Optimizing Build Performance: A Personal Guide to Streamlining Your CI/CD Pipeline
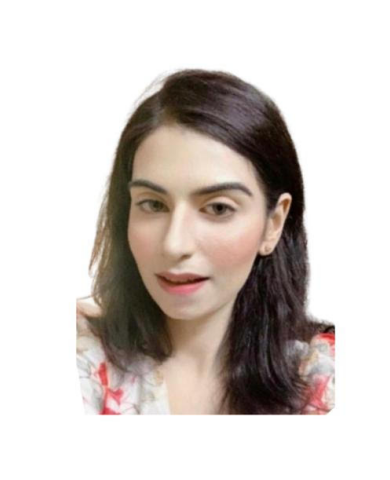
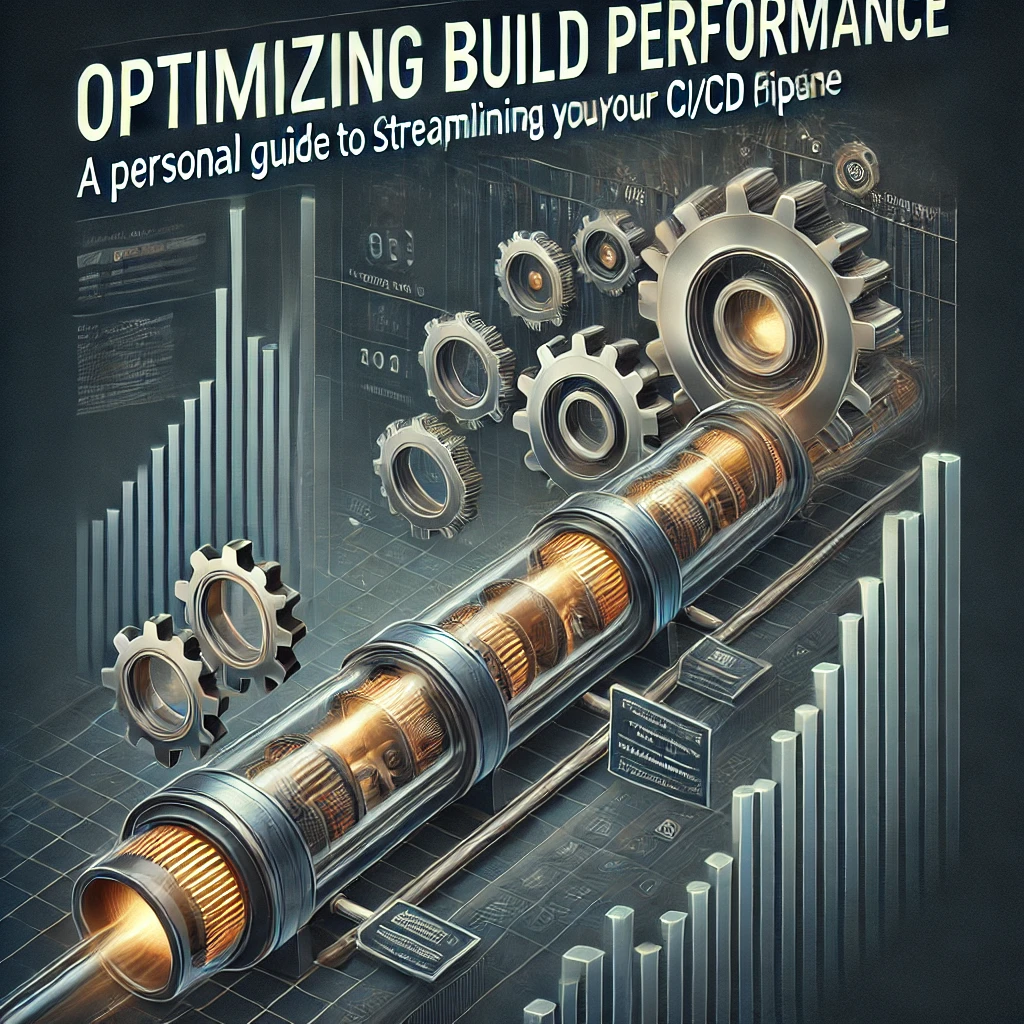
I’ve been through the grind of slow builds, watching as valuable time slips away while my codebase crawls through a sluggish CI/CD pipeline. But the good news? We can fix this together.
Let’s walk through some practical strategies that I’ve personally found effective in speeding up build times, and I’ll share some code examples to make things crystal clear.
1. Incremental Builds: Only Rebuild What’s Changed
When I first started dealing with large codebases, I noticed that every single change triggered a full rebuild. It’s like repainting the entire house when all you wanted was to touch up a single room. Incremental builds fix this.
What I Do: I configure my build system to only recompile the parts of the code that have actually changed. This way, I’m not wasting time on code that hasn’t been touched.
Example in C/C++ with Makefile:
myapp: main.o module1.o module2.o
gcc -o myapp main.o module1.o module2.o
main.o: main.c
gcc -c main.c
module1.o: module1.c
gcc -c module1.c
module2.o: module2.c
gcc -c module2.c
Why It Works: By focusing on just the modified files, we slash build times without compromising on what matters.
2. Modularization: Break It Down
When my projects started growing in complexity, I found myself drowning in long build times. The solution? Modularization. Instead of building everything at once, I broke down my codebase into smaller, independent modules.
What I Do: I organize my projects into separate modules or services, each with its own build process. This way, I only build what’s necessary.
Example in Maven (Java):
mvn clean install -pl module1 -am
Why It Works: This approach means I’m not bogged down by unrelated code when making changes to specific parts of the project.
3. Parallel Builds: Use All Your Resources
One day, it hit me—my build process was only using a fraction of my computer’s power. Parallel builds were the answer. Why compile one file at a time when I could do it simultaneously?
What I Do: I configure my build system to run multiple build tasks concurrently, using all available CPU cores.
Example with GNU Make:
make -j4
Why It Works: By running tasks in parallel, we drastically reduce the time spent waiting for the build to complete. It’s like having a team instead of working solo.
4. Build Caching: Don’t Repeat Yourself
I’ve always hated repeating myself—whether it’s in conversation or in code. Build caching follows the same principle. Why rebuild or reinstall something that hasn’t changed?
What I Do: I cache dependencies and build artifacts to avoid redundant work in future builds.
Example with Docker:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
Why It Works: Docker uses cached layers, so if nothing has changed in package.json
, I’m not wasting time reinstalling dependencies. It’s all about efficiency.
5. Use of Precompiled Headers (C/C++):
One thing I’ve found particularly useful in C/C++ projects is using precompiled headers. When large headers are included across multiple files, compiling them over and over can slow things down.
What I Do: I precompile commonly used headers so they don’t have to be compiled repeatedly.
Example:
// Precompiled header file (pch.h)
#ifndef PCH_H
#define PCH_H
// Add headers that you want to pre-compile here
#include <iostream>
#include <vector>
#include <string>
#endif //PCH_H
Why It Works: This reduces the time spent on redundant compilation of common headers, speeding up the overall build process.
6. Optimized Dependency Management:
Managing dependencies efficiently is crucial. I used to let my build scripts download dependencies from scratch each time—big mistake. The solution was straightforward.
What I Do: I cache dependencies and only update them when necessary.
Example in Node.js:
"scripts": {
"install": "npm ci"
}
Why It Works: By using npm ci
instead of npm install
, I ensure that dependencies are installed exactly as specified, leveraging caching for speed while avoiding unnecessary updates.
7. Minimize Resource Usage:
One day, I realized my builds were choking because of resource limits—especially memory. To fix this, I started tweaking resource allocations.
What I Do: I limit the resources each build step uses, ensuring that my system doesn’t get overwhelmed.
Example in Docker:
version: '3.7'
services:
app:
build:
context: .
dockerfile: Dockerfile
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
Why It Works: By capping resource usage, I prevent my builds from consuming all available memory or CPU, which helps in environments with limited resources.
Wrapping It Up:
Optimizing build performance is a journey, not a destination. By applying incremental builds, modularization, parallel builds, and build caching, we can make our CI/CD pipelines not just faster, but smarter. I’ve been there, and I know these strategies work because they’ve saved me countless hours of frustration.
So, let’s take back control of our build times and keep our development process as efficient as possible. After all, our time is too valuable to spend waiting on slow builds.
Let me know how these strategies work out for you, and if you have any other tips, I’m all ears. We’re all in this together, and sharing what works is how we all get better.
Subscribe to my newsletter
Read articles from Tanishka Marrott directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
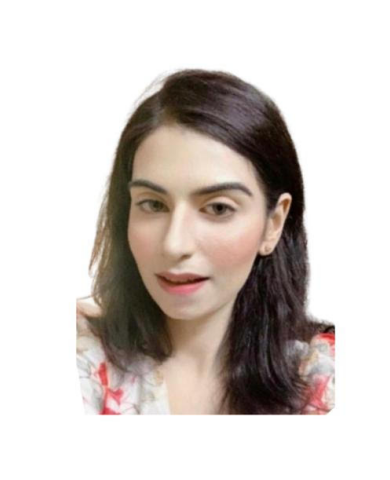
Tanishka Marrott
Tanishka Marrott
I'm a results-oriented cloud architect passionate about designing resilient cloud solutions. I specialize in building scalable architectures that meet business needs and are agile. With a strong focus on scalability, performance, and security, I ensure solutions are adaptable. My DevSecOps foundation allows me to embed security into CI/CD pipelines, optimizing deployments for security and efficiency. At Quantiphi, I led security initiatives, boosting compliance from 65% to 90%. Expertise in data engineering, system design, serverless solutions, and real-time data analytics drives my enthusiasm for transforming ideas into impactful solutions. I'm dedicated to refining cloud infrastructures and continuously improving designs. If our goals align, feel free to message me. I'd be happy to connect!