A Simple Guide to Establishing Serial Communication Between A Computer and Arduino with Python
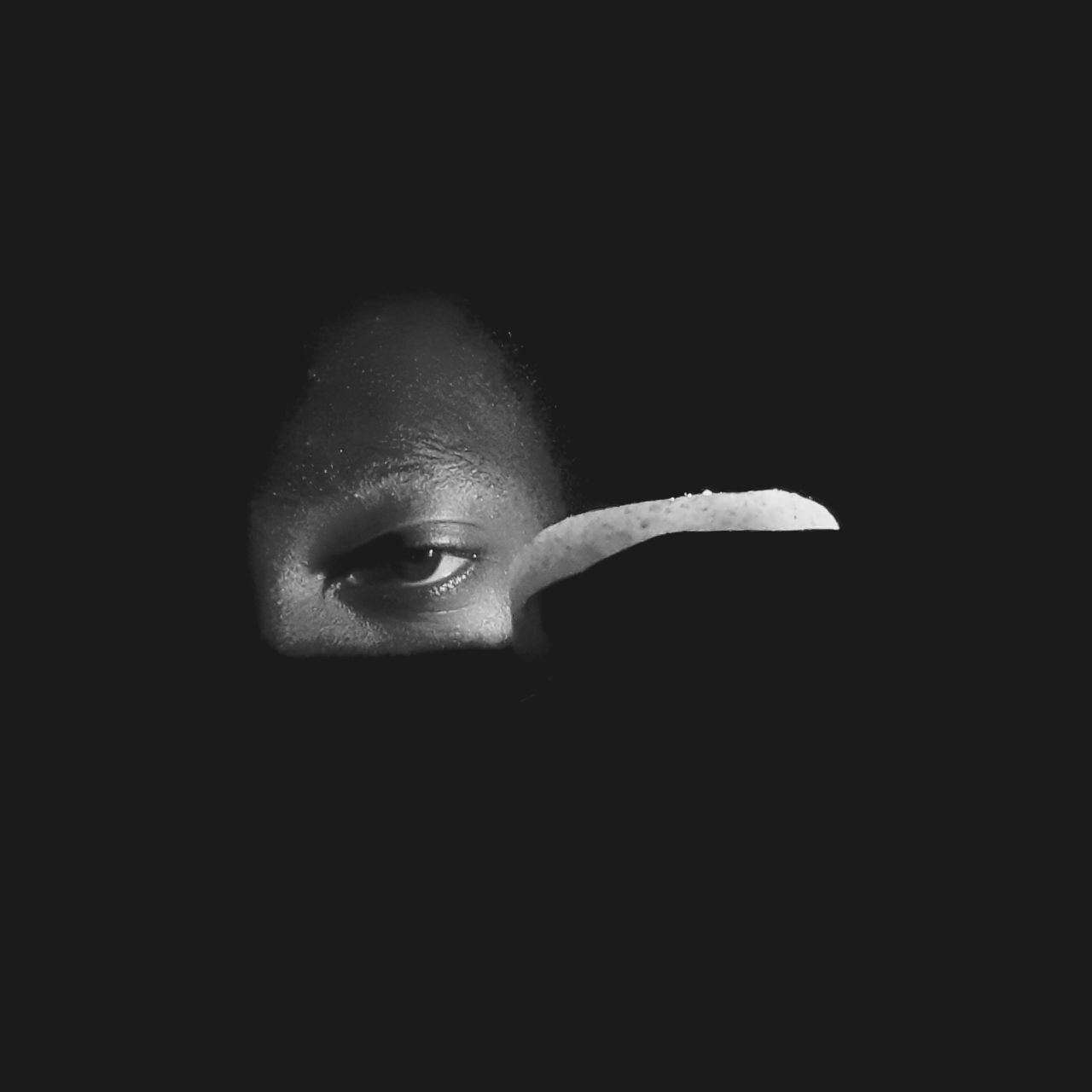
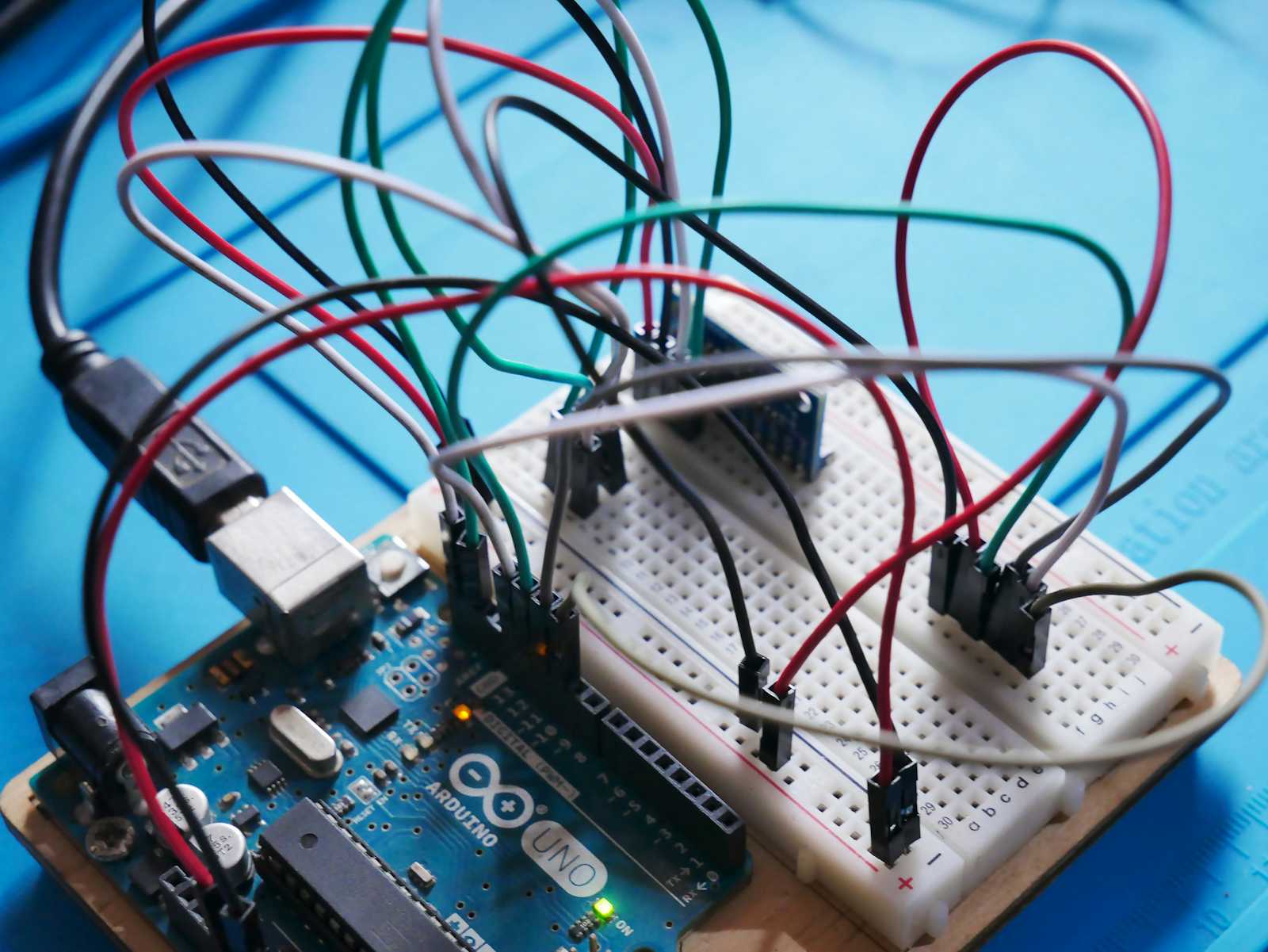
It may be of interest to you if you have long desired to establish communication between your Python script and your Arduino script via your USB. This is indeed achievable. We will commence from the basics, constructing a straightforward circuit in the process. Stay engaged as we go through this tutorial.
Materials
A computer with functioning USB ports
An Arduino board (UNO, Mega, Pro Mini, Nano, ESP32, all are suitable)
A USB cable to link your board and computer
A breadboard
1 x 220-ohm resistor (or any resistor, really, just to protect the LEDs from burning out)
Jumper cables
Installations
Python
Before you can run a Python script for communication, there are some libraries you should consider downloading. These include the pyserial and the tkinter library—only necessary if you want a simple GUI; otherwise, communication is done in a terminal.
Ensure you have python
installed on your computer. You can do this by typing:
If you don't see this:
Download from the official Python website.
You can check out pypi.com for Python packages to install. Next, proceed with installing the pyserial library.
pip3 install pyserial
Please note that you need to be connected to the internet to install
pyserial.
After installation, you can refer to the pyserial documentation (optional).
Arduino
Also, make sure you have downloaded and set up an IDE to program the Arduino microcontroller. It's best to use the Arduino IDE or set up the PlatformIO extension in your favorite code editor.
Note:Note: IDE stands for Integrated Development Environment.
The Python Script for the Terminal
This script helps us communicate with our Arduino board through the serial port. Feel free to adjust the PORT, BAUDRATE, and TIMEOUT according to your needs. This is the command-line version of the project, and the graphical user interface project will be covered in a separate blog.
import serial as ser
import time
# define relevant variables
PORT = 'dev/tty/ACM0' # the port may be COM3
BAUDRATE = 9600 # baudrate define in arduino
TIMEOUT = 30 # timeout
# valid characters
CHARACTERS = ['A', 'B', 'X']
# define the port object
port = ser.Serial(PORT, BAUDRATE, TIMEOUT)
print('Serial port is ready!')
# a sleep function : 1 second
time.sleep(1)
# a function to send an encoded command to the arduino
def send(character):
if character in CHARACTERS:
port.write(character.encode())
else:
print('Invalid')
############
try:
while port:
response = input('Enter a character: ')
send(response)
if character == 'X':
send(response)
break
except KeyboardInterrupt | Exception:
print('Keyboard Interrupt or Exception')
port.close()
print('Port closed')
# run the script
if __name__ == '__main__':
pass
The script features a simple if-else statement that continuously prompts the user for input from the command-line to a designated port. Upon receiving a character, it processes the input and verifies if it matches 'X'. If the character is 'X', a response is sent, and the loop is exited.
In the event of a KeyboardInterrupt or any other exception, the program gracefully handles the situation by displaying a message about the interruption or exception, proceeds to close the port.
Otherwise, it encodes and transmits the inputted characters through the serial port. This script is crafted to operate efficiently, effectively handling input characters while ensuring a reliable response mechanism.
// a simple serial communication program to control an LED
// Written on the 6th April 2024 by Prince Larbi
// define the pin for the LED
#define LED_PIN 13
// define the character read from the serial port
char data;
void setup{
// initialize the serial port
Serial.begin(9600);
// set the LED pin as an output
pinMode(LED_PIN, OUTPUT);
}
void loop() {
if (Serial.available()) {
char data = Serial.read();
if (data == 'A') {
digitalWrite(LED_PIN, HIGH);
}
else if (data == 'B') {
digitalWrite(LED_PIN, LOW);
}
}
}
The Arduino code runs continuously in a loop, listening for incoming characters from the serial port and responding according to the predefined instructions.
This info might be relevant to you!
Name | Quantity | Component | |
2 | U1 | 1 | Arduino Uno R3 |
3 | D1 | 1 | Red LED |
4 | R1 | 1 | 1 kΩ Resistor |
You can access the code and schematic in this GitHub repository:
https://github.com/PhidLarkson/simple-serial-communication
I will show you how to use the pyserial library with tkinter for a GUI in the next blog post.
One love... happy coding!
Need help? Reach out to me here
Subscribe to my newsletter
Read articles from Prince Larbi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
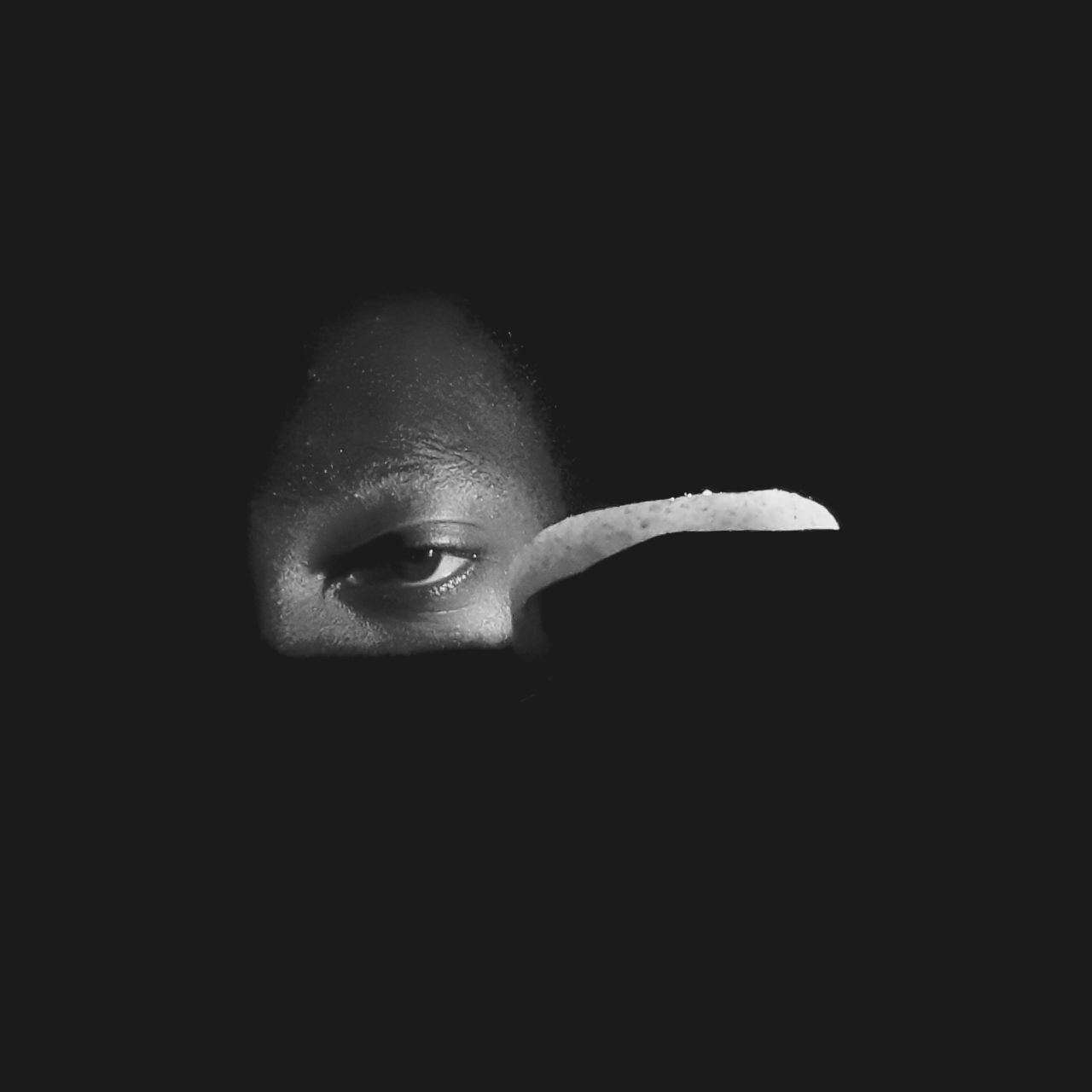
Prince Larbi
Prince Larbi
Enjoy the journey. yoghurt over coffee!! ...any day