Content Personalization and Recommendation Systems in Video Streaming Services

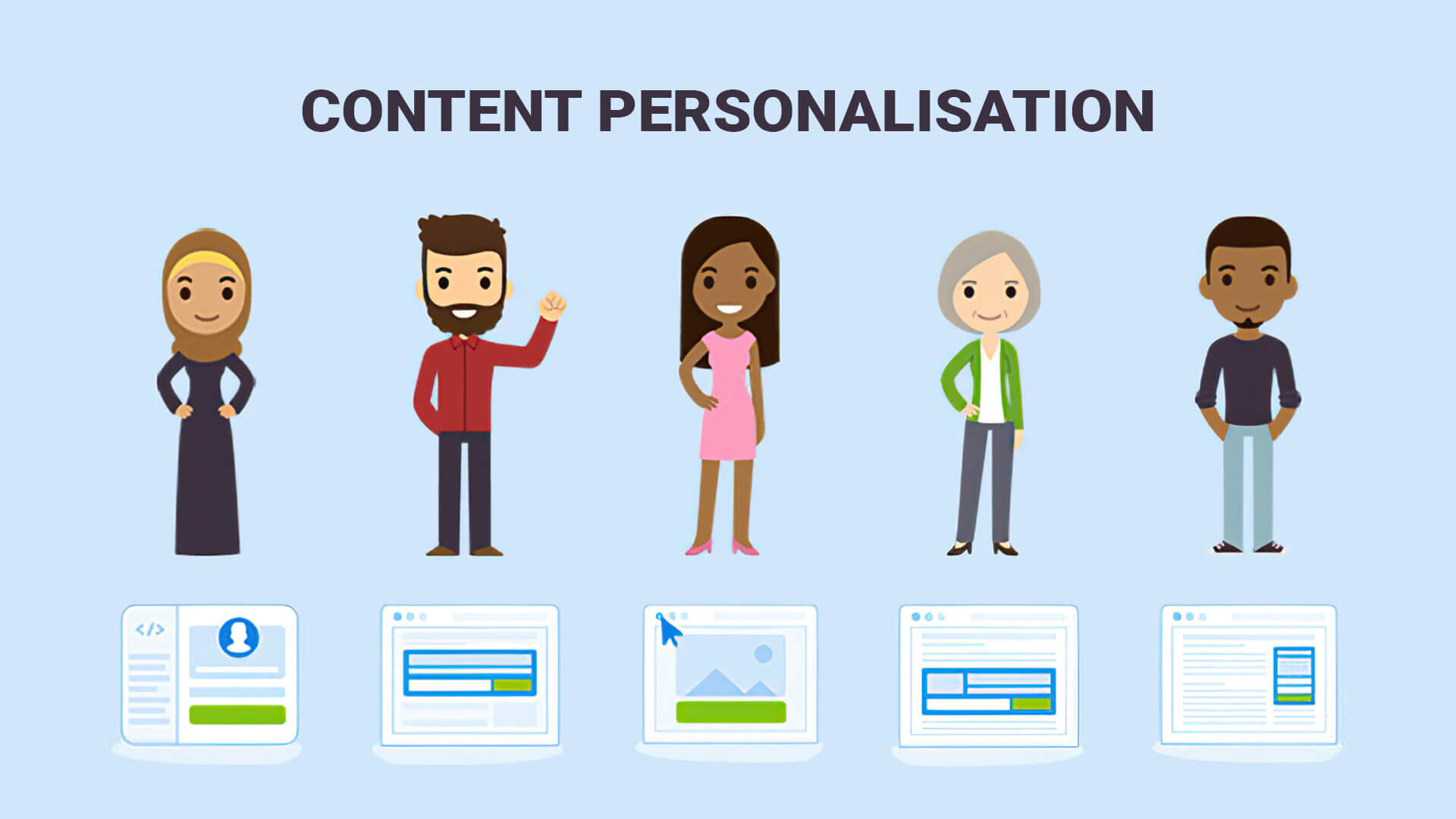
Introduction
In the world of video streaming, where content libraries often span millions of videos, providing a personalized experience to users is not just a luxury—it's a necessity. Content personalization and recommendation systems are at the heart of platforms like Netflix, YouTube, and Amazon Prime, helping to increase user engagement by suggesting relevant content tailored to individual preferences. This article will delve deep into the mechanisms behind content personalization and recommendation systems, exploring the flow of concepts, coding examples, and industry practices to give you a comprehensive understanding of how these systems work.
Understanding Content Personalization
Content personalization refers to the process of tailoring content to meet the unique preferences of individual users. The goal is to enhance the user experience by presenting them with content that is most relevant to their tastes, thereby increasing engagement and satisfaction.
1. User Profiling
The first step in content personalization is building a detailed profile for each user. This profile is constructed using:
Demographic Information: Age, gender, location, language, etc.
Behavioral Data: Watch history, search queries, likes/dislikes, and interaction patterns.
Explicit Feedback: Ratings, reviews, and user-provided preferences.
Example:
# Example of user profiling data in Python
user_profile = {
"user_id": 12345,
"demographics": {
"age": 30,
"gender": "male",
"location": "New York",
"language": "English"
},
"behavioral_data": {
"watch_history": ["movie_1", "movie_2", "series_1"],
"search_queries": ["comedy movies", "action movies"],
"likes": ["comedy", "thriller"],
"dislikes": ["romance"]
},
"explicit_feedback": {
"ratings": {"movie_1": 4, "movie_2": 5},
"preferences": ["high definition", "fast loading"]
}
}
2. Content Analysis
Once the user profile is established, the next step is to analyze the content available in the library. Each piece of content is tagged with various attributes, such as genre, director, cast, language, and keywords. This metadata is crucial for matching content with user preferences.
Example:
# Example of content metadata in Python
content_metadata = {
"movie_1": {
"title": "Action Movie 1",
"genre": ["Action", "Thriller"],
"cast": ["Actor A", "Actor B"],
"director": "Director X",
"language": "English",
"keywords": ["explosions", "chase", "hero"]
},
"series_1": {
"title": "Comedy Series 1",
"genre": ["Comedy"],
"cast": ["Comedian A", "Comedian B"],
"director": "Director Y",
"language": "English",
"keywords": ["humor", "stand-up", "family"]
}
}
3. Matching and Recommendation
With the user profile and content metadata in place, the next step is to match users with content that aligns with their preferences. This is where recommendation algorithms come into play.
Recommendation Systems
Recommendation systems are the engines that drive personalized content delivery. They analyze user profiles and content metadata to suggest the most relevant content to users. There are several types of recommendation systems, each with its unique approach:
1. Collaborative Filtering
Collaborative filtering is one of the most widely used techniques in recommendation systems. It works by finding patterns in user behavior and identifying similar users or items. There are two main types of collaborative filtering:
User-Based Collaborative Filtering: Recommends content based on what similar users have liked.
Item-Based Collaborative Filtering: Recommends content based on similar items that the user has liked.
Example:
# Example of item-based collaborative filtering in Python
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# User-item interaction matrix
user_item_matrix = np.array([
[5, 3, 0, 1],
[4, 0, 0, 1],
[1, 1, 0, 5],
[0, 0, 5, 4],
[0, 0, 4, 5]
])
# Compute cosine similarity between items
item_similarity = cosine_similarity(user_item_matrix.T)
# Predict ratings for a user
user_id = 0
user_ratings = user_item_matrix[user_id]
predicted_ratings = item_similarity.dot(user_ratings) / np.array([np.abs(item_similarity).sum(axis=1)])
2. Content-Based Filtering
Content-based filtering recommends content based on the features of the items that the user has interacted with. This approach leverages the metadata associated with content to find similar items.
Example:
# Example of content-based filtering in Python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import linear_kernel
# Content metadata
content = [
"Action movie with explosions and chase scenes",
"Comedy movie with humorous dialogues",
"Thriller movie with suspense and mystery",
"Romantic movie with love story",
"Comedy series with stand-up and humor"
]
# Vectorize the content
tfidf = TfidfVectorizer(stop_words='english')
tfidf_matrix = tfidf.fit_transform(content)
# Compute cosine similarity between content
cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix)
# Get recommendations for a specific item
item_idx = 0
similar_items = list(enumerate(cosine_sim[item_idx]))
similar_items = sorted(similar_items, key=lambda x: x[1], reverse=True)
3. Hybrid Models
Hybrid models combine collaborative filtering and content-based filtering to enhance the accuracy of recommendations. By leveraging the strengths of both approaches, hybrid models can provide more personalized recommendations.
Example:
# Example of a hybrid model in Python
from sklearn.decomposition import TruncatedSVD
# Combine user-item matrix with content-based features
user_content_matrix = np.hstack((user_item_matrix, tfidf_matrix.toarray()))
# Apply dimensionality reduction
svd = TruncatedSVD(n_components=50)
reduced_matrix = svd.fit_transform(user_content_matrix)
# Compute similarity between users and items
user_similarity = cosine_similarity(reduced_matrix)
item_similarity = cosine_similarity(reduced_matrix.T)
# Generate hybrid recommendations
user_id = 0
hybrid_recommendations = (user_similarity[user_id] + item_similarity.T).dot(user_item_matrix[user_id])
Industry Practices and Tools
In the industry, recommendation systems are often implemented using a combination of machine learning algorithms, big data tools, and cloud infrastructure. Here are some of the tools and techniques commonly used:
1. Machine Learning Frameworks
TensorFlow and PyTorch: Popular frameworks for building and training recommendation models, including deep learning approaches.
Scikit-Learn: A versatile library for implementing traditional machine learning algorithms like collaborative filtering and content-based filtering.
2. Big Data Processing
Apache Spark: Used for processing large-scale user interaction data and building recommendation models in distributed environments.
Hadoop: A framework for distributed storage and processing of large datasets, often used in conjunction with Spark.
3. Cloud Infrastructure
AWS SageMaker: A cloud-based platform for building, training, and deploying machine learning models at scale.
Google AI Platform: Provides tools for building and deploying ML models, including recommendation systems.
4. Real-Time Personalization
Kafka: A distributed streaming platform used for real-time data processing, allowing recommendation systems to update in real-time as new user data comes in.
Redis: An in-memory data store used for caching user profiles and recommendation results, enabling fast retrieval.
Flow of Concepts in Coding
To build a recommendation system for a video streaming service, here’s a conceptual flow that outlines the coding and processes involved:
Data Collection: Collect user interaction data (watch history, ratings, search queries) and content metadata (genre, cast, keywords).
# Collect data from user interactions and content library user_data = fetch_user_interactions() content_data = fetch_content_metadata()
Data Preprocessing: Clean and preprocess the data, including handling missing values, normalizing ratings, and extracting features from content.
# Preprocess data user_data = preprocess_user_data(user_data) content_data = preprocess_content_data(content_data)
Model Training: Choose a recommendation model (e.g., collaborative filtering, content-based filtering, hybrid) and train it on the preprocessed data.
# Train recommendation model recommendation_model = train_model(user_data, content_data)
Prediction and Recommendation: Use the trained model to predict user preferences and generate content recommendations.
# Generate recommendations recommendations = generate_recommendations(recommendation_model, user_id)
Evaluation and Optimization: Evaluate the performance of the recommendation system using metrics like precision, recall, and user satisfaction. Optimize the model by fine-tuning hyperparameters or incorporating additional features.
# Evaluate and optimize model evaluate_model(recommendation_model) optimize_model(recommendation_model)
Deployment: Deploy the recommendation system in a production environment, integrating it with the video streaming platform.
# Deploy
Subscribe to my newsletter
Read articles from ritiksharmaaa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ritiksharmaaa
ritiksharmaaa
Hy this is me Ritik sharma . i am software developer