Understanding The Basics Of LangChain

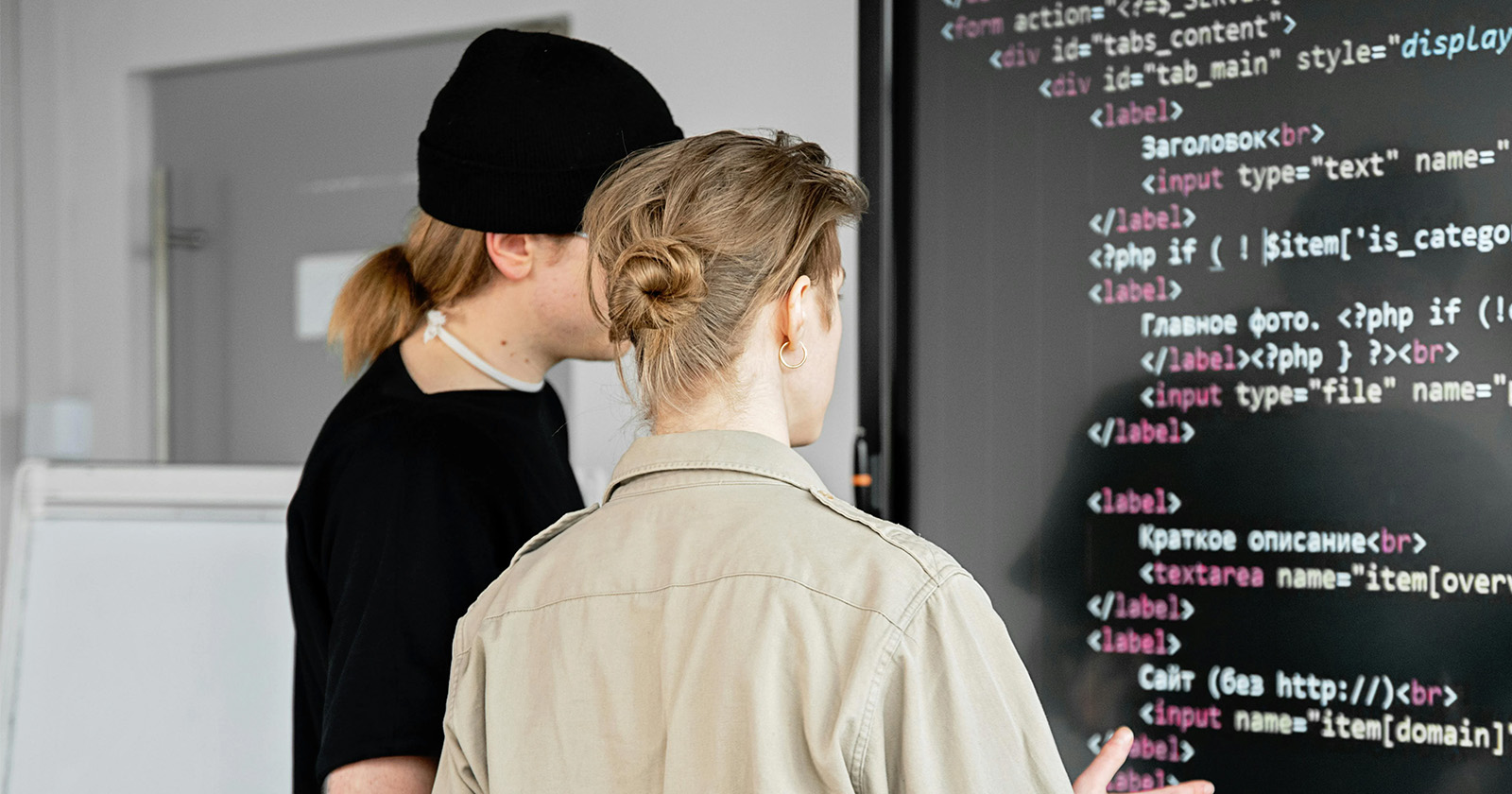
LangChain is turning into an awesome framework that simplifies building applications with Large Language Models (LLMs). As generative AI continues to grow, it's crucial for developers and engineers to understand how to use tools like LangChain effectively. In this post, I'll walk you through the basics of LangChain, its components, and how it works with LLMs to create advanced applications.
1. Generative AI And Large Language Models (LLMs)
Generative AI is about creating text, images, or other media from input data using artificial intelligence. These systems learn patterns from huge datasets and use that knowledge to make new content. The magic behind Generative AI comes from Neural Networks, especially those used in Deep Learning.
Large Language Models (LLMs) are a special kind of Generative AI that focuses on understanding and creating human-like text. They're trained on tons of text data and can do all sorts of language tasks like translation, summarization, and conversation.
2. What Is LangChain?
LangChain is a framework that helps you create applications that interact with LLMs. It provides tools and add-ons that make it easier to integrate and use LLMs, allowing developers to build, customize, and deploy advanced language model-based applications efficiently.
LangChain works with several popular LLM providers, including:
OpenAI: Famous for models like GPT-3 and GPT-4.
Anthropic: Creators of Claude.
Google: Known for advanced language models like BERT and T5.
3. What You Can Build With LangChain?
Here are some popular types of applications where LLMs are particularly powerful:
Chatbots and Conversational Agents: Virtual assistants for customer support, personal assistants for scheduling and reminders, and interactive educational tutors.
Content Generation: Creative writing assistance (stories, poems), marketing copy generation (ads, product descriptions).
Document Processing and Analysis: Summarization of long documents, automated report generation.
Question Answering Systems: Knowledge base assistants for technical support, interactive FAQ systems, and personalized information retrieval.
4. Let's Get Started With LangChain
4.1. LangChain Components
LangChain's architecture is modular, made up of several key components that work together to make developing LLM-based applications easier.
4.1.1. Models
LangChain models are interfaces that let you interact with large language model APIs. There are two main types of models in LangChain:
LLM Models: LLM models in LangChain refer to text completion models. These models take a string as input and return another string as output.
# import the OpenAI class from the langchain_openai module from langchain_openai import OpenAI # create an instance to interact with OpenAI language models llm = OpenAI() # input text text = "What would be one good meal for breakfast?" # the "invoke" method sends the input text to the model and waits it to generate a response # the response is a string llm_output = llm.invoke(text) print(llm_output)
Chat Models: Chat models are specifically tuned for conversational purposes. They take a list of messages as input and return a message as output.
# import the ChatOpenAI class from the langchain_openai module from langchain_openai import ChatOpenAI # this class is used to create message objects that represent human inputs from langchain_core.messages import HumanMessage # create an instance specifying which model use passing the model name as a parameter chat_model = ChatOpenAI(model="gpt-4o-mini") # input text = "What would be one good meal for breakfast?" messages = [HumanMessage(content=text)] # the "invoke" method sends the input to the model and waits it to generate a response # the response is an object of type AIMessage chat_model_output = chat_model.invoke(messages) print(chat_model_output.content)
LangChain supports various providers and platforms. For example, you can use the OpenAI API with an API key or run models locally with platforms like Ollama.
# use the llama2 model running locally
# the code is basically the same as in previous examples
from langchain.chat_models import ChatOllama
chat_model = ChatOllama(model="llama2")
output = chat_model.invoke("Hi!")
print(output.content)
4.1.2. Prompt Templates
Prompt templates in LangChain take the user's inputs and turn them into the final text or messages sent to the model.
Basic Prompt Templates: Handle simple transformations of user input.
# creating a basic prompt from langchain.prompts import PromptTemplate template_string = "List the ingredients of the following recipe: {meal}" prompt_template = PromptTemplate.from_template(template_string) prompt_template.format(meal="waffles")
Chat Prompt Templates: Used for structuring chat messages, including roles and content guidelines.
# creating a chat_prompt using a tuple from langchain.prompts.chat import ChatPromptTemplate system_message = "You are a famous chef." human_template = "List the ingredients of the following recipe: {meal}" chat_prompt_tuple = ChatPromptTemplate.from_messages([ ("system", system_message), ("human", human_template) ]) chat_prompt_tuple.format_messages(meal="waffles")
# creating a chat_prompt using objects from langchain.prompts import HumanMessagePromptTemplate from langchain_core.messages import SystemMessage systen_message = SystemMessage("You are a famous chef.") human_template = HumanMessagePromptTemplate.from_template("List the ingredients of the following recipe: {meal}") chat_prompt_objects = ChatPromptTemplate.from_messages([ systen_message, human_template ]) chat_prompt_objects.format_messages(meal="waffles")
4.1.3. Output Parsers
Output parsers in LangChain take the raw output from LLMs and turn it into a more user-friendly format, like structured data (e.g., a comma-separated list or JSON blob).
LangChain provides different types of output parsers. Check this link to view all the types and more info about them.
# string output parser example
from langchain_core.output_parsers import StrOutputParser
content = "I like Waffles"
str_output_parser = StrOutputParser()
str_output_parser.parse(content)
# csv output parser example
from langchain_core.output_parsers import CommaSeparatedListOutputParser
content = "2 cups flour, 1 teaspoon salt, 2 eggs, 2 tablespoons white sugar, 1.5 cups warm milk"
csv_output_parser = CommaSeparatedListOutputParser()
csv_output_parser.parse(content)
# json output parser example
from langchain_core.output_parsers import JsonOutputParser
content = '{"recipe": "waffles", "ingredients": "2 cups flour, 1 teaspoon salt, 2 eggs, 2 tablespoons white sugar, 1.5 cups warm milk"}'
json_output_parser = JsonOutputParser()
json_output_parser.parse(content)
4.2. Chains In LangChain
Chains are components that link prompts, LLMs, and output parsers into a building block, enabling you to create more interesting and complex functionality.
A chain connects the basic components (model, prompt template, and output parser) into a block that can be run separately. This allows you to turn workflows using LLMs into a modular process of composing components.
$$Chain = Model + Prompt + OutputParser$$
4.2.1. LangChain Expression Language (LCEL)
LangChain Expression Language (LCEL) is an easy way to create chains. It allows you to build complex chain pipelines using a simple, standard interface.
The building blocks and abstractions provided by LangChain make this library unique. It offers tools you didn't know you needed to create amazing projects powered by LLMs.
chain = prompt | llm | output_parser
4.2.2. LCEL - Runnables
The "Runnable Protocol" makes it easier to create custom chains and use them in a standard way. You can read more about the "Runnable Protocol" at this link.
from langchain_openai import ChatOpenAI
from langchain.prompts import PromptTemplate
from langchain_core.output_parsers import CommaSeparatedListOutputParser
chat_model = ChatOpenAI(model="gpt-4o-mini")
template_string = "List the ingredients of the following recipe: {meal}"
prompt_template = PromptTemplate.from_template(template_string)
output_parser = CommaSeparatedListOutputParser()
# using LCEL to declare a custom chain
chain = prompt_template | chat_model | output_parser
chain.invoke({"meal": "waffles"})
5. Bonus Material
If this blog post catches your attention, you should check out this Jupyter Notebook about LangChain.
Conclusion
Here's a quick image that sums up the main points we talked about in this post.
LangChain is a versatile and powerful framework for building applications that use large language models. By getting to know its components and how they work together, developers can create strong and efficient LLM-based applications. Whether you're working on chatbots, search engines, or educational tools, LangChain gives you the essential building blocks for success.
Subscribe to my newsletter
Read articles from Olman Ureña directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Olman Ureña
Olman Ureña
Full Stack Software Engineer skilled in Traditional and Generative AI