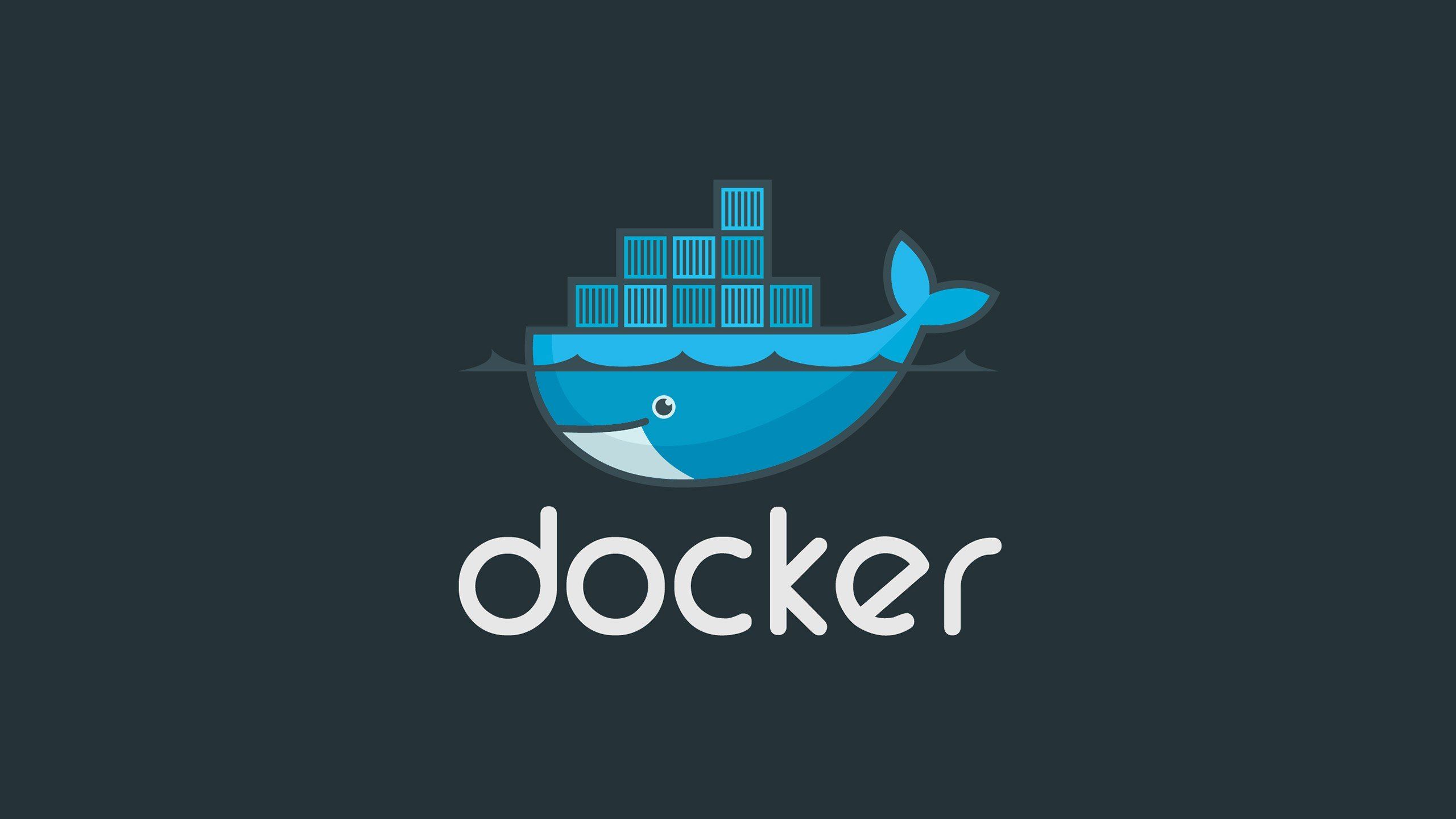
Dockerfile
A Dockerfile is a crucial component in Docker that automates the process of building Docker images. It consists of a series of instructions that Docker uses to create a container image, detailing how the application inside the container should be set up.
Understanding Dockerfile Syntax:
FROM
: Specifies the base image to use for the Docker image.RUN
: Executes commands in a new layer on top of the current image and commits the results.COPY
andADD
: Copies files/directories from the host to the container.CMD
: Provides the default command to run when a container starts.ENTRYPOINT
: Configures a container to run as an executable.ENV
: Sets environment variables.EXPOSE
: Informs Docker that the container listens on the specified network ports.VOLUME
: Creates a mount point with the specified path and marks it as holding externally mounted volumes from the host or other containers.WORKDIR
: Sets the working directory for subsequent instructions.
Example :
# Use an official Node.js image as a base
FROM node:14
# Set the working directory
WORKDIR /usr/src/app
# Copy package.json and install dependencies
COPY package*.json ./
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose port 3000 and start the application
EXPOSE 3000
CMD ["npm", "start"]
Docker Images
Docker images are the building blocks of containerized applications. They are immutable, lightweight, and portable files that include everything needed to run an application, such as the code, runtime, libraries, environment variables, and configurations.
1. Understanding Docker Images
What is a Docker Image?
- A Docker image is a read-only template used to create Docker containers. It includes everything needed to run a piece of software, from the operating system (OS) to the application code.
Image Layers
Docker images are composed of multiple layers. Each layer represents an instruction in the Dockerfile, such as
FROM
,COPY
, orRUN
.Layers are stacked on top of each other and form a single unified view in the final image.
Layers are shared across images, making Docker images lightweight and efficient.
2. Creating Docker Images
Using a Dockerfile
- The most common way to create a Docker image is by writing a Dockerfile, a simple text file containing instructions on how to build the image.
Example Dockerfile :
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y nginx
COPY index.html /var/www/html/
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
Build the image :
docker build -t my-nginx-image .
Building from an Existing Container
- You can also create a Docker image from an existing container using the
docker commit
command. This is useful for creating images from manually configured containers.
docker commit <container_id> my-custom-image
3. Managing Docker Images
Image Tags
- Tags are used to identify different versions of a Docker image. A tag typically includes the software version or build information.
Example :
docker build -t my-image:1.0 .
docker build -t my-image:latest .
4 . Docker Image Commands
docker images
: Lists all Docker images on the system.docker rmi
: Removes one or more images from the local storage.docker pull
: Downloads an image from a Docker registry (e.g., Docker Hub).docker push
: Uploads an image to a Docker registry.
Docker Container
Docker containers are the core of Docker's functionality, enabling the packaging and running of applications in isolated environments. Containers provide a lightweight, portable, and consistent runtime environment, making them a popular choice for deploying applications across different environments.
1. Understanding Docker Containers
What is a Docker Container?
A Docker container is an instance of a Docker image, running as an isolated process on the host operating system.
Containers encapsulate an application and its dependencies, ensuring consistency across development, testing, and production environments.
Containers vs Virtual Machines
Containers: Share the host OS kernel, making them lightweight and fast to start. Each container runs in its own isolated user space.
Virtual Machines (VMs): Include a full OS with its own kernel, making them heavier and slower to start compared to containers.
2. Creating and Running Docker Containers
Starting a Container:
Basic command to start a container
docker run -d --name my-container nginx
This command runs an Nginx container in detached mode (-d
) with the name my-container
.
Container Lifecycle :
Create:
docker create
- Creates a container but does not start it.Start:
docker start
- Starts an existing container.Stop:
docker stop
- Stops a running container.Pause/Unpause:
docker pause
/docker unpause
- Pauses/resumes a container’s processes.Remove:
docker rm
- Deletes a stopped container.
Interactive Containers :
Running a container interactively:
docker run -it ubuntu bash
The -it
flag allows you to interact with the container’s shell.
Networking in Containers :
- Containers are connected to a virtual network by default. Docker provides several network drivers, including
bridge
,host
,none
, andoverlay
.
Example of exposing a port :
docker run -d -p 8080:80 nginx
This maps port 8080 on the host to port 80 in the container.
3. Managing Docker Containers
Inspecting Containers
The
docker inspect
command provides detailed information about a container, including its configuration, state, and network settings.Example:
docker inspect my-container
Viewing Logs
To view logs from a running container, use:
docker logs my-container
Monitoring Containers
Docker provides tools like
docker stats
for real-time monitoring of container resource usage.Example:
docker stats my-container
Managing Container Volumes
Volumes are used to persist data generated by containers. They can be shared between containers or backed up/restored.
Example:
docker run -d -v my-volume:/data busybox
Subscribe to my newsletter
Read articles from Dinesh Kumar K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dinesh Kumar K
Dinesh Kumar K
Hi there! I'm Dinesh, a passionate Cloud and DevOps enthusiast. I love to dive into the latest new technologies and sharing my journey through blog.