Understanding Arrays in Go: A Comprehensive Guide with Examples

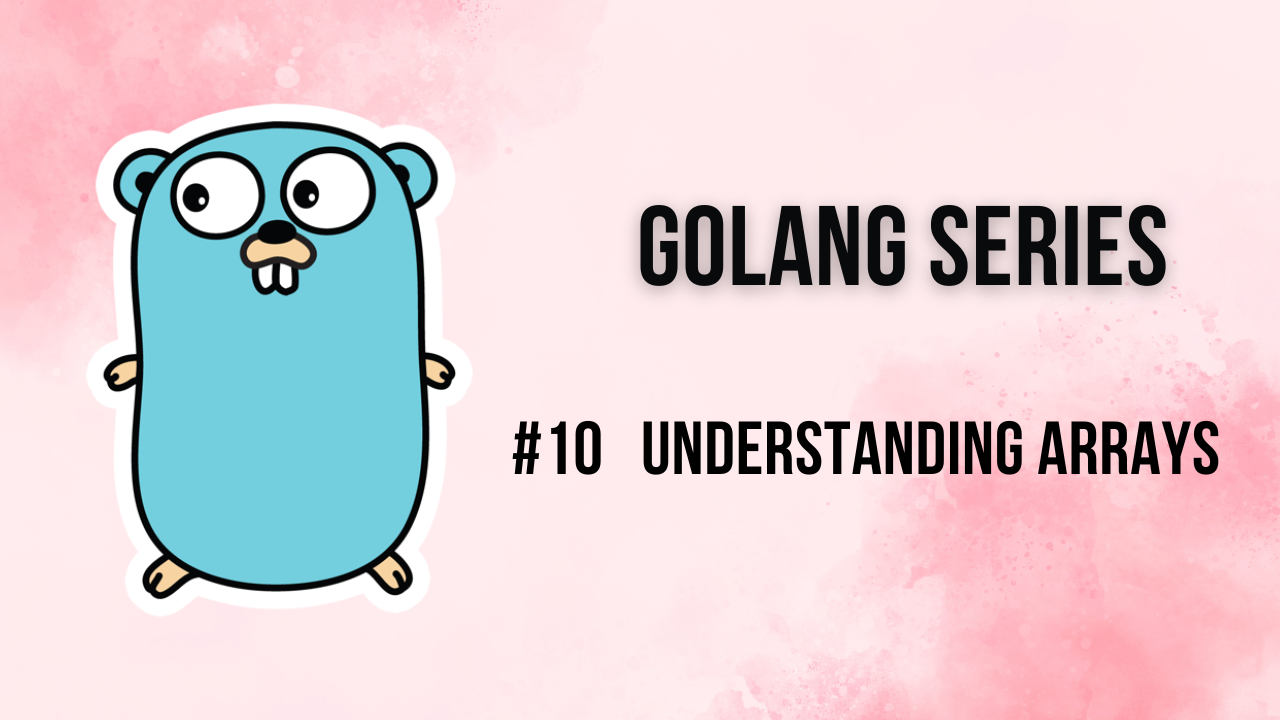
Arrays are an essential part of Go programming. They provide a way to store multiple elements of the same type together in a fixed-size collection. In this blog, we’ll dive deep into arrays in Go, covering their definition, how to declare and use them, and some practical examples.
1. What is an Array?
An array in Go is a collection of elements with a fixed size. All elements in an array must be of the same data type. Once an array is declared, its size cannot change.
2. Declaring an Array in Go
You can declare an array by specifying the size and the type of elements it holds. The syntax is:
var arrayName [size]elementType
Example:
var numbers [5]int
In the above example, we declared an array named numbers
that can hold 5 integers.
You can also initialize an array during declaration:
var fruits = [3]string{"Apple", "Banana", "Cherry"}
If you don’t specify the size, Go infers it based on the number of elements:
numbers := [...]int{1, 2, 3, 4, 5}
3. Accessing and Modifying Array Elements
You can access an array element using its index. Indexes in Go start from 0.
Example:
fruits := [3]string{"Apple", "Banana", "Cherry"}
fmt.Println(fruits[0]) // Output: Apple
To modify an element:
fruits[1] = "Mango"
fmt.Println(fruits) // Output: [Apple Mango Cherry]
4. Iterating Over Arrays
You can use a for
loop to iterate over array elements:
Example:
numbers := [5]int{10, 20, 30, 40, 50}
for i := 0; i < len(numbers); i++ {
fmt.Println(numbers[i])
}
Alternatively, you can use a for range
loop:
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
5. Practical Examples
Here’s a practical example to demonstrate array usage in Go:
Example 1: Calculate the sum of elements in an array
package main
import "fmt"
func main() {
numbers := [5]int{5, 10, 15, 20, 25}
sum := 0
for _, value := range numbers {
sum += value
}
fmt.Printf("The sum of the array is: %d\n", sum)
}
Output:
The sum of the array is: 75
Example 2: Finding the maximum value in an array
package main
import "fmt"
func main() {
numbers := [5]int{8, 15, 22, 3, 10}
max := numbers[0]
for _, value := range numbers {
if value > max {
max = value
}
}
fmt.Printf("The maximum value in the array is: %d\n", max)
}
Output:
The maximum value in the array is: 22
6. Conclusion
Arrays in Go are powerful but have a fixed size, making them less flexible compared to slices (which we’ll cover in another post). They’re perfect when you know the exact number of elements you need to store. Arrays provide a simple, fast, and efficient way to manage collections of data in Go.
In this blog, we covered the basics of arrays in Go, from declaration to practical usage examples. Understanding arrays is foundational for mastering more advanced data structures in Go.
This guide should give you a solid understanding of arrays in Go. In the next part of this series, we’ll explore slices, which are more flexible and widely used in Go programming.
Happy coding!
Subscribe to my newsletter
Read articles from Navya A directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Navya A
Navya A
👋 Welcome to my Hashnode profile! I'm a passionate technologist with expertise in AWS, DevOps, Kubernetes, Terraform, Datree, and various cloud technologies. Here's a glimpse into what I bring to the table: 🌟 Cloud Aficionado: I thrive in the world of cloud technologies, particularly AWS. From architecting scalable infrastructure to optimizing cost efficiency, I love diving deep into the AWS ecosystem and crafting robust solutions. 🚀 DevOps Champion: As a DevOps enthusiast, I embrace the culture of collaboration and continuous improvement. I specialize in streamlining development workflows, implementing CI/CD pipelines, and automating infrastructure deployment using modern tools like Kubernetes. ⛵ Kubernetes Navigator: Navigating the seas of containerization is my forte. With a solid grasp on Kubernetes, I orchestrate containerized applications, manage deployments, and ensure seamless scalability while maximizing resource utilization. 🏗️ Terraform Magician: Building infrastructure as code is where I excel. With Terraform, I conjure up infrastructure blueprints, define infrastructure-as-code, and provision resources across multiple cloud platforms, ensuring consistent and reproducible deployments. 🌳 Datree Guardian: In my quest for secure and compliant code, I leverage Datree to enforce best practices and prevent misconfigurations. I'm passionate about maintaining code quality, security, and reliability in every project I undertake. 🌐 Cloud Explorer: The ever-evolving cloud landscape fascinates me, and I'm constantly exploring new technologies and trends. From serverless architectures to big data analytics, I'm eager to stay ahead of the curve and help you harness the full potential of the cloud. Whether you need assistance in designing scalable architectures, optimizing your infrastructure, or enhancing your DevOps practices, I'm here to collaborate and share my knowledge. Let's embark on a journey together, where we leverage cutting-edge technologies to build robust and efficient solutions in the cloud! 🚀💻