Linux Shell Scripting #Day5
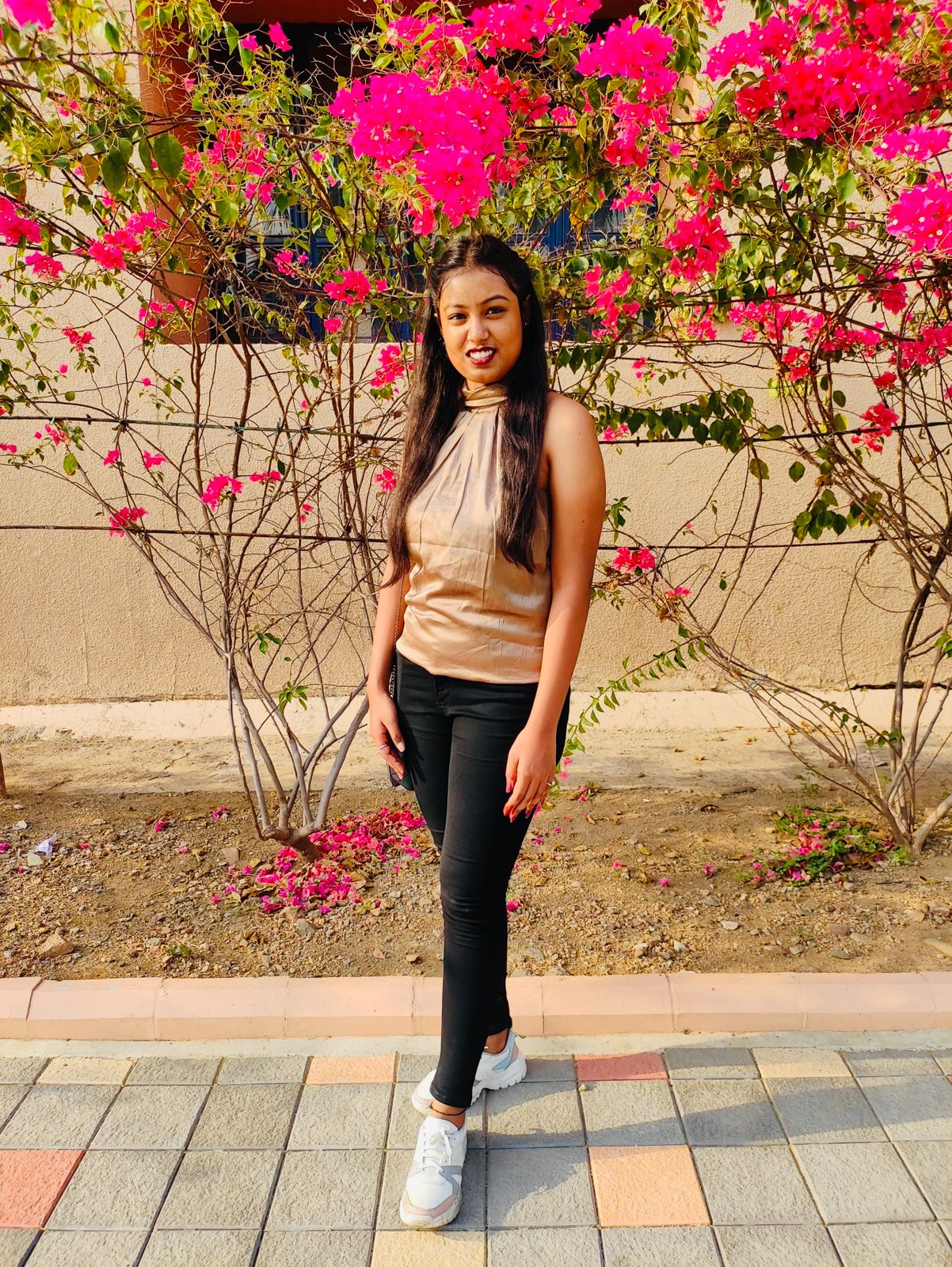
Table of contents
- Contents
- Why is Shell Scripting Important for DevOps? 🔄
- Basic Components of Shell Scripting 💻
- 1. Lightweight and Simple 🪶
- 2. Flexibility and Control 🎛️
- 3. Perfect for Small and Quick Tasks ⚡
- 4. Integration with Existing Tools 🔗
- 5. First Step in Automation Learning 🧑💻
- 6. Ubiquitous Across Systems 🌐
- 7. Immediate Feedback for Troubleshooting 🔍
- How to Get Started With Shell Scripting? 🛠️
- Popular Shell Scripting Languages 🗣️
- Shell Scripting FAQs ❓
- Conclusion 📝
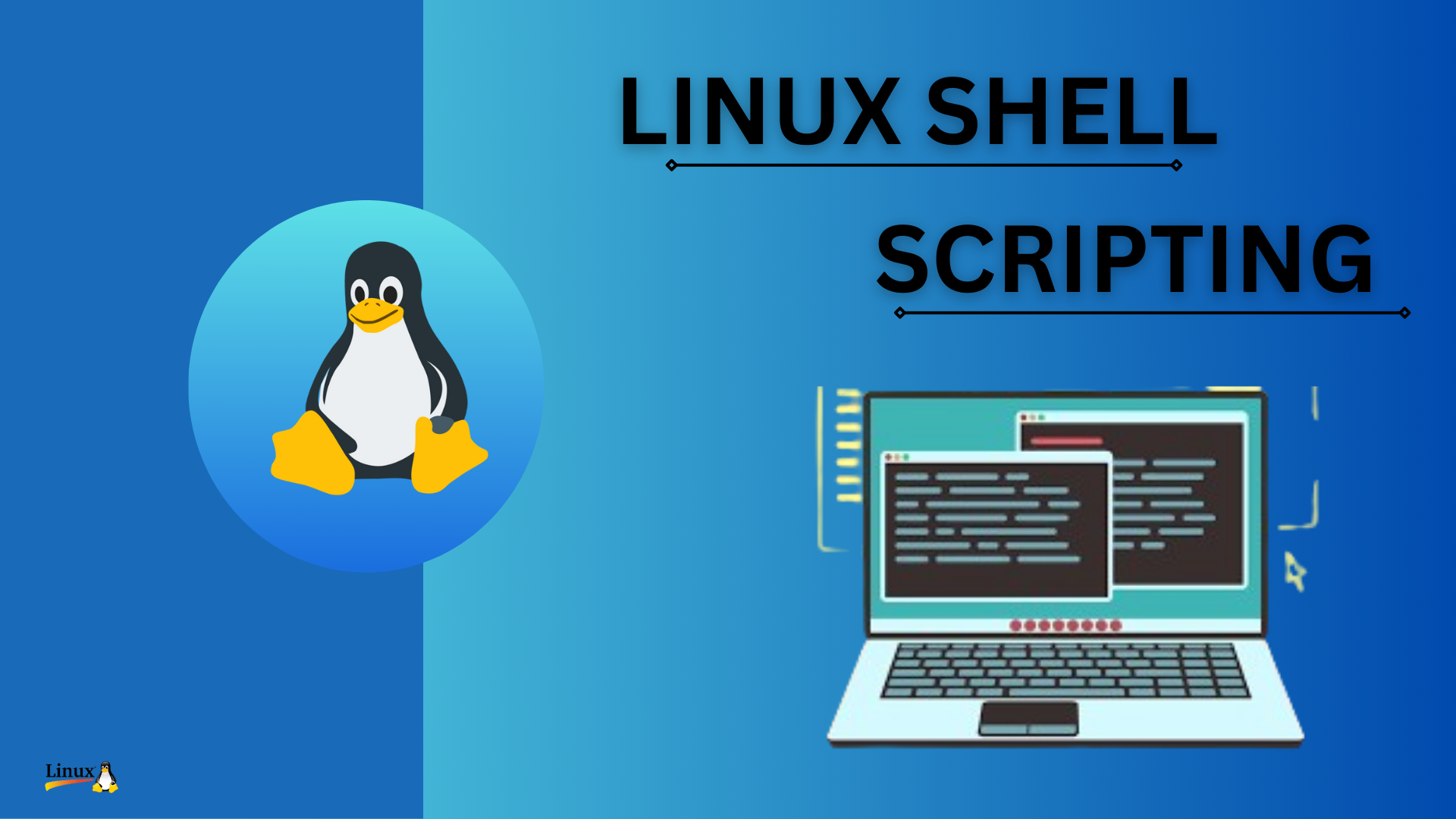
Contents
1. Introduction to Shell Scripting 👋
What is Shell Scripting?🤔
Shell scripting involves writing a series of commands in a script file that the shell (command-line interface) can run automatically. The shell is like a command interpreter that executes each line in the script. This process helps automate tasks that would otherwise be done manually, such as running programs, managing files, or setting up environments.
Why is Shell Scripting Important for DevOps? 🔄
In DevOps, automation is key to efficiency. Shell scripts allow you to automate repetitive tasks, reducing manual effort and minimizing errors. For instance, you can automate system updates, monitor server health, deploy applications, or schedule backups. By writing scripts, DevOps engineers can handle multiple tasks consistently and quickly.
Basic Components of Shell Scripting 💻
Commands: Instructions executed by the shell to perform actions like listing files or navigating directories.
Variables: Used to store data like text, numbers, or file paths for easy access and reuse within the script.
Operators: Used for operations like calculations, comparisons, and logical decisions within the script.
Why Are We Still Using Shell Scripting Despite the Availability of Modern Automation and Configuration Management Tools? 🤔
While there are many advanced tools available for automation, infrastructure management, and configuration (like Ansible, Terraform, and Puppet), shell scripting remains relevant for several key reasons:
1. Lightweight and Simple 🪶
Shell scripts are lightweight and require minimal setup. Unlike complex tools that might need installation, configuration, and specific environments, a shell script can run directly in any Unix/Linux-based system, making it quick and easy to use.
2. Flexibility and Control 🎛️
Shell scripting offers complete control over the system. You can execute custom commands, manipulate files, and perform system tasks exactly the way you want without depending on predefined modules or functions that come with automation tools.
3. Perfect for Small and Quick Tasks ⚡
For simple or one-time tasks, using a large tool might be overkill. Shell scripts are ideal for these small jobs, like cleaning up log files, checking disk space, or scheduling cronjobs, without the need for heavy automation tools.
4. Integration with Existing Tools 🔗
Even with modern tools like Jenkins or Ansible, shell scripting is often used in conjunction. For instance, you might use a shell script within a Jenkins pipeline to perform specific steps like env ironment setup or custom validation that isn’t easily handled by those tools alone.
5. First Step in Automation Learning 🧑💻
For beginners in DevOps, shell scripting is a foundational skill. It’s often the starting point before moving to more advanced tools. Understanding shell scripting helps in grasping key concepts like automation, task scheduling, and basic system operations.
6. Ubiquitous Across Systems 🌐
Shell scripts are universally supported across all Unix-based systems, including macOS and Linux servers. They provide a common way to automate tasks across different environments without worrying about compatibility issues.
7. Immediate Feedback for Troubleshooting 🔍
Shell scripts provide quick feedback when things go wrong, making debugging straightforward. For critical, low-level tasks like server recovery or network diagnostics, this is crucial and can be faster than debugging more complex automation tools.
8. Not for Full-Fledged Automation, But Essential for Ad-Hoc Tasks and Tool Integration 🛠️
These days, we rarely rely on shell scripts for large-scale automation. Instead, shell scripts are often used alongside modern automation tools or for quick ad-hoc tasks that don’t require a full solution. For example:
AWS User Data: Often contains shell scripts to configure instances during launch.
Packer AMI Creation: Uses shell scripts to customize AMIs during the build process.
Configuration Management Tools: Even with Ansible or Chef, shell scripts may be used for custom steps that aren’t natively supported.
How to Get Started With Shell Scripting? 🛠️
Getting started with shell scripting is simpler than you might think! Let’s break down the basic steps you need to follow:
1. Basic Setup: Linux Terminal and Text Editor 📄✍️
To start writing shell scripts, you’ll need:
Linux Terminal: The shell, often Bash, is where you’ll run your scripts. Most Linux distributions and macOS already have a terminal built in.
Text Editor: You can use a command-line editor like Vim or Nano. These editors are lightweight and easy to use. You can also use GUI-based editors like Visual Studio Code, but learning a terminal-based editor can be handy when working directly on remote servers.
Here’s how to open a terminal and start editing a file:
nano first_script.sh
This command opens a new file called first_script.sh
in Nano editor.
2. Writing Your First Script: A Simple "Hello, World!" 🌍👋
The classic first step in any programming or scripting language is to create a simple "Hello, World!" program. Here’s what that looks like in a shell script:
#!/bin/bash
echo "Hello, World! "
Explanation:
#!/bin/bash
: This line is called the shebang. It tells the system which interpreter to use to run the script (in this case, Bash).echo "Hello, World!"
: Theecho
command outputs the text to the terminal.
After saving the file, you can run it to see the output.
3. Understanding File Permissions: chmod +x
Explained 🔑
Before running your script, you need to make it executable. By default, scripts you write aren’t executable, which means the system won’t run them. This is where file permissions come in.
You can use the chmod
command to change file permissions:
chmod +x first_script.sh
Explanation:
chmod +x
: This command adds execute permissions to your script.Analogy: Think of it like a locked door 🏠. You need a key 🔑 (permissions) to open (execute) it. Without the right permissions, the script won’t run, no matter what’s inside.
Once permissions are set, you can run your script with:
./first_script.sh
You should now see your greeting: Hello, World!
Popular Shell Scripting Languages 🗣️
When it comes to shell scripting, different shells offer varying features and capabilities. Let’s dive into the most commonly used shell scripting languages.
1. Bash: The Most Common, Especially for Linux Users 🐧
Bash (Bourne Again Shell) is the most widely used shell on Linux systems. It’s known for its simplicity, flexibility, and extensive support across different environments.
Why is Bash so Popular?
Bash is the default shell on most Linux distributions and macOS, making it the go-to choice for shell scripting. It supports essential programming features like loops, conditionals, and functions, allowing you to create complex scripts that automate tasks.Tip:
Think of Bash as the Swiss Army knife 🔪 of shell scripting—versatile, reliable, and packed with useful tools.
Example Use Cases:
Automating server maintenance tasks.
Writing deployment scripts.
Creating backup scripts for databases.
2. Zsh: A Powerful Alternative with More Features ⚙️
Zsh (Z Shell) is another popular shell that offers all the features of Bash, plus more advanced capabilities. It’s highly customizable and comes with features like better auto-completion, command correction, and plugin support.
What Makes Zsh Stand Out?
Zsh is often favored by power users and developers who want more efficiency and flexibility. It offers better scripting options, robust error handling, and support for complex patterns and globbing.Why Choose Zsh?
If you need a more feature-rich environment and want a shell with built-in enhancements for productivity, Zsh is a great choice.
Example Use Cases:
Customized and interactive command-line environments.
Advanced automation scripts requiring better control and error handling.
Enhancing productivity with plugins and themes (e.g., Oh My Zsh).
3. KornShell (ksh) and Other Options 🚀
KornShell (ksh) is another scripting language that combines the best features of both the original Bourne shell (sh) and C shell (csh). It’s known for being faster and more efficient, with better scripting capabilities.
- What Makes KornShell Unique?
KornShell was designed for performance and offers advanced features like better arithmetic operations and built-in support for job control and co-processes. Although it’s not as commonly used today, it’s still found in legacy systems and specialized environments.
Other Shells:
Fish (Friendly Interactive Shell): Known for user-friendly features and beautiful syntax highlighting.
Dash (Debian Almquist Shell): A lightweight and fast shell, often used in systems where performance is critical.
Example Use Cases for KornShell:
Legacy systems that require compatibility with older scripts.
High-performance environments where speed is critical.
Shell Scripting FAQs ❓
What’s the Difference Between Bash and Shell? 🤷♂️
Shell refers to any command-line interface that interacts with the operating system, like Bash, Zsh, or KornShell.
Bash is a specific type of shell (Bourne Again Shell), commonly used on Linux. It’s a superset of the original Bourne shell (
sh
), adding more features and flexibility.
In short: All Bash scripts are shell scripts, but not all shell scripts are written in Bash.
Can I Use Shell Scripts on Windows? 🖥️
Yes, you can use shell scripts on Windows! Windows Subsystem for Linux (WSL) allows you to run a Linux environment directly on Windows, where you can execute Bash scripts. Additionally, tools like Git Bash, Cygwin provide Bash-like environments on Windows.
In short: With WSL or similar tools, you can easily run shell scripts on Windows.
What’s the Best Way to Debug a Script? 🧐
bash -x script.sh :You use this when running the entire script. The
-x
option is added directly when invoking the script to enable debugging. It prints each command and its expanded arguments before executing it.set -x: You place this line inside the script to start debugging from that point onward. It’s useful if you only want to debug specific sections of your script. You can turn it off later with set +x.
Use Echo Statements: Add echo commands to print variable values or messages at key points.
Check for Syntax Errors: Use bash -n script.sh to identify syntax issues without executing the script.
In short: Using -x
, echo statements, and syntax checks are effective ways to debug shell scripts.
#!/bin/bash
echo "Starting script"
set -x # Start debugging
echo "This is a debugged section"
set +x # Stop debugging
echo "Script completed"
Conclusion 📝
Shell scripting remains essential in DevOps, even with many automation tools available. While it's no longer used for full-scale automation, it's still critical for ad-hoc tasks, integrating with tools, and simplifying routine processes. Shell scripts also enhance your problem-solving skills, which are often tested in interviews. Whether you're setting up environments or automating small tasks, shell scripting is a valuable skill that remains relevant. Keep scripting simple, and you'll find it to be an effective tool in your DevOps journey! 🚀
Subscribe to my newsletter
Read articles from Vaishnavi Modakwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
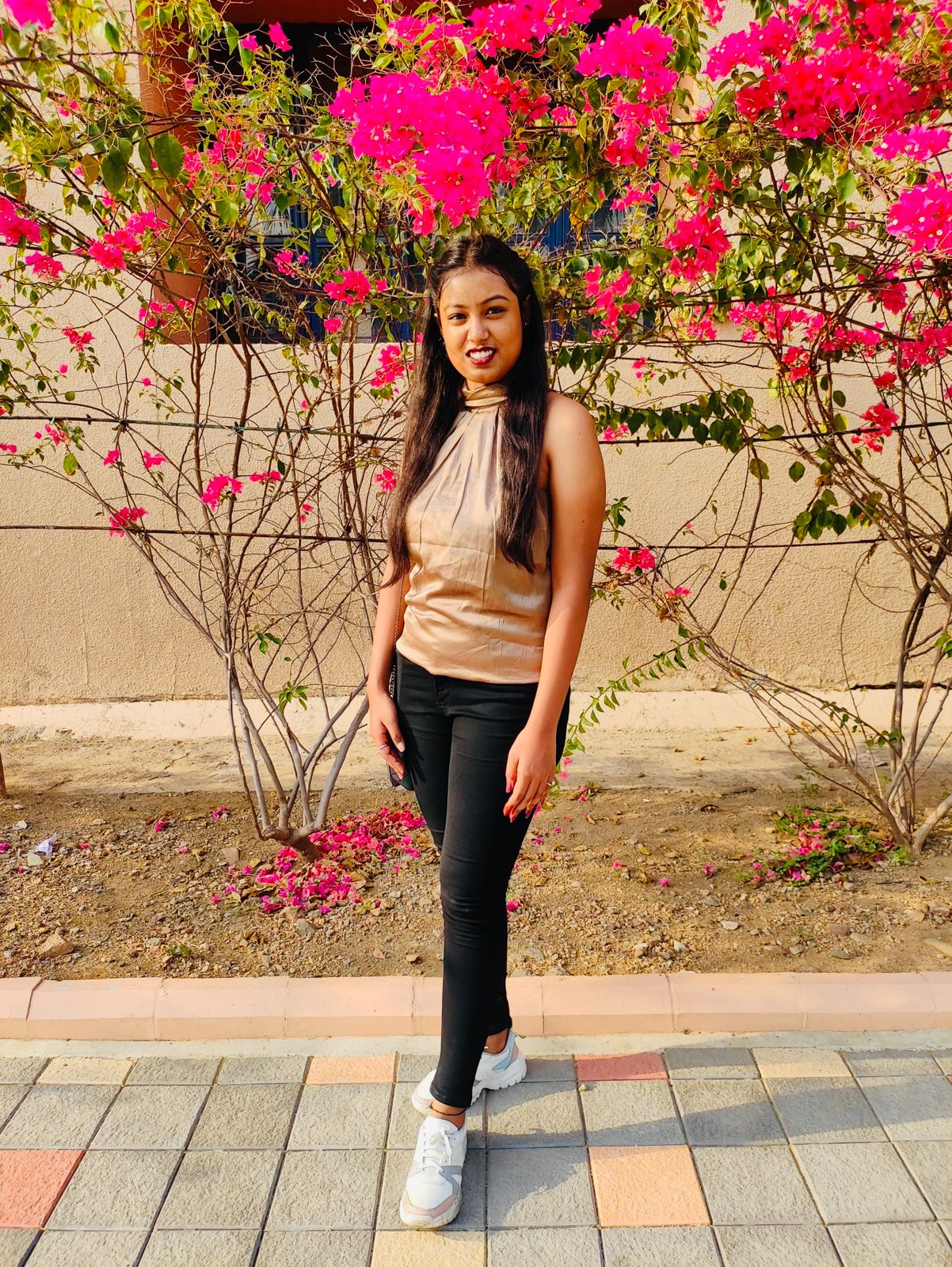
Vaishnavi Modakwar
Vaishnavi Modakwar
👋 Hi there! I'm Vaishnavi Modakwar, a dedicated DevOps and Cloud Engineer with 2 years of hands-on experience in the tech industry. My journey in DevOps has been fueled by a passion for optimizing and automating processes to deliver high-quality software efficiently. Skills: Cloud Technologies: AWS, Azure. Languages: Python, YAML, Bash Scripting. Containerization: Docker, ECS, Kubernetes. IAC: Terraform, Cloud Formation. Operating System: Linux and MS Windows. Tools: Jenkins, Selenium, Git, GitHub, Maven, Ansible. Monitoring: Prometheus, Grafana. I am passionate about demystifying complex DevOps concepts and providing practical tips on automation and infrastructure management. I believe in continuous learning and enjoy keeping up with the latest trends and technologies in the DevOps space. 📝 On my blog, you'll find tutorials, insights, and stories from my tech adventures. Whether you're looking to learn about CI/CD pipelines, cloud infrastructure, or containerization, my goal is to share knowledge and inspire others in the DevOps community. Let's Connect: I'm always eager to connect with like-minded professionals and enthusiasts. Feel free to reach out for discussions, collaborations, or feedback. Wave me at vaishnavimodakwar@gmail.com