Coercion JS Part 2
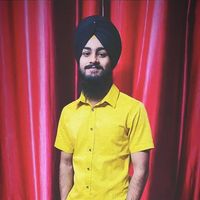
Type Conversion
ToPrimitive(input,PreffredType )
PreffredType is optional argument
In case where input can be converted into multiple type, we take the decesion using this.
Convert the input into non-object type.
Type Conversion discusses the conversion of input into non-object types, highlighting the optional PreferredType argument used to decide the type when multiple conversions are possible.
Assert: input is an ECMAScript language value: This means the code is expecting something that JavaScript understands.
Check if input is an object: If the input is an object (like an array, function, or custom object), the code continues; otherwise, it's already a primitive and can be returned.
Determine the hint: Based on a preference (called
PreferredType
), the code decides whether to try converting the object to a string or a number. If no preference is given, it defaults to number.Look for a custom conversion method: Some objects might have a special method to convert themselves to primitive values. If found, use that method.
Check the result: If the result of the conversion is not an object, it's a primitive and can be returned. Otherwise, an error occurs.
Default to number conversion: If the original hint was "default", try to convert the object to a number.
Try a standard conversion: If the above steps fail, use a standard method to convert the object to a primitive based on the hint.
If all else fails: Return the original input as it is.
let myObject = { value: 42 }; // Trying to add myObject to a number directly would cause an error let result = myObject + 10; // This will throw an error // The code above would try to convert myObject to a number using the steps outlined above. // If successful, result would be 52.
OridinaryToPrimitive (input,hint)
When the abstract operator OrdinaryToPrimitive is called with argumwnt 0 and hint.
Type hint either "String" or "Number"
"String" --> ["tostring","valueof"] --> if the string method give it primitive return if give it primitive return then call valueof if it give primitive type return if not return type error.
let obj={}
console.log(obj.toString());
// [object Object]
"Number" -->["valueof","tostrring"] (method)
let obj1 = {x:9,y:9};
console.log(100-obj1) // NaN
let obj2 = {x:7,valueOf() {return 99}};
console.log(100-obj2) // 1
let obj3 = {x:7,valueOf() {return "99"}};
console.log(100-obj3) // 11
let obj4 = {x:7,valueOf() {return {}};
console.log(100-obj12) // type error
ToNumber(10): The number 10 is already a number, so no conversion is needed. It remains 10.
ToNumber(obj): This is where the conversion of the object
obj
to a number begins.ToPrimitive(obj, hint Number): The object
obj
is passed to theToPrimitive
function with a hint of "Number." This indicates that the system prefers a numeric conversion if possible.OrdinaryToPrimitive(obj, hint Number): Since
obj
doesn't have a custom@@toPrimitive
method, the standardOrdinaryToPrimitive
function is used.Hint Number: The hint remains "Number" as the initial preference.
[value of, "toString"]: The
OrdinaryToPrimitive
function attempts to call thevalueOf()
method on the object.obj.valueOf(): This returns the object itself, as it's not a primitive value.
Object (Non-object type): The result of
valueOf()
is still an object, not a primitive.obj.toString(): Since
valueOf()
didn't produce a primitive, thetoString()
method is called on the object.[object Object] type is String: The
toString()
method typically returns a string representation of the object, which is "[object Object]" in this case.Return String: The
toString()
method returns the string "[object Object]."ToNumber(String): The returned string "[object Object]" is then passed to the
ToNumber
function for conversion to a number.NaN: The conversion of "[object Object]" to a number results in
NaN
(Not a Number).10 + NaN: Finally, the original operation
10 + obj
becomes10 + NaN
, which results inNaN
.
Conclusion:
The summary demonstrates that when an object is added to a number in JavaScript, the object is first converted to a primitive value using ToPrimitive
and then to a number using ToNumber
. If these conversions fail to produce a valid number, the result is NaN
.
Addition Operator (+)
let obj1 = { value: 10 }; let obj2 = { value: 20 };
let result = obj1 + obj2; // Output: "[object Object][object Object]"
Abstract Equality (==) and Strict Equality (===)
The Myth
"Myth: In Internet, == (Not check type, only check value)"
This is not entirely accurate. Both ==
and ===
perform type checks. The difference lies in what happens when the types are different.
Abstract Equality (==)
Type Check: If the types of the operands are the same, the comparison proceeds directly.
Type Conversion (if necessary): If the types are different, JavaScript attempts to convert one or both operands to a common type before comparing their values.
Value Comparison: Once the types are compatible, the values are compared.
Example:
JavaScript
console.log(5 == "5"); // Output: true
In this case, the number 5
is converted to the string "5"
before comparison, resulting in true
.
Strict Equality (===)
Type Check: If the types of the operands are different, the comparison immediately returns
false
.Value Comparison: Only if the types are identical does JavaScript proceed to compare the values.
Example:
console.log(5 === "5"); // Output: false
Here, since the types are different (number and string), the comparison is false
without any value conversion.
Key Points to Remember
Use
===
whenever possible to avoid unexpected type coercion and potential errors.Use
==
cautiously, understanding that it might perform type conversions.Be aware of the common type conversions that JavaScript performs (e.g.,
null
andundefined
are equal with==
, numbers and strings can be compared after conversion).
Subscribe to my newsletter
Read articles from Ranjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
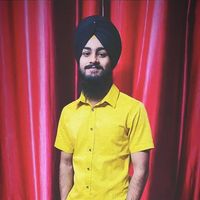