Creating Views and Templates to Display Blog Posts
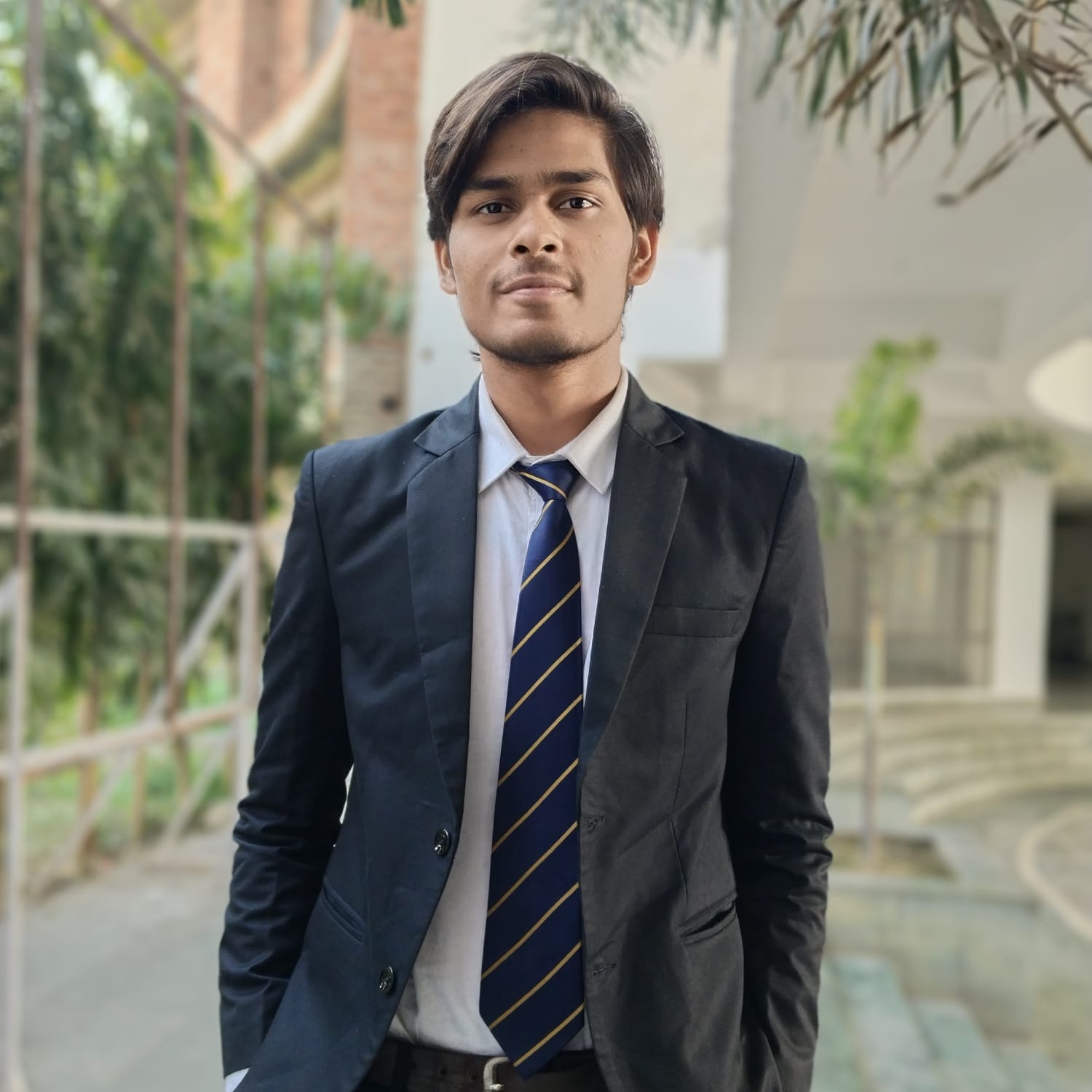
Welcome back! After setting up our Django project and creating a blog app, it’s time to make those blog posts visible to users on our website. In this article, we'll cover creating views and templates to display the blog posts in a web page. If you think of Django’s views as the "brains" that process data and templates as the "face" that displays it, we’re going to bring these two parts together to show your blog posts.
1. Creating Views
Views in Django are functions that handle requests and return responses. They control what content is displayed to the user and how it's displayed. In this step, we’ll create a view that fetches all the blog posts from the database and sends them to a template for display.
Open Your
views.py
File:Navigate to the
blog
directory in your project.Open the
views.py
file in your text editor or IDE.
Define the View Function: Add the following code to
views.py
:from django.shortcuts import render from .models import BlogPost def index(request): posts = BlogPost.objects.all() # Fetch all blog posts from the database return render(request, 'blog/index.html', {'posts': posts})
Explanation:
from django.shortcuts import render
: Imports therender
function, which is used to combine a template with context data.from .models import BlogPost
: Imports theBlogPost
model so we can query the database for blog posts.def index(request):
: Defines a view function namedindex
. Therequest
argument contains information about the current web request.posts = BlogPost.objects.all()
: Retrieves all instances of theBlogPost
model from the database. This will be a list of all blog posts.return render(request, 'blog/index.html', {'posts': posts})
: Uses therender
function to generate the HTML response. It takes three arguments:request
: The request object.'blog/index.html'
: The path to the template that will be used to display the data.{'posts': posts}
: A dictionary where the key'posts'
will be available in the template, holding the list of blog posts.
2. Creating Templates
Templates are HTML files that Django uses to render content. They define how the content will look on the web page. Let’s create a template to display the list of blog posts.
Create the Templates Directory:
Inside the
blog
directory, create a new directory namedtemplates
.Within the
templates
directory, create another directory namedblog
. Your directory structure should look like this:blog/ templates/ blog/ index.html
Create
index.html
:Inside the
blog/templates/blog
directory, create a file namedindex.html
.Add the following HTML code to
index.html
:<!DOCTYPE html> <html> <head> <title>Blog Posts</title> </head> <body> <h1>Blog Posts</h1> <ul> {% for post in posts %} <li> <h2>{{ post.title }}</h2> <p>{{ post.content }}</p> <p><em>Published on {{ post.pub_date }}</em></p> </li> {% empty %} <li>No blog posts available.</li> {% endfor %} </ul> </body> </html>
Explanation:
<!DOCTYPE html>
: Declares that this is an HTML5 document.<html>...</html>
: Wraps all the content of your web page.<head>...</head>
: Contains meta-information about the document, such as the title.<body>...</body>
: Contains the content of the web page.{% for post in posts %} ... {% endfor %}
: A Django template tag that loops through theposts
list. For each post, it creates an HTML list item displaying the title, content, and publication date.{% empty %}
: Provides a fallback message if there are no blog posts to display.
3. Configuring URLs
To ensure that users can access the page that displays blog posts, we need to map a URL to our view.
Create
urls.py
in the Blog Directory:- In the
blog
directory, create a file namedurls.py
.
- In the
Add URL Patterns:
Add the following code to
urls.py
:from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), ]
Explanation:
from django.urls import path
: Imports thepath
function used to define URL patterns.from . import views
: Imports theviews
module where our view function is defined.path('', views.index, name='index')
: Maps the root URL of the blog app (''
) to theindex
view function. This means that when users visithttp://127.0.0.1:8000/
, Django will call theindex
function and use theindex.html
template to display the content.
Include Blog URLs in the Project’s URL Configuration:
Explanation:
path('admin/',
admin.site
.urls)
: Includes the URL patterns for Django’s admin interface.path('', include('blog.urls'))
: Includes the URL patterns defined in theblog.urls
module. This means that the root URL (''
) will be handled by theblog
app.
4. Testing the Blog Page
Now it’s time to see your blog posts in action!
Start the Development Server:
Make sure your Django development server is running. If it’s not, start it with:
python manage.py runserver
Visit the Blog Page:
Open your web browser and navigate to
http://127.0.0.1:8000/
.You should see a list of blog posts displayed on the page. If you don’t see anything, check the following:
Make sure the server is running and there are no errors in the terminal.
Verify that you have correctly set up your view function, URL patterns, and template.
Congratulations! You’ve successfully created a view to display blog posts and set up a template to render them on your website. You’ve also configured URL patterns to make sure users can access your blog posts. In the next article, we’ll add more features to our blog, such as individual blog post pages and navigation options. Stay tuned for more exciting steps in building your blog app!
Subscribe to my newsletter
Read articles from Shivay Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
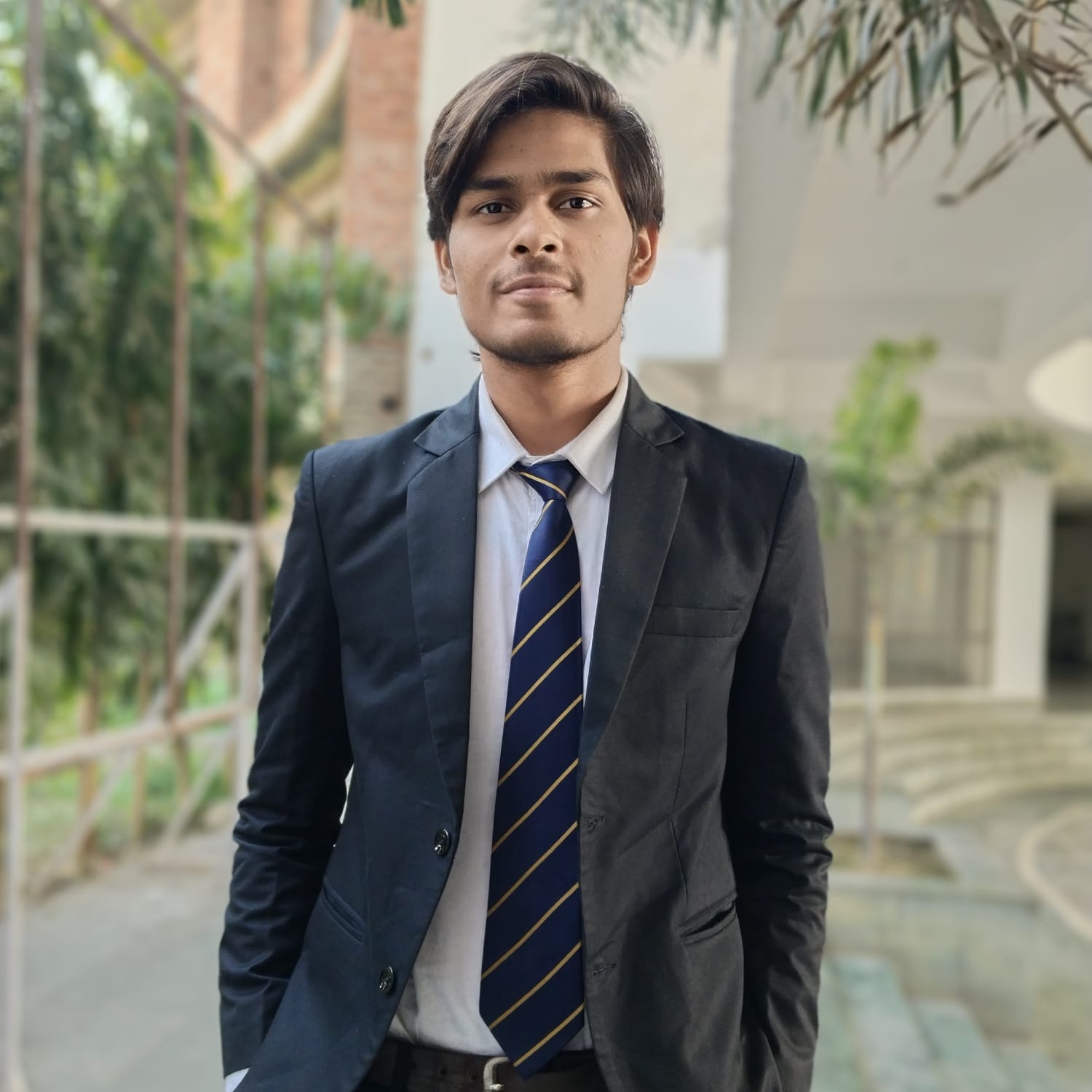