Lists and Tuples in Python

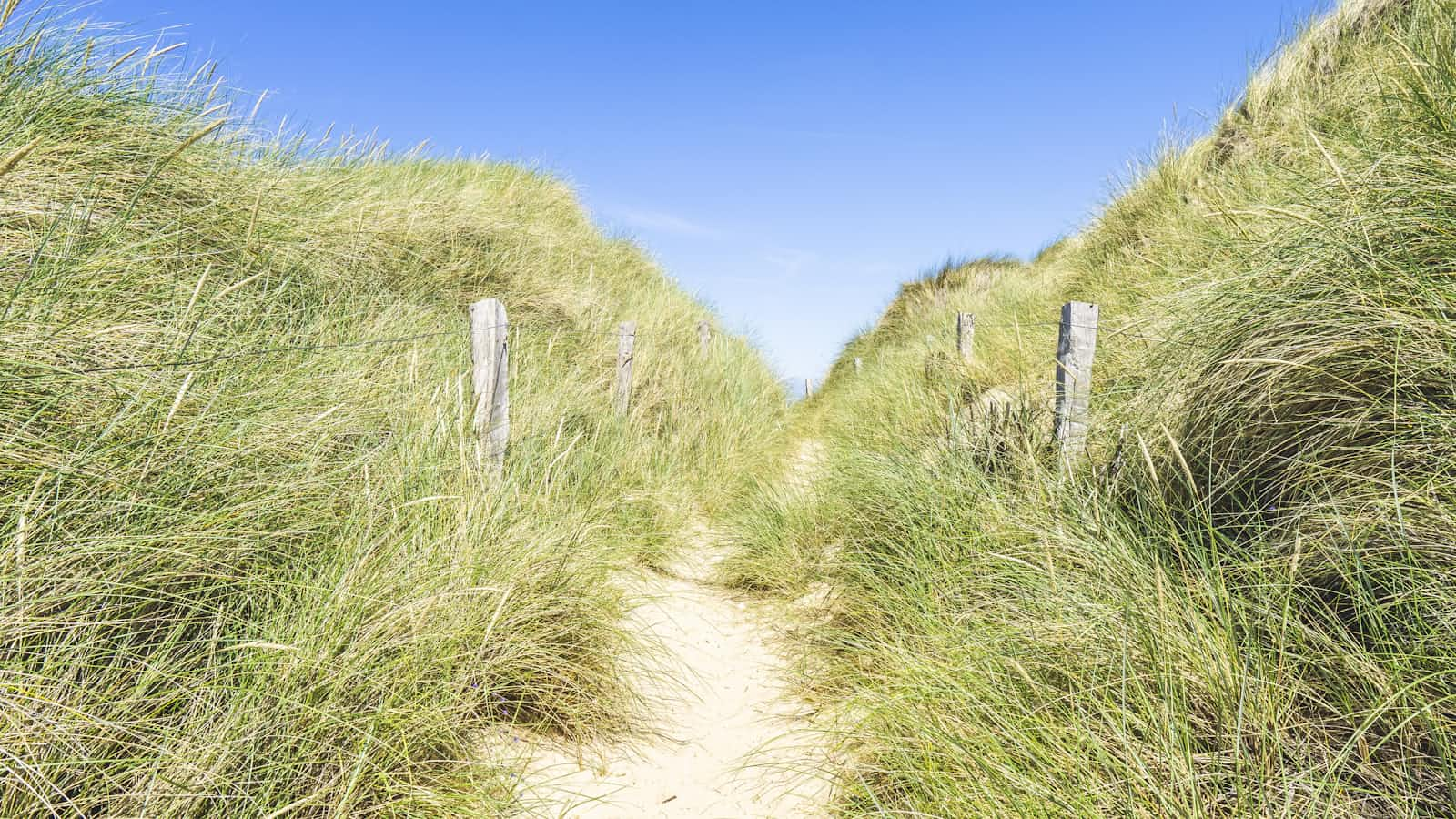
Imagine you have a bunch of numbers and you assign a variable to each of these numbers. Now everytime you want to access these set of numbers you need to remember the variable name for each. This is a time consuming and tedious task right. This has been solved in python by using Lists and Tuples. These are sort of like arrays in C, which you can learn about in my previous blogs, now lets concentrate on Lists and Tuples.
Lists-A built in datatype that stores set of values. It can storeelements of different types. This is similar to array. But this can store elements of different datatype. Slicing is possable.
Syntax-name=[name,age,number]
print(type(marks)) # This returns the type of data in a list.
print(name[1])
name[1:4] #to slice
List methods
list=[2,1,3]
list.append(4) #adds one element at the end
list = [2, 1, 3,4]
list.insert(1,5) #insert element at index
list.sort( ) #sorts in ascending order[1,2,3]
list.reverse() #reverses list
list.sort( reverse=True ) #sorts in descending order[3,2,1]
list.remove(1) #removes first occurrence of element
list = [2, 1, 3, 1]
list.pop(3) #removes element at idx
[2, 3, 1]
#There are many more list functions which can be explored.
Tuples-A built in data type that lets us create immutable sequences of values If you want to store a single data put a comma at the last. Then the compiler reads it as a tuple. tuples can slice.
#Syntax
Name=(e1,e2,e3)
tup=(12,34,56)
tup.index( el ) #returns index of first occurrence
tup = (2, 1, 3, 1)
tup.count( element ) #counts total occurrences tup.count(1) is 2 tup.index(1) is 1
Q1 Program to ask the user his favourite movies and store them in a list.
n=int(input("Enter the number of movies you like:"))
m=[]
for i in range(n):
movie=input("Enter the name of the movie:")
m.append(movie)
print(m)
Q2 Program to check if the list is a palandrome or not.
l=[1,1,1,1]
m=l.copy()
l.reverse()
if m==l:
print("Yes")
else:
print("NO")
Q3 Program to find the number of students who got an A in a list.
tup=("A","B","C","D","C","B","A")
a=tup.count("A")
print(a)
Q4 Program to store above grade in a list and sort them.
tup=["A","B","C","D","C","B","A"]
tup.sort()
print(tup)
Hope you learnt something in this blog. This was just an introduction to the topic. Feel free to explore. Dictionaries are similar to tuples and lists but they are an unordered collection of key-value pairs. Don't forget to like and follow for more coding related blogs.
Subscribe to my newsletter
Read articles from Gagan G Saralaya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
