Publish and Subscribe Data with Wemos D1 Mini Using MQTT Protocol
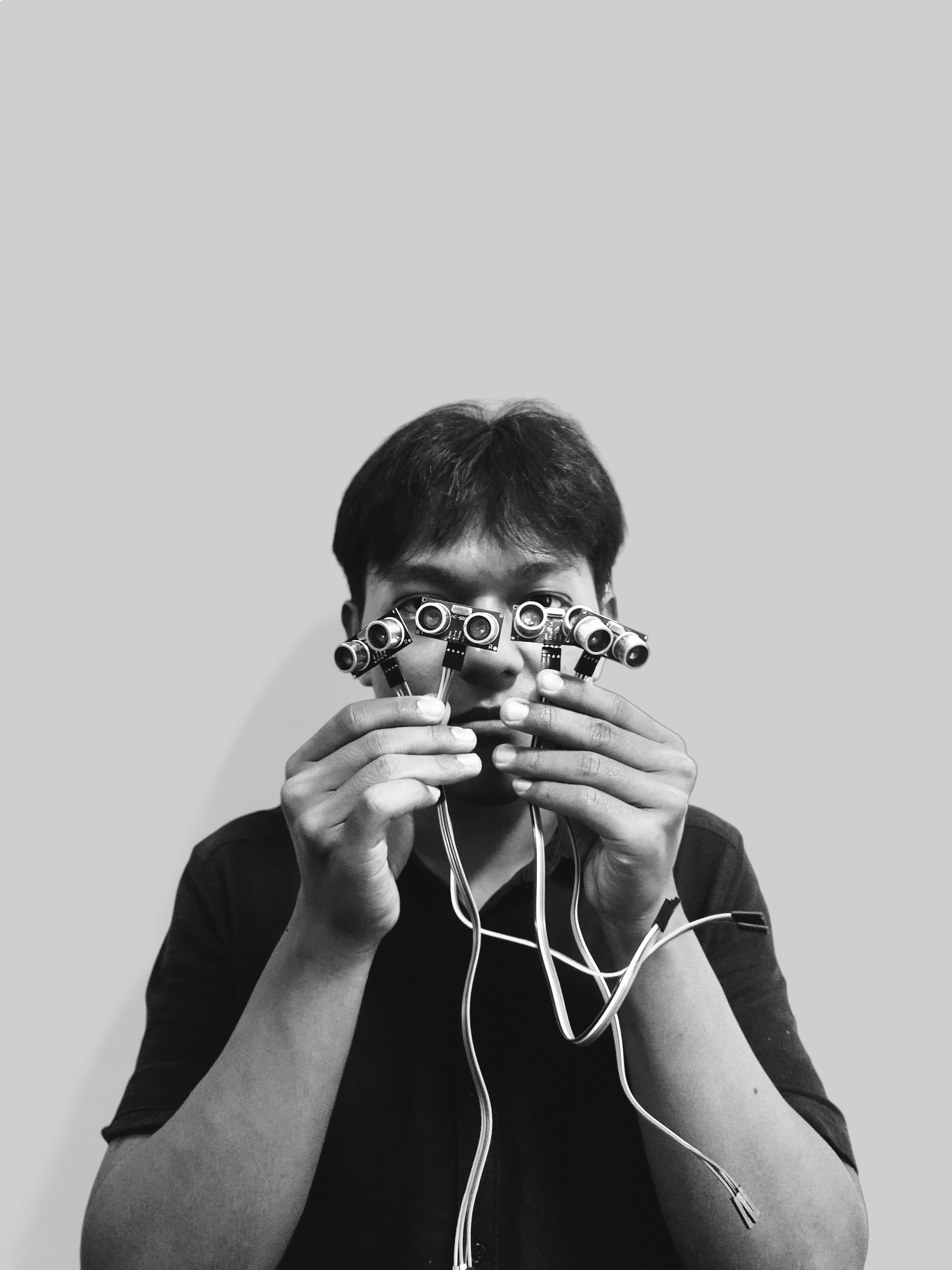
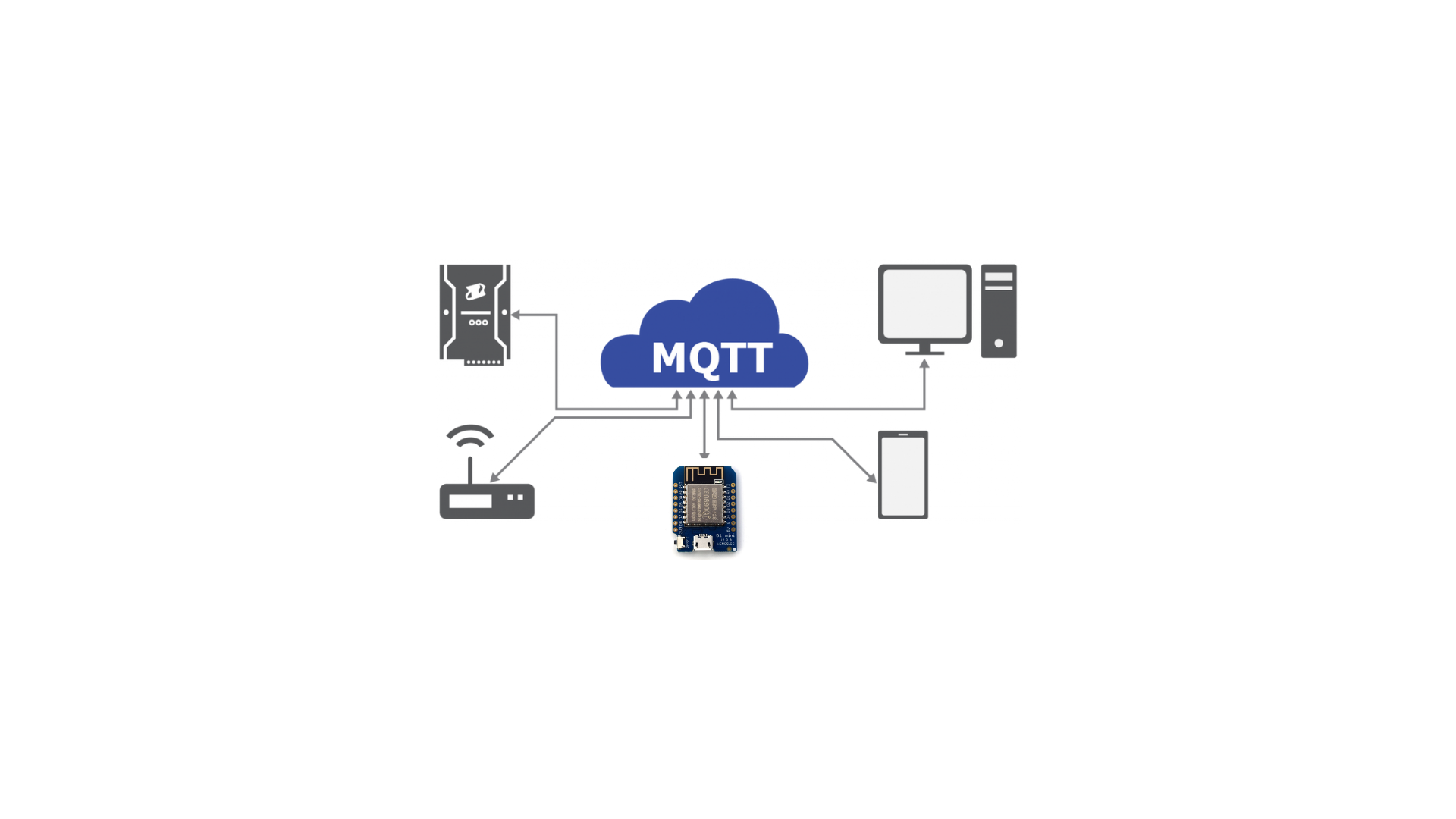
Introduction
The Wemos D1 Mini is a compact, Wi-Fi-enabled development board based on the ESP8266 chip, making it a popular choice for IoT projects. This guide demonstrates how to use the Wemos D1 Mini to both publish and subscribe to data using the MQTT protocol. By following this guide, you will learn how to set up the Wemos D1 Mini to collect data from sensors, publish that data to an MQTT broker, and subscribe to a topic to receive and act upon incoming messages.
Overview of Wemos D1 Mini
The Wemos D1 Mini is a small development board that offers built-in Wi-Fi capabilities, making it ideal for Internet of Things (IoT) projects. It is based on the ESP8266 chip, which provides the power and versatility needed for various applications, including data acquisition, remote monitoring, and control systems.
Key features include:
Wi-Fi Connectivity: Connect to wireless networks for remote data transmission and control.
Compact Size: Ideal for projects with space constraints.
Arduino IDE Compatibility: Program the Wemos D1 Mini using the popular Arduino IDE, which is beginner-friendly and widely supported.
Breadboard-Friendly: Easy integration with breadboards for prototyping.
Explanation of MQTT Protocol
MQTT (Message Queuing Telemetry Transport) is a lightweight messaging protocol designed for small sensors and mobile devices in low-bandwidth, high-latency, or unreliable networks. MQTT follows a publish/subscribe model, where clients publish messages to topics, and other clients subscribe to those topics to receive the messages.
Key concepts include:
Broker: The server that manages all the communication between clients.
Topics: Named channels through which messages are sent.
Quality of Service (QoS): Levels that define the guarantee of message delivery.
Importance of Data Publishing and Subscribing
In MQTT, data is exchanged between devices using the publish/subscribe model. A device can publish data to a specific topic, and any device subscribed to that topic will receive the data. This method allows for real-time communication and decouples the data producers from consumers, enhancing scalability and efficiency in IoT systems.
Hardware and Software Requirements
Necessary Components
Wemos D1 Mini: The main microcontroller board used for both publishing and subscribing to data.
Sensors and Actuators: Sensors (e.g., temperature, humidity) for data collection and actuators (e.g., LEDs, relays) for responding to received data.
Software Tools
Arduino IDE: The primary development environment for programming the Wemos D1 Mini.
MQTT Broker (e.g., Mosquitto): The server that handles the routing of messages between MQTT clients.
MQTT Client Tools: Tools like MQTT.fx or MQTT Explorer for testing and monitoring the MQTT communication.
Setting Up the Development Environment
Installing Arduino IDE
Download and Install: Obtain the Arduino IDE from the official Arduino website.
Configure for ESP8266: Add support for the ESP8266 by including the appropriate board manager URL and installing the ESP8266 package via the Boards Manager.
Adding Required Libraries
ESP8266WiFi: This library allows the Wemos D1 Mini to connect to Wi-Fi networks.
PubSubClient: A library for MQTT communication, enabling the Wemos D1 Mini to publish and subscribe to MQTT topics.
Install these libraries using the Arduino IDE’s Library Manager.
Configuring the MQTT Broker
To enable MQTT communication, set up an MQTT broker like Mosquitto:
Install Mosquitto: Follow installation instructions for your operating system.
Configure Broker: Customize the broker configuration as needed, including security settings, topic management, and user authentication.
Start the Broker: Ensure that the Mosquitto service is running and accessible.
Basic Concepts of MQTT Protocol
MQTT Architecture
In MQTT, communication is managed by a central broker that connects multiple clients. Clients can publish messages to topics, and the broker ensures that these messages are delivered to all clients subscribed to the corresponding topics.
Clients
In this project, the Wemos D1 Mini acts as an MQTT client that can both publish data to and subscribe to topics managed by the MQTT broker.
Broker
The MQTT broker is the intermediary that handles all message routing between clients. It ensures that messages published to a topic are delivered to all subscribed clients.
Topics and Messages
Messages in MQTT are organized into topics. Devices publish messages to topics, and other devices can subscribe to these topics to receive the messages. Topics are hierarchical and can be structured to organize data efficiently.
Quality of Service (QoS) Levels
MQTT provides three QoS levels:
QoS 0: At most once delivery, where the message is delivered once with no confirmation.
QoS 1: At least once delivery, where the message is guaranteed to be delivered at least once but may be delivered multiple times.
QoS 2: Exactly once delivery, ensuring the message is delivered only once and with confirmation from the receiver.
Programming the Wemos D1 for Data Publishing and Subscribing
Connecting Sensors and Actuators to Wemos D1
First, connect your sensors and actuators to the Wemos D1 Mini:
Sensors: Connect sensors to the appropriate GPIO pins. For instance, a temperature sensor could be connected to a digital pin like D1.
Actuators: Connect actuators (e.g., an LED) to other GPIO pins to allow the Wemos D1 Mini to act on received data.
Writing Sketch for Data Collection
Create an Arduino sketch that reads data from the connected sensor:
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* mqtt_server = "your_MQTT_SERVER";
WiFiClient espClient;
PubSubClient client(espClient);
const int sensorPin = A0; // Analog sensor connected to pin A0
const int actuatorPin = D1; // Actuator connected to pin D1
void setup() {
pinMode(actuatorPin, OUTPUT);
Serial.begin(115200);
setup_wifi();
client.setServer(mqtt_server, 1883);
client.setCallback(callback);
reconnect();
}
void setup_wifi() {
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("WiFi connected");
}
void reconnect() {
while (!client.connected()) {
if (client.connect("WemosClient")) {
client.subscribe("topic/sub"); // Subscribe to the topic for receiving data
} else {
delay(5000);
}
}
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
int sensorValue = analogRead(sensorPin);
String payload = "Sensor value: " + String(sensorValue);
client.publish("topic/pub", payload.c_str()); // Publish sensor data to the topic
delay(5000); // Delay between readings and publishing
}
This sketch reads data from a sensor connected to A0
, publishes the data to an MQTT topic, and subscribes to another topic to receive commands.
Implementing MQTT Callback for Subscribing
The callback function processes incoming messages from the subscribed topic and can trigger an actuator:
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print("Message arrived [");
Serial.print(topic);
Serial.print("]: ");
String message;
for (int i = 0; i < length; i++) {
message += (char)payload[i];
}
Serial.println(message);
// Example: Act on incoming data (e.g., turn on/off an LED)
if (message == "ON") {
digitalWrite(actuatorPin, HIGH); // Turn on actuator
} else if (message == "OFF") {
digitalWrite(actuatorPin, LOW); // Turn off actuator
}
}
This callback function listens for messages on the subscribed topic, and if it receives "ON," it turns on an actuator (e.g., an LED), and if it receives "OFF," it turns the actuator off.
Testing and Debugging
Initial Tests for Connectivity
Verify that the Wemos D1 Mini can connect to your Wi-Fi network and the MQTT broker. Use the Serial Monitor to check for successful connections.
Verifying Data Publishing and Subscribing
Use an MQTT client tool like MQTT.fx or MQTT Explorer to:
Monitor the Published Data: Subscribe to the topic where the Wemos D1 Mini publishes sensor data and verify the messages.
Test Subscribing: Send commands (e.g., "ON" or "OFF") to the topic the Wemos D1 Mini is subscribed to and observe the behavior of the connected actuator.
Common Issues and Troubleshooting
Wi-Fi Connection Issues: Ensure the correct SSID and password are used, and that the Wi-Fi signal is strong.
MQTT Connection Issues: Verify the broker's address, port, and ensure that the broker is running and accessible.
Data Not Published/Subscribed: Check the topic names and ensure that both publishing and subscribing are configured correctly in the code.
Conclusion
Summary of Steps
This guide covered how to use the Wemos D1 Mini to both publish and subscribe to data using the MQTT protocol. We started by setting up the development environment, connecting sensors and actuators, programming the Wemos D1 Mini, and then testing the entire setup.
Benefits of Using MQTT with Wemos D1 Mini
Real-Time Data Exchange: Immediate and reliable communication between devices.
Scalability: Easily add more devices without significant changes to the system.
Efficiency: Lightweight and efficient communication, making it ideal for IoT projects.
Future Enhancements and Projects
Possible extensions of this project include:
Security Enhancements: Implement SSL/TLS for secure communication over MQTT.
Advanced Automation: Use more complex logic to automate actions based on incoming data.
Cloud Integration: Send data to cloud platforms for analysis, storage, or integration with other services.
These improvements can help you create more advanced, secure, and feature-rich IoT solutions.
Subscribe to my newsletter
Read articles from M Ishlah Buana Angkasa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
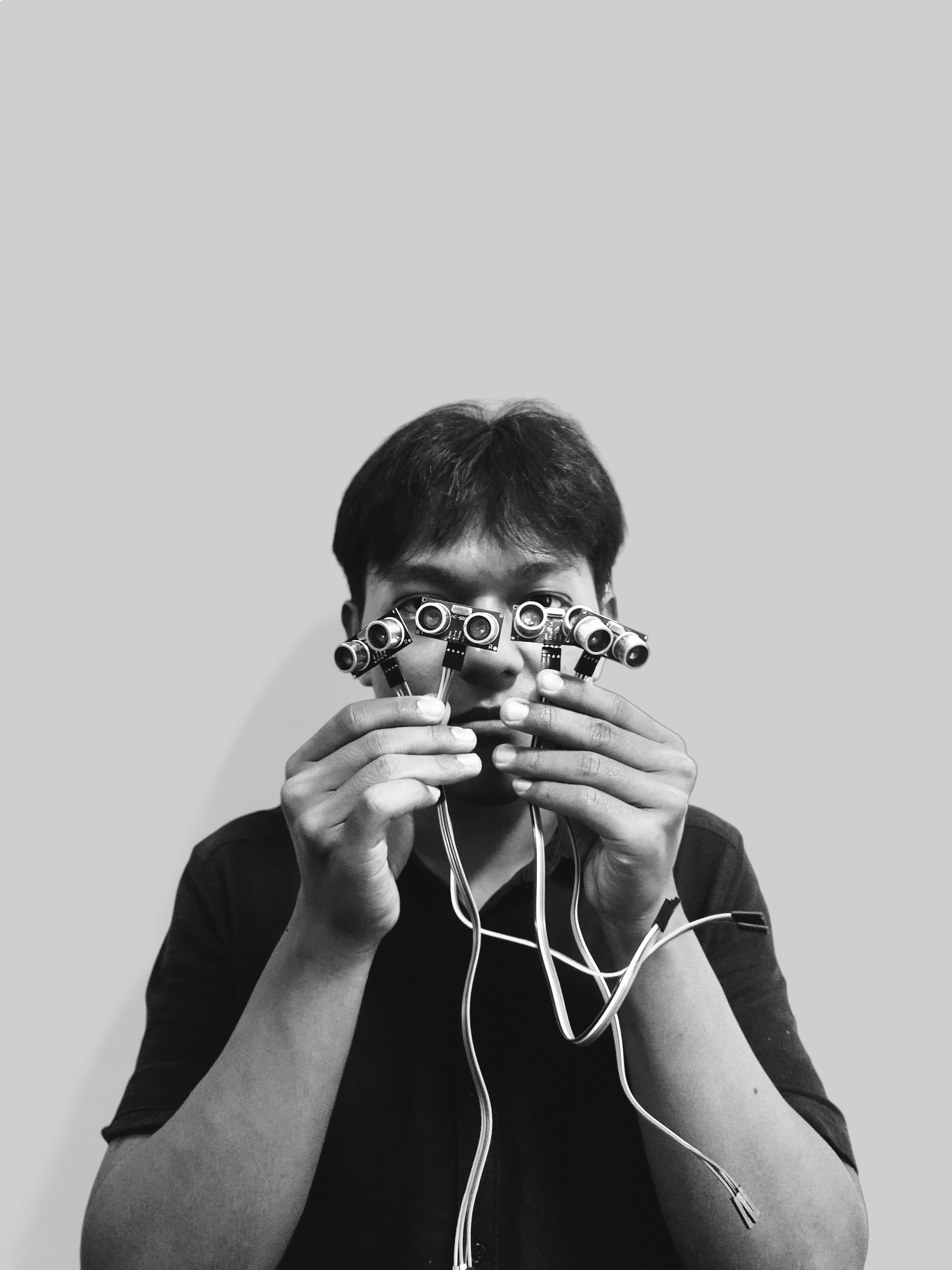
M Ishlah Buana Angkasa
M Ishlah Buana Angkasa
IoT Enthusiast