Change the ClaimsIdentity.AuthenticationType in ASP.NET Core with multiple Entra ID schemes.
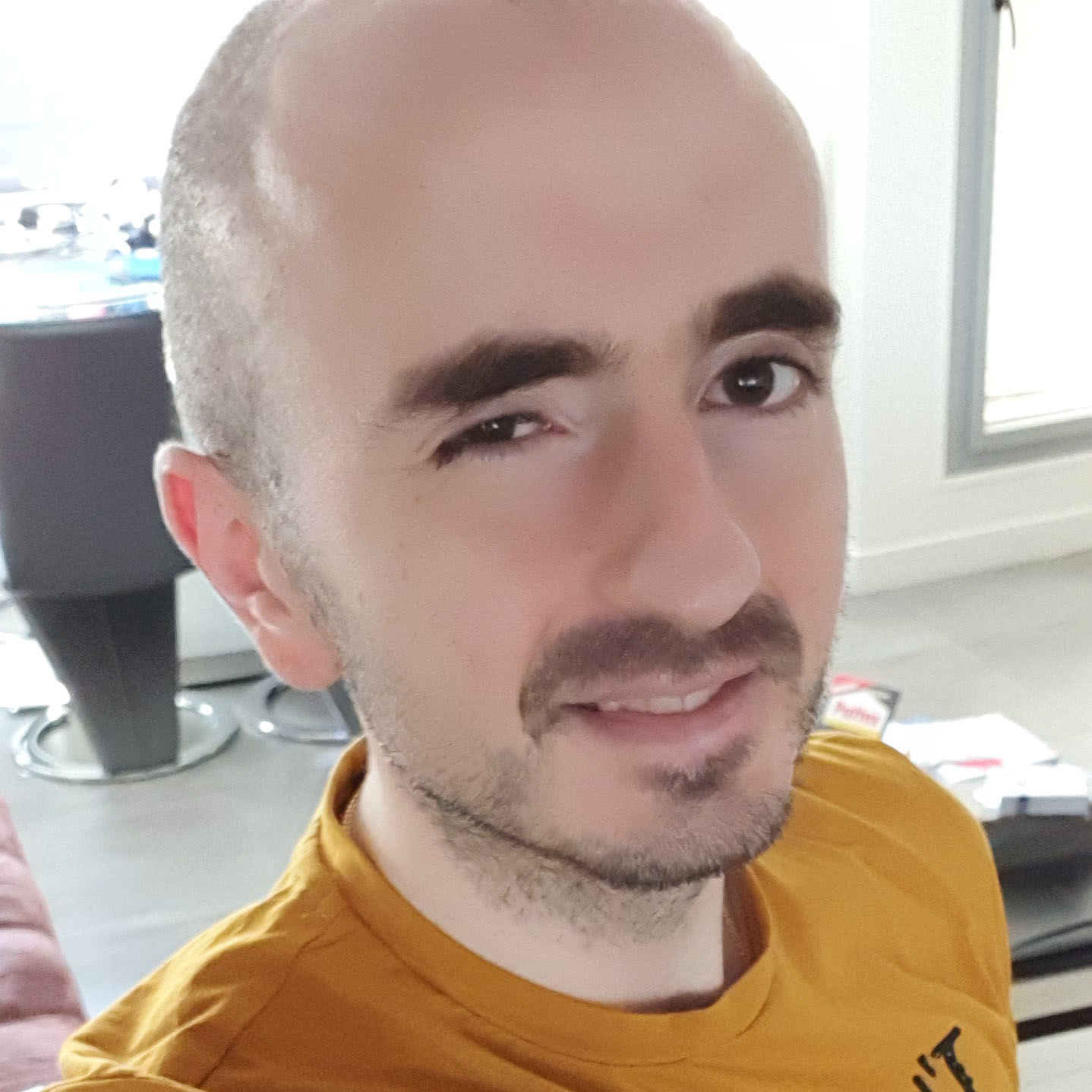
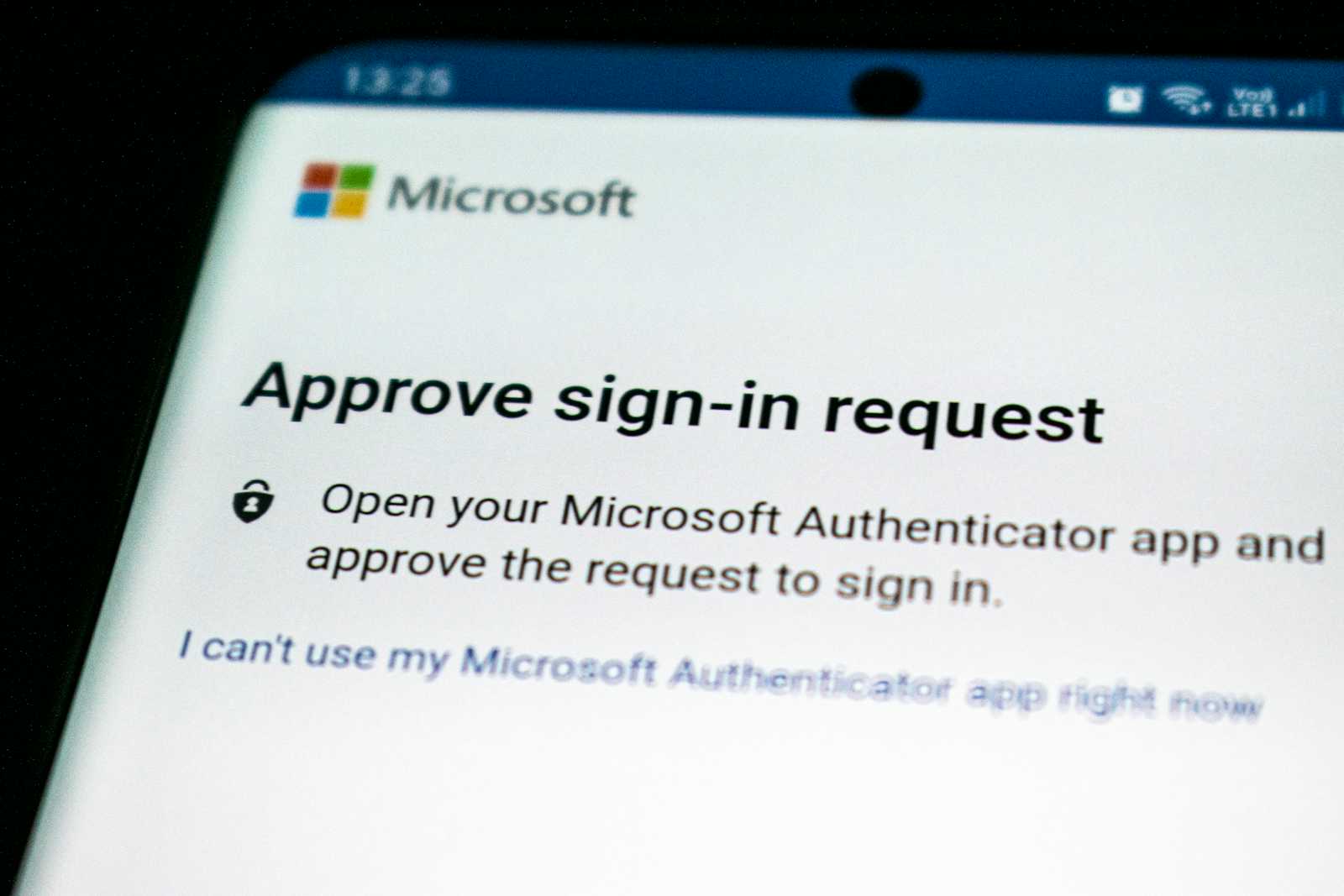
Introduction
When building modern web applications, security is always a top priority. In ASP.NET Core, authentication is a key part of ensuring that only authorized users can access specific parts of your application. You may often need to support multiple authentication schemes, such as different Azure Entra ID (formerly Azure AD) configurations, within the same application.
One challenge when using multiple authentication schemes is identifying which scheme authenticated a user. This is where the ClaimsIdentity.AuthenticationType
property comes into play. Setting this property correctly can help you easily determine the authentication source for a user.
Understanding HttpContext.User
In ASP.NET Core, the HttpContext.User
property represents the current authenticated user. This user is represented by a ClaimsPrincipal
object, which contains one or more ClaimsIdentity
objects. Each ClaimsIdentity
represents a specific identity associated with the user, such as a name, roles, and other claims.
By default, the AuthenticationType
is set to "AuthenticationTypes.Federation"
. However, when working with multiple authentication schemes, you might want to know exactly which authentication scheme was used to create a particular ClaimsIdentity
. This is where setting a custom AuthenticationType
can be very useful.
For example, let’s say you want to retrieve the AuthenticationType
of the currently authenticated user to determine how they were authenticated:
public IActionResult GetAuthenticationType()
{
var identity = HttpContext.User.Identity as ClaimsIdentity;
if (identity != null)
{
string authenticationType = identity.AuthenticationType;
return Ok($"User authenticated using: {authenticationType}");
}
return BadRequest("No identity found.");
}
This example demonstrates how you can access the AuthenticationType
in your controller to gain insights into the user's authentication method.
The Problem with Multiple Authentication Schemes
When you have multiple authentication schemes, such as two different configurations for Azure Entra ID, it's not always clear which scheme authenticated the user. Without setting the AuthenticationType
, you may not be able to distinguish between the identities created by different schemes. This can be problematic in scenarios where your application's logic depends on the authentication source.
Setting the AuthenticationType
To set the AuthenticationType
in ASP.NET Core, you need to configure it in the TokenValidationParameters
when setting up your authentication schemes. The TokenValidationParameters.AuthenticationType
property allows you to specify a unique identifier for the authentication scheme, which is then assigned to the ClaimsIdentity.AuthenticationType
.
For example, let's say you have two Azure Entra ID configurations: one for internal users and another for external partners. You can set up your authentication schemes as follows:
public void ConfigureServices(IServiceCollection services)
{
// Internal users authentication scheme
services.AddAuthentication("InternalScheme")
.AddMicrosoftIdentityWebApi(options =>
{
options.Instance = "https://login.microsoftonline.com/";
options.Domain = "internaldomain.com";
options.ClientId = "your-client-id";
options.TenantId = "your-tenant-id";
// Set the AuthenticationType for the internal scheme
options.TokenValidationParameters.AuthenticationType = "Internal";
});
// External partners authentication scheme
services.AddAuthentication("ExternalScheme")
.AddMicrosoftIdentityWebApi(options =>
{
options.Instance = "https://login.microsoftonline.com/";
options.Domain = "externaldomain.com";
options.ClientId = "your-client-id";
options.TenantId = "your-tenant-id";
// Set the AuthenticationType for the external scheme
options.TokenValidationParameters.AuthenticationType = "External";
});
}
Retrieving the AuthenticationType
Now that you've configured the AuthenticationType
for each scheme, you can retrieve it later in your code to determine which scheme authenticated the user:
public IActionResult GetUserIdentity()
{
var identity = HttpContext.User.Identity as ClaimsIdentity;
if (identity != null)
{
string authenticationType = identity.AuthenticationType;
return Ok($"User authenticated using: {authenticationType}");
}
return BadRequest("No identity found.");
}
Conclusion
By setting the AuthenticationType
in the TokenValidationParameters
, you can clearly distinguish between different authentication schemes in ASP.NET Core. This approach is especially useful when your application needs to support multiple authentication sources, such as different Azure Entra ID configurations. With this setup, you'll be able to write more precise and secure authentication logic in your application.
Subscribe to my newsletter
Read articles from Gilles TOURREAU directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
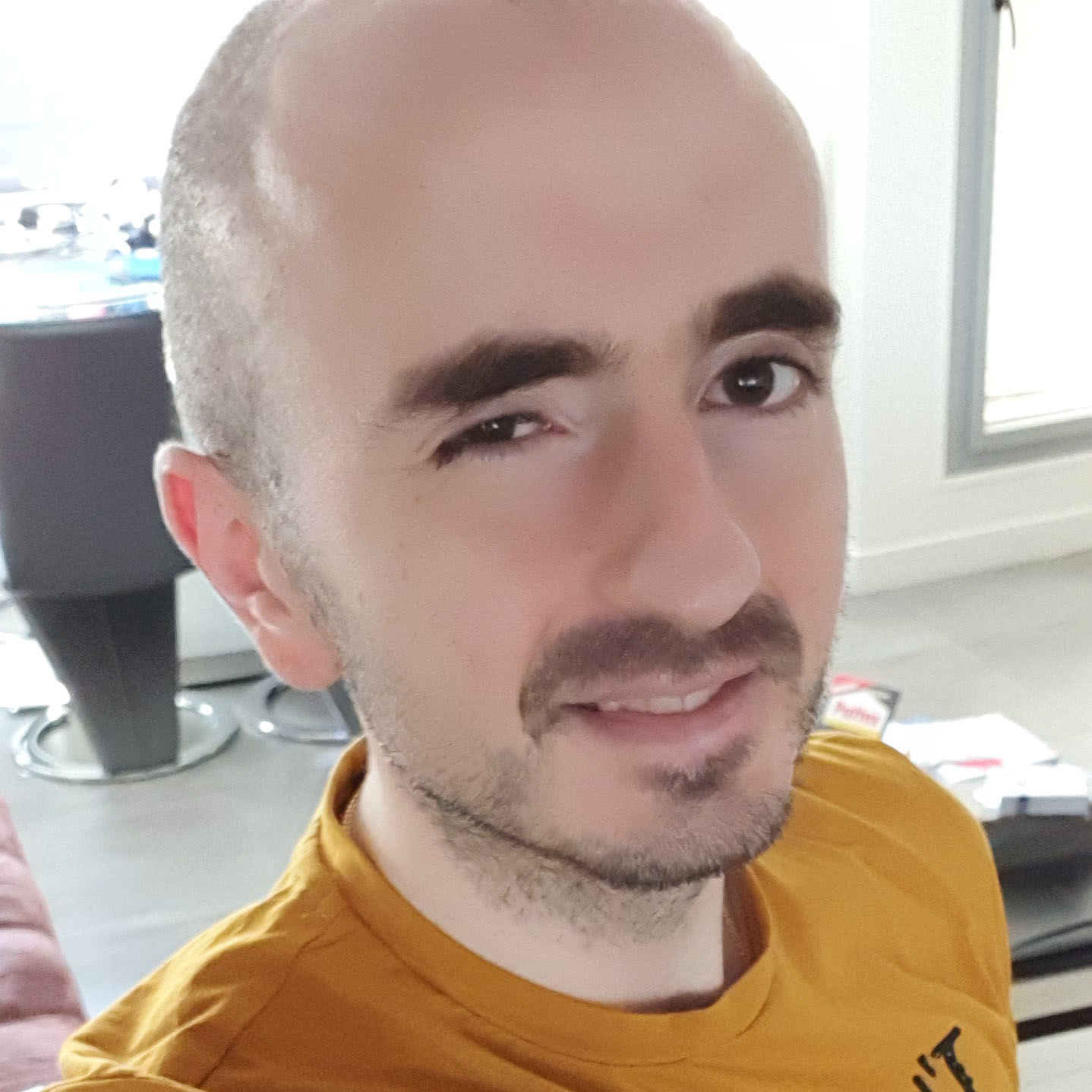
Gilles TOURREAU
Gilles TOURREAU
I'm a French seasoned architect and developer specializing in Microsoft technologies such as .NET and Azure. As a freelance professional, I've lent my expertise to diverse sectors including Energy, Banking, Insurance, Software Publishing, Transportation, and Industry. With over 25 years of dedicated experience in Microsoft technologies, I've established three software publishing companies, initially on-premises and later transitioning to SaaS models. My journey has been driven by a passion for innovation and a commitment to delivering top-notch solutions in the ever-evolving tech landscape. Humorously attributing my baldness to solving countless tech puzzles. Passionate about travel, having worked extensively abroad in Indonesia, Hong Kong, Singapore, and Australia. Philippines holds my heart, magnetized by its heavenly beaches. My English might not be top-notch, but it’s enough to bicker with my Filipina partner! 😁