Implementing Calculated Columns in MongoDB: Techniques and Best Practices
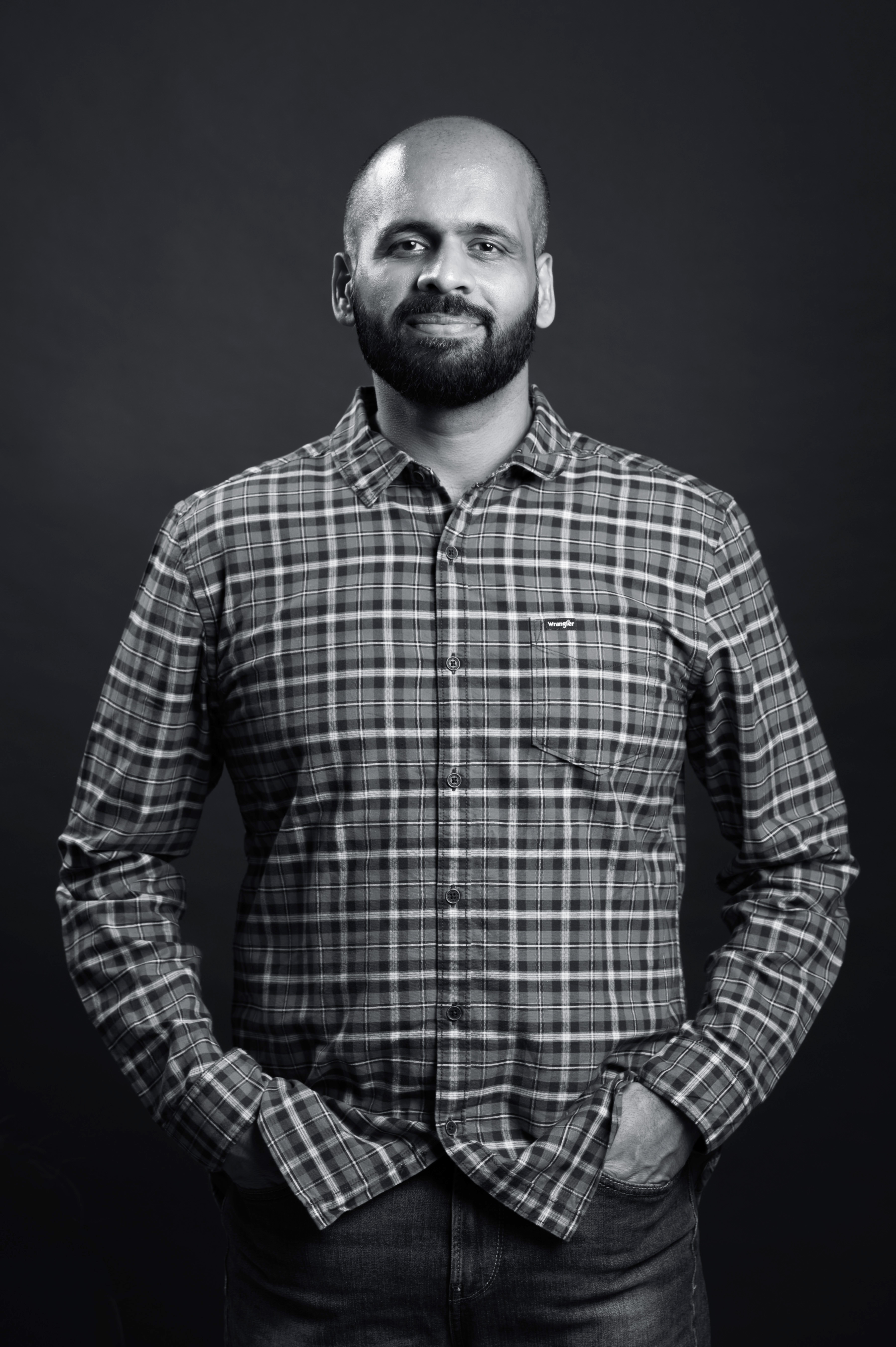
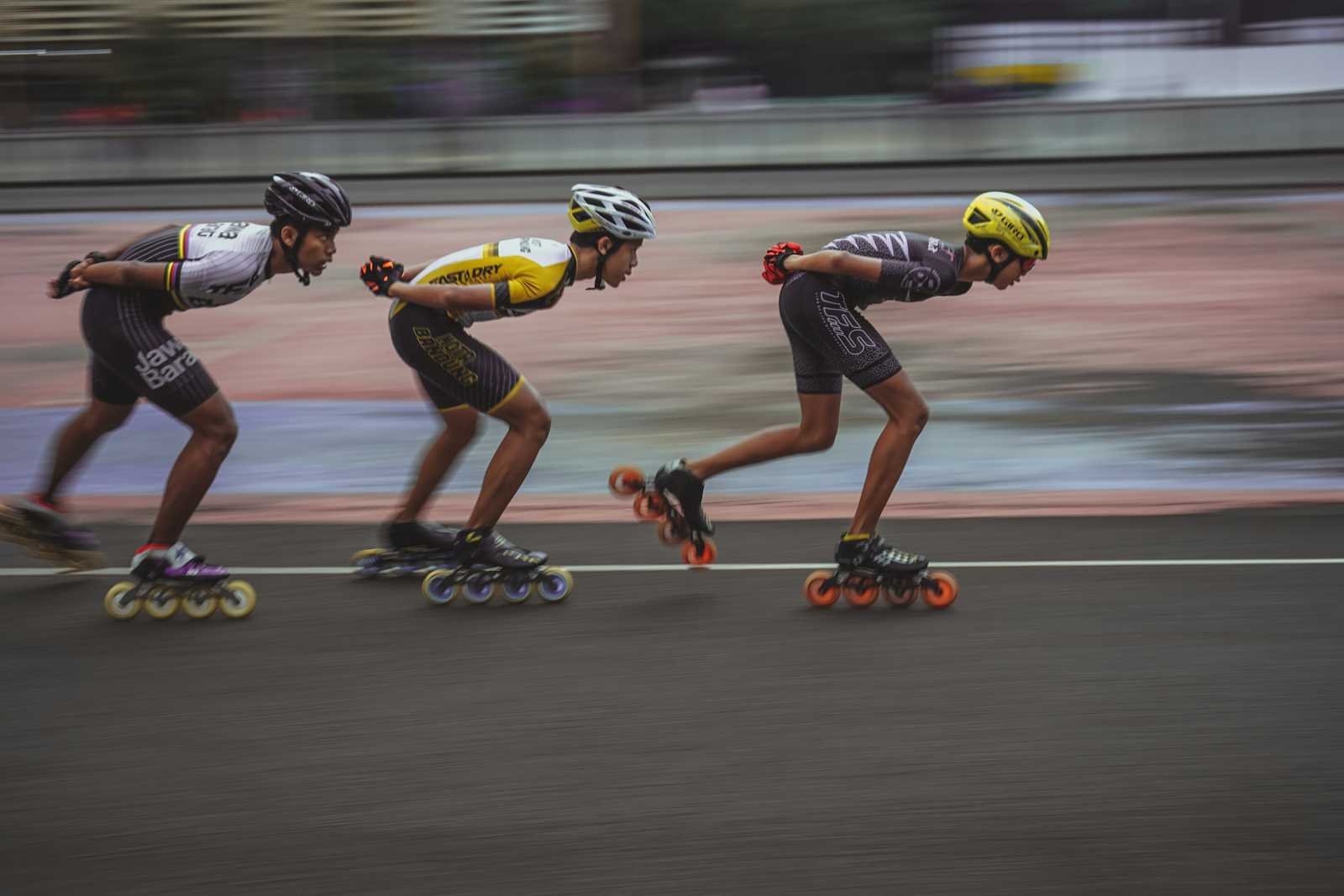
In MongoDB, calculated or derived columns (sometimes referred to as computed fields) are not natively supported as part of the schema like in traditional relational databases. However, you can implement calculated fields using several techniques, depending on your needs. Here are the most common approaches:
1. Aggregation Framework
The MongoDB Aggregation Framework allows you to compute fields on the fly during a query. This method is suitable for scenarios where you need to calculate values dynamically without storing them in the database.
Example:
Let's assume you have a sales
collection with quantity
and price
fields, and you want to calculate the total
as quantity * price
.
{
"_id": 1,
"item": "apple",
"quantity": 10,
"price": 0.5
}
You can calculate the total
using the $addFields
stage in an aggregation pipeline:
db.sales.aggregate([
{
$addFields: {
total: { $multiply: ["$quantity", "$price"] }
}
}
])
This query will output documents with an additional total
field that is the result of quantity * price
.
2. Computed Fields in Queries
You can calculate fields directly in query projections using the $project
stage. This approach is useful for one-off queries where you need a calculated value without altering the database schema.
Example:
db.sales.aggregate([
{
$project: {
item: 1,
quantity: 1,
price: 1,
total: { $multiply: ["$quantity", "$price"] }
}
}
])
This will return a result set that includes the calculated total
field along with the original fields.
3. Using Update Operations with $set
In cases where you need to persist calculated values in the database, you can use the $set
update operator to store the result of a calculation in a new field.
Example:
To add a total
field to each document based on the multiplication of quantity
and price
:
db.sales.updateMany(
{},
[
{ $set: { total: { $multiply: ["$quantity", "$price"] } } }
]
)
This operation will update all documents in the sales
collection by adding a total
field.
4. Precomputed Fields
If performance is critical and recalculating fields on the fly is too costly, you can precompute and store calculated values when documents are inserted or updated. This can be done within your application logic or by using MongoDB triggers such as Change Streams combined with an external processing service.
Example:
When inserting a document:
db.sales.insertOne({
item: "apple",
quantity: 10,
price: 0.5,
total: 10 * 0.5
})
Here, the total
field is calculated before the document is inserted.
5. Using MongoDB Views
MongoDB Views allow you to create virtual collections with computed fields by defining them as an aggregation pipeline on an existing collection. This is a read-only solution, useful for creating calculated fields that are frequently needed.
Example:
db.createView(
"salesWithTotal",
"sales",
[
{
$addFields: {
total: { $multiply: ["$quantity", "$price"] }
}
}
]
)
You can query the salesWithTotal
view just like a regular collection, and it will return documents with the total
field computed on the fly.
Conclusion
While MongoDB doesn't support calculated columns in the traditional sense, these methods allow you to effectively implement and work with computed fields, depending on your use case. Whether you choose to calculate fields dynamically, persist them for performance, or use views for convenience, MongoDB provides flexible tools to meet your needs.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
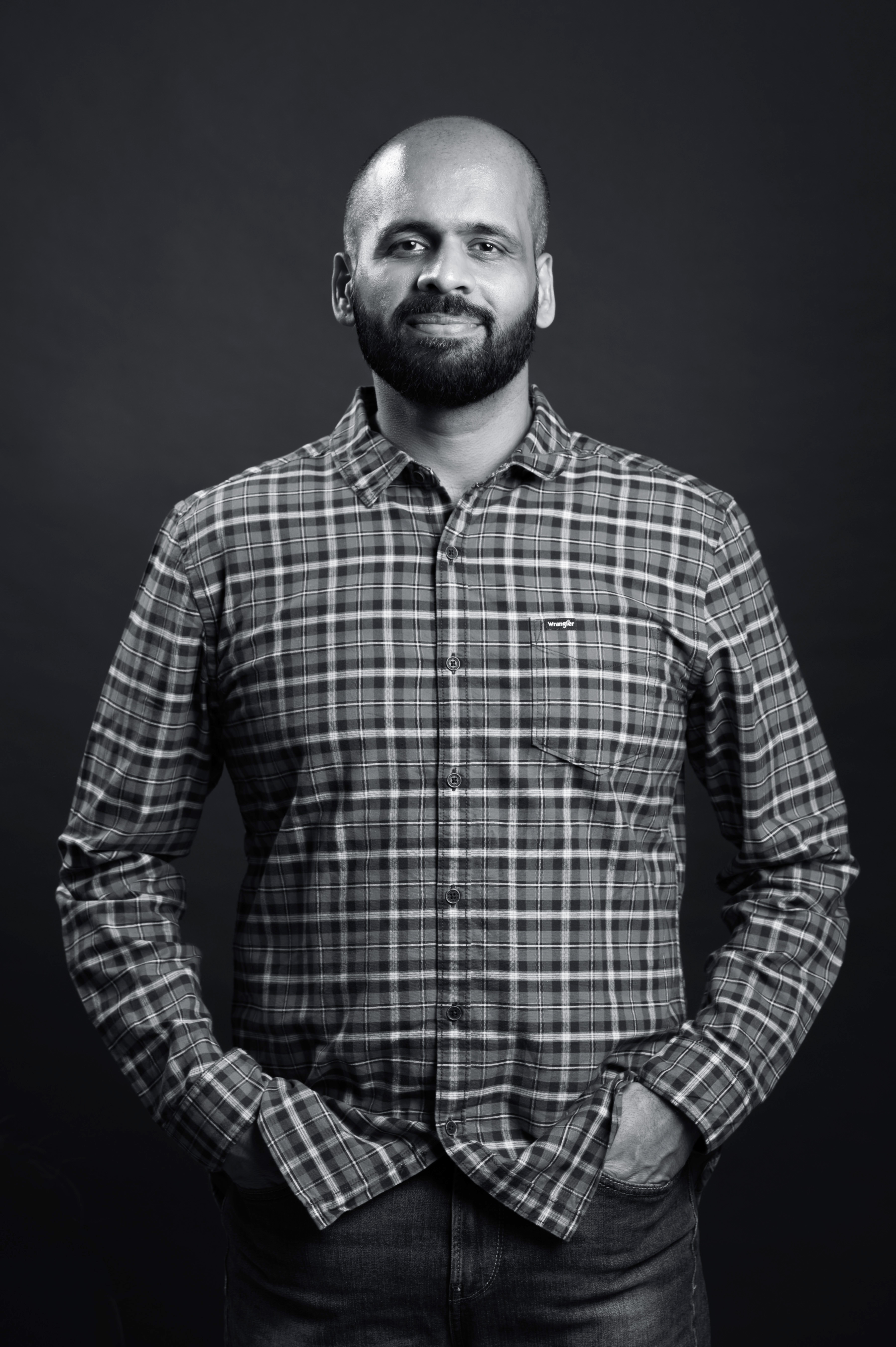
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.