How to Use Python for Automatic File Organization
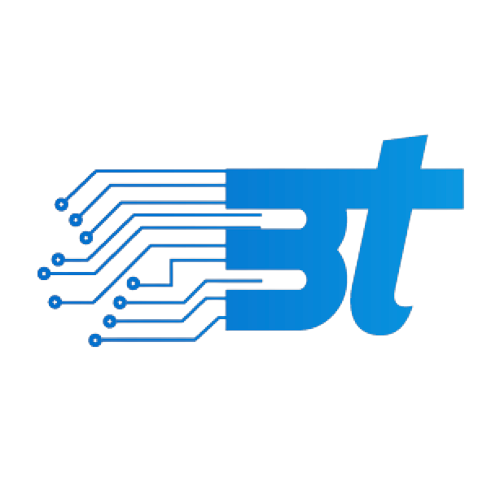
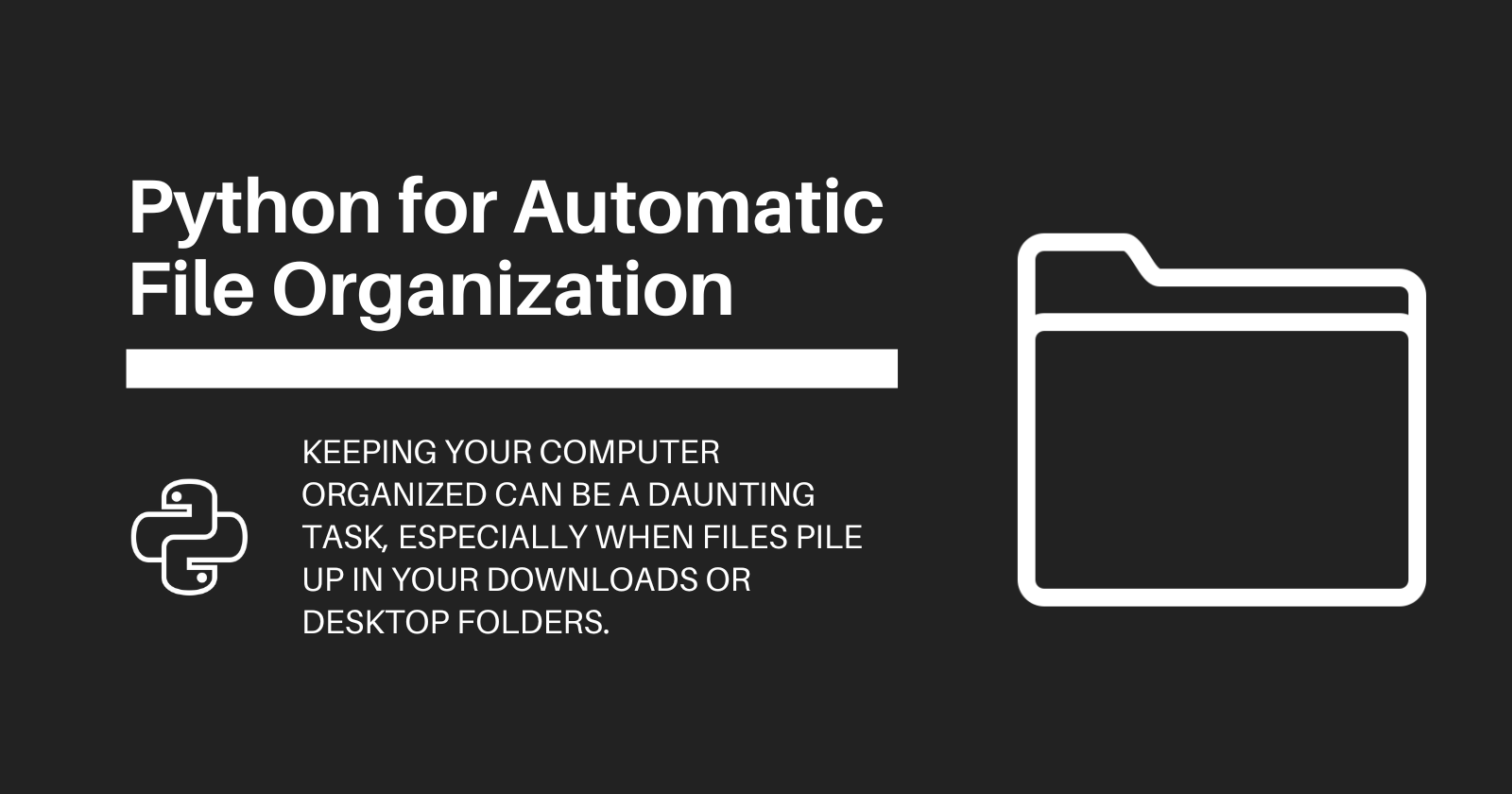
Keeping your computer organized can be a daunting task, especially when files pile up in your Downloads or Desktop folders. Manually sorting these files into appropriate folders can be time-consuming. In this blog, we’ll create a Python script to automate the organization of your files based on their types, extensions, or other criteria. This script will save you time and keep your workspace clutter-free.
What You’ll Learn:
How to use the
os
andshutil
libraries for file operations.Organizing files by type (e.g., documents, images, videos).
Moving files to specific directories based on extensions.
Handling duplicate files and errors gracefully.
1. Setting Up the Environment
Before we start coding, make sure you have Python installed on your system. We'll use the os
and shutil
libraries, which come pre-installed with Python.
2. Defining the File Organization Logic
Step 1: Create a Directory Structure
First, let's define the directories where we want to move our files. We'll create folders like Documents
, Images
, Videos
, etc., within a target directory.
import os
def create_directories(base_directory, directories):
for directory in directories:
path = os.path.join(base_directory, directory)
if not os.path.exists(path):
os.makedirs(path)
# Example usage
base_directory = "/path/to/your/target_directory"
directories = ["Documents", "Images", "Videos", "Music", "Others"]
create_directories(base_directory, directories)
Step 2: Define File Types
We need to map file extensions to their respective directories.
file_types = {
"Documents": [".pdf", ".docx", ".txt", ".xlsx", ".pptx"],
"Images": [".jpg", ".jpeg", ".png", ".gif", ".bmp"],
"Videos": [".mp4", ".mkv", ".flv", ".avi"],
"Music": [".mp3", ".wav", ".aac"],
}
3. Writing the File Organization Script
Step 3: Organizing Files by Type
Now, let's write a function to move files based on their extensions.
import shutil
def organize_files(source_directory, base_directory, file_types):
for filename in os.listdir(source_directory):
source_path = os.path.join(source_directory, filename)
if os.path.isfile(source_path):
file_extension = os.path.splitext(filename)[1].lower()
moved = False
for directory, extensions in file_types.items():
if file_extension in extensions:
destination_path = os.path.join(base_directory, directory, filename)
shutil.move(source_path, destination_path)
moved = True
break
if not moved:
# If file type is not recognized, move to "Others"
destination_path = os.path.join(base_directory, "Others", filename)
shutil.move(source_path, destination_path)
# Example usage
source_directory = "/path/to/your/source_directory"
organize_files(source_directory, base_directory, file_types)
Step 4: Handling Duplicates
What if a file with the same name already exists in the target directory? Let’s add a function to handle duplicates by appending a number to the filename.
def handle_duplicates(destination_path):
base, extension = os.path.splitext(destination_path)
counter = 1
new_destination = destination_path
while os.path.exists(new_destination):
new_destination = f"{base}_{counter}{extension}"
counter += 1
return new_destination
Now, we can integrate this into the organize_files
function:
def organize_files(source_directory, base_directory, file_types):
for filename in os.listdir(source_directory):
source_path = os.path.join(source_directory, filename)
if os.path.isfile(source_path):
file_extension = os.path.splitext(filename)[1].lower()
moved = False
for directory, extensions in file_types.items():
if file_extension in extensions:
destination_path = os.path.join(base_directory, directory, filename)
destination_path = handle_duplicates(destination_path)
shutil.move(source_path, destination_path)
moved = True
break
if not moved:
destination_path = os.path.join(base_directory, "Others", filename)
destination_path = handle_duplicates(destination_path)
shutil.move(source_path, destination_path)
4. Enhancing the Script
Step 5: Adding a Command-Line Interface
We can make the script more user-friendly by adding a CLI so users can specify the source and target directories.
import argparse
def main():
parser = argparse.ArgumentParser(description="Automate File Organization")
parser.add_argument('source_directory', type=str, help="Path to the directory with files to organize")
parser.add_argument('target_directory', type=str, help="Path to the directory where files will be organized")
args = parser.parse_args()
create_directories(args.target_directory, directories)
organize_files(args.source_directory, args.target_directory, file_types)
if __name__ == "__main__":
main()
5. Running the Script
You can run the script from the command line:
python organize_files.py /path/to/source_directory /path/to/target_directory
Conclusion
By building and using this tool, you’ll not only improve your productivity but also gain a deeper understanding of file handling in Python. Feel free to experiment with the code, and let me know how you plan to enhance this script in the comments!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
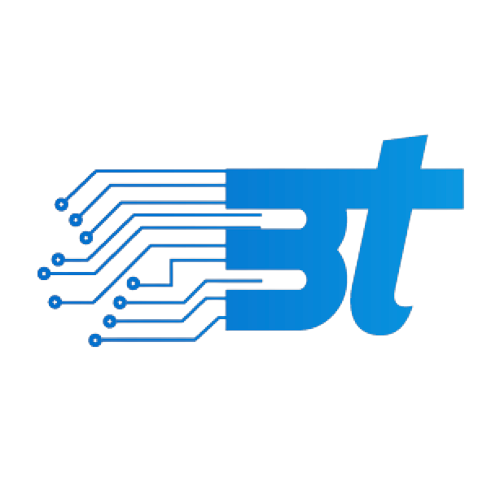
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.