Implement Feature toggles in dotnet in 5 mins

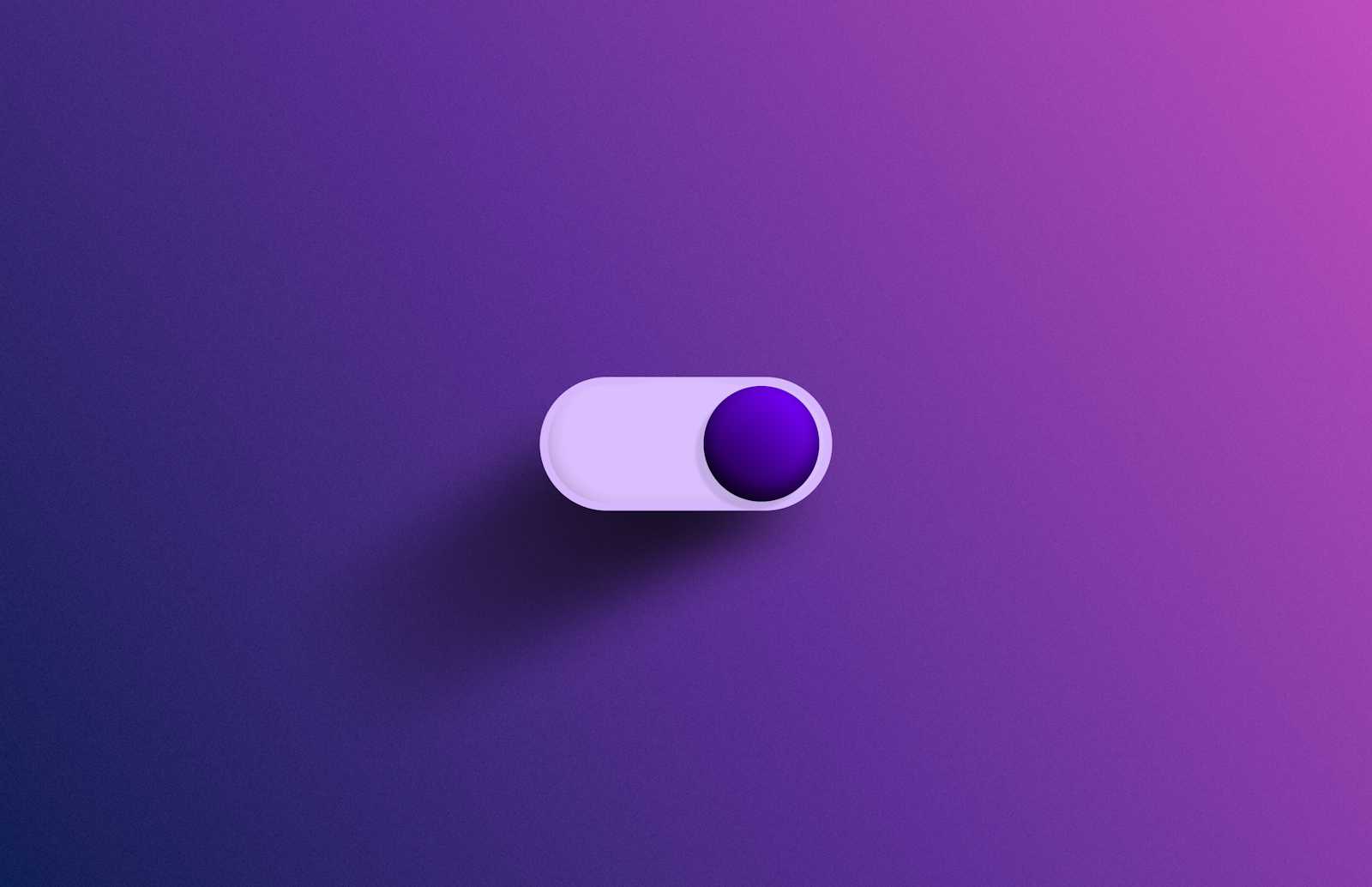
When discussing feature flags, there are numerous aspects to consider.
Which library to use for feature flags, how to integrate with the library, how administrators will manage the feature flags, whether there will be a UI for this purpose or if technical assistance will be required, and so on.
All these questions can be addressed with a single service provided by Azure: App Configurations.
App Configurations can answer all the above questions and offer much more.
The benefits provided by App Config are detailed in this document.
Let's get started.
Create Azure App Config store
Follow this article to create an instance of the App Configuration store.
Once it is created, navigate to the Feature Manager section, Click on Create then select Feature Flag.
Check the Enable feature flag and set "Feature1" in the Name field; all other inputs can be ignored for now.
Click on Apply to finish creating the Feature Flag.
Now, it's time to code.
Integrate in code
Create a simple dotnet web api.
Modify the Startup.cs
file to have below code:
using Microsoft.FeatureManagement;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddLogging(configure => configure.AddConsole());
//Specify how to load configurations from App Config.
builder.Configuration.AddAzureAppConfiguration(options =>
{
options.Connect(builder.Configuration.GetConnectionString("AppConfig"));
options.UseFeatureFlags();
});
// Add Azure App Configuration middleware to the container of services.
builder.Services.AddAzureAppConfiguration();
// Add feature management to the container of services.
builder.Services.AddFeatureManagement();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.MapGet("/feature-flag/Feature1", async (IFeatureManager featureManager) =>
{
var feature1 = await featureManager.IsEnabledAsync("Feature1");
return new
{
FeatureFlag = $"Feature 1 is '{feature1}'"
};
})
.WithName("FeatureFlagDemo")
.WithOpenApi();
app.Run();
Go to appsettings.json
and add below section:
"ConnectionStrings": {
"AppConfig": "Endpoint=https://<you-app>.azconfig.io;Id=1Kz8;Secret=<key>"
}
Now you are ready to run your app hit F5
or dotnet run
and you should be able to connect to Azure App Config and check whether the flag is enabled or not.
Go to the Azure App Config again and disable the Feature1, by default it takes 30s to reflect the changes.
Hit the /feature-flag/Feature1
again and it should response with "Feature 1 is 'False'"
There are many features of Feature Flags that help in rolling out new or experimental features provided by App Config. Some examples include:
Simple On/Off
Time-based
Percentage-based, such as 20% of users being able to use the new feature
Targeted based on user groups, like users who have signed up for beta testing
Browser-based
Custom
Thanks for reading! Find more about feature flags in the references below.
.NET feature flag management - Azure App Configuration | Microsoft Learn
What is Azure App Configuration? | Microsoft Learn
Quickstart for adding feature flags to ASP.NET Core apps - Azure App Configuration | Microsoft Learn
Subscribe to my newsletter
Read articles from Kishan Vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
