Week 2 of My DevOps Journey: Advanced Git, Shell Scripting, and Linux Mastery
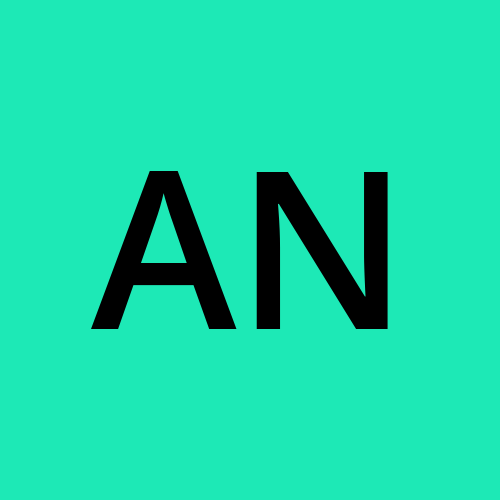
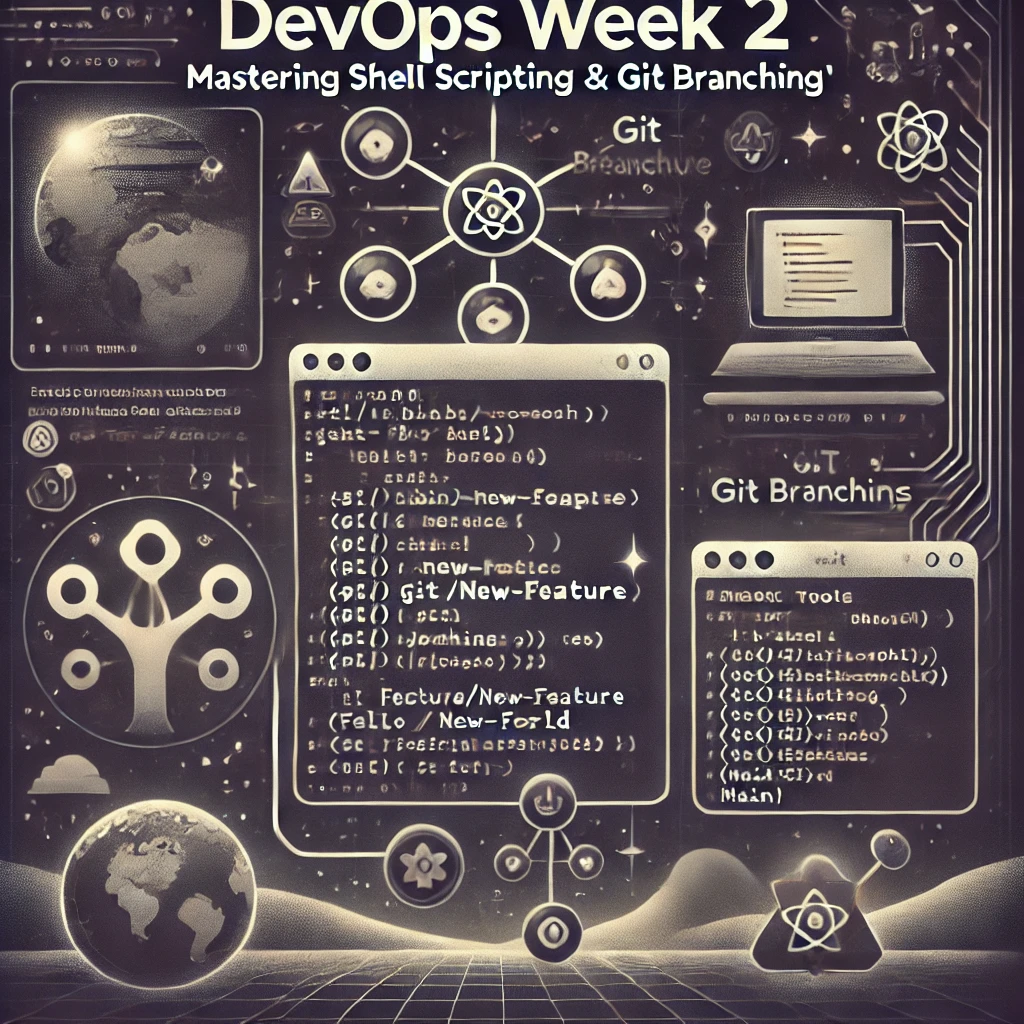
Introduction
Continuing my journey into the world of DevOps, Week 2 was all about deepening my understanding of shell scripting, mastering Git branching strategies, and preparing for real-world applications of these tools. Following Abhishek Veeramalla’s "DevOps Zero to Hero" playlist on YouTube has been immensely rewarding. Here’s a summary of my progress and the key concepts I’ve mastered.
Learning Shell Scripting Basics
One of the highlights this week was advancing through the "Learning Linux Shell Scripting" course. I covered essential topics like control structures, environment variables, and the creation of functions. Here’s a brief overview of the chapters I completed:
Chapter 1: Basics of Shell Scripting
Topics covered include printing to the console, changing file permissions with
chmod
, and using the shebang (#!/bin/bash
).Example: A simple script to print a message to the console.
#!/bin/bash echo "Hello, DevOps World!"
Chapter 2: Control Structures
Learned how to use
if
,else
,elif
statements, and loops (for
,while
) to control the flow of scripts.Example: Using an
if
statement to check if a file exists.if [ -f /path/to/file ]; then echo "File exists." else echo "File does not exist." fi
Chapter 3: Environment Variables
Explored how to read and manipulate environment variables in scripts.
Example: Accessing the
$USER
environment variable.echo "The current user is $USER"
Chapter 4: Functions
Focused on writing reusable code blocks using functions, including passing parameters.
Example: A function to display disk usage.
disk_usage() { df -h } disk_usage
Chapter 5: File Operations
Delved into reading from and writing to files using shell scripts and understanding file checksums for verifying data integrity.
Example: Using the
sha256sum
command to generate a file checksum.sha256sum filename.txt
Chapter 6: Managing Processes
Learned how to manage processes, including using the
sleep
command and running scripts in the background.Example: A script that pauses for a specified time before continuing.
#!/bin/bash sleep 10 echo "Waited for 10 seconds."
Chapter 7: Interactive Scripts
Explored creating interactive scripts that prompt users for input and handle errors gracefully. A fun project in this chapter was creating a simple guessing game.
Example: A script that prompts the user to guess a number.
#!/bin/bash COMPUTER=49 while true; do read -p "Guess the number: " USER if [ $USER -eq $COMPUTER ]; then echo "Congratulations! You guessed it right!" break elif [ $USER -lt $COMPUTER ]; then echo "Too low!" else echo "Too high!" fi done
Advanced Git and Branching Strategies
Understanding Git branching strategies is pivotal for any DevOps engineer. This week, I delved into advanced concepts of Git, particularly how branching works in complex projects like Kubernetes.
Branching Strategy: Learned how to use feature branches, release branches, and hotfix branches to manage development workflows.
Practical Example: Applied these concepts in a simulated project environment to see how branches like
feature/new-feature
,release/v1.0
, andhotfix/urgent-fix
can be managed effectively.Example: Creating and merging a feature branch.
git checkout -b feature/new-feature # Make changes git add . git commit -m "Added new feature" git checkout main git merge feature/new-feature
Interview Prep: I also went through common Git interview questions and practiced answering them to solidify my understanding.
Shell Scripting Projects: GitHub API Integration
One of the practical projects I worked on involved integrating shell scripts with the GitHub API. This project taught me how to automate the retrieval of user access information from GitHub repositories, a common task for DevOps engineers managing multiple repositories.
Project Overview: The script lists all users with access to a specific GitHub repository, which is useful for auditing and managing permissions.
Code Snippet:
#!/bin/bash repo="your-repo-name" curl -H "Authorization: token $GITHUB_TOKEN" \ https://api.github.com/repos/your-username/$repo/collaborators
Key Takeaways
Advanced Shell Scripting: Gained a deeper understanding of shell scripting, focusing on automation, environment management, and reusable code blocks.
Git Mastery: Learned to implement and manage complex Git branching strategies, ensuring smooth collaboration in development projects.
Certification: Completed a certification course on "Learning Linux Shell Scripting".
Next Steps
Looking ahead, I’m excited to dive into the following topics in the coming weeks
Ansible: Automating configuration management and application deployment.
Terraform: Infrastructure as code (IaC) to manage and provision cloud resources.
Jenkins: Setting up CI/CD pipelines to automate software builds, testing, and deployment processes.
These tools are vital for modern DevOps practices, and I look forward to integrating them into my workflow.
Connect with Me
GitHub Repository: DevOps Progress
LinkedIn Profile: Adarsh N
Resources
Subscribe to my newsletter
Read articles from Adarsh N directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
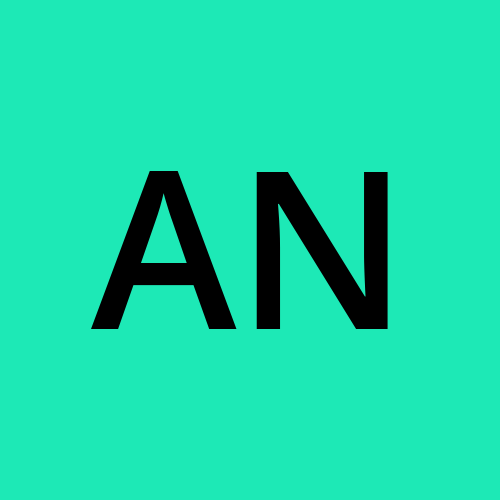
Adarsh N
Adarsh N
DevOps Enthusiast | Continuous Learner | Sharing My Journey Passionate about all things DevOps. Documenting my journey with tips and insights on automation, CI/CD, and cloud infrastructure.