Top JavaScript Closure Mistakes Every Developer Should Avoid
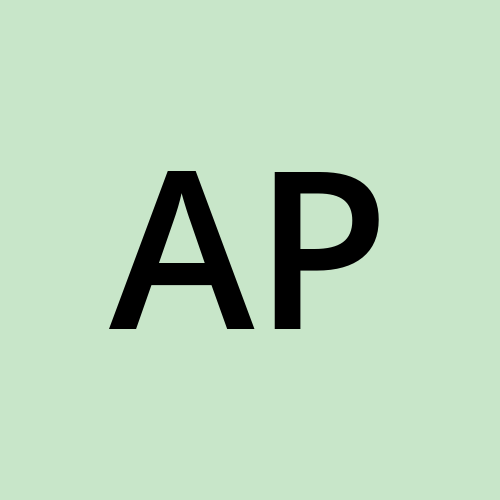
Common Mistakes Developers Make with Closures in JavaScript
Closures in JavaScript are a powerful feature that allow functions to have access to variables from their outer scope, even after the outer function has finished executing. This ability can lead to highly efficient and elegant code, but it also comes with its own set of common pitfalls. Here’s a look at some of the most frequent mistakes developers make with closures and how to avoid them.
1. Not Understanding Variable Scope
One of the fundamental mistakes is misunderstanding how closures interact with variable scope. Developers often expect that each closure will have its own copy of a variable when, in reality, closures share access to the same variable instance.
Example:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter1 = createCounter();
const counter2 = createCounter();
console.log(counter1()); // 1
console.log(counter2()); // 1
Mistake: Developers might expect counter1
and counter2
to share the same count
variable, but each createCounter
invocation creates a new scope, so the counters are independent.
Solution: Always be mindful of where and how variables are initialized and used within closures. If you need shared state across closures, consider using a shared object or a different approach.
2. Overusing Closures for Private Data
Closures are often used to create private variables and methods. While this is a good practice for encapsulation, overusing closures can lead to memory leaks and performance issues, especially if closures retain large amounts of data or are created in a loop.
Example:
function createLargeDataClosure() {
let largeArray = new Array(1000000).fill(0);
return function() {
// Some operation on largeArray
};
}
const largeDataClosure = createLargeDataClosure();
Mistake: If largeDataClosure
is not cleaned up properly, the largeArray
will remain in memory, potentially leading to memory leaks.
Solution: Be judicious in using closures for private data. Ensure you release references to large data when no longer needed and consider alternative encapsulation techniques if memory usage becomes a concern.
3. Confusing Closures with Async Operations
Closures and asynchronous operations can interact in complex ways, leading to unexpected results. A common mistake is assuming that a closure will "capture" a value at a specific point in time, especially inside loops.
Example:
function createFunctions() {
const functions = [];
for (var i = 0; i < 5; i++) {
functions.push(function() {
return i;
});
}
return functions;
}
const funcs = createFunctions();
console.log(funcs.map(fn => fn())); // [5, 5, 5, 5, 5]
Mistake: All functions in funcs
return 5
because the variable i
is shared and modified in each iteration. By the time the functions are called, i
is 5
.
Solution: Use let
instead of var
to create a new binding in each iteration:
function createFunctions() {
const functions = [];
for (let i = 0; i < 5; i++) {
functions.push(function() {
return i;
});
}
return functions;
}
4. Forgetting About Closure Side Effects
Closures can sometimes lead to unexpected side effects if not carefully managed. This is particularly true when closures are used inside loops or with asynchronous callbacks.
Example:
function createHandlers() {
const handlers = [];
for (let i = 0; i < 3; i++) {
handlers.push(() => {
setTimeout(() => console.log(i), 1000);
});
}
return handlers;
}
const handlers = createHandlers();
handlers.forEach(handler => handler()); // Prints 3 three times after 1 second
Mistake: Closures capture the loop variable, and by the time the setTimeout
callback executes, i
is 3
.
Solution: Use an immediately invoked function expression (IIFE) to create a new scope for each iteration:
function createHandlers() {
const handlers = [];
for (let i = 0; i < 3; i++) {
handlers.push(((i) => {
return () => {
setTimeout(() => console.log(i), 1000);
};
})(i));
}
return handlers;
}
5. Ignoring Performance Implications
Closures can sometimes introduce performance issues if not used wisely. Keeping too many references or creating too many closures in a performance-critical section of code can lead to inefficiencies.
Example:
function generateHandlers() {
const handlers = [];
for (let i = 0; i < 1000; i++) {
handlers.push(() => console.log(i));
}
return handlers;
}
Mistake: Generating a large number of closures can increase memory usage and reduce performance, especially in high-load scenarios.
Solution: Optimize by minimizing the number of closures or using alternative patterns if necessary.
Conclusion
Closures are a fundamental and powerful concept in JavaScript that, when used correctly, can lead to more modular and maintainable code. However, it's crucial to understand their nuances and potential pitfalls. By being aware of these common mistakes and applying the solutions provided, you can leverage closures effectively while avoiding common traps.
Feel free to share this blog with fellow developers or use it as a reference in your coding journey!
Subscribe to my newsletter
Read articles from Akshara Purwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
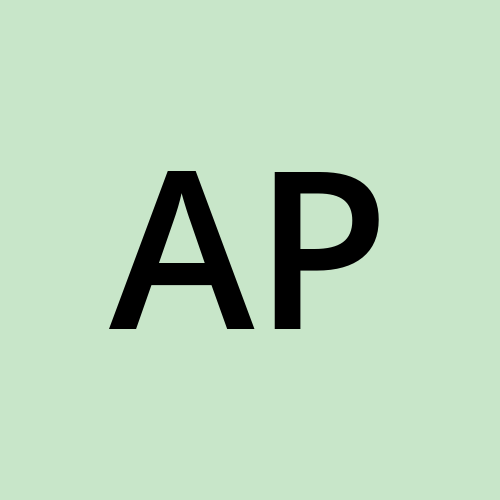