Introduction to Mapbox and GeoJSON.io in Web Technologies

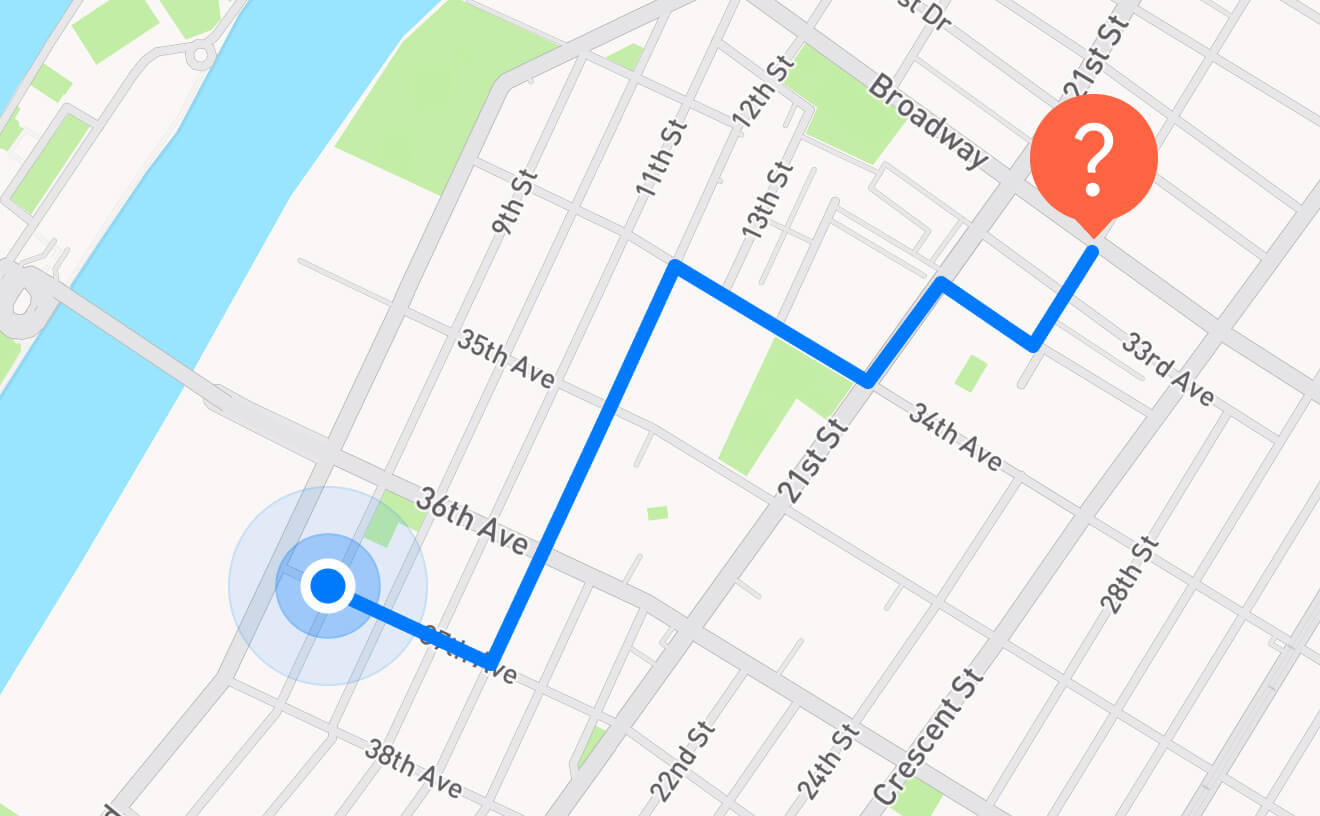
Web development is ever-evolving, and tools like Mapbox and GeoJSON.io have made it easier to integrate interactive maps and geospatial data into your web applications. Whether you're building a simple location-based service or a complex geospatial analytics platform, these tools can significantly enhance the user experience.
This blog will walk you through the practical integration of Mapbox and GeoJSON.io in a React application, focusing on real-world use cases and hands-on examples.
What is Mapbox?
Mapbox is a powerful mapping and location platform that allows developers to create highly customizable maps for their web and mobile applications. It offers an extensive API for embedding maps, adding custom markers, handling user interactions, and much more.
What is GeoJSON.io?
GeoJSON.io is a web-based tool that lets you easily create, edit, and visualize GeoJSON data. GeoJSON is a format for encoding a variety of geographic data structures, making it ideal for handling geospatial data in your applications.
Use Cases in React
Before diving into the code, let’s explore some practical use cases for integrating Mapbox and GeoJSON.io in a React app:
Interactive Store Locator: Allow users to find stores or service locations nearby.
Real-Time Tracking: Display live tracking of vehicles, people, or shipments.
Custom Geospatial Analysis: Visualize data like heatmaps, population density, or climate zones.
Event Mapping: Show event locations, routes, or attendee density in real-time.
Getting Started with Mapbox in React
To start using Mapbox in a React app, you'll need an API key from Mapbox. You can sign up and obtain a free key from the Mapbox website.
1. Setting Up the Project
First, create a new React project:
npx create-react-app mapbox-demo
cd mapbox-demo
Install the required dependencies:
npm install react-map-gl mapbox-gl
2. Creating a Map Component
Create a new component called Map.js
:
import React, { useState } from 'react';
import ReactMapGL, { Marker } from 'react-map-gl';
const Map = () => {
const [viewport, setViewport] = useState({
latitude: 18.5204,
longitude: 73.8567,
zoom: 10,
width: '100%',
height: '400px',
});
return (
<ReactMapGL
{...viewport}
mapboxApiAccessToken={process.env.REACT_APP_MAPBOX_TOKEN}
onViewportChange={viewport => setViewport(viewport)}
mapStyle="mapbox://styles/mapbox/streets-v11"
>
<Marker latitude={18.5204} longitude={73.8567}>
<div>
<img src="marker-icon.png" alt="marker" />
</div>
</Marker>
</ReactMapGL>
);
};
export default Map;
In this example, replace REACT_APP_MAPBOX_TOKEN
with your actual Mapbox API token in your .env
file:
REACT_APP_MAPBOX_TOKEN=your_mapbox_token_here
3. Integrating the Map Component
Now, integrate the Map
component into your main App.js
file:
import React from 'react';
import Map from './Map';
function App() {
return (
<div className="App">
<h1>My Mapbox Demo</h1>
<Map />
</div>
);
}
export default App;
Run your project :
npm start
You should see a map centered on Pune with a custom marker.
Using GeoJSON.io with Mapbox
GeoJSON.io can be used to create and export GeoJSON data, which can then be integrated into your Mapbox maps.
1. Creating GeoJSON Data
Go to GeoJSON.io and create a simple polygon or point. Once you're done, export the data as a GeoJSON file.
2. Loading GeoJSON Data in Mapbox
You can now load this GeoJSON data into your Mapbox map. Modify the Map.js
component to include GeoJSON data:
import React, { useState, useEffect } from 'react';
import ReactMapGL, { Source, Layer } from 'react-map-gl';
const Map = () => {
const [viewport, setViewport] = useState({
latitude: 18.5204,
longitude: 73.8567,
zoom: 10,
width: '100%',
height: '400px',
});
const geojson = {
type: 'FeatureCollection',
features: [
{
type: 'Feature',
geometry: {
type: 'Point',
coordinates: [18.5204, 73.8567],
},
properties: {
title: 'Pune',
},
},
],
};
return (
<ReactMapGL
{...viewport}
mapboxApiAccessToken={process.env.REACT_APP_MAPBOX_TOKEN}
onViewportChange={viewport => setViewport(viewport)}
mapStyle="mapbox://styles/mapbox/streets-v11"
>
<Source id="my-data" type="geojson" data={geojson}>
<Layer
id="point"
type="circle"
paint={{
'circle-radius': 10,
'circle-color': '#007cbf',
}}
/>
</Source>
</ReactMapGL>
);
};
export default Map;
Expanding the Use Cases
With Mapbox and GeoJSON.io, the possibilities are nearly endless. Here are a few ideas to expand on the basic example:
User-Generated Content: Allow users to draw and save their own GeoJSON data on the map.
Data-Driven Styling: Use Mapbox's powerful styling options to visually represent different datasets, such as weather patterns or traffic conditions.
Advanced Filtering: Implement filters to show or hide specific features based on user input.
Conclusion
Mapbox and GeoJSON.io are invaluable tools for adding geospatial features to your web applications. In this blog, we’ve covered how to set up a basic React app with Mapbox, load custom GeoJSON data, and explored practical use cases. With these skills, you can create dynamic, interactive maps that significantly enhance your application's functionality and user experience.
Get started with your own Mapbox and GeoJSON projects today, and bring the power of interactive mapping to your web applications!
Subscribe to my newsletter
Read articles from NITYOM TIKHE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NITYOM TIKHE
NITYOM TIKHE
I am a dedicated Frontend Engineer with a strong focus on building responsive and innovative web applications. Proficient in React, Vite, and Framer Motion, I specialize in creating dynamic user experiences. With a background in both web development and algorithmic problem-solving, I bring technical expertise and creativity to every project. Let's connect and collaborate on exciting tech ventures!