A Comprehensive Guide to PHP Laravel: Mastering the Popular PHP Framework

Table of contents
- What is Laravel?
- Key Features of Laravel:
- Why Choose Laravel for Web Development?
- Setting Up a Laravel Development Environment
- Understanding the MVC Architecture in Laravel
- Routing in Laravel
- Controllers in Laravel
- Working with Views in Laravel
- Models and Eloquent ORM in Laravel
- Database Migrations and Seeding in Laravel
- Working with Views in Laravel
- Models and Eloquent ORM in Laravel
- Database Migrations and Seeding in Laravel
- Form Handling and Validation in Laravel
- Error Handling and Debugging in Laravel
- Deploying a Laravel Application
- Conclusion
- FAQs
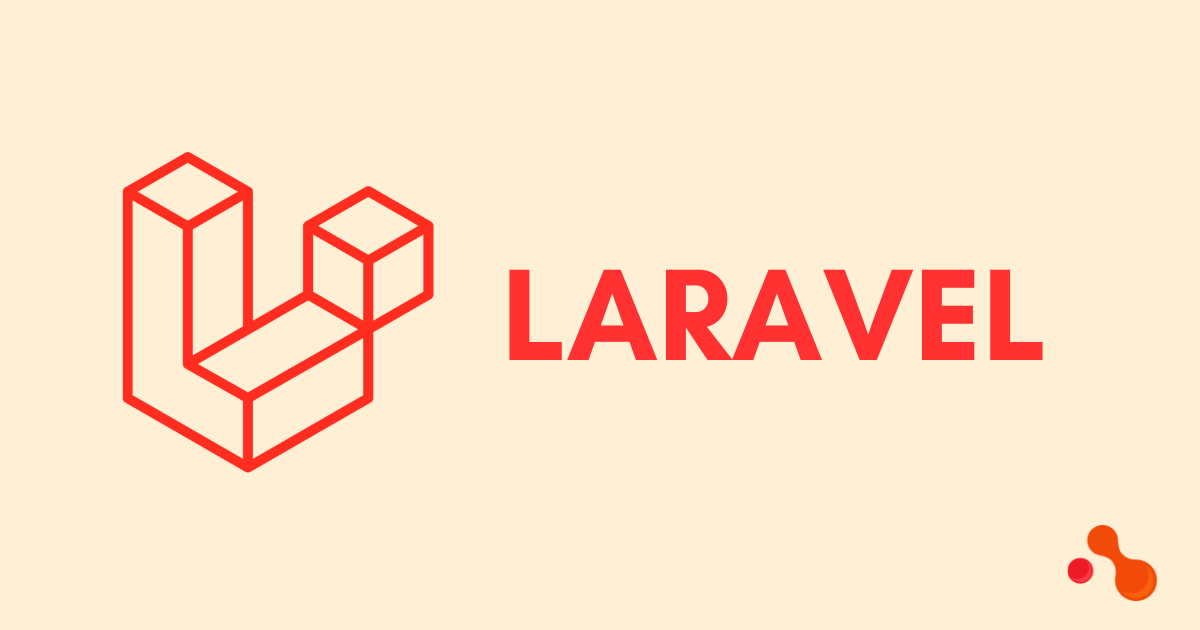
If you've been around web development circles, you've probably heard the name Laravel thrown around quite a bit. And there's a good reason for that.
Laravel is one of the most popular PHP frameworks, renowned for its elegant syntax, developer-friendly features, and the ability to handle complex web applications with ease.
Whether you're a seasoned developer or just starting, Laravel offers a solid foundation to build modern, robust web applications.
This guide aims to take you through everything you need to know to master Laravel, from setting up your environment to deploying your application.
What is Laravel?
Laravel is a PHP framework designed to make web development more accessible and enjoyable.
Launched by Taylor Otwell in 2011, Laravel has quickly gained traction due to its expressive, elegant syntax and its ability to streamline the development process.
Laravel takes the pain out of repetitive tasks like routing, authentication, and sessions, allowing developers to focus on the creative aspects of web development.
Key Features of Laravel:
Elegant Syntax: Laravel's syntax is intuitive and easy to learn, making coding a more pleasant experience.
MVC Architecture: Laravel follows the Model-View-Controller (MVC) pattern, promoting separating concerns and organized code.
Eloquent ORM: Laravel's built-in ORM makes database interactions simple and fluent.
Blade Templating: Laravel's Blade templating engine allows you to create dynamic views effortlessly.
Comprehensive Documentation: Laravel's thorough and accessible documentation is a boon for developers at all levels.
Compared to PHP frameworks like Symfony or CodeIgniter, Laravel stands out for its simplicity, robust features, and active community support.
It’s not just a tool; it’s a complete ecosystem that empowers developers to build powerful applications quickly and efficiently.
Why Choose Laravel for Web Development?
With so many frameworks available, you might wonder what makes Laravel a top choice.
Here are some compelling reasons:
Simplicity and Ease of Use: Laravel's clean and elegant syntax means you spend less time wrestling with the framework and more time building your application.
Elegant Syntax and Developer-Friendly Structure: The syntax of Laravel is designed to be both expressive and simple, making it easier to understand and maintain your codebase.
Robust Security Features: Laravel comes with built-in security features such as hashed passwords, CSRF protection, and SQL injection prevention, ensuring your application is secure by default.
Comprehensive Documentation and Community Support: Laravel boasts extensive documentation and a vibrant community, providing plenty of resources, tutorials, and forums to help you out when needed.
In essence, Laravel is designed to take the hassle out of development while providing all the tools you need to build top-notch web applications.
Setting Up a Laravel Development Environment
Before diving into Laravel, you need to set up your development environment. Here’s how to get started:
Prerequisites:
PHP: Ensure that you have PHP installed on your machine (preferably PHP 8.x).
Composer: Laravel uses Composer, a dependency manager for PHP, to install and manage its packages.
Web Server: You can use Apache or Nginx, though Laravel also comes with its own built-in development server.
Installing Laravel via Composer:
To install Laravel, open your terminal and run the following command:
composer global require laravel/installer
Once installed, you can create a new Laravel project by running:
laravel new project-name
Configuring Your Development Environment:
Laravel’s .env
file allows you to configure your application’s environment settings, such as database connection details, application URL, and more.
Setting Up Your First Laravel Project: Navigate to your project directory and start the built-in development server:
php artisan serve
You should now see your Laravel application running at http://localhost:8000
.
Understanding the MVC Architecture in Laravel
Laravel follows the MVC (Model-View-Controller) architectural pattern, which separates your application into three main components:
Model: Manages the data and business logic.
View: Handles the display and presentation.
Controller: Acts as a bridge between the Model and the View.
This separation of concerns makes your code cleaner, more organized, and easier to maintain.
Laravel’s implementation of MVC ensures that your application logic is neatly divided, making it easier to scale and modify.
Routing in Laravel
Routing in Laravel is both simple and powerful.
It’s how Laravel responds to various URL requests.
Defining Routes:
Routes are defined in the routes/web.php
file.
Here’s a simple example:
Route::get('/home', function () {
return view('home');
});
Route Parameters and Constraints:
Laravel allows you to pass parameters through routes:
Route::get('user/{id}', function ($id) {
return 'User '.$id;
});
You can also apply constraints to these parameters:
Route::get('user/{id}', function ($id) {
// Only numeric IDs are allowed
})->where('id', '[0-9]+');
Named Routes and Route Groups:
Named routes provide a convenient way of generating URLs or redirecting to specific routes.
Route groups allow you to apply middleware or prefix routes, making route management more efficient.
Controllers in Laravel
Controllers are responsible for handling requests and returning responses.
They are essential in the MVC architecture as they handle the logic behind your routes.
Creating and Using Controllers:
You can create a controller using the Artisan command:
php artisan make:controller UserController
Once created, you can define methods within your controller to handle different routes.
Resource Controllers and Route Model Binding:
Resource controllers provide a convenient way to create CRUD (Create, Read, Update, Delete) operations.
Laravel also supports route model binding, automatically injecting model instances into your routes.
Working with Views in Laravel
Laravel’s Blade templating engine is a powerful tool for creating dynamic views.
Blade allows you to use plain PHP code within your templates and provides useful directives for common tasks.
Creating and Using Views:
Views are stored in the resources/views
directory and can be returned using the view()
function:
return view('welcome');
Blade Directives and Components:
Blade includes several directives like @if
, @foreach
, and @include
, making it easy to create dynamic and reusable content.
Models and Eloquent ORM in Laravel
Eloquent is Laravel’s built-in Object-Relational Mapping (ORM) system. It makes interacting with your database easy and intuitive.
Defining and Using Models: Models in Laravel represent your database tables. You can define a model using the Artisan command:
php artisan make:model User
Relationships, Querying, and Eager Loading: Eloquent simplifies defining relationships between tables, such as hasOne
, hasMany
, and belongsTo
. Eager loading helps in reducing the number of queries when retrieving related models.
Database Migrations and Seeding in Laravel
Laravel’s migration system allows you to version control your database schema, while seeding helps in populating your database with initial data.
Creating and Running Migrations:
Migrations are created using the Artisan command:
php artisan make:migration create_users_table
Once created, you can define methods within your controller to handle different routes.
Resource Controllers and Route Model Binding:
Resource controllers provide a convenient way to create CRUD (Create, Read, Update, Delete) operations. Laravel also supports route model binding, automatically injecting model instances into your routes.
Working with Views in Laravel
Laravel’s Blade templating engine is a powerful tool for creating dynamic views. Blade allows you to use plain PHP code within your templates and provides useful directives for common tasks.
Creating and Using Views: Views are stored in the resources/views
directory and can be returned using the view()
function:
return view('welcome');
Blade Directives and Components: Blade includes several directives like @if
, @foreach
, and @include
, making it easy to create dynamic and reusable content.
Models and Eloquent ORM in Laravel
Eloquent is Laravel’s built-in Object-Relational Mapping (ORM) system. It makes interacting with your database easy and intuitive.
Defining and Using Models:
Models in Laravel represent your database tables. You can define a model using the Artisan command:
php artisan make:model User
Relationships, Querying, and Eager Loading:
Eloquent simplifies defining relationships between tables, such as hasOne
, hasMany
, and belongsTo
. Eager loading helps in reducing the number of queries when retrieving related models.
Database Migrations and Seeding in Laravel
Laravel’s migration system allows you to version control your database schema, while seeding helps in populating your database with initial data.
Creating and Running Migrations:
Migrations are created using the Artisan command;
php artisan make:migration create_users_table
Once created, you can run your migrations using;
php artisan migrate
Database Seeding and Factories:
Seeding allows you to insert dummy data into your database, while factories help in generating model instances for testing or seeding.
Form Handling and Validation in Laravel
Laravel makes form handling and validation straightforward and efficient.
Creating Forms in Laravel:
You can create forms in your Blade templates using HTML or Laravel’s Form Builder.
Validating Form Input:
Laravel’s validation system is powerful and flexible. You can validate input directly in your controllers:
$request->validate([
'name' => 'required|max:255',
'email' => 'required|email',
]);
Displaying Validation Errors:
Validation errors are automatically flashed to the session, and you can display them in your views using Blade’s @error
directive.Authentication and Authorization in Laravel
Laravel provides a comprehensive authentication system out of the box, making it easy to implement login, registration, and password reset functionalities.
Built-In Authentication Features:
Using Laravel’s Artisan commands, you can scaffold authentication views and controllers:
php artisan make:auth
Role-Based Authorization:
Laravel’s authorization system allows you to define roles and permissions, ensuring that only authorized users can access certain resources.
Customizing Authentication and Authorization Logic:
You can customize Laravel’s authentication and authorization logic to fit your application’s needs by modifying the authentication controllers or using gates and policies.
Error Handling and Debugging in Laravel
Handling errors and debugging is a crucial part of development. Laravel provides several tools to help with this.
Handling Errors in Laravel:
Laravel has a global error handler that catches exceptions and displays them in a friendly format.
You can customize error handling by modifying the App\Exceptions\Handler
class.
Logging and Debugging Tools:
Laravel integrates with Monolog for logging, and you can configure different logging channels.
For debugging, Laravel’s dd()
function and the Laravel Debugbar package are invaluable.
Common Troubleshooting Tips:
Sometimes things go wrong, and knowing where to look can save you a lot of headaches.
Always check your logs, use breakpoints, and ensure your environment is correctly set up.
Deploying a Laravel Application
Once your application is ready, deploying it to a live server is the next step.
Preparing Your Application for Production:
Before deployment, make sure to run the following commands to optimize your application:
php artisan optimize
php artisan config:cache
php artisan route:cache
Deploying to Various Hosting Environments:
Laravel applications can be deployed to shared hosting, VPS, or cloud platforms like AWS and DigitalOcean.
Laravel Forge is also an excellent tool for automating the deployment process.
Best Practices for Laravel Deployment:
Always use environment variables to manage sensitive information, ensure your application is up-to-date with security patches, and use a robust backup strategy.
Conclusion
Laravel is a powerful and versatile PHP framework that can handle projects of any size.
By mastering Laravel, you open up a world of possibilities in web development, from simple websites to complex applications.
This guide has provided a comprehensive overview, but the best way to learn is by doing.
So dive in, start building, and explore the full potential of Laravel.
FAQs
What makes Laravel stand out from other PHP frameworks?
Laravel's elegant syntax, comprehensive features, and strong community support make it one of the most developer-friendly frameworks available.
Can I use Laravel for small projects?
Absolutely! Laravel is scalable and can be used for both small and large projects.
How does Laravel handle security concerns?
Laravel has several built-in security features, including CSRF protection, hashed passwords, and SQL injection prevention.
What are some common mistakes to avoid when using Laravel?
Common mistakes include not using environment variables, failing to optimize for production, and neglecting security best practices.
How can I contribute to the Laravel community?
You can contribute by reporting bugs, submitting pull requests, writing tutorials, or participating in the Laravel forums and discussions.
Subscribe to my newsletter
Read articles from Abdulmunim Abdulsalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
