Git branching and merging.

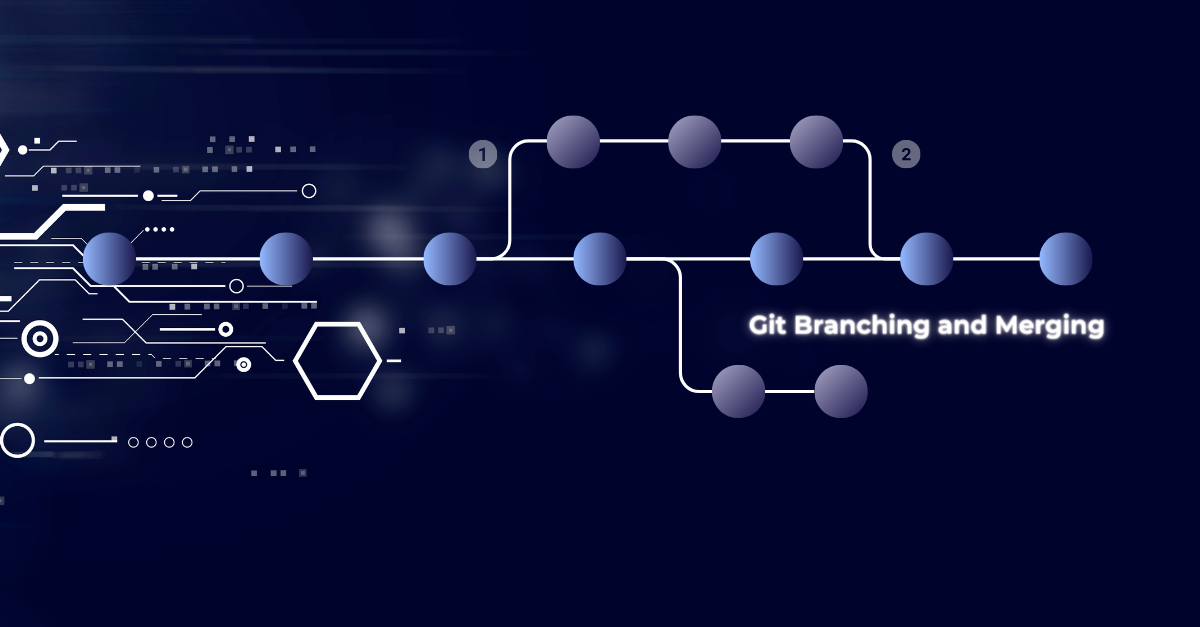
In the world of creating cool software, there's a secret sauce called "version control." Imagine you're working on a digital masterpiece with your buddies, each tweaking and improving the code. Without version control, things can get messy—like a crazy art project with no plan.
That's where Git comes in. It's not just a tool; it's like a superhero for developers. Created by the genius behind Linux, Git helps us keep track of changes, go back in time, and work together without chaos.
In this journey, we'll explore Git's cool features, focusing on something important: branching and merging. These are like the magic spells that let developers try out new ideas, work on different things at once, and then put it all together seamlessly.
So, get ready to explore the world of Git branches and merges. We're about to unravel the secrets of working together on code, making it awesome, and having a blast while doing it!
Basics of Git
Before we dive into the exciting world of Git branching and merging, let's lay down the foundation by exploring the basics of Git. Git is like a time-traveling wizard for code, allowing developers to track changes, collaborate seamlessly, and undo mistakes. Let's unravel the fundamental concepts that make Git the superhero of version control.
1. Understanding Git's Essence
Version Control: In the digital realm of software development, version control is akin to a safety net. It enables developers to track changes made to their code, roll back to previous versions, and collaborate effectively with others. Git, designed by Linus Torvalds, takes this concept to a whole new level.
Distributed Version Control: Git operates on a distributed version control system, meaning each developer has a complete copy of the project's history on their local machine. This decentralization empowers teams to work independently and merge their changes later.
2. The Three States of Git
Working Directory: This is where you make changes to your files. The working directory is like your sandbox, where you can add, modify, or delete files as you please.
Staging Area (Index): Before changes are permanently recorded, they need to be added to the staging area. Think of it as a checkpoint where you decide which modifications are ready to be saved.
Repository (HEAD): Once changes are staged, you commit them to the Git repository. The repository, often referred to as HEAD, is your project's memory, preserving each committed version of your code.
3. Key Git Commands
git init: Initialize a new Git repository in your project.
git add [file]: Add changes to the staging area before committing.
git commit -m "[commit message]": Save changes to the repository with a descriptive commit message.
git status: Check the status of your working directory, staging area, and repository.
git log: View the commit history, showing who made changes and when.
4. Ignoring Unwanted Files
Create a .gitignore
file to specify files or directories that Git should ignore, such as temporary files or dependencies.
5. Connecting with Remote Repositories
git remote add origin [remote repository URL]: Link your local repository to a remote repository (e.g., on GitHub or GitLab).
git push origin [branch]: Upload your local changes to the remote repository.
git pull origin [branch]: Download changes from the remote repository to your local machine.
These basics set the stage for our journey into Git branching and merging. As we delve deeper, remember that Git is not just a tool; it's a powerful ally that empowers developers to collaborate, experiment, and build extraordinary software. Now, let's embark on the next leg of our adventure: Git branching!
Branching in Git
In the realm of Git, branching is the wizardry that allows developers to create parallel universes of code, experiment with new features, and collaborate without stepping on each other's toes. Let's embark on a journey into the enchanting world of Git branching.
1. The Essence of Branches
What is a Branch? In Git, a branch is like a separate timeline within your project. It allows you to work on features or fixes without affecting the main codebase. Think of it as an alternate reality where you can tinker, explore, and innovate.
Why Branch? Branching serves several purposes:
Isolation: Changes made in one branch don't immediately affect others.
Collaboration: Multiple developers can work on different features simultaneously.
Experimentation: Test new ideas without disrupting the main project.
2. Creating a New Branch
Command Line Magic: Creating a new branch is as simple as a command:
git branch [branch_name]
To switch to the new branch:
git checkout [branch_name]
Or, a more concise way:
git checkout -b [branch_name]
This command not only creates a branch but also switches you to it in one go.
3. Visualizing Branches
git branch: To see a list of branches and identify the active one, use:
git branch
git log --graph: For a visual representation of your branch history:
git log --graph --oneline --all
4. Switching Between Branches
git checkout [branch_name]: To move between branches:
git checkout [branch_name]
Or, with Git version 2.23 and later:
git switch [branch_name]
5. The Main Branch (Master or Main)
In Git, you often encounter a default main branch (commonly named master
or main
). This is the primary branch and is considered stable. When starting a new feature or fix, it's a good practice to create a new branch from the main branch.
6. Deleting Branches
git branch -d [branch_name]: To delete a branch (after merging changes):
git branch -d [branch_name]
To force delete without merging:
git branch -D [branch_name]
Branching in Git is the secret sauce that allows developers to innovate fearlessly. With the power to branch, experiment, and merge seamlessly, Git sets the stage for collaborative coding adventures. In the next chapter, we'll unravel the art of committing changes to these branches. Stay tuned!
Why Create a New Branch?
Creating a new branch in Git serves several crucial purposes in the development workflow:
Isolation of Changes: By creating a new branch, you can isolate your work from the main codebase. This isolation ensures that any changes or experiments you undertake do not directly affect the stable version of the software.
Feature Development: Branches are commonly used to develop new features or functionalities. You can work on implementing a new feature without interfering with ongoing development in other parts of the project.
Bug Fixes: Similarly, branches are useful for addressing bugs or issues in the codebase. By creating a dedicated branch for bug fixes, you can focus on resolving the issue without disrupting other development efforts.
Experimentation: Branches provide a safe space for experimentation. Developers can try out different approaches, test new libraries or technologies, and explore creative solutions without risk to the main project.
Creating a New Branch
Using git checkout -b
:
To create a new branch and switch to it in one step, you can use the git checkout -b
command followed by the desired branch name.
git checkout -b new_feature_branch
This command simultaneously creates a new branch named new_feature_branch
and switches you to it, allowing you to start working on your new feature immediately.
Using git switch -c
(Git version 2.23 and later):
Alternatively, if you're using Git version 2.23 or later, you can use the git switch -c
command to achieve the same result.
git switch -c new_feature_branch
Like git checkout -b
, this command creates a new branch named new_feature_branch
and switches you to it in a single step.
Verifying the New Branch:
To confirm that the new branch has been created successfully, you can use the git branch
command:
git branch
This command lists all branches in the repository, highlighting the current branch with an asterisk (*). You should see your newly created branch listed among the others.
Committing Changes to a Branch: Capturing Progress in Git
Once you've created a new branch in Git and started working on your feature or fix, the next step is to commit your changes. Committing changes records your progress and creates a snapshot of the current state of your code. Let's delve into how to make changes in a branch and commit them using Git.
Making Changes in a Branch
Navigate to Your Branch: Before making changes, ensure you're in the correct branch where you want to work. Use the
git checkout
orgit switch
command to switch to your branch if you're not already in it:git checkout your_branch_name
or
git switch your_branch_name
Modify Files: Make the necessary changes to your code using your preferred text editor or IDE. You can add, edit, or delete files as required to implement your feature or fix bugs.
Verify Changes: After making modifications, you can use the
git status
command to see which files have been modified. This command provides an overview of the changes you've made since the last commit:git status
Committing Changes
Stage Changes: Before committing, you need to stage the changes you want to include in the commit. Use the
git add
command followed by the filenames or directories of the modified files:git add file1.py file2.py directory/
Verify Staging: To verify that your changes have been staged successfully, you can again use the
git status
command. Staged changes will be listed under "Changes to be committed."Commit Changes: Once your changes are staged, you're ready to commit them. Use the
git commit
command followed by a descriptive commit message that summarizes the changes you've made:git commit -m "Add feature X functionality"
Emphasizing the Isolation of Changes
One of the key benefits of Git branching is the isolation it provides for changes. When you commit changes to a branch, those changes are confined to that branch until they're merged into another branch. This isolation allows you to work independently on different features or fixes without affecting the main codebase or other developers' work.
By committing changes to a branch, you maintain a clean and organized development workflow. Each branch represents a specific task or set of changes, making it easier to track progress, review code, and collaborate effectively with team members.
Merging in Git: A Comprehensive Guide
Introduction
Merging in Git is a fundamental operation that combines the changes from one branch into another. Whether you're integrating a feature branch into the main branch or resolving conflicts between different development streams, mastering the Git merge process is crucial for smooth collaboration in software development.
In this guide, we’ll cover:
Types of Merges in Git
Common Merge Scenarios
Step-by-Step Process for Merging
Handling Merge Conflicts
Best Practices for Effective Merging
1. Types of Merges in Git
a) Fast-Forward Merge
A fast-forward merge happens when the target branch has not diverged from the current branch. In this case, Git simply moves the pointer forward, making it appear as though the branches were always in sync.
shCopy codegit checkout main
git merge feature-branch
If the main
branch has not changed since feature-branch
was created, the merge will be a simple fast-forward.
b) Three-Way Merge
When the branches have diverged and contain independent changes, Git performs a three-way merge. It uses the common ancestor of the two branches to produce a merged result.
shCopy codegit checkout main
git merge feature-branch
If both branches have unique commits, Git will perform a three-way merge, combining the changes from both branches.
c) Rebase (Alternative to Merging)
Rebasing is another way to integrate changes from one branch into another. Instead of merging, rebasing rewrites the commit history by placing your changes on top of the target branch.
shCopy codegit checkout feature-branch
git rebase main
Rebasing is often used to keep a clean, linear commit history.
2. Common Merge Scenarios
Feature Branch Integration: Merging a feature branch back into the
main
branch after development is complete.Hotfix Branch Integration: Merging a hotfix branch into the
main
anddevelop
branches simultaneously.Team Collaboration: Merging changes from multiple developers working on different branches.
3. Step-by-Step Process for Merging
a) Start by Updating Your Local Repository:
First, make sure your local repository is up to date.
shCopy codegit fetch origin
git pull origin main
b) Switch to the Target Branch:
Move to the branch where you want to integrate changes.
shCopy codegit checkout main
c) Perform the Merge:
Merge the feature branch into your current branch.
shCopy codegit merge feature-branch
Git will attempt to automatically merge the changes. If it succeeds without conflicts, a merge commit is created.
d) Push the Merged Changes:
After a successful merge, push the updated branch back to the remote repository.
shCopy codegit push origin main
4. Handling Merge Conflicts
Merge conflicts occur when Git cannot automatically resolve differences between branches. Here’s how to handle them:
a) Identify Conflicted Files:
Git will list the files with conflicts during the merge.
shCopy codegit status
b) Resolve the Conflicts Manually:
Open each conflicted file. Git marks the conflicting sections like this:
plaintextCopy code<<<<<<< HEAD
Your changes
=======
Changes from feature-branch
>>>>>>> feature-branch
Manually edit the file to choose the correct content, then remove the conflict markers (<<<<<<<
, =======
, >>>>>>>
).
c) Mark the Conflict as Resolved:
After editing the file, mark it as resolved.
shCopy codegit add path/to/file
d) Complete the Merge:
Finish the merge with a commit.
shCopy codegit commit -m "Resolved conflicts and merged feature-branch"
e) Push the Resolved Merge:
Push the changes back to the remote repository.
shCopy codegit push origin main
5. Best Practices for Effective Merging
Keep Branches Up to Date: Regularly sync your feature branches with the target branch to minimize conflicts.
Commit Frequently: Smaller, well-defined commits make conflict resolution easier.
Use Descriptive Commit Messages: Clear commit messages help teammates understand changes and resolve conflicts.
Avoid Large Merge Commits: Break down large changes into smaller, manageable chunks for easier merging.
Test After Merging: Always run tests and verify functionality after performing a merge to ensure nothing breaks.
Conclusion
Branching and 2Merging is a powerful feature in Git that allows teams to work independently while maintaining a single, integrated codebase. By understanding the different types of merges, handling conflicts efficiently, and following best practices, you can streamline your development process and avoid common pitfalls.
Happy merging!
This guide should give you a comprehensive understanding of how to approach branching and merging in Git, whether you are using Bitbucket, GitHub, or any other Git-based platform.
Subscribe to my newsletter
Read articles from Nainaz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
