๐ Continuous Integration and Deployment (CI/CD) with GitHub Actions: A Comprehensive Guide ๐ฏ
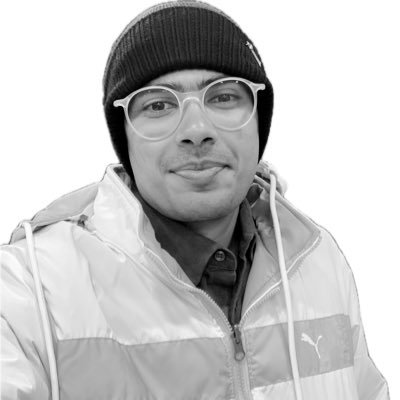
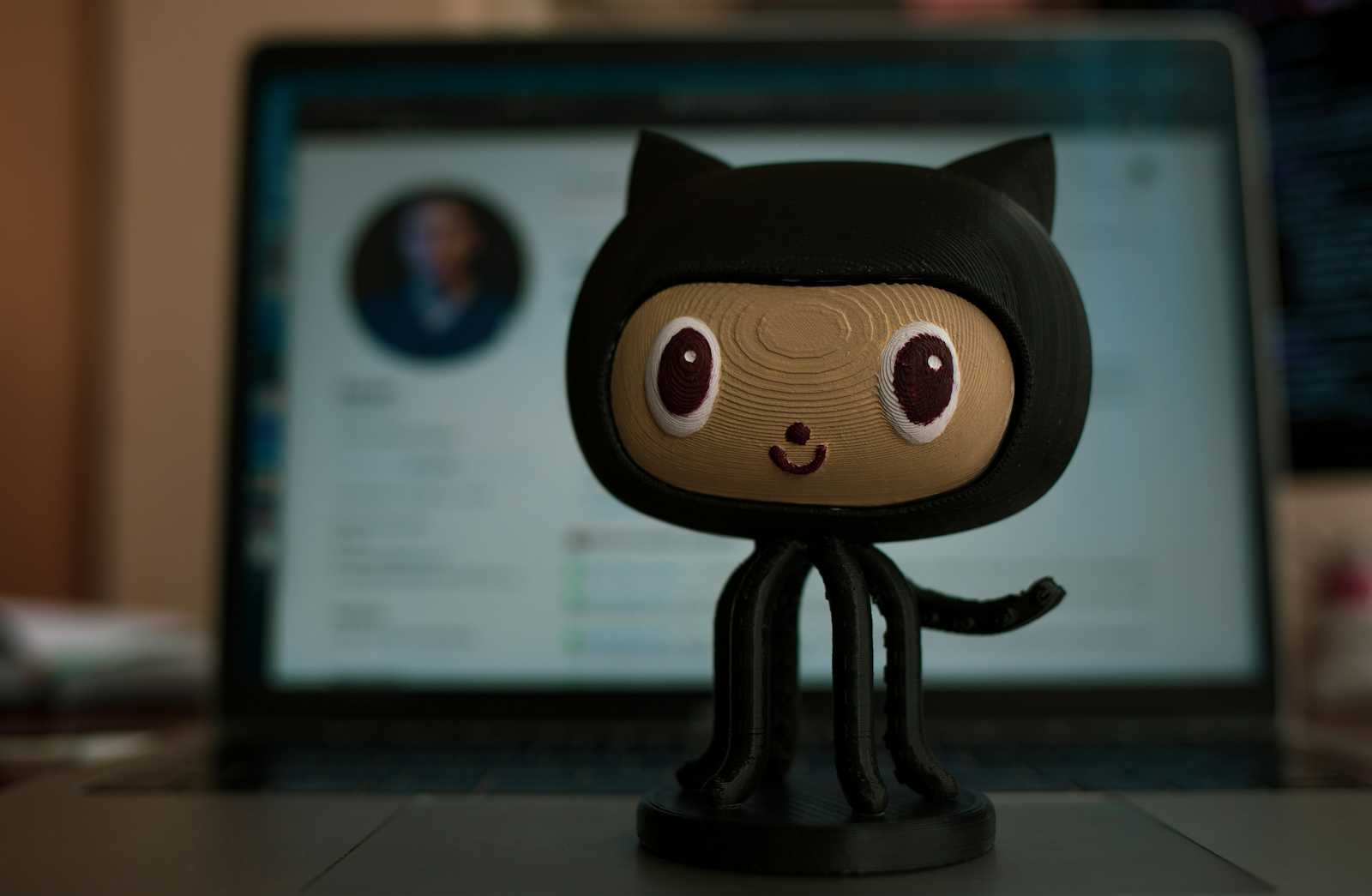
In the modern software development lifecycle, Continuous Integration and Continuous Deployment (CI/CD) have become essential practices for ensuring that code changes are seamlessly integrated, tested, and deployed to production environments. GitHub Actions, a powerful automation tool provided by GitHub, allows developers to set up CI/CD pipelines directly within their GitHub repositories. In this guide, we'll walk through the process of setting up a CI/CD pipeline using GitHub Actions to automate the testing and deployment of your application. ๐ป
๐ฏ What is CI/CD?
Continuous Integration (CI) is the practice of automatically integrating code changes into a shared repository several times a day. This process includes running automated tests to catch any issues early.
Continuous Deployment (CD) takes CI one step further by automatically deploying the integrated code to a production environment if all tests pass. This ensures that new features, bug fixes, and updates are delivered to users quickly and efficiently.
๐ง Why Use GitHub Actions for CI/CD?
GitHub Actions is a flexible and powerful tool that integrates seamlessly with your GitHub repository. It allows you to automate your development workflows, including CI/CD, with custom scripts called "actions" that can be triggered by specific events, such as pushing code to a branch or creating a pull request.
Key Benefits of GitHub Actions:
Integration with GitHub: GitHub Actions is natively integrated with GitHub, making it easy to set up and manage workflows directly from your repository.
Customizability: You can create custom workflows tailored to your specific needs, from running tests to deploying applications.
Scalability: GitHub Actions can scale with your projects, whether you're working on a small personal project or a large enterprise application.
Marketplace: Access to a wide range of pre-built actions in the GitHub Marketplace that can simplify your workflow setup.
๐ฏ Setting Up a CI/CD Pipeline with GitHub Actions
Let's dive into setting up a CI/CD pipeline for a Node.js application using GitHub Actions. The pipeline will automatically run tests when code is pushed to the repository and deploy the application to a production server if the tests pass.
Step 1: Create a GitHub Repository
First, create a new GitHub repository for your application if you haven't already. If you have an existing repository, you can skip this step.
# Initialize a new GitHub repository
git init
git add .
git commit -m "Initial commit"
git branch -M main
git remote add origin https://github.com/yourusername/your-repository.git
git push -u origin main
Step 2: Add a GitHub Actions Workflow
In your repository, create a .github/workflows
directory if it doesn't already exist. Inside this directory, create a new file named ci-cd-pipeline.yml
.
# .github/workflows/ci-cd-pipeline.yml
name: CI/CD Pipeline
# Trigger the workflow on push and pull request events
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
deploy:
runs-on: ubuntu-latest
needs: build
if: github.ref == 'refs/heads/main' && github.event_name == 'push'
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Deploy to Production Server
run: |
echo "Deploying to production server..."
# Add your deployment script here, e.g., SSH into your server and pull the latest code
Step 3: Define Your Test Script
Make sure your package.json
file includes a test script that can be executed by the CI job:
{
"scripts": {
"test": "jest"
}
}
Replace "jest"
with your preferred testing framework if you're not using Jest.
Step 4: Add Deployment Script
In the deploy
job, replace the run: |
section with your actual deployment commands. This could involve SSH-ing into your server, pulling the latest code, and restarting your application.
Example:
ssh user@yourserver.com "cd /path/to/your/app && git pull origin main && npm install && pm2 restart all"
Step 5: Commit and Push
Commit your changes and push them to your GitHub repository:
git add .
git commit -m "Set up CI/CD pipeline with GitHub Actions"
git push origin main
Once you push your changes, the GitHub Actions workflow will be triggered. You can monitor the progress of your CI/CD pipeline by navigating to the "Actions" tab in your GitHub repository.
๐ Congratulations!
You've successfully set up a CI/CD pipeline using GitHub Actions! Now, every time you push code to the main branch or open a pull request, your application will be automatically tested and deployed if all tests pass. This automation ensures that your application remains in a stable and deployable state at all times, enabling you to deliver new features and updates faster.
Happy coding! ๐
Feel free to customize the pipeline further to suit your specific project needs. Whether it's adding additional jobs for code linting, integrating with other services, or deploying to different environments, GitHub Actions offers the flexibility to tailor your CI/CD process to perfection.
If you found this guide helpful, don't forget to share it with your fellow developers! ๐ปโจ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
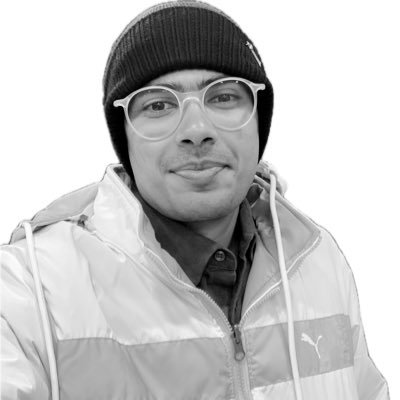
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on โจ๏ธ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix ๐จ๐ปโ๐ป