How Does a Browser Render a Web Page?
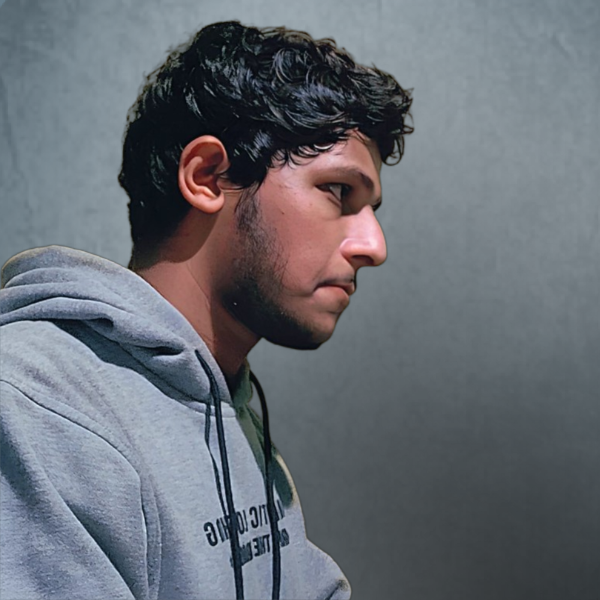
Introduction
Browsers are sophisticated software applications primarily written in languages like C++ and are designed to compile and interpret HTML, CSS, and JavaScript files. In the frontend world, developers are mainly concerned with how the browser renders the user interface (UI), while the other data files are managed by the browser in the background.
Let's dive into the step-by-step process of how a browser takes raw HTML and CSS and turns it into a fully rendered web page.
1. Loading and Parsing the HTML
When you enter a URL or open an HTML file, the browser starts by loading the file from the server. This file is in raw data format, typically binary (0s and 1s), which the browser needs to decode.
Character Conversion: The browser first converts these bits into a sequence of characters, which it can then interpret as text.
Tokenization: The browser takes this sequence of characters and breaks it down into tokens. Tokens are the basic building blocks of the page, representing different elements like tags, attributes, and content.
2. Building the DOM (Document Object Model)
The tokens are then organized into a tree-like structure called the DOM. The DOM is a hierarchical representation of the HTML document where each node represents an HTML element. This structure allows the browser to understand the layout and structure of the page.
HTML to NodeList: As the HTML is parsed, it is transformed into a NodeList format that allows the browser to interact with and display the content.
Scripts Execution: During this phase, if any
<script>
tags are encountered, the browser pauses the parsing of the HTML to execute the JavaScript. This ensures that any dynamic content generated by JavaScript is incorporated into the DOM.
3. Parsing the CSS and Creating the CSSOM
Parallel to the DOM creation, the browser also parses the CSS.
Character Conversion: Like HTML, the CSS is first converted from raw data into characters.
Tokenization: The CSS characters are tokenized into objects that represent different styling rules.
Building the CSSOM (CSS Object Model): These tokens are then organized into the CSSOM, which is a structure similar to the DOM but specifically for CSS. It represents all the styles associated with the elements in the DOM.
4. Constructing the Render Tree
The DOM and CSSOM are then combined to create the Render Tree. This tree represents the visual elements that need to be displayed on the page, including their styles and layout.
Layout Calculation: The browser calculates the size, position, and dimensions of each element based on the styles defined in the CSSOM and the structure of the DOM.
Render Tree: This tree contains only the elements that need to be displayed (e.g., elements with
display: none
are excluded).
5. Painting
Once the Render Tree is constructed, the browser begins the painting process.
- Painting: Painting is the process of filling in the pixels on the screen. The browser takes the information from the Render Tree and converts it into visual output. This step is what you actually see on your screen.
6. Compositing
For complex pages, the browser may break the page into layers and composite them together. This is especially common in pages with animations, complex layouts, or heavy use of CSS effects.
- Compositing: Each layer is painted separately and then combined to form the final image displayed on the screen. This helps in optimizing the rendering performance, especially when parts of the page need to be repainted (e.g., during scrolling or animations).
JavaScript’s Role in Rendering
JavaScript can significantly impact the rendering process. When the browser encounters a <script>
tag, it may pause the construction of the DOM and CSSOM until the script is executed. This is why JavaScript is often given priority over other resources like the DOM and CSSOM, as it can manipulate the content and structure of the page.
- Async and Defer: Modern web development practices often involve using the
async
anddefer
attributes on<script>
tags to prevent JavaScript from blocking the rendering process. This allows the browser to continue building the DOM and CSSOM while the JavaScript is being loaded.
Conclusion
Understanding how a browser renders a web page gives us deeper insight into the performance aspects of web development. By knowing the steps involved—from loading and parsing HTML to constructing the DOM and CSSOM, and finally rendering and painting—we can optimize your code for faster, more efficient web pages.
Remember, the DOM and CSSOM work independently but come together to form the Render Tree, which ultimately determines how your page looks and feels. With this knowledge, you can fine-tune your pages to ensure a smooth and responsive user experience.
Subscribe to my newsletter
Read articles from Himanshu Bhoir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
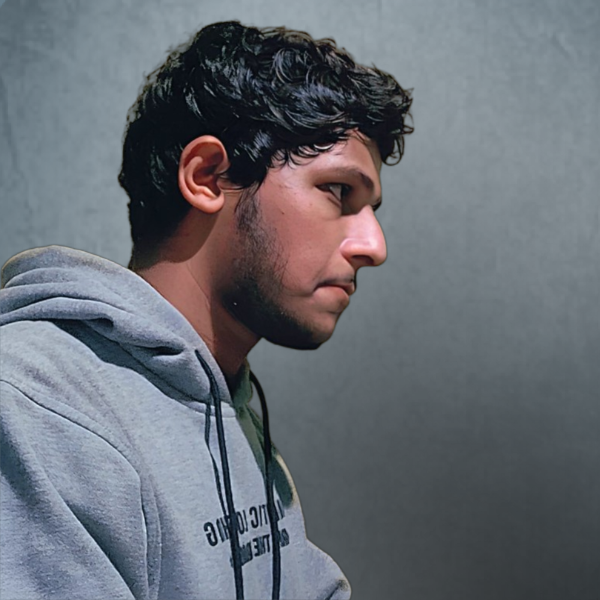
Himanshu Bhoir
Himanshu Bhoir
Obsessed with Web Development | Full-Stack Specialist in React, Node.js, and Spring Boot | Top 5% LeetCode Programmer