What is the Membership Operator in Python? A Simple Guide
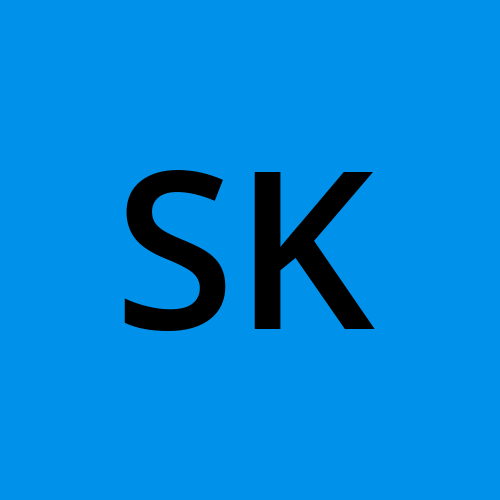
Membership operator "in"
ka primary purpose hai check karna ki ek value kisi sequence (like list, tuple, string, etc.) me present hai ya nahi. Yeh true ya false return karta hai agar aap ise direct if
condition ke saath use karte hain.
Lekin jab aap "in"
operator ko for
loop ke saath use karte ho, to wo operator sequence ke har element ke upar iterate karta hai. Iska matlab hai ki for
loop sequence me se ek ek element ko uthata hai aur loop ke body me use karta hai.
Agar aap sum calculate karne ke liye for
loop aur "in"
operator ka use kar rahe hain, to wo loop sequence ke har element ko add karega.
Example:
numbers = [1, 2, 3, 4, 5]
total_sum = 0
for num in numbers: # "in" operator is used to iterate over each element in the list
total_sum += num
print("Sum:", total_sum)
Explanation:
for num in numbers:
me"in"
operator listnumbers
ke har element konum
variable me assign karega sequentially.Loop ke har iteration me
total_sum
menum
ki value add hoti hai.Jab loop complete ho jata hai, to
total_sum
me list ke sabhi elements ka sum store hota hai.
Is code mein humne ek list of numbers ko iterate karke unka sum calculate kiya hai. Chaliye, step-by-step samajhte hain ki ye code kya kar raha hai:
Step 1: List Initialization
numbers = [1, 2, 3, 4, 5]
- Yeh line ek list banati hai jisme numbers
1, 2, 3, 4, 5
hain.
- Yeh line ek list banati hai jisme numbers
Step 2: Initializing the Sum
total_sum = 0
total_sum
ek variable hai jise 0 se initialize kiya gaya hai. Yeh variable humare final sum ko store karega.
Step 3: Iterating Over the List
for num in numbers:
- Yeh line ek
for
loop start karti hai jonumbers
list ke har element par iterate karega.num
har iteration mein list ke ek element ko represent karega.
- Yeh line ek
Step 4: Adding Each Number to the Sum
total_sum += num
Is line mein har
num
kototal_sum
mein add kiya jata hai.+=
operator ka matlab hai "add and assign", matlabtotal_sum = total_sum + num
.Example ke liye:
First iteration:
total_sum = 0 + 1
(total_sum ab 1 ho gaya)Second iteration:
total_sum = 1 + 2
(total_sum ab 3 ho gaya)Third iteration:
total_sum = 3 + 3
(total_sum ab 6 ho gaya)Fourth iteration:
total_sum = 6 + 4
(total_sum ab 10 ho gaya)Fifth iteration:
total_sum = 10 + 5
(total_sum ab 15 ho gaya)
Step 5: Printing the Result
print("Sum:", total_sum)
- Last mein,
print
statement use hota hai jo finaltotal_sum
ko print karta hai. Is example mein final sum15
print hoga.
- Last mein,
Final Output:
Sum: 1
Is code mein humne ek list of numbers ka sum calculate kiya hai by iterating over each element of the list and adding it to a running total (total_sum
). Last mein, final sum ko print kiya gaya hai.
Samajhne ke liye hum ek basic concept par focus karte hain:
List and Looping in Python:
numbers
List: Yeh listnumbers = [1, 2, 3, 4, 5]
hai, jisme multiple values (1, 2, 3, 4, 5) store hain.for
Loop: Jab humfor num in numbers:
likhte hain, to Python automaticallynumbers
list ke har element ko ek-ek karke loop ke andar laata hai, aur us element ko temporarilynum
variable mein store karta hai.
Kyon num
variable?
num
ek temporary variable hai, jo sirf current iteration ke liye list ke ek specific element ko hold karta hai.Kya hoga agar
numbers
ko hi use karein?: Agar aapnumbers
ko directly modify karenge, toh aap list ki original value ko change karenge, jo kai baar aap nahi karna chahenge.num
variable ke use se, aap list ke har element ko individually access kar paate hain, bina original list ko change kiye.
Example to Clarify:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print("Current number is:", num)
Is code mein:
num
sirf us particular element ko store karta hai jo us waqt loop ke andar process ho raha hota hai.numbers
list original form mein as it is rehti hai, jab kinum
variable har iteration mein ek naye element ko temporarily hold karta hai.
Why Not Directly numbers
?
Agar aap
numbers
list ko directly loop ke andar modify karenge, toh aap list ki integrity ko lose kar sakte hain.num
variable ka use karne se, list ke har element par sequentially kaam kiya ja sakta hai bina list ke baaki elements ko affect kiye.
num
variable ka use isliye hota hai kyunki yeh ek temporary holder ki tarah kaam karta hai, jo list ke har element ko ek-ek karke process karta hai. Aap original list ko modify karne se bachte hain, aur code readability aur safety barh jati hai.
Key Point:
Jab aap "in"
operator ko for
loop ke saath use karte hain, tab wo membership test ke liye nahi, balki sequence ke elements ke upar iterate karne ke liye use hota hai. Isliye wo true ya false return nahi karta, balki loop ko sequence ke elements ke saath iterate karne me help karta hai.
Membership operator "in"
ke basic use case me, agar aap directly condition check karenge, jaise:
if 3 in numbers:
print("3 is in the list")
To yeh true ya false return karega based on condition. Lekin for
loop me yeh sequence ko iterate karne ke kaam aata hai, isliye usme true/false evaluate nahi hota.
Membership operators Python me do types ke hote hain: in
aur not in
. Ye operators yeh check karte hain ki ek element kisi sequence (jaise list, tuple, string, set, etc.) me exist karta hai ya nahi.
Features of Membership Operators:
True/False Evaluation:
in
operator yeh check karta hai ki kya element specified sequence me hai ya nahi. Agar element sequence me hota hai, to yehTrue
return karta hai, warnaFalse
.not in
operator iska ulta kaam karta hai. Agar element sequence me nahi hota, to yehTrue
return karta hai, warnaFalse
.
Versatility:
- Membership operators ko aap different data structures me use kar sakte hain, jaise lists, tuples, strings, sets, and dictionaries. In dictionaries, membership operators keys ke upar operate karte hain, values ke upar nahi.
Iterative Checking:
- Jab membership operator ko
for
loop ke saath use kiya jata hai, to yeh element-wise iteration karta hai, aur loop ko sequence ke saath iterate karne me help karta hai. Is case me, operator directly True/False return nahi karta, balki loop me elements iterate karne ka kaam karta hai.
- Jab membership operator ko
Efficiency:
- Python me membership operators optimized hain. For example, strings me substring check karna ya sets me membership test karna typically fast hota hai.
Use in Conditions:
- Membership operators commonly
if
statements me use hote hain jaha pe specific conditions check karni hoti hain.
- Membership operators commonly
Architecture of Membership Operators:
Python me membership operators ka implementation uske underlaying data structures pe depend karta hai. Alag-alag data structures membership test perform karne ke liye alag-alag algorithms use karte hain:
Lists and Tuples:
- Lists aur tuples me membership test ek linear search algorithm use karta hai. Iska matlab yeh hota hai ki list/tuple ke elements ko ek-ek karke check kiya jata hai, jab tak match nahi mil jata ya phir pura sequence nahi check ho jata. Iska time complexity O(n) hota hai, jaha
n
sequence ka size hai.
- Lists aur tuples me membership test ek linear search algorithm use karta hai. Iska matlab yeh hota hai ki list/tuple ke elements ko ek-ek karke check kiya jata hai, jab tak match nahi mil jata ya phir pura sequence nahi check ho jata. Iska time complexity O(n) hota hai, jaha
Sets and Dictionaries:
- Sets aur dictionaries me membership test hashing ka use karta hai, jo generally kaafi fast hota hai. Hashing ke wajah se in data structures me membership check ki time complexity average case me O(1) hoti hai.
Strings:
- Strings me membership test substring matching algorithms ka use karta hai. Jab string me substring search ki jati hai, to internally comparison hoti hai. Agar substring mil jati hai to
True
return hota hai, warnaFalse
.
- Strings me membership test substring matching algorithms ka use karta hai. Jab string me substring search ki jati hai, to internally comparison hoti hai. Agar substring mil jati hai to
Examples:
in
Operator:numbers = [1, 2, 3, 4, 5] print(3 in numbers) # True print(6 in numbers) # False
not in
Operator:fruits = ["apple", "banana", "mango"] print("grape" not in fruits) # True print("banana" not in fruits) # False
In a Dictionary:
my_dict = {'a': 1, 'b': 2, 'c': 3} print('a' in my_dict) # True, checks for keys print(2 in my_dict) # False, values are not checked
Conclusion:
Membership operators simple hain, lekin kaafi powerful tools hain sequence data structures ke saath kaam karne ke liye. Yeh operators Python ke core architecture me tightly integrated hain, jaha data structures ka underlying algorithmic implementation in operators ke performance ko decide karta hai.
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
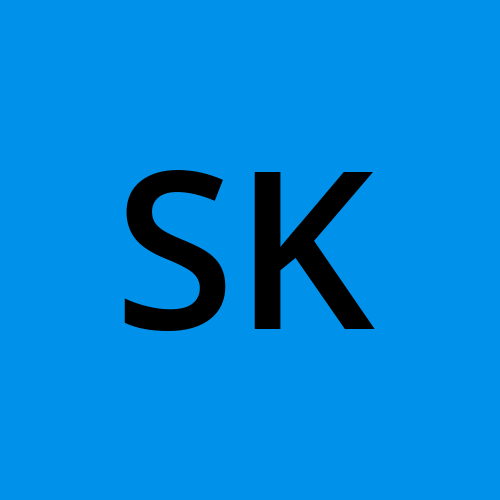
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.