The Art and Science of Debugging and Troubleshooting Code as a Backend Developer
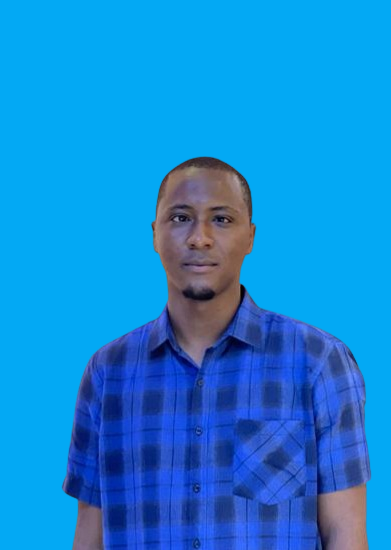
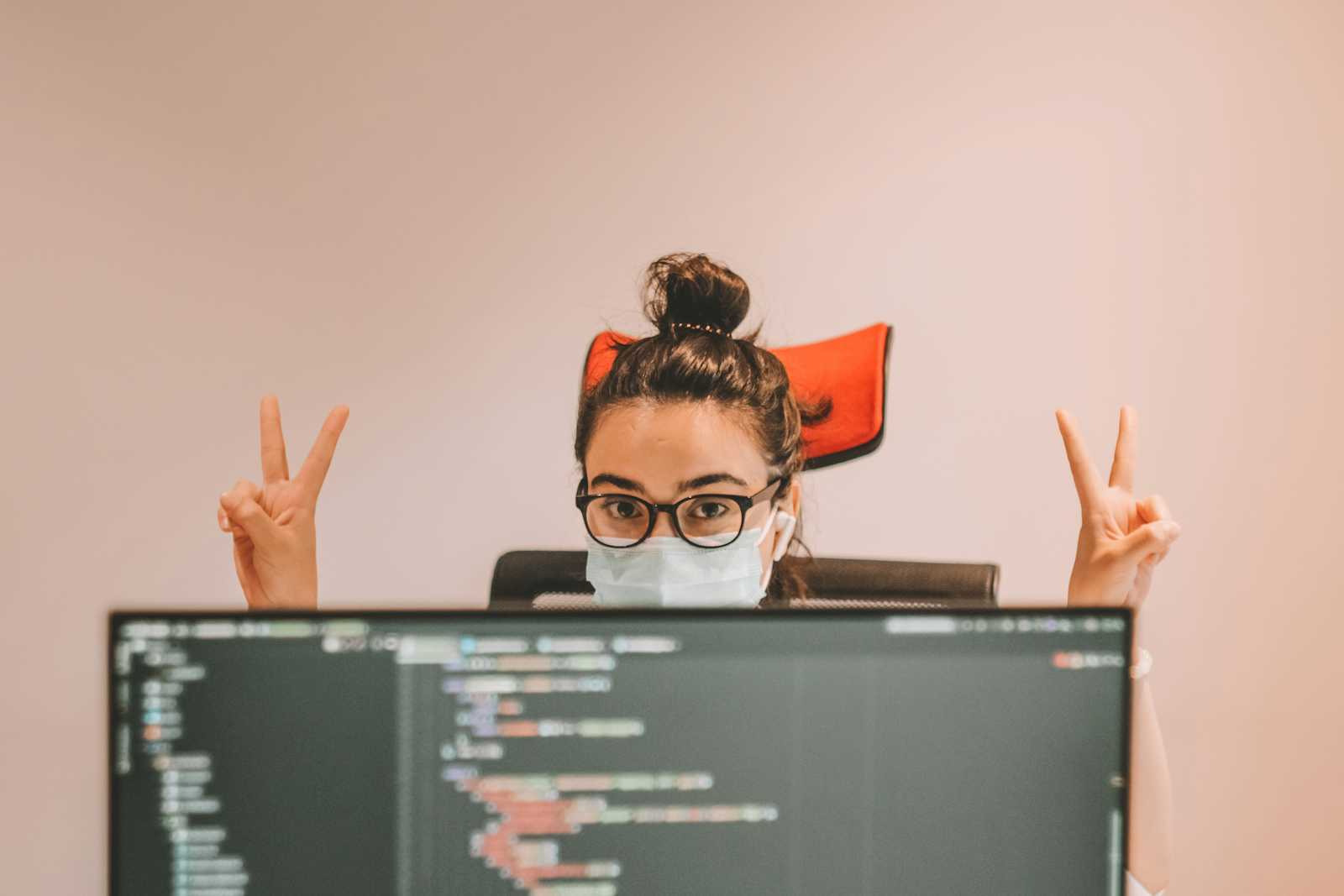
Introduction
Imagine you're a detective called to solve a mystery, but instead of searching for clues in the physical world, you’re diving deep into lines of code. Debugging, to many, is exactly this—an intricate process of uncovering hidden issues that disrupt the flow of a program. As backend developers, we often find ourselves in the midst of this detective work, piecing together the logic, identifying flaws, and ensuring the software runs smoothly. Debugging and troubleshooting are not just tasks; they are essential skills that can make or break the efficiency and reliability of backend systems. This article explores the techniques, tools, and mindset required to master the art and science of debugging and troubleshooting as a backend developer.
Understanding Debugging and Troubleshooting
Debugging and troubleshooting are fundamental to software development, but they often get conflated. While closely related, they serve different purposes:
Debugging is the process of identifying and fixing bugs in the code. It’s a reactive task that occurs after an issue is discovered, focusing on pinpointing the exact cause of the problem and correcting it.
Troubleshooting is a broader process, involving the systematic investigation of a problem to determine the root cause. It includes debugging but also extends to solving configuration issues, understanding performance bottlenecks, and fixing integration problems.
Backend developers frequently encounter a wide range of challenges, from simple syntax errors to complex architectural flaws. Understanding these challenges and how to approach them methodically is the first step in becoming an effective problem solver.
The Debugging Mindset
Effective debugging starts with the right mindset. Unlike writing code, where creativity and innovation are often at the forefront, debugging requires a disciplined, analytical approach. Here’s what defines a good debugging mindset:
Patience and Persistence: Bugs can be frustrating, especially when the solution isn’t immediately apparent. A calm, methodical approach is essential. Rushing can lead to oversight, so take your time and approach the problem step by step.
Curiosity: A good debugger is naturally curious. When something goes wrong, it’s an opportunity to learn. This mindset turns debugging from a chore into a puzzle that you’re eager to solve.
Critical Thinking: Debugging is about asking the right questions. What changed? What assumptions am I making? Where could things have gone wrong? Critical thinking allows you to approach the problem logically, eliminating assumptions and focusing on facts.
Tools of the Trade
As a backend developer, your toolkit is your best ally in the debugging process. Here are some essential tools and techniques:
Integrated Development Environments (IDEs): Most modern IDEs come with powerful debugging tools. Visual Studio Code, WebStorm, and PyCharm, for example, offer features like breakpoints, variable inspection, and step-by-step execution that can make finding bugs easier.
Logging: Logging is your eyes and ears in a running application. Properly placed log statements can reveal the flow of execution and the state of your application at crucial points. It’s important to log enough information to be useful without overwhelming yourself with too much data.
Version Control: Systems like Git are invaluable for tracking changes and identifying when a bug was introduced. The ability to revert to a previous version or view a detailed history of changes can make troubleshooting significantly easier.
Debugging Libraries: Language-specific debugging tools, such as Node.js's built-in debugger or Python’s
pdb
, allow you to pause execution, inspect variables, and step through your code. These tools are essential for digging deep into the behavior of your application.Error Monitoring Tools: Tools like Sentry, New Relic, and Datadog help monitor your application in production, catching errors that occur in real time. These services provide insights into where and why your code is failing, often before you even know there’s an issue.
Effective Debugging Techniques
Having the right tools is essential, but knowing how to use them effectively is what makes a great debugger. Here are some techniques to enhance your debugging process:
Reproducing the Bug: The first step in debugging is to reliably reproduce the issue. If you can’t recreate the bug, it’s much harder to fix. Make sure you understand the environment and conditions that cause the problem, whether it’s a specific input, user action, or system state.
Divide and Conquer: Break the problem down. If your application is large, isolate the section of code where the issue is likely to reside. By narrowing down the problem space, you can focus on a smaller area, making it easier to find the bug.
Rubber Duck Debugging: This quirky but effective technique involves explaining your code, line by line, to an inanimate object (often a rubber duck). The act of verbalizing your thoughts forces you to slow down and can help you see flaws you might otherwise miss.
Binary Search: If you’re unsure where the bug lies, you can perform a binary search by systematically commenting out or bypassing sections of code to determine where the problem does not exist. By halving the potential problem area with each step, you can zero in on the bug more quickly.
Unit Testing: Writing unit tests not only helps prevent bugs but also aids in debugging by allowing you to test specific components in isolation. If a bug is discovered, you can often write a test case that reproduces the issue, making it easier to fix.
Peer Reviews: Another set of eyes can often spot issues you’ve overlooked. Pair programming or code reviews are invaluable for catching bugs early and learning new debugging techniques from your peers.
Common Pitfalls and How to Avoid Them
Even experienced developers can fall into common traps when debugging. Here are a few pitfalls to watch out for:
Overcomplicating the Problem: It’s easy to assume that a bug must have a complex cause, but often the simplest explanation is correct. Before diving into deep technical analysis, check for common issues like typos, configuration errors, or missing dependencies.
Ignoring the Basics: Don’t overlook basic checks like ensuring your development environment is properly configured, your code is up to date, and your dependencies are correctly installed. These simple checks can save hours of frustration.
Not Using Version Control Properly: Failing to commit changes regularly or not documenting commits can make it difficult to track down when and why a bug was introduced. Use version control systematically to keep a clear history of your codebase.
Real-World Examples
Let’s consider a few real-world scenarios where debugging and troubleshooting skills come into play:
Case Study 1: A backend service starts to experience performance issues. After thorough logging and analysis, the issue is traced to a poorly optimized database query that was added during a recent update. The developer uses version control to identify when the change was made and corrects the query, restoring performance.
Case Study 2: An API endpoint returns incorrect data. The developer uses binary search debugging to isolate the problem to a specific function. Further inspection reveals a logic error in how input parameters are handled. The error is corrected, and additional unit tests are added to prevent future regressions.
Case Study 3: An intermittent bug occurs in production, but it cannot be reproduced in the development environment. By using an error monitoring tool like Sentry, the developer gathers detailed reports from users experiencing the bug, identifying a rare edge case that was previously missed. The code is updated to handle this case, resolving the issue.
Each of these examples highlights the importance of methodical debugging and the use of appropriate tools to solve complex issues.
Conclusion
Debugging and troubleshooting are indispensable skills for backend developers. By approaching these tasks with the right mindset, utilizing the right tools, and applying effective techniques, you can turn even the most daunting bugs into manageable challenges. Remember, debugging is not just about fixing code—it’s about learning, improving, and becoming a better developer with each issue you resolve.
Call to Action
Debugging is a journey every developer must undertake. What are your favorite debugging techniques? Have you faced a particularly challenging bug that taught you something new? Share your experiences and insights with the community in the comments below or on social media. Let’s learn from each other and continue to grow as developers.
This article provides a comprehensive exploration of debugging and troubleshooting, with actionable advice and real-world examples to illustrate key points. It’s designed to resonate with backend developers at any stage in their careers, offering both practical tips and a deeper understanding of the mindset needed to tackle code issues effectively.
Subscribe to my newsletter
Read articles from Aliyu Gambo Aliyu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
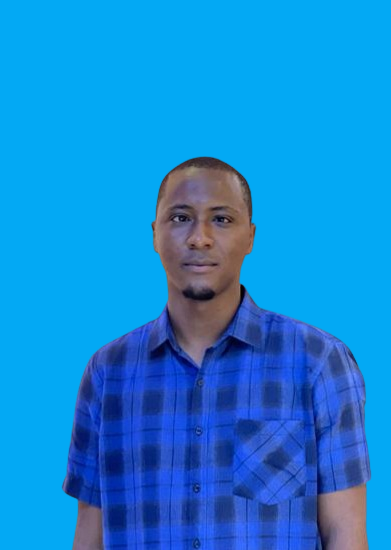
Aliyu Gambo Aliyu
Aliyu Gambo Aliyu
I am a Software Developer from Nigeria. Here's a quick summary about me: 😊 Pronouns: He/him 💡 Fun fact: I'm currently studying at AltSchool Africa School of Software Engineering Class of 2022 and also at HyperVerge Academy Class of 2023. 🌱 I’m currently learning JavaScript and Nodejs. 😊 I’m looking for help with open source projects, hackathons, internships, and entry-level opportunities. 💼 Job interests: Software Engineer, Front Engineer, Backend Engineer(Intern or Junior level). 📫 You can view my resume @mafuztechsolution.live and contact me by email.