Building an Emotion-Detecting Telegram Bot with Python and Node.js
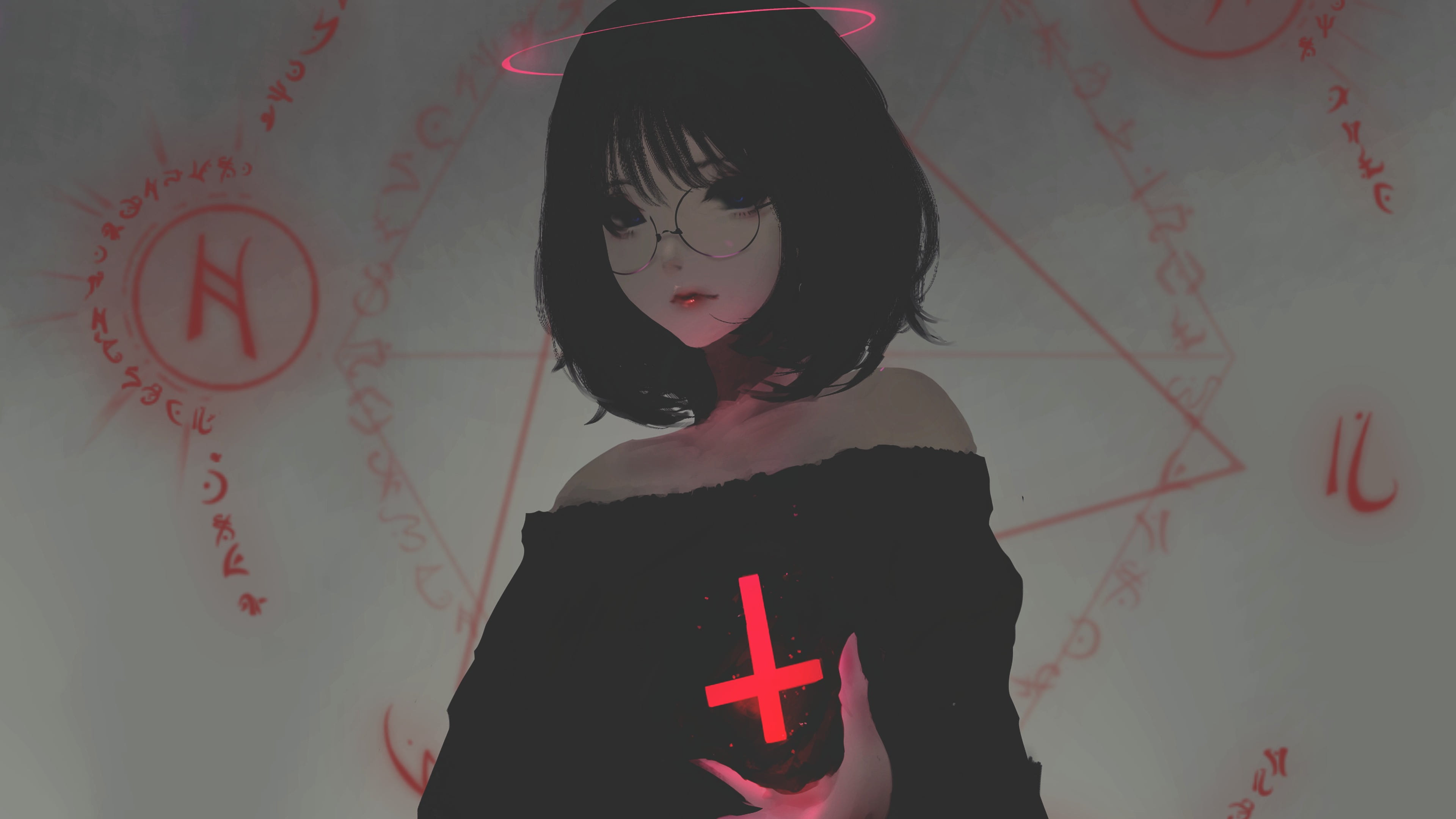
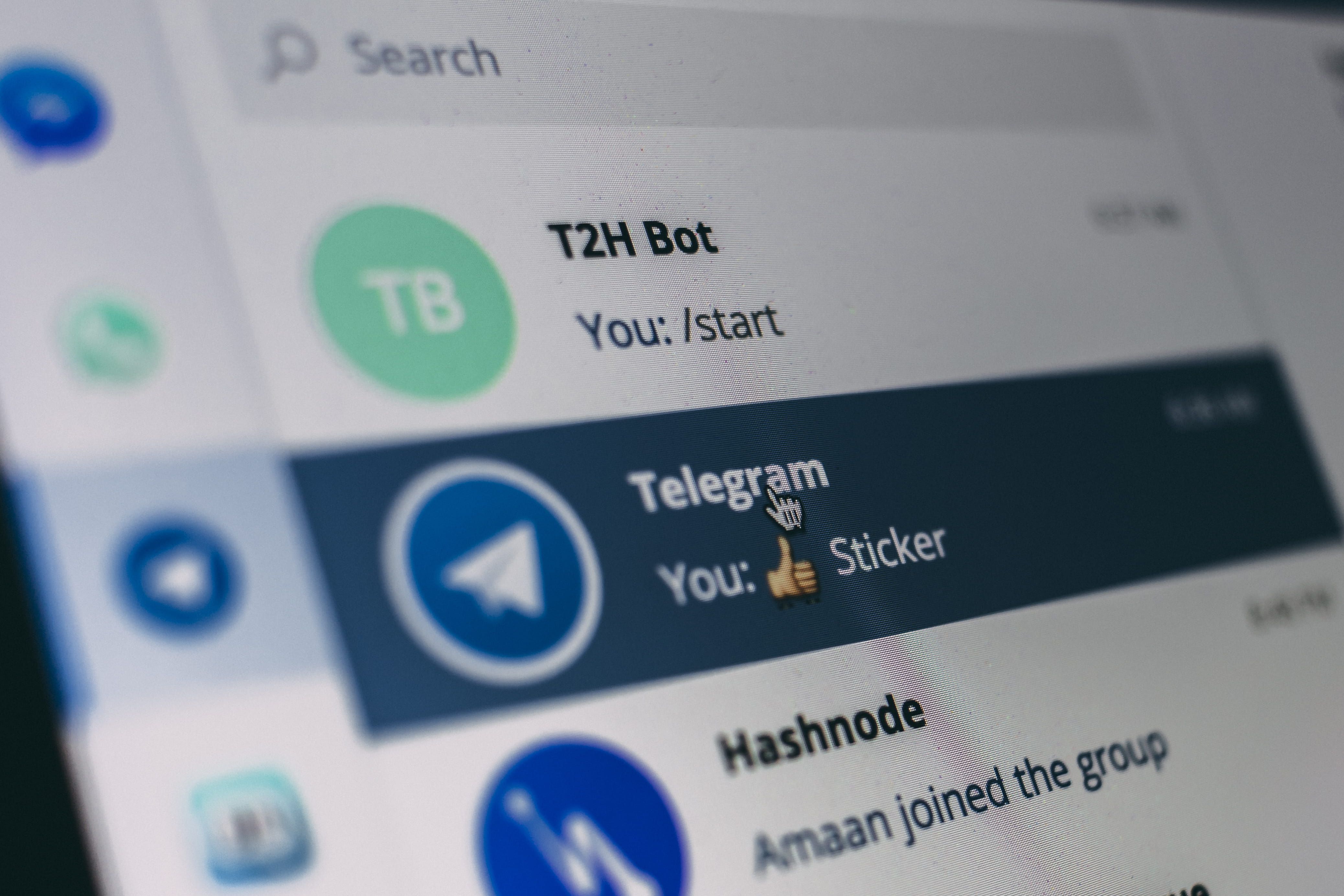
Introduction
In today’s world, where human-computer interaction is becoming more advanced, understanding and responding to user emotions is a big step forward. This blog will show you how I built a Telegram bot that can detect emotions from user input and cheer them up with a joke when needed.
Overview
The idea behind this project was simple: create a Telegram bot that interacts with users and predicts emotions based on their text inputs. If a negative emotion is detected, the bot will offer to cheer up the user by telling a joke. This project combines various technologies, including Python for machine learning, Node.js for backend logic, and the Telegram Bot API for interfacing with Telegram.
Technologies Used
Node.js: To handle the backend logic and manage the bot’s interaction.
Telegram Bot API: For communication between the bot and Telegram.
Express and Flask: Flask runs the emotion prediction API, while Express could be used for additional server-side tasks.
Axios: To handle HTTP requests between the bot and the Flask API.
Python & scikit-learn: For creating the emotion prediction model.
Setting Up the Environment
Cloning the Repository
The first step is to clone the repository from GitHub:
git clone https://github.com/RishabhRaj43/Emotion-Detector-Telegram-Bot.git
Installing Node.js Dependencies
To get started with the Node.js part of the project, install the necessary dependencies:
npm install axios dotenv node-telegram-bot-api
Setting Up Environment Variables
Create a .env
file in the root directory to store your Telegram bot token:
TOKEN = "your-telegram-bot-token"
Installing Python Dependencies
Next, install the required Python packages to set up the Flask server and the emotion detection model:
pip install flask flask-cors scikit-learn joblib
Preparing the Emotion Model
The heart of the project is the emotion detection model. You need to train a model (or use an existing one) and save it as emotion.pkl
in the root directory. This model will be used by the Flask API to predict emotions from text.
Learn How to Train the Model
Building the Flask API
The Flask API is responsible for predicting the emotion from the user's input. Here’s the code for the Flask app:
import joblib
from flask_cors import CORS
from flask import Flask, request, jsonify
app = Flask(__name__)
CORS(app)
@app.route('/predict', methods=['POST'])
def index():
data = request.json
with open('emotion.pkl', 'rb') as f:
model = joblib.load(f)
pred = model.predict([data])
return jsonify({'emotion': pred[0]})
if __name__ == '__main__':
app.run(debug=True)
This script loads the pre-trained emotion detection model and serves it through a REST API. When the bot sends a text input, this API returns the predicted emotion.
Building the Telegram Bot
The Telegram bot is built using Node.js and the node-telegram-bot-api
package. Here’s how the bot is structured:
Basic Commands: The bot responds to basic commands like
/start
,/help
, and/command
by providing information and displaying a custom keyboard.Emotion Prediction: The bot prompts the user for text input and sends this text to the Flask API for emotion analysis.
Jokes: If a negative emotion is detected, the bot offers to cheer up the user with a joke fetched from an external API.
Here’s a snippet of the bot’s main logic:
import telegram from "node-telegram-bot-api";
import dotenv from "dotenv";
import axios from "axios";
import command from "./routes/commands.js";
import sendMessage from "./routes/sendMsg.js";
import predict from "./routes/predict.js";
dotenv.config();
const token = process.env.TOKEN;
const bot = new telegram(token, { polling: true });
let prediction = false;
bot.onText(/\/start/, (msg) => {
bot.sendMessage(msg.chat.id, "Hello there! How can I help you?");
command(bot, msg);
});
bot.onText(/\/checkemotion/, (msg) => {
prediction = true;
sendMessage(bot, msg.chat.id, "Enter the text you want to check");
});
bot.on("message", (msg) => {
if (prediction) {
predict(bot, msg);
prediction = false;
} else {
sendMessage(bot, msg.chat.id, "Sorry I can't reply to other messages");
}
});
Running the Bot
To run the bot, you need to start both the Flask server and the Node.js bot:
Run the Flask Server:
python app.py
Start the Telegram Bot:
npm start
Interacting with the Bot
Once everything is set up, you can interact with the bot using the following commands:
/start
: Start the bot and get a welcome message./checkemotion
: Enter text to analyze your emotion./joke
: Get a random joke if you need a laugh./command
: Show the custom keyboard for easy command access.
Conclusion
This project shows how to combine Python and Node.js to create a powerful tool that interacts with users on Telegram. By detecting emotions and responding appropriately, the bot creates a more engaging and empathetic user experience.
Building this bot taught me a lot about integrating machine learning models into real-world applications. I hope this blog inspires you to explore the intersection of AI and chatbot development!
Source Code
If you're interested in exploring the full source code of this project, you can find it on GitHub:
GitHub Repository: Emotion-Detector-Telegram-Bot
Feel free to clone the repository, contribute, or use it as a reference for your own projects!
Subscribe to my newsletter
Read articles from Rishabh Raj Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
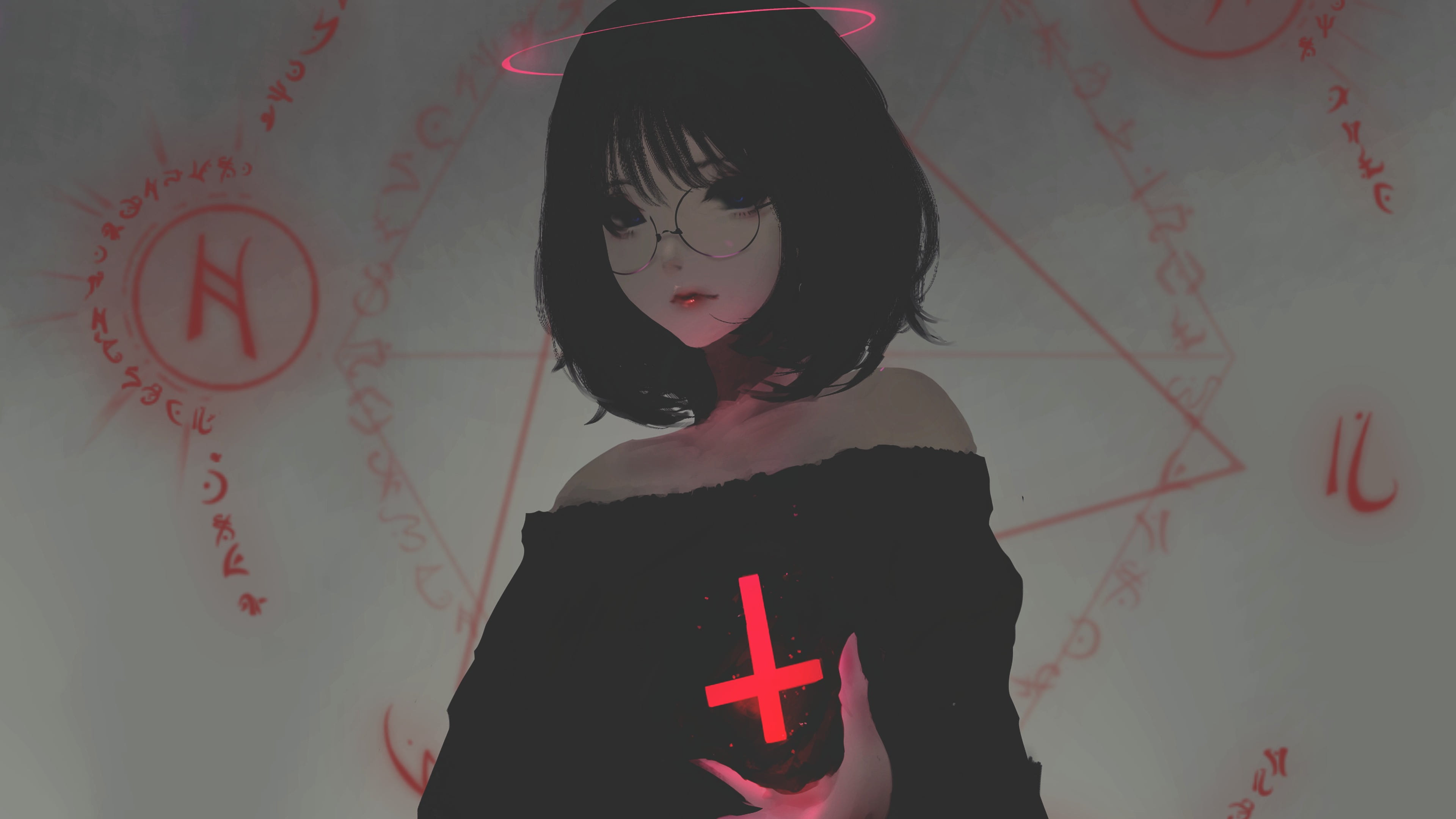
Rishabh Raj Verma
Rishabh Raj Verma
I’m a Full-Stack Developer skilled in the MERN stack with a strong understanding of Machine Learning. I enjoy building responsive web applications and using AI to solve problems. I’m always excited to learn new technologies and apply them to create innovative solutions.