Understanding Temporary and Permanent Redirects in Django

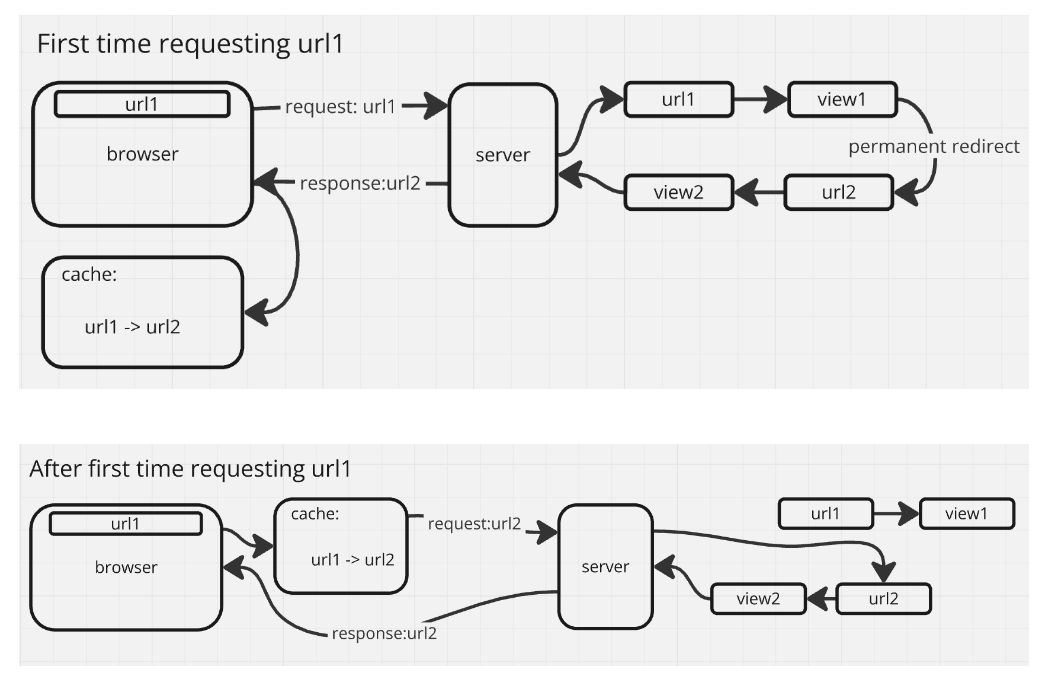
When building a web application, there may be times when you need to send users from one URL to another. This can happen for various reasons: a page has moved, a resource is temporarily unavailable, or you want to guide users to a new version of a page. In Django, this is easily done using the redirect
function from django.shortcuts
. However, the way you choose to redirect can have important implications, especially when it comes to search engine optimization (SEO) and user experience. This blog will explore the difference between temporary and permanent redirects, how to implement them in Django, and their impact on your website.
What is a Redirect?
A redirect is a way to send both users and search engines to a different URL from the one they originally requested. Think of it as a signpost that says, "The page you're looking for has moved—follow this new path instead."
In Django, you can create redirects using the redirect
function. This function allows you to specify where users should be sent and whether the redirect should be temporary or permanent.
Temporary Redirect (HTTP 302)
A temporary redirect tells browsers and search engines that the requested URL is temporarily unavailable, but it might be back in the future. In Django, this is the default behavior when you use the redirect
function without specifying the permanent
parameter or setting it to False
.
Example:
from django.shortcuts import redirect
def temporary_redirect_view(request):
return redirect('temporary-destination', permanent=False)
In this example, when a user visits the view associated with temporary_redirect_view
, they are temporarily redirected to temporary-destination
.
How It Affects SEO:
SEO Impact: Search engines understand that the original URL may come back, so they will continue to index it. However, the new URL may not receive full SEO benefits like link equity.
User Impact: Browsers don't cache the redirect, meaning users will be redirected every time they visit the original URL.
Permanent Redirect (HTTP 301)
A permanent redirect tells browsers and search engines that the requested URL has permanently moved to a new location. This is done in Django by setting the permanent
parameter to True
in the redirect
function.
Example:
from django.shortcuts import redirect
def permanent_redirect_view(request):
return redirect('permanent-destination', permanent=True)
In this example, when a user visits the view associated with permanent_redirect_view
, they are permanently redirected to permanent-destination
.
How It Affects SEO:
SEO Impact: Search engines understand that the original URL is no longer relevant and should be replaced with the new one in their index. The new URL inherits the SEO benefits of the old one, including link equity.
User Impact: Browsers cache the redirect, meaning users will be automatically sent to the new URL on subsequent visits without the need for another redirect.
When to Use Temporary vs. Permanent Redirects
Use a Temporary Redirect When:
The original page is temporarily down for maintenance.
You are testing a new page but plan to bring the old one back.
You want to redirect users based on certain conditions (e.g., mobile vs. desktop).
Use a Permanent Redirect When:
The old URL is obsolete, and the new URL will replace it permanently.
You are migrating your site to a new domain or structure.
You want to consolidate multiple URLs into one.
Conclusion
Choosing between a temporary and permanent redirect is not just a technical decision; it has implications for your site's SEO and user experience. Temporary redirects (HTTP 302) are ideal for situations where the change is short-term, while permanent redirects (HTTP 301) are better suited for long-term changes. By understanding the impact of each, you can make informed decisions that benefit both your users and your site's search engine rankings.
Whether you're restructuring your website, moving content, or managing short-term outages, understanding the nuances of redirects will ensure that your site continues to perform well both for users and in search engine results.
Subscribe to my newsletter
Read articles from Prakash Tajpuriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prakash Tajpuriya
Prakash Tajpuriya
Web Developer