For Loops in Python
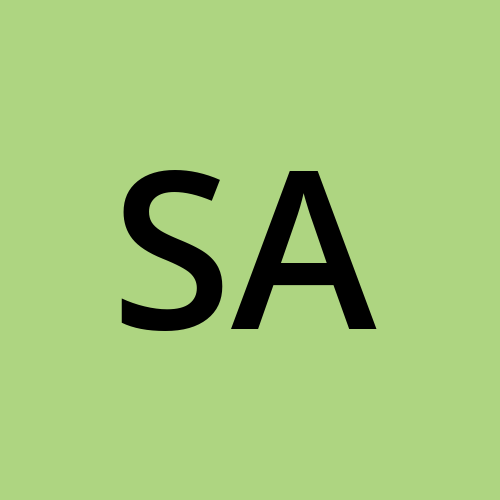
Table of contents
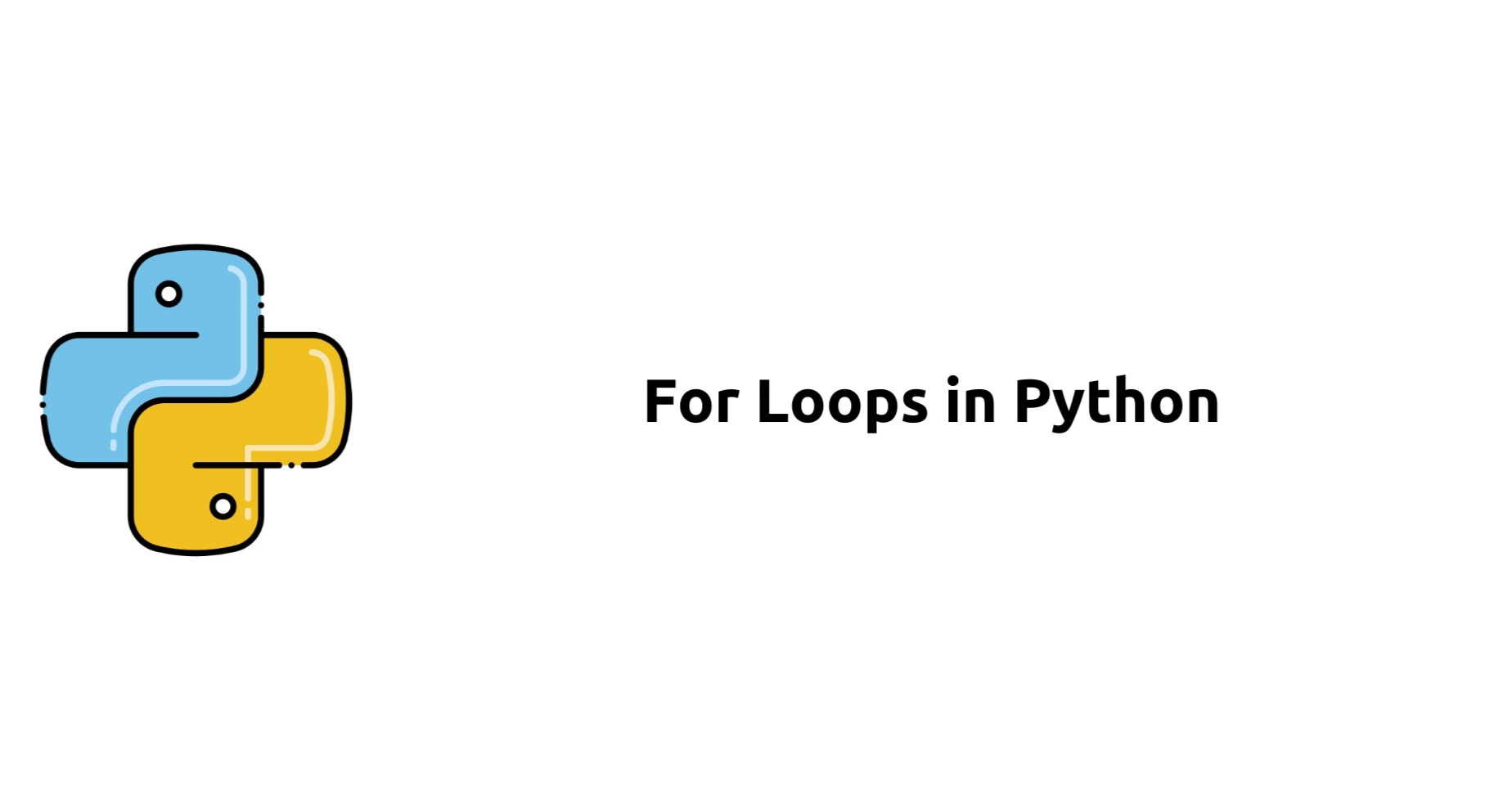
Introduction
In the realm of DevOps automation, efficient iteration and processing of data are essential for managing complex infrastructure and streamlining deployment workflows. For loops, a fundamental control structure in Python, offer a powerful mechanism for iterating over collections of data, executing tasks iteratively, and automating repetitive operations. In this comprehensive guide, we'll explore the versatility of for loops, uncover practical use cases for DevOps engineers, delve into their advantages, and provide insights into optimizing their usage for efficient automation.
Understanding For Loops in Python
What are For Loops? A for loop in Python is used to iterate over a sequence (such as a list, tuple, or string) and perform operations on each element sequentially. It allows DevOps engineers to execute a block of code repeatedly for each item in the sequence, facilitating efficient data processing and automation.
Syntax of For Loop: The syntax of a for loop in Python is as follows:
for item in sequence:
# Execute code block for each item
# Indentation is crucial to denote the block of code
print(item)
Advantages of Using For Loops in DevOps
Efficient Data Processing: For loops enable DevOps engineers to iterate over large datasets efficiently, processing each element sequentially without manual intervention.
Automation of Repetitive Tasks: By automating repetitive tasks, for loops reduce manual effort and enhance productivity in DevOps workflows, such as configuration management and deployment.
Scalability: For loops scale seamlessly with the size of the dataset, allowing DevOps engineers to handle diverse scenarios, from managing a few servers to orchestrating complex infrastructures.
Versatility: For loops can iterate over various types of sequences, including lists, tuples, dictionaries, and strings, providing flexibility in data processing and automation.
Practical Use Cases for DevOps
Use Case 1: Server Configuration Management
For loops can be used to iterate over a list of server IP addresses, applying configuration changes or executing commands on each server in a batch.
servers = ['10.0.0.1', '10.0.0.2', '10.0.0.3']
for server in servers:
configure_server(server)
Use Case 2: Deployment Pipeline Automation
In a deployment pipeline, for loops can iterate over a list of deployment tasks, executing deployment scripts or triggering actions for each task in the pipeline.
deployment_tasks = ['Deploy App 1', 'Deploy App 2', 'Deploy App 3']
for task in deployment_tasks:
execute_deployment(task)
Use Case 3: Log Analysis and Monitoring
For loops can iterate over log files or monitoring data, analyzing each entry for anomalies, errors, or performance metrics in real-time.
log_files = ['access.log', 'error.log', 'system.log']
for logfile in log_files:
analyze_logs(logfile)
Best Practices for Optimizing For Loops
Iterating Directly Over Sequences: Instead of using range-based loops, iterate directly over sequences whenever possible to simplify code and improve readability.
Avoiding Nested Loops: Minimize the use of nested for loops to prevent performance bottlenecks, especially when dealing with large datasets.
Optimizing Data Structures: Choose appropriate data structures for iteration to optimize performance and memory usage, such as lists, sets, or generators.
Utilizing Built-in Functions: Leverage built-in functions like
enumerate()
,zip()
, andfilter()
to enhance the functionality of for loops and streamline data processing.
Conclusion
For loops are indispensable tools in the arsenal of DevOps engineers, empowering them to automate repetitive tasks, process data efficiently, and orchestrate complex workflows with ease. By leveraging the advantages of for loops and adhering to best practices for optimization, DevOps professionals can enhance the efficiency, reliability, and scalability of their automation scripts. Through practical use cases and examples tailored to the DevOps perspective, this guide has highlighted the versatility and utility of for loops in DevOps automation. As you continue your journey in DevOps, harness the power of for loops to unlock new possibilities, streamline infrastructure management, and propel your automation efforts to greater heights.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
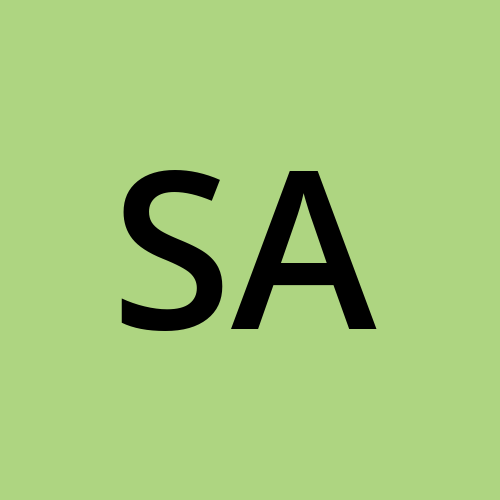
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.