How to Build a Multi-Agent System Using AutoGen
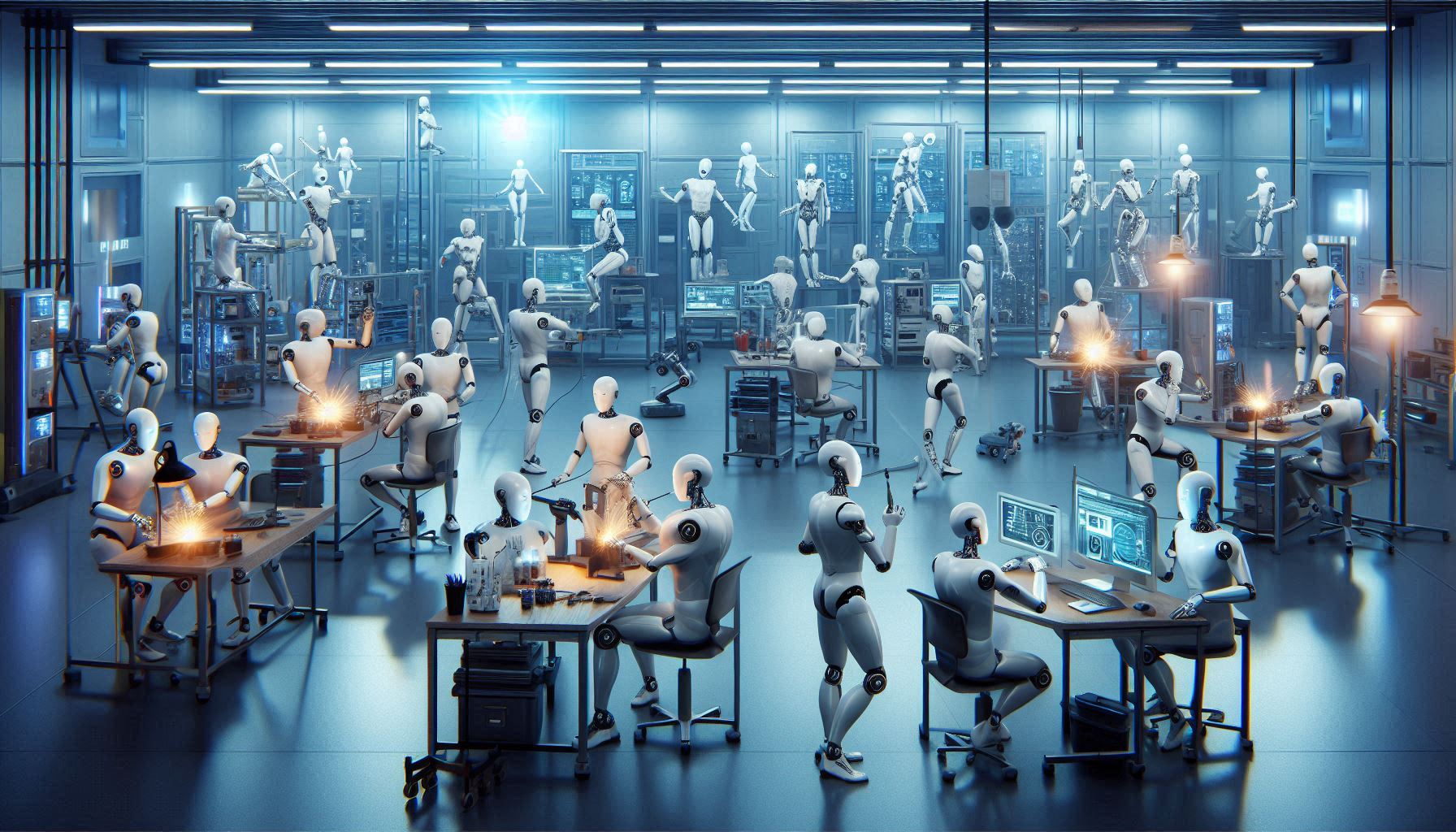
Introduction
In this guide, I'll walk through using Multi-Agent Systems (MAS) with AutoGen to build a simple application with the collaboration of multiple agents. This example will help you understand how to set up different agents for specific tasks, communicate between them, and execute code dynamically. In this example, we will build a simple landing page, but you can create anything by adjusting your prompts.
Explanation of Multiagent Systems
Multi-Agent Systems (MAS) involve multiple interacting agents that work together to solve complex problems. Each agent has its own goals and abilities, and they collaborate through communication and coordination. MAS are used in various fields to handle tasks that are too challenging for a single agent, such as in robotics, traffic management, and AI applications.
AutoGen
According to the AutoGen documentation, AutoGen is an open-source library from Microsoft designed for creating AI agents and facilitating their collaboration on various tasks. Much like PyTorch simplifies deep learning, AutoGen aims to streamline and enhance the development and research of agent-based AI systems. It offers a user-friendly and adaptable environment that supports conversational agents, integrates with LLMs and tools, accommodates both autonomous and human-in-the-loop workflows, and facilitates complex multi-agent interactions.
Setup
Libraries
pip install pyautogen python-dotenv
Importing required libraries
import os
import tempfile
from autogen import ConversableAgent, GroupChat, GroupChatManager
from autogen.coding import LocalCommandLineCodeExecutor
from dotenv import load_dotenv
Set up an .env
file in the same directory and add your OpenAI API in there
OPENAI_API_KEY="your-api-key-here"
Use python-dotenv
to load environment variables from a .env
file:
load_dotenv()
Create a Directory for Code Files
code_files_dir = 'code_files'
os.makedirs(code_files_dir, exist_ok=True)
Initialize the Code Executor
Set up a local command line code executor with a specified timeout and working directory:
executor = LocalCommandLineCodeExecutor(
timeout=10, # Timeout for each code execution in seconds.
work_dir=code_files_dir, # Directory to store the code files.
)
Define Agents
Code Executor Agent
This agent executes code files:
code_executor_agent = ConversableAgent(
name="Code_Executor_Agent",
llm_config=False, # Turn off LLM for this agent.
code_execution_config={"executor": executor}, # Use the local command line code executor.
human_input_mode="NEVER", # This lets it run fully by itself, you can set ALWAYS to control what the agents are coding
)
Product Manager Agent
Manages the project and coordinates between agents:
product_manager = ConversableAgent(
name="Product_Manager",
system_message="""As the Product Manager, your role is to oversee the development of the App. Coordinate
between all the agents. You are responsible for making
sure that the app is functional, user-friendly, and aligned with our goals.""",
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
human_input_mode="NEVER",
)
Coder Agent
Implements core logic and writes code:
coder = ConversableAgent(
name="Coder",
system_message="""As the Coder, your job is to implement the core logic of the app. You will write code
that follows the instructions of the product manager, save all files, and one final execution file so the human can afterwards check
if it all works fine. Ensure the code is efficient,
well-documented, and easy to maintain. Your code will be executed by the code executor agent""",
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
human_input_mode="NEVER",
)
Set Up the Group Chat
Create a group chat involving all agents:
# Create a GroupChat instance to manage interactions among the agents.
group_chat = GroupChat(
# List of agents participating in the group chat.
agents=[product_manager, coder, code_executor_agent],
# Initialize with an empty list of messages; messages will be added dynamically.
messages=[],
# Set the maximum number of rounds for the conversation.
max_round=12,
# Option to automatically send introductory messages from each agent.
send_introductions=True,
# Method for selecting the speaker for each round; set to "auto" to let the system decide.
speaker_selection_method="auto"
)
Initialize the Group Chat Manager
Manage the group chat and initiate communication:
group_chat_manager = GroupChatManager(
groupchat=group_chat,
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
)
Start the Chat
Begin the chat with an initial message outlining the task:
chat_result = group_chat_manager.initiate_chat(
group_chat_manager,
message="""Let's start building a simple landing page for our new product with HTML and CSS and run it in a Python file.
Create three files that can be run and tested from the Python file.""",
summary_method="reflection_with_llm"
)
Now execute the code and watch as your agents interact with each other to build the app. They will store the files in the code_files folder. You have to rename them according to what the agents instructed and run them. Here is an example landing page they built with the above code.
Conclusion
This guide demonstrates how to set up and use Multi-Agent Systems with AutoGen to manage a collaborative project involving multiple agents. By following these steps, you can effectively coordinate tasks, manage code execution, and ensure smooth collaboration among agents. Feel free to adapt and expand upon this framework based on your specific project needs.
You can also check the official docs to add custom functions to specific agents and allow them to only choose from these functions. This way you can automate web scraping and other tasks fully. Happy to hear your applications in the comments!
Happy coding!
I'm Jonas, and you can connect with me on LinkedIn or follow me on Twitter @jonasjeetah.
Full Code
import os
import tempfile
from autogen import ConversableAgent, GroupChat, GroupChatManager
from autogen.coding import LocalCommandLineCodeExecutor
from dotenv import load_dotenv
load_dotenv()
# Define the directory to store the code files.
code_files_dir = 'code_files'
# Ensure the directory exists.
os.makedirs(code_files_dir, exist_ok=True)
# Create a local command line code executor.
executor = LocalCommandLineCodeExecutor(
timeout=10, # Timeout for each code execution in seconds.
work_dir=code_files_dir, # Use the specified directory to store the code files.
)
# Create an agent with code executor configuration.
code_executor_agent = ConversableAgent(
name="Code_Executor_Agent",
llm_config=False, # Turn off LLM for this agent.
code_execution_config={"executor": executor}, # Use the local command line code executor.
human_input_mode="NEVER", # Always take human input for this agent for safety.
)
# Create a Product Manager agent.
product_manager = ConversableAgent(
name="Product_Manager",
system_message="""As the Product Manager, your role is to oversee the development of the App. Coordinate
between all the agents, You are responsible for making
sure that the app is functional, user-friendly, and aligned with our goals.""",
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
human_input_mode="NEVER",
)
# Create a Coder agent.
coder = ConversableAgent(
name="Coder",
system_message="""As the Coder, your job is to implement the core logic of the app. You will write code
that follows the instructions of the product manager, save all files and one final execution file so the human can afterwards check
if it all works fine. Ensure the code is efficient,
well-documented, and easy to maintain. Your code will be executed by the code executor agent""",
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
human_input_mode="NEVER",
)
# Create the group chat.
group_chat = GroupChat(
agents=[product_manager, coder, code_executor_agent],
messages=[],
max_round=12,
send_introductions=True,
speaker_selection_method="auto"
)
# Create a GroupChatManager to manage the group chat.
group_chat_manager = GroupChatManager(
groupchat=group_chat,
llm_config={"config_list": [{"model": "gpt-4o-mini", "api_key": os.environ["OPENAI_API_KEY"]}]},
)
# Initiate the chat and include an initial message.
chat_result = group_chat_manager.initiate_chat(
group_chat_manager,
message="""Let's start building a simple landing page for our new product with html and css and run it in a python file.
So create three files please that can be run and tested from the python file.""",
summary_method="reflection_with_llm"
)
Subscribe to my newsletter
Read articles from Jonas Gebhardt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Jonas Gebhardt
Jonas Gebhardt
๐ Hello! I'm Jonas, a passionate Data Scientist from Berlin, Germany. At 31 years I decided to quit my career in Supply Chain to get into coding and start building awesome products. Come follow me on my journey.