Day-1 creating a UI for my Home Service App
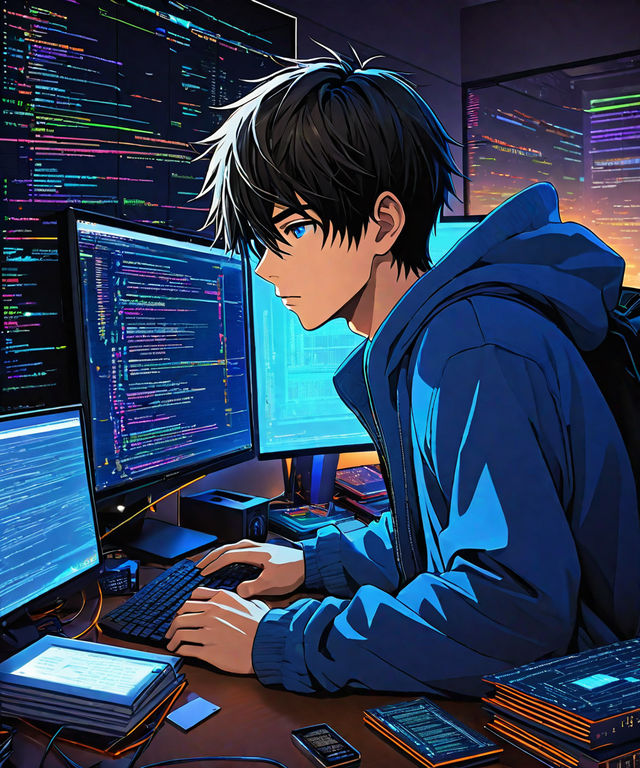
4 min read
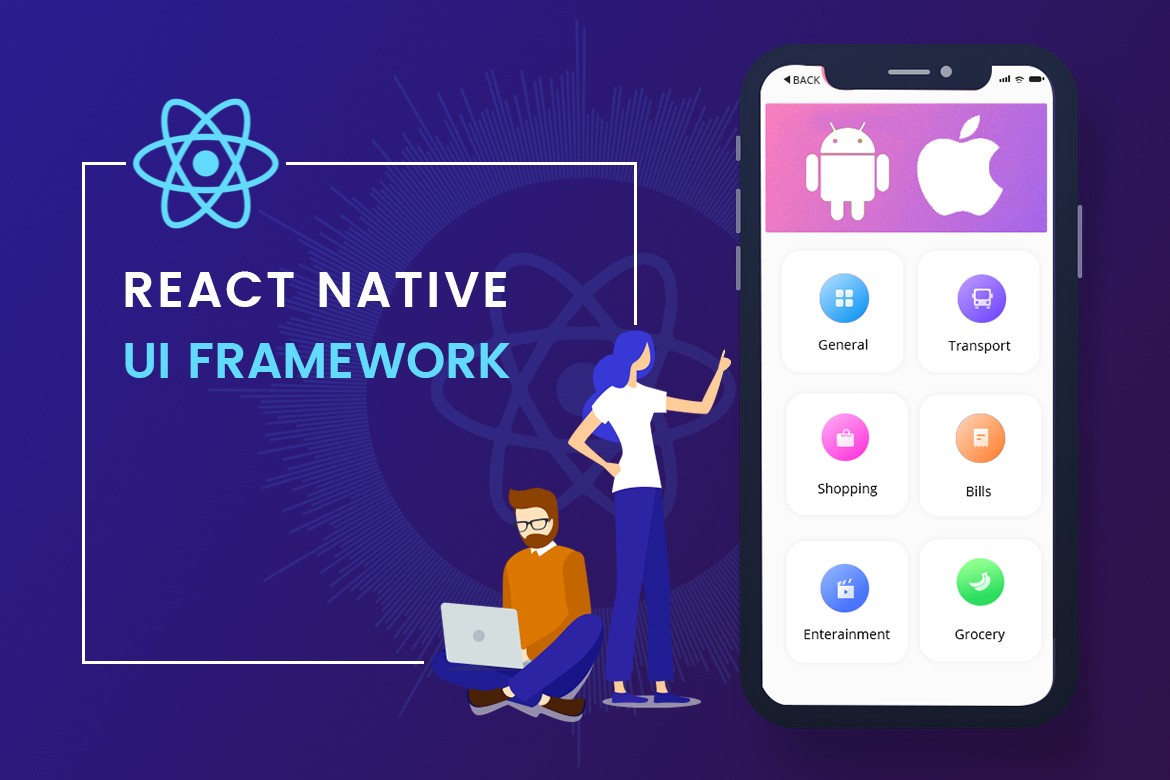
React-Native
import React from "react";
import {
Text,
View,
Image,
Pressable,
Dimensions,
PixelRatio,
ScrollView,
} from "react-native";
import Octicons from "@expo/vector-icons/Octicons";
import Ionicons from "@expo/vector-icons/Ionicons";
import FontAwesome6 from "@expo/vector-icons/FontAwesome6";
import Entypo from "@expo/vector-icons/Entypo";
const { width, height } = Dimensions.get("window");
const responsiveWidth = (percent) => {
return PixelRatio.roundToNearestPixel((width * percent) / 100);
};
const responsiveHeight = (percent) => {
return PixelRatio.roundToNearestPixel((height * percent) / 100);
};
const index = () => {
return (
<ScrollView style={{ flex: 1 }}>
<View
style={{
padding: responsiveWidth(3),
height: responsiveHeight(25),
backgroundColor: "lightgray",
}}
>
<View
style={{
flexDirection: "row",
alignItems: "center",
justifyContent: "space-between",
}}
>
<Image
style={{
width: responsiveWidth(50),
height: responsiveHeight(6),
resizeMode: "cover",
}}
source={{
uri: "https://i.postimg.cc/cJkrx4T3/Metro-Mate-Photoroom.png",
}}
/>
<Octicons name="three-bars" size={responsiveWidth(6)} color="black" />
</View>
<View
style={{
marginTop: responsiveHeight(2.5),
flexDirection: "row",
alignItems: "center",
justifyContent: "space-between",
}}
>
<View>
<Text
style={{
fontSize: responsiveWidth(3.5),
fontWeight: "bold",
}}
>
Hi Kisan!
</Text>
<Text
style={{
marginTop: responsiveHeight(0.5),
fontWeight: "bold",
color: "#0066b3",
}}
>
Home | Surat - 394210
</Text>
</View>
<View
style={{
flexDirection: "row",
alignItems: "center",
gap: responsiveWidth(1),
}}
>
<Ionicons
name="information-circle-outline"
size={responsiveWidth(6)}
color="black"
/>
<Text
style={{
width: responsiveWidth(15),
fontSize: responsiveWidth(3),
color: "#0066b3",
fontWeight: "bold",
}}
>
ANY HELP
</Text>
</View>
</View>
</View>
<View
style={{
padding: responsiveWidth(2),
backgroundColor: "white",
width: responsiveWidth(86),
marginLeft: "auto",
marginRight: "auto",
borderRadius: 10,
position: "absolute",
top: responsiveHeight(18),
left: "50%",
transform: [{ translateX: -responsiveWidth(42.5) }],
}}
>
<View
style={{
flexDirection: "row",
gap: responsiveWidth(2.5),
}}
>
<Ionicons
name="notifications-outline"
size={responsiveWidth(6)}
color="black"
/>
<View>
<Text
style={{
fontSize: responsiveWidth(4),
color: "#0066b3",
fontWeight: "bold",
}}
>
QUICK SERVICES
</Text>
<Text
style={{
marginTop: responsiveHeight(0.5),
fontSize: responsiveWidth(3.2),
}}
>
Book your service with wide variety
</Text>
<Text
style={{
marginTop: responsiveHeight(0.5),
fontSize: responsiveWidth(3.2),
}}
>
We will be at your doorstep on time
</Text>
<View
style={{
flexDirection: "row",
alignItems: "center",
justifyContent: "center",
marginTop: responsiveHeight(1),
gap: responsiveWidth(1),
}}
>
<FontAwesome6
name="truck-arrow-right"
size={responsiveWidth(6)}
color="#034694"
/>
<Pressable
style={{
backgroundColor: "#0066b3",
paddingHorizontal: responsiveWidth(2.5),
paddingVertical: responsiveHeight(0.5),
borderRadius: 4,
}}
>
<Text
style={{
fontSize: responsiveWidth(4),
fontWeight: "bold",
color: "white",
}}
>
BOOK NOW
</Text>
</Pressable>
</View>
</View>
</View>
</View>
<View
style={{
marginTop: responsiveHeight(11),
marginHorizontal: responsiveWidth(3),
flexDirection: "row",
alignItems: "center",
justifyContent: "space-evenly",
gap: responsiveWidth(2.5),
}}
>
<View
style={{
backgroundColor: "white",
padding: responsiveWidth(2),
borderRadius: 10,
}}
>
<View
style={{
flexDirection: "row",
alignItems: "center",
gap: responsiveWidth(2),
}}
>
<View>
<Text
style={{
color: "#0066b3",
fontSize: responsiveWidth(3.4),
fontWeight: "bold",
}}
>
Ultimate <Text style={{ color: "gray" }}>Club</Text>
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Just book your service
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Subscribe and get the benefits
</Text>
</View>
<Entypo
name="triangle-right"
size={responsiveWidth(6)}
color="#0066b3"
/>
</View>
</View>
<View
style={{
backgroundColor: "white",
padding: responsiveWidth(2),
borderRadius: 10,
}}
>
<View
style={{
flexDirection: "row",
alignItems: "center",
gap: responsiveWidth(2),
}}
>
<Ionicons
name="basket-outline"
size={responsiveWidth(6)}
color="#0066b3"
/>
<View>
<Text
style={{
color: "#0066b3",
fontSize: responsiveWidth(3.4),
fontWeight: "bold",
}}
>
Place Your <Text style={{ color: "#0066b3" }}>Order</Text>
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Select Services from the catagory below,
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
and book your service. It's about time.
</Text>
</View>
</View>
</View>
</View>
<View
style={{
marginTop: responsiveHeight(2),
marginHorizontal: responsiveWidth(2),
flexDirection: "column",
alignItems: "flex-start",
justifyContent: "center",
gap: responsiveWidth(2.5),
left: "1.5%",
}}
>
<View
style={{
backgroundColor: "white",
padding: responsiveWidth(1.8),
borderRadius: 10,
}}
>
<View
style={{
flexDirection: "row",
alignItems: "center",
gap: responsiveWidth(2),
}}
>
<View>
<Text
style={{
color: "#0066b3",
fontSize: responsiveWidth(3.4),
fontWeight: "bold",
}}
>
AFFORDABLE <Text style={{ color: "gray" }}>PRICE</Text>
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "500",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Get our list of price
</Text>
{/* <Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Subscribe and get the benefits
</Text> */}
</View>
<Entypo
name="triangle-right"
size={responsiveWidth(6)}
color="#0066b3"
/>
</View>
</View>
<View
style={{
backgroundColor: "white",
padding: responsiveWidth(2),
borderRadius: 10,
}}
>
<View
style={{
flexDirection: "row",
alignItems: "center",
gap: responsiveWidth(2),
}}
>
<View>
<Text
style={{
color: "#0066b3",
fontSize: responsiveWidth(3.4),
fontWeight: "bold",
}}
>
QUICK <Text style={{ color: "gray" }}>ASSISTANCE</Text>
</Text>
<Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "500",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Help from experts
</Text>
{/* <Text
style={{
fontSize: responsiveWidth(3),
fontWeight: "300",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(30),
}}
>
Subscribe and get the benefits
</Text> */}
</View>
<Entypo
name="triangle-right"
size={responsiveWidth(6)}
color="#0066b3"
/>
</View>
</View>
<View
style={{
backgroundColor: "white",
padding: responsiveWidth(2),
flexDirection: "row",
alignItems: "center",
borderRadius: 10,
position: "absolute",
top: 0.1,
left: "54%",
}}
>
<View>
<Text
style={{
color: "#0066b3",
fontSize: responsiveWidth(5),
fontWeight: "bold",
width: responsiveWidth(30),
}}
>
WHAT'S THE <Text style={{ color: "gray" }}>PLAN</Text>
</Text>
<Text
style={{
fontSize: responsiveWidth(5),
fontWeight: "bold",
width: responsiveWidth(30),
}}
>
FOR YOUR HOME
</Text>
</View>
<Entypo
name="triangle-right"
size={responsiveWidth(6)}
color="#0066b3"
/>
</View>
</View>
<View
style={{
marginTop: responsiveHeight(2),
backgroundColor: "white",
padding: responsiveWidth(2),
borderRadius: 10,
marginRight: "auto",
marginLeft: "auto",
}}
>
<View style={{ flexDirection: "row", alignItems: "center", gap: 5 }}>
<Ionicons
name="notifications-circle-sharp"
size={24}
color="#0066b3"
/>
<Text style={{ fontSize: responsiveWidth(3.8), fontWeight: "bold" }}>
Next Available
</Text>
</View>
<Text
style={{
fontSize: responsiveWidth(4),
fontWeight: "bold",
marginTop: responsiveHeight(0.5),
width: responsiveWidth(42),
color: "gray",
}}
>
Order within 10min to catch the{" "}
<Text style={{ color: "#0066b3" }}>Service </Text>slot
</Text>
<Pressable
style={{
backgroundColor: "#0066b3",
paddingHorizontal: responsiveHeight(1),
paddingVertical: responsiveWidth(1),
justifyContent: "center",
alignItems: "center",
borderWidth: 0.7,
width: responsiveWidth(40),
marginTop: responsiveHeight(1),
borderRadius: 10,
}}
>
<Text
style={{
color: "white",
fontWeight: "bold",
fontSize: responsiveWidth(3.5),
}}
>
ADD SERVICE
</Text>
</Pressable>
</View>
</ScrollView>
);
};
export default index;
Output:
A Responsive Design for all devices
0
Subscribe to my newsletter
Read articles from Kisan Majumdar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
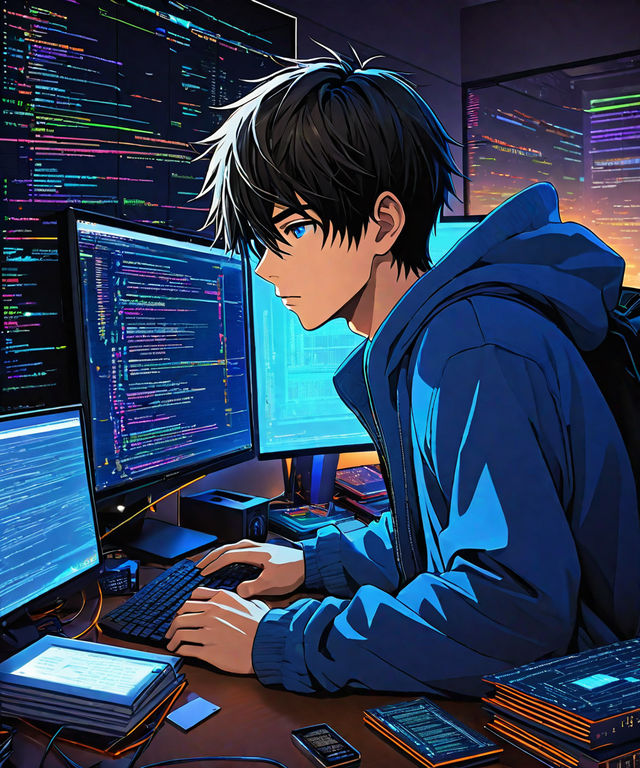
Kisan Majumdar
Kisan Majumdar
I am student pursuing btech computer engineering.Getting knowledge in web and app for building great projects and practice to improve my skills and consistency for creating some tech enthusiam blogs to.