Network Policies
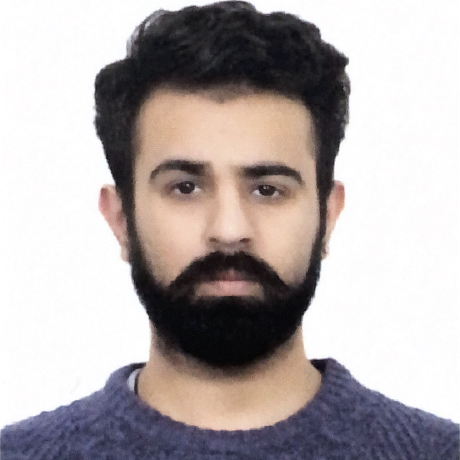
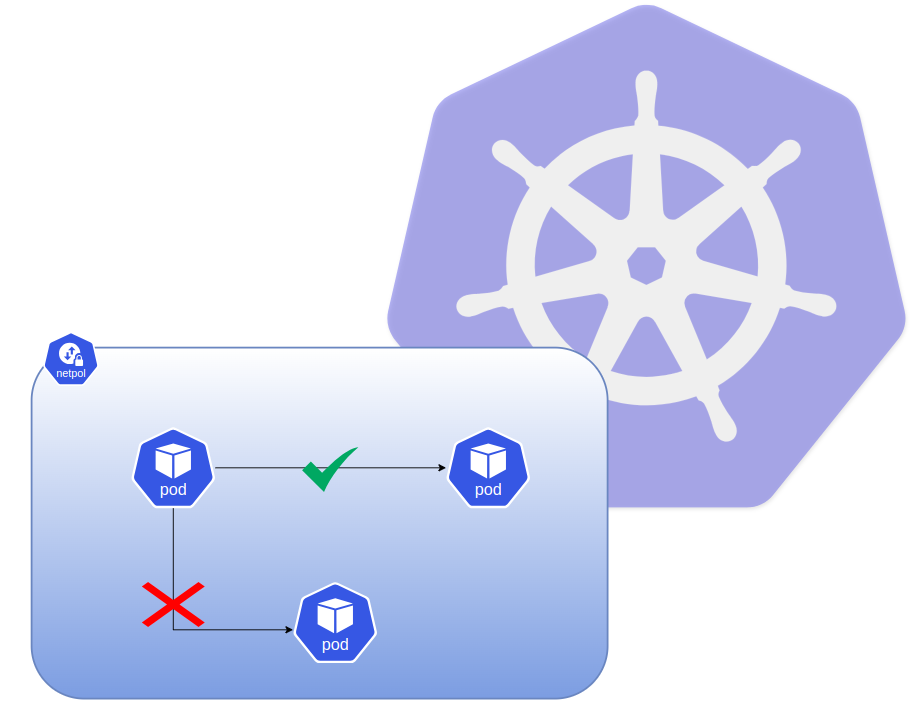
What are Kubernetes Network Policies?
Network Policies are a mechanism for controlling network traffic flow in Kubernetes clusters. They allow you to define which of your Pods are allowed to exchange network traffic.
Think of Kubernetes Network Policies as traffic cops for your Kubernetes cluster. They set the rules for how data can flow between different parts of your cluster. Just like a traffic cop controls the flow of cars, Network Policies control the flow of network traffic between your applications.
How do they work?
Define Rules: You create a Network Policy object that specifies the rules for a particular set of Pods. These rules can include:
Ingress: Who can talk to these Pods?
Egress: Who can these Pods talk to?
Pod Selector: Which Pods does this policy apply to?
Enforcement: Kubernetes enforces these rules by inspecting every packet of network traffic and checking if it complies with the defined policies.
Each Network Policy you create targets a group of Pods and sets the Ingress (incoming) and Egress (outgoing) network endpoints those Pods can communicate with.
There are three different ways to identify target endpoints:
You can also check the official documentation -> Kubernetes Network Policy
Specific Pods (Pods matching a label are allowed)
Specific Namespaces (all Pods in the namespace are allowed)
IP address blocks (endpoints with an IP address in the block are allowed)
Imagine your Kubernetes cluster as a city. Different parts of the city (Pods) need to communicate with each other, but you want to make sure they're only talking to the right people. That's where Kubernetes Network Policies come in. They're like traffic cops that set the rules for how data can move around in your cluster.
Here are some examples of how Network Policies can be used:
Protecting Databases: You can use Network Policies to make sure a database Pod can only be accessed by the app that needs it. This is like saying, "Only this specific app can talk to the bank."
Isolating Sensitive Pods: Some Pods might contain very important or sensitive data. You can use Network Policies to block all incoming traffic to these Pods, making them like a private room in a public building.
Controlling Communication Between Apps: If you have different apps running in different parts of your cluster, you can use Network Policies to decide which apps can talk to each other. This is like setting up rules for who can visit different neighborhoods in the city.
When you start using Kubernetes, your Pods can talk to any other Pod by default. This is like having an open door policy for your network traffic.
However, if you want to control who can talk to your Pods, you can create Kubernetes Network Policies. These policies act as rules for network traffic. If you have a policy for a Pod, only the traffic explicitly allowed in that policy can reach it. Think of it as a bouncer at a club, only letting in people on the guest list.
Ingress network policies allows inbound traffic from other pods.
Network policies apply to pods within a specific namespace. Policies can include one or more ingress rules. To specify which pods in the namespace the network policy applies to, use a pod selector. Within the ingress rule, use another pod selector to define which pods allow incoming traffic, and the ports field to define on which ports traffic is allowed.
Allow ingress traffic from pods in the same namespace.
In this following example , incoming traffic to pods with label color=blue are ally only if they come from a pod with color=red on port 80 in the same namespace.
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: ingress-allow-same-ns
namespace: blue
spec:
podSelector:
matchLabels:
color: blue
ingress:
- from:
- podSelector:
matchLabels:
color: red
ports:
- port: 80
Allow ingress traffic from pods in a different namespace
Now to allow traffic from the pods in a different namespace , one have to use the namespace selector in the ingress policy rule. The namespace selector matches one or more Kubernetes namespaces and is combined with pod selector that selects within those namespaces.
In this following example, the incoming traffic is only allowed if it is coming from a pod with a label color=red that is in the namespace labelled env=red , on port
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: ingress-allow-diff-ns
namespace: blue
spec:
podSelector:
matchLabels:
color: blue
ingress:
- from:
- podSelector:
matchLabels:
color: red
namespaceSelector:
matchLabels:
env: red
ports:
- port: 80
Egress policies allows outbound traffic from the pods.
Egress policies in Kubernetes control which external resources your pods can communicate with. Think of them as a gatekeeper that determines who your pods can talk to outside of your cluster. By defining egress policies, you can prevent your pods from accidentally connecting to unauthorized or malicious external resources.
Allow egress traffic to the pods in the same namespace
In this following example , this egress policy allows pod to outbound traffic to other pods in the same namespace that matches the pod selector.The outbound traffic is allowed only if they go to a pod with label color=red on port 80.
apiVersion: networking k8s.io/v1
kind: NetworkPolicy
metadata:
name: egress-allow-same-ns
namespace: blue
spec:
podSelector:
matchLabels:
color: blue
egress:
- to:
- podSelector:
matchLabels:
color: red
ports:
- port: 80
Allow egress traffic to the pods in the different namespace
In this example the outbound traffic is only allowed if they go to pod with label color=red located in namespace labelled env=red on port 80.
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: egress-allow-diff-ns
namespace: blue
spec:
podSelector:
matchLabels:
color: blue
egress:
- to:
- podSelector:
matchLabels:
color: red
namespaceSelector:
matchLabels:
env: red
ports:
- port: 80
Allow egress traffic to ip addresses or cidr range
In this example it is shown that the Egress policies can also be used to allow traffic to specific IP addresses and CIDR ranges. Typically , IP addresses/ranges are used to handle traffic that is external to the cluster.
Here, the egress policy allows outbound traffic to any IP address within the CIDR block 10.0.1.0/24.
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: egress-allow-cidr
namespace: blue
spec:
podSelector:
matchLabels:
color: blue
egress:
- to:
- ipBlock:
cidr: 10.0.1.0/24
ports:
- port: 80
Creating a Default Deny-All Network Policy
Why a Default Deny-All Policy?
In Kubernetes, it's often considered best practice to create a default network policy that denies all traffic by default. This helps ensure that your applications are not accidentally exposed to unauthorized access. If you don't have a default deny-all policy, any pod in a namespace can communicate freely with all other pods unless you explicitly define a policy to restrict traffic.
Implementing a Default Deny-All Policy
Here's a sample network policy that implements a default deny-all ingress and egress policy for the policy-demo
namespace:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: deny-all
namespace: policy-demo
spec:
podSelector: {}
ingress: []
egress: []
Explanation:
podSelector: {}
: This selects all pods in thepolicy-demo
namespace.ingress: []
: This explicitly denies all incoming traffic.egress: []
: This explicitly denies all outgoing traffic.
How It Works:
By default, when a network policy applies to a pod, only the traffic explicitly allowed by the policy is permitted. Since this policy doesn't allow any traffic, all traffic to and from pods in the policy-demo
namespace is denied unless there are other policies that explicitly allow specific traffic.
Additional Considerations:
Overriding the Default: If you want to allow specific traffic for certain pods, you can create additional network policies that explicitly allow the desired traffic. These policies will override the default deny-all policy for the affected pods.
Namespace-Specific Policies: You can create default deny-all policies for each namespace to enforce different security rules for different groups of applications.
By implementing a default deny-all network policy, you can help ensure that your Kubernetes applications are protected from unauthorized access and that you have granular control over network traffic within your clusters.
Subscribe to my newsletter
Read articles from Aditya Tanwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
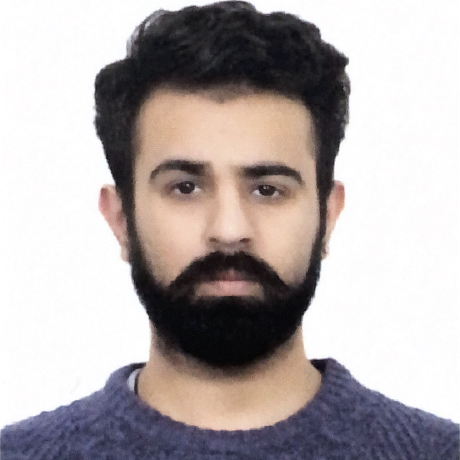