Safeguard your App from Cross-Site Scripting (XSS) Attack
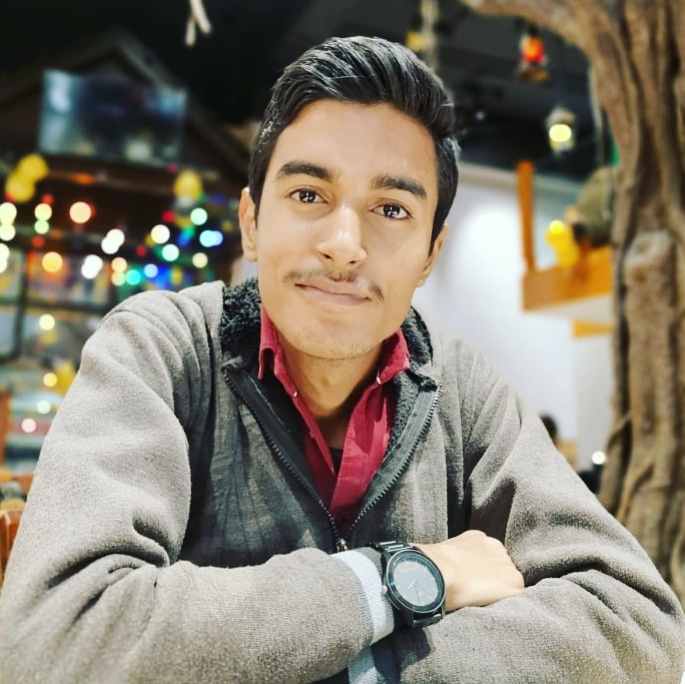
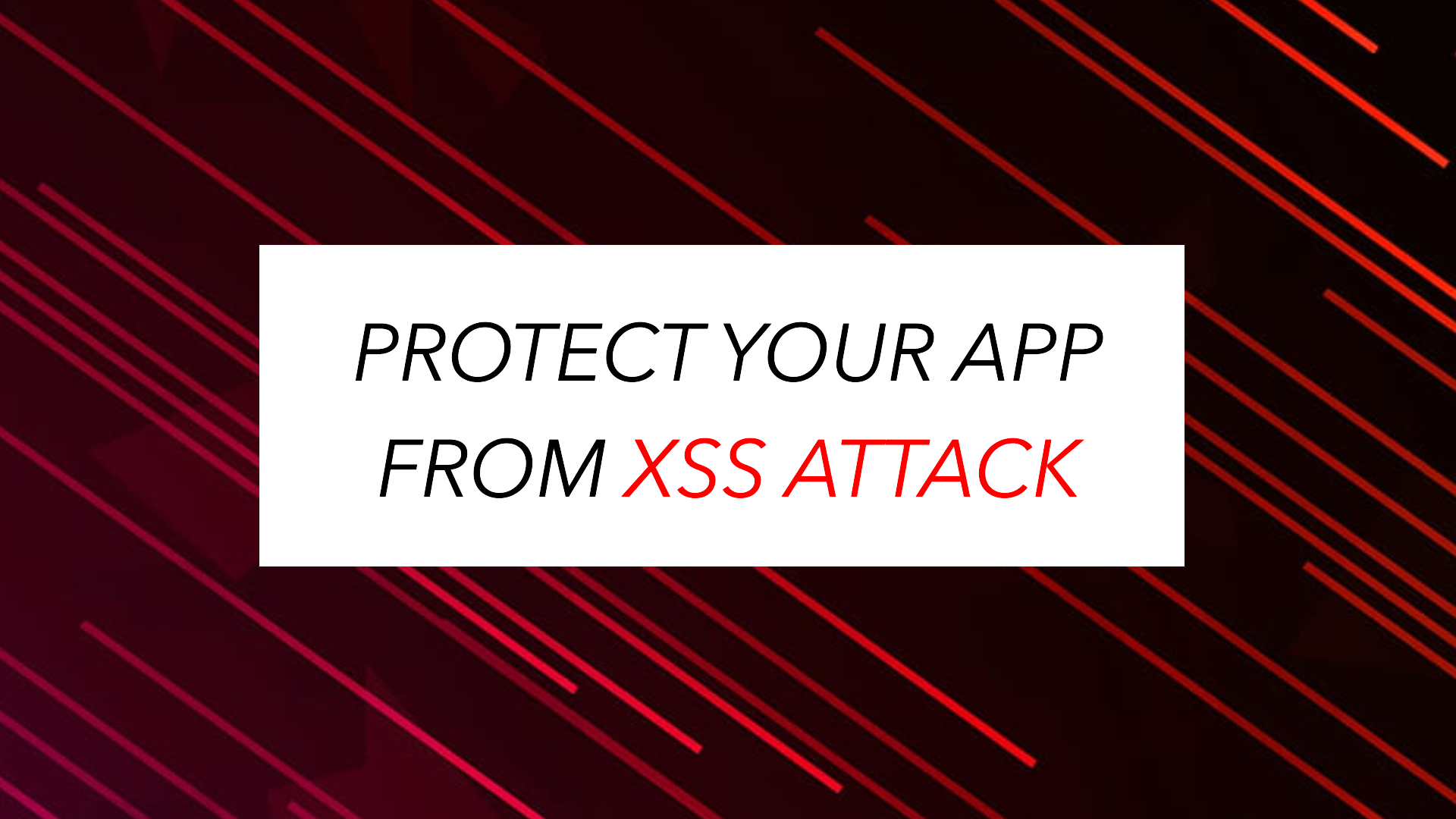
What is Cross-Site Scripting (XSS)?
Cross-site scripting (XSS) is a type of injection attack that allows malicious scripts to be injected into trusted websites. When a vulnerable site includes content from an untrusted source without proper validation, an attacker can inject client-side script into web pages viewed by other users.
How Does XSS Work?
There are primarily three types of XSS attacks:
Reflected XSS: The malicious script is reflected off the web server to the user's browser.
- Example: A search box on a website that doesn't properly sanitize input. An attacker could inject a malicious script into the search query, and when another user clicks on the search result, the script executes.
Stored XSS: The malicious script is permanently stored on the server.
- Example: A comment section on a blog that doesn't sanitize input. An attacker can inject malicious script into a comment, and any user who views the comment will execute the script.
DOM-based XSS: The malicious script is executed within the client-side document object model (DOM).
- Example: A website that dynamically generates content based on user input without proper sanitization can be vulnerable to DOM-based XSS.
Impact of XSS Attacks
XSS attacks can have severe consequences:
Data theft: Attackers can steal sensitive information such as cookies, session tokens, or credit card details.
Session hijacking: Attackers can hijack user sessions to perform actions on behalf of the user.
Malicious code execution: Attackers can execute malicious code on the victim's machine, leading to malware infection or system compromise.
Defacement: Attackers can modify the website's appearance.
Phishing: Attackers can trick users into revealing sensitive information.
Prevention and Mitigation
To protect against XSS attacks, follow these best practices:
Input Validation: Always validate and sanitize user input before using it in dynamic content.
Output Encoding: Properly encode output to prevent malicious script execution.
HTTP Headers: Use HTTP headers like
Content-Security-Policy
to restrict content sources.Web Application Firewalls (WAF): Implement a WAF to detect and block XSS attacks.
Regular Updates: Keep web applications and libraries up-to-date with the latest security patches.
Code Review: Conduct regular code reviews to identify potential vulnerabilities.
Example of input validation in PHP:
<?php
function sanitize_input($data) {
$data = trim($data);
$data = stripslashes($data);
$data = htmlspecialchars($data);
return $data;
}
?>
By understanding the mechanics of XSS attacks and implementing robust security measures, you can significantly reduce the risk of falling victim to this threat. Remember, prevention is always better than cure.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
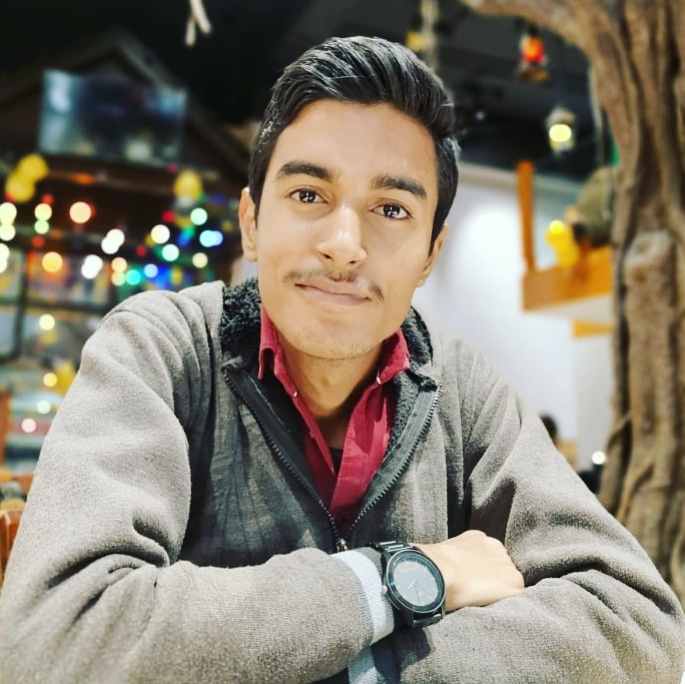
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.