Flow Terminal Operators
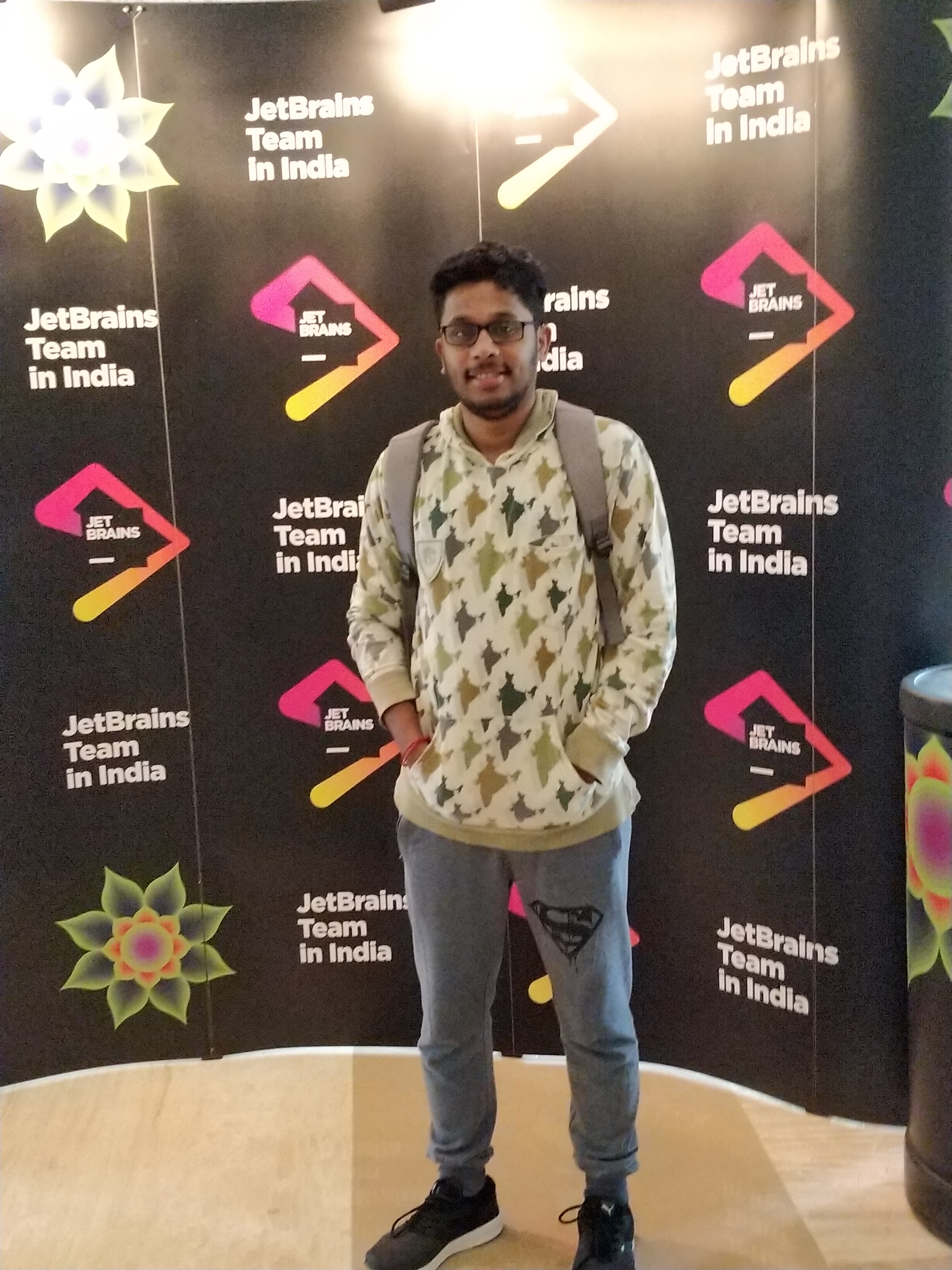
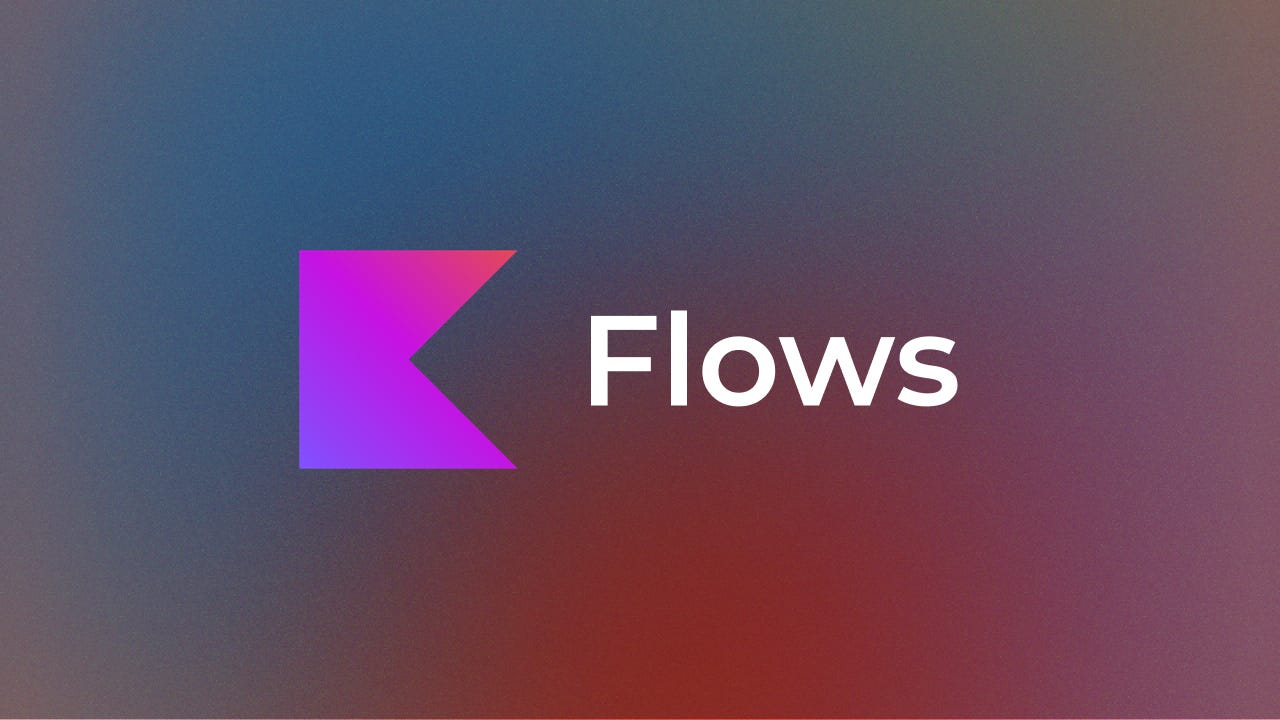
In this series of articles , we are discussing about Kotlin flows and in this article we will discuss about Flows Terminal operators
Code gist can be found in https://gist.github.com/vprabhu/20bf26e73451ae9ea0876e3780537d4a
Whats is Terminal Operators?
Its a suspend function
Starts the flow's collection
Terminal Operators List
There are some terminal operators which we can used to start the collection of the flow
Collect()
collects the given flow
commonly used with onEach() , onCompletion() and catch to collect all emitted values
val flow = flow { delay(200) emit(1) delay(200) emit(2) delay(200) emit(2) } flow.collect { receivedValue -> println("Collect Received value 1: $receivedValue") /** Output : * Collect Received value 1: 1 * Collect Received value 1: 2 * Collect Received value 1: 2 */ }
first() and last()
first() returns the first element emitted by flow and cancels the flow's cancellation.
last() returns the last element emitted by the flow.
val itemFirst = flow.first()
println("first() flow : $itemFirst")
/** Output :
* first() flow : 1
*/
val itemLast = flow.last()
println("last() flow : $itemLast")
/**
* last() flow : 2
*/
single()
Collects the only and one value from flow
If flow emits multiple values , it throws the following error
Exception in thread "main" java.lang.IllegalArgumentException: Flow has more than one element
val singleFlow = flow {
emit("Single Emitted value")
}
val itemSingle = singleFlow.single()
println("single() flow : $itemSingle")
/**
* output : single() flow : Single Emitted value
*/
toSet()
- converts the flow output to set
val setFLow = flow.toSet()
println("toSet() flow : $setFLow")
/**
* Output : toSet() flow : [1, 2]
*/
toList()
- converts the flow to list
val listFLow = flow.toList()
println("toList() flow : $listFLow")
/**
* toList() flow : [1, 2, 2]
*/
In the next article , we will discuss about Exception Handling in Flow.
Please leave your comments to improve.
Happy and Enjoy coding
Subscribe to my newsletter
Read articles from Vignesh Prabhu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
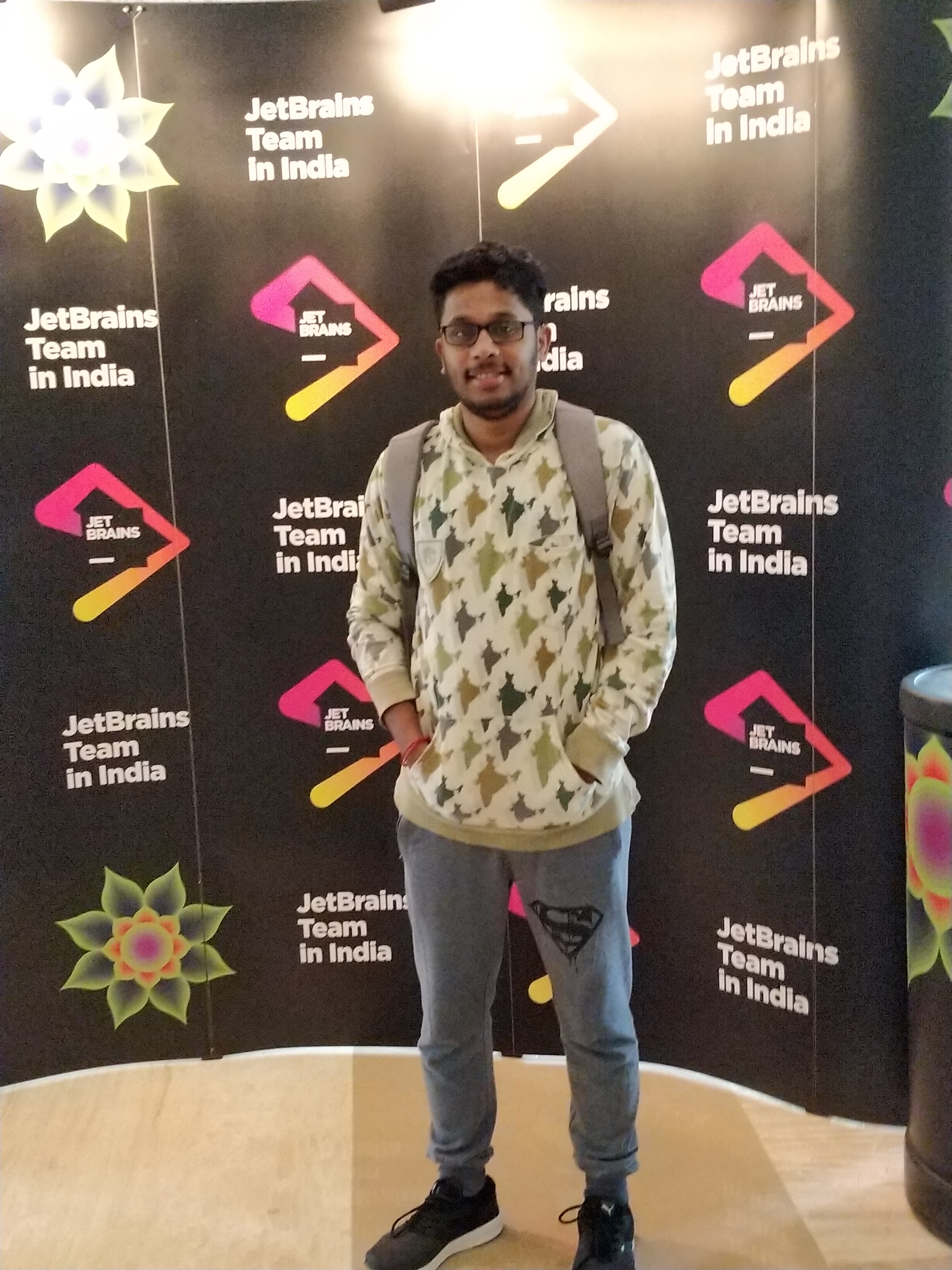
Vignesh Prabhu
Vignesh Prabhu
I am an Android application developer who is looking for new challenges to solve , love to learn and implement new things in coding