How to Create a Personal Finance Tracker with Python
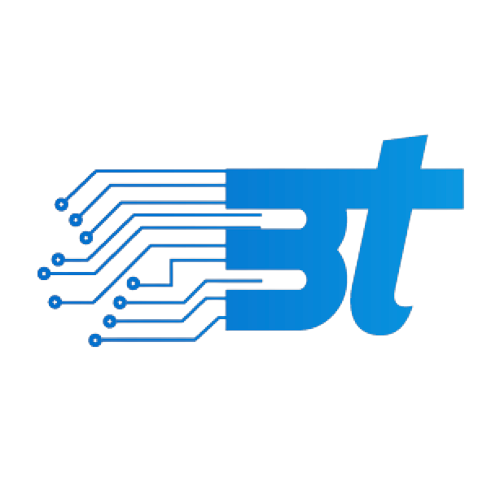
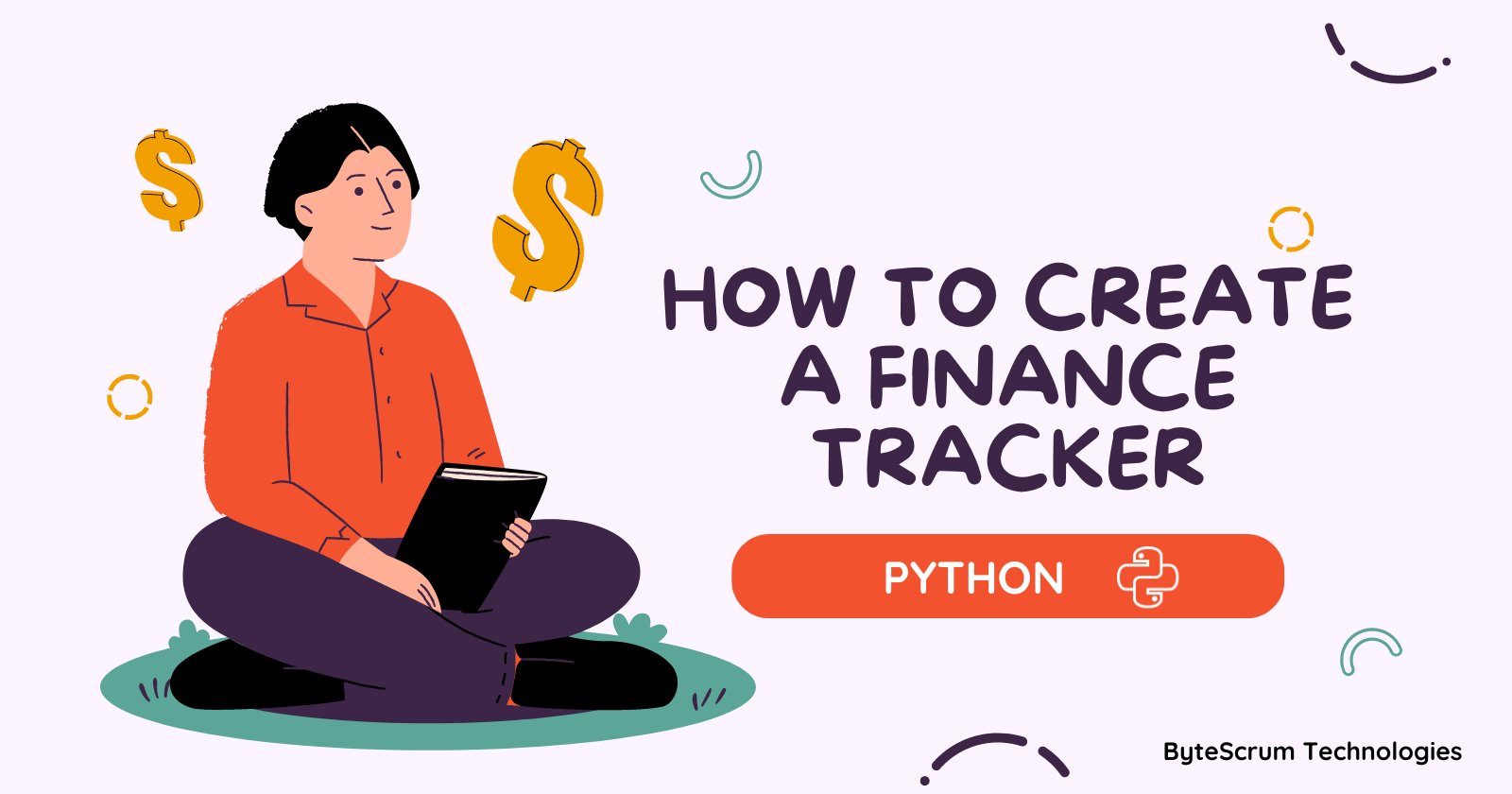
Managing personal finances is more than just tracking income and expenses. As your financial situation grows more complex, you may need a more sophisticated tool to keep track of investments, budgets, savings goals, and financial forecasting. In this blog, we’ll walk through building an advanced personal finance tracker using Python. This script will not only track your transactions but also provide insights into your spending patterns, help you set and achieve savings goals, and even predict your future financial status.
What You’ll Learn:
Advanced data manipulation with
pandas
.Using
matplotlib
andplotly
for interactive visualizations.Implementing budgeting and savings goals.
Tracking investments and net worth.
Financial forecasting with time series analysis.
1. Setting Up the Environment
Install Additional Libraries:
In addition to pandas
and matplotlib
, we’ll use plotly
for interactive charts and statsmodels
for financial forecasting.
pip install pandas matplotlib plotly statsmodels
2. Enhancing the Data Structure
To accommodate more complex financial data, we'll extend our DataFrame to include columns for tracking budgets, savings goals, and investments.
Step 1: Define the Data Structure
We'll create a DataFrame that tracks not only transactions but also the current balance, budget, savings goals, and investment performance.
import pandas as pd
from datetime import datetime
# Extended Data Structure
columns = ['Date', 'Description', 'Category', 'Amount', 'Type', 'Account', 'Balance', 'Budget', 'Savings Goal', 'Investment Value']
transactions = pd.DataFrame(columns=columns)
# Initial Setup
accounts = {'Cash': 0, 'Bank': 0, 'Investment': 0}
budgets = {'Food': 500, 'Rent': 1000, 'Entertainment': 200}
savings_goals = {'Emergency Fund': 5000, 'Vacation': 2000}
investments = {'Stocks': 10000, 'Bonds': 5000}
def update_balance(transaction):
account = transaction['Account']
transactions['Balance'] = accounts[account] + transactions['Amount'].cumsum()
accounts[account] = transactions['Balance'].iloc[-1]
3. Adding Features
Step 2: Budget Tracking
Allow users to set budgets for various categories and track their spending against these budgets.
def add_transaction(date, description, category, amount, trans_type, account):
global transactions
transaction = {
'Date': datetime.strptime(date, '%Y-%m-%d'),
'Description': description,
'Category': category,
'Amount': amount,
'Type': trans_type,
'Account': account,
'Balance': 0,
'Budget': budgets.get(category, 0),
'Savings Goal': savings_goals.get(category, 0),
'Investment Value': investments.get(category, 0)
}
transactions = transactions.append(transaction, ignore_index=True)
update_balance(transaction)
# Example Usage
add_transaction('2024-08-01', 'Salary', 'Income', 3000, 'Income', 'Bank')
add_transaction('2024-08-02', 'Groceries', 'Food', -150, 'Expense', 'Cash')
Step 3: Tracking Savings Goals
Automatically track progress toward savings goals and visualize them.
def plot_savings_goals():
goals = pd.DataFrame(list(savings_goals.items()), columns=['Goal', 'Target'])
progress = transactions[transactions['Type'] == 'Income'].groupby('Category').sum()['Amount']
goals['Progress'] = progress
goals['Remaining'] = goals['Target'] - goals['Progress']
goals.plot(kind='barh', stacked=True, color=['#00aaff', '#ffcc00'])
plt.title('Savings Goals Progress')
plt.xlabel('Amount')
plt.show()
plot_savings_goals()
4. Investment Tracking
Step 4: Integrating Investment Performance
Track the performance of your investments, including stocks, bonds, and other assets.
def update_investments(investment_name, current_value):
global investments
investments[investment_name] = current_value
transactions.loc[transactions['Category'] == investment_name, 'Investment Value'] = current_value
# Example Usage
update_investments('Stocks', 10500)
update_investments('Bonds', 5100)
Step 5: Plotting Investment Performance
Use plotly
to create interactive plots of your investment performance.
import plotly.graph_objs as go
def plot_investment_performance():
investment_data = pd.DataFrame(list(investments.items()), columns=['Investment', 'Value'])
fig = go.Figure(data=[go.Bar(x=investment_data['Investment'], y=investment_data['Value'])])
fig.update_layout(title='Investment Performance', xaxis_title='Investment', yaxis_title='Value')
fig.show()
plot_investment_performance()
5. Financial Forecasting
Step 6: Predicting Future Financial Status
Use time series analysis to predict future income, expenses, and overall financial health.
import statsmodels.api as sm
def forecast_finances():
# Aggregate monthly data
monthly_data = transactions.resample('M', on='Date').sum()
# Predict next 6 months of income and expenses
model = sm.tsa.statespace.SARIMAX(monthly_data['Amount'], order=(1, 1, 1), seasonal_order=(1, 1, 1, 12))
results = model.fit()
forecast = results.get_forecast(steps=6)
forecast_ci = forecast.conf_int()
ax = monthly_data['Amount'].plot(label='Observed', figsize=(10, 6))
forecast.predicted_mean.plot(ax=ax, label='Forecast')
ax.fill_between(forecast_ci.index, forecast_ci.iloc[:, 0], forecast_ci.iloc[:, 1], color='k', alpha=.2)
ax.set_xlabel('Date')
ax.set_ylabel('Amount')
plt.legend()
plt.show()
forecast_finances()
6. Building a User-Friendly Interface
Step 7: Creating a Command-Line Interface (CLI)
Enhance the script by adding a CLI that makes it easier to interact with your finance tracker.
import argparse
def main():
parser = argparse.ArgumentParser(description="Advanced Personal Finance Tracker")
parser.add_argument('--add', nargs=5, metavar=('date', 'description', 'category', 'amount', 'type', 'account'),
help="Add a new transaction")
parser.add_argument('--plot-savings', action='store_true', help="Plot savings goals progress")
parser.add_argument('--plot-investments', action='store_true', help="Plot investment performance")
parser.add_argument('--forecast', action='store_true', help="Forecast future finances")
args = parser.parse_args()
if args.add:
add_transaction(*args.add)
if args.plot_savings:
plot_savings_goals()
if args.plot_investments:
plot_investment_performance()
if args.forecast:
forecast_finances()
if __name__ == "__main__":
main()
Conclusion
By implementing this tracker, you can gain deeper insights into your finances, set and achieve financial goals, and plan for the future with confidence. As you continue to develop this tool, consider integrating it with online financial services, adding machine learning models for more accurate predictions, or even creating a web or mobile app version.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
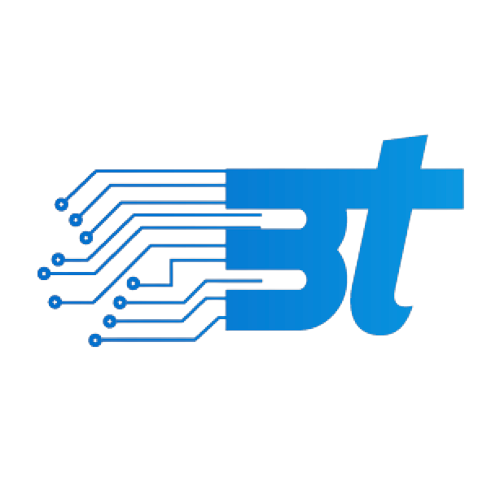
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.