Args and Kwargs: The Dynamic Duo of Python Programming - A Comedy of Errors and Efficiency
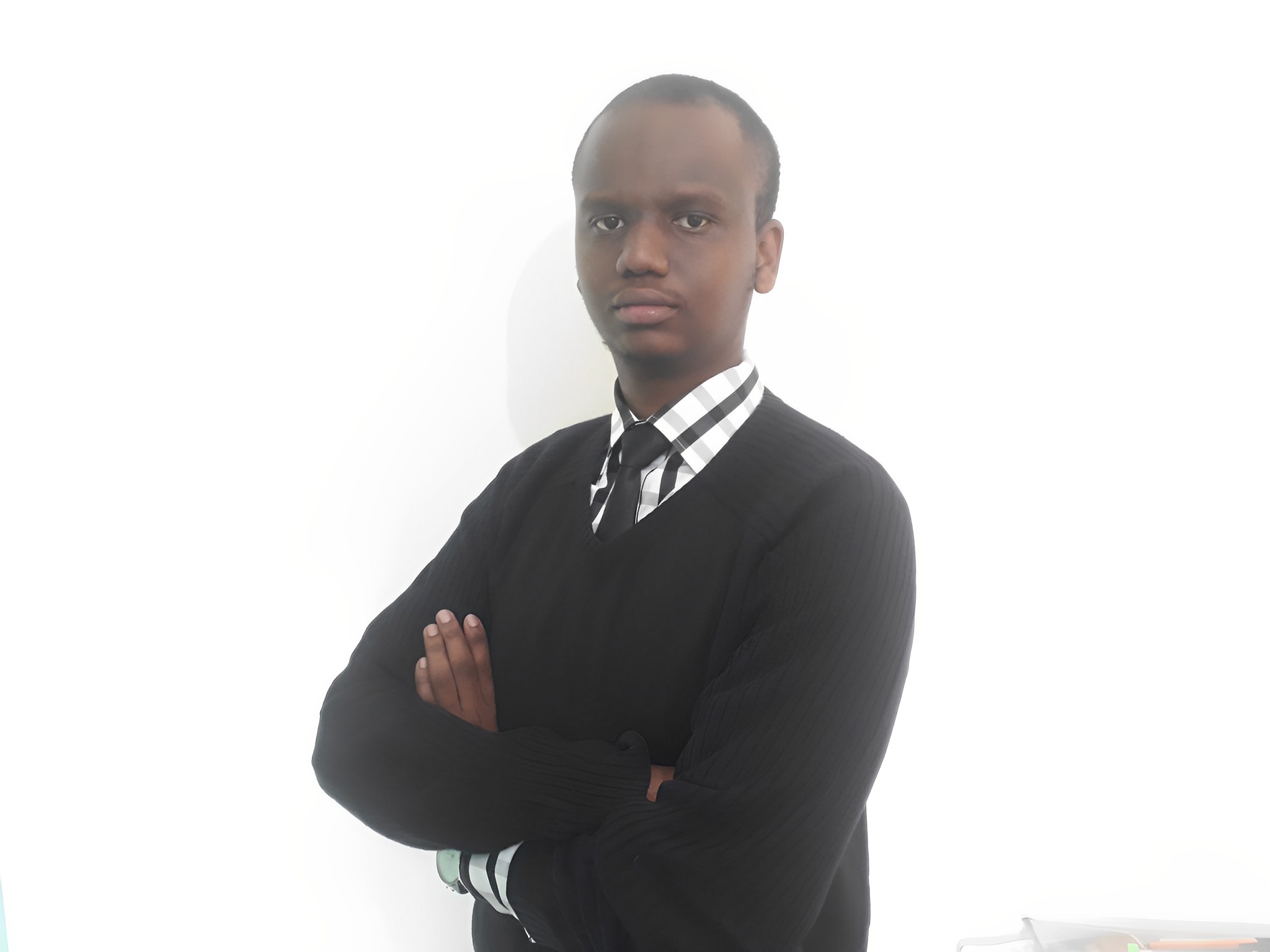
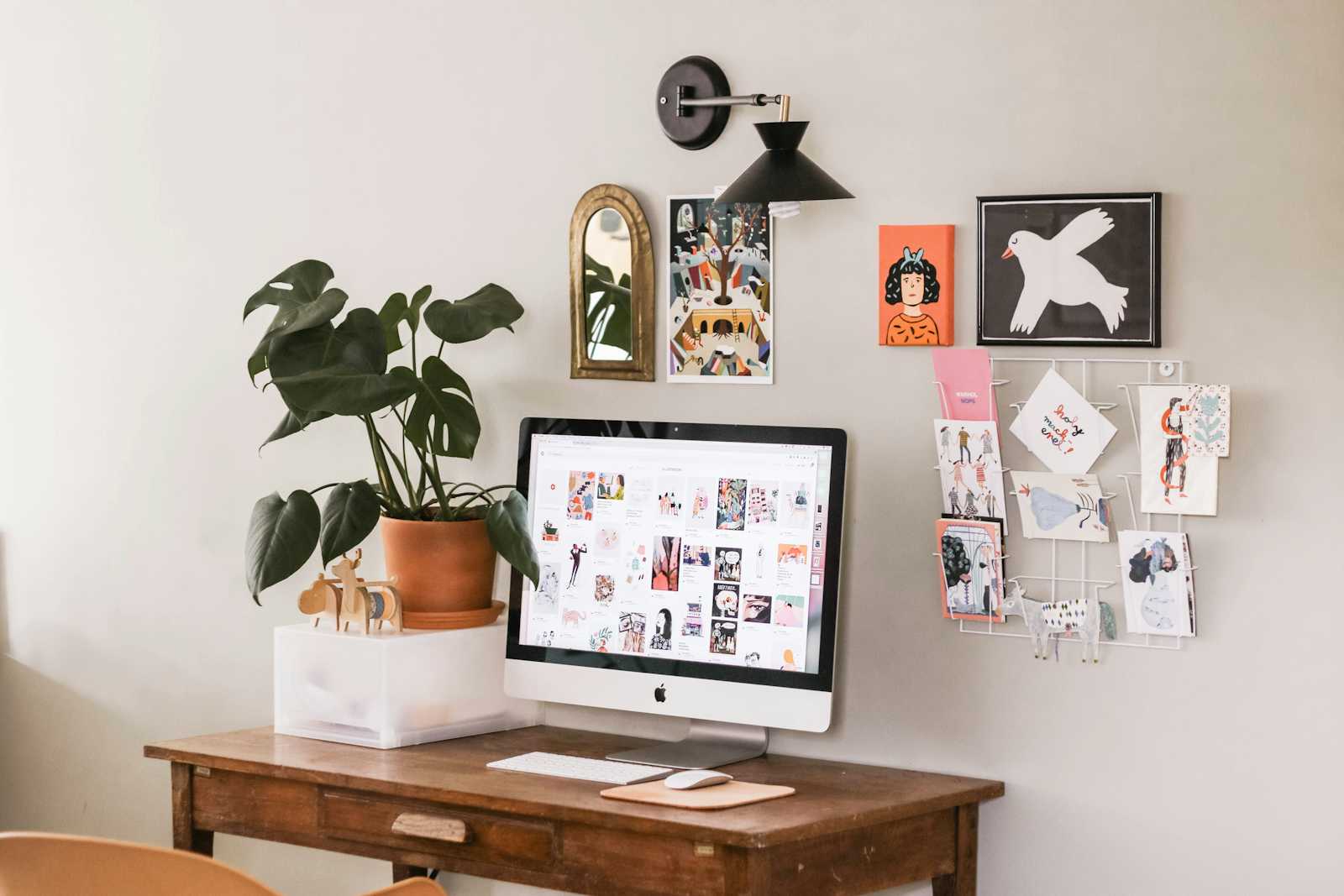
In the last article, I mentioned that we would build a receipt generator using Python's args
and kwargs
. We'll start with a simple version using args
and then move on to a more complex version. I'll do my best to make everything easy to understand.
Example 1
The first to do is to define a function named
generate_receipt
*items: str
: The*items
parameter allows the function to accept any number of positional arguments. The type hintstr
indicates that each item is expected to be a string.-> None
: This type of hint specifies that the function does not return a value.The
print("Receipt:")
prints the word "Receipt:" to the console, indicating the start of the receipt.for item in items:
: This line starts a loop that iterates over each item in theitems
tuple.print(f"- {item}")
: For each item, this line prints the item preceded by a hyphen and a space. Thef
before the string denotes an f-string, which allows embedding expressions inside string literals.Finally, let's call the
generate_receipt
function with three string arguments: "Apples", "Bananas", and "Cherries" and you should see the below output:Example 2
Next, we'll use the input function to let the buyer add as many groceries as they want and then print them out on a receipt. You'll notice I've divided the code with # comments. For example, the first part of the code has:
# Start of part 1
Python code appears here
# End of part 1
The code's first part is like this. Let's explain it.
- The first part of the function works the same way as it does in example 1
items = []
: Initialize an empty list to store the itemsThe
while True
: Start an infinite loop to continuously prompt the user for inputitem = input("Enter an item (or type 'done' to finish): ")
: Prompt the user to enter an itemif item.lower() == 'done': break
: If the user types 'done', exit the loop.items.append(item)
: Add the entered item to the list.The function is called with the user-provided items. The
*items
unpacks the list into individual arguments. Therefore, you should get the below result.
Example 3
The last code will get a bit more complicated. Let's begin.
You don't have to use dict[str, Union[int, float]]
or import them from typing import Union
. You can make a basic dictionary; that is all you need. I like to write my dictionaries that way. If you want to understand the topic of dictionaries, see my article titled "Python Dictionaries: The Most Fun Way to Organize Your Data". Let's break down the below function.
- Function definition
- The function
generate_receipt
takes any number of item names as arguments (using*items
).
Generating Receipt
It starts by printing "Receipt:".
It then loops through each item the user provided:
If the item exists in the
item_prices
dictionary, it prints the item and its price.If the item isn’t in the dictionary, it prints "Not found".
It keeps adding up the prices to calculate the total cost.
The user's input is handled by the code below. Please note that it’s not part of the function above. Everything from this point onward is outside the function.
This part collects item names from the user until they type 'done'. See my article on while loops titled "Python While Loops: Because Life Shouldn't Be a Never-Ending Loop".
The entered items are stored in a list called
items
.
Call the function:
Finally, the function is called with the list of items the user provides, generating a detailed receipt.
The user types in grocery items one by one.
When the user is done, the program prints out each item's price and calculates the total cost. If an item isn’t in the dictionary, it’s marked as "Not found" in the receipt below.
I hope you enjoyed the article and found it helpful! In the next article, we'll dive into local and global variables, or variable scope, to understand how they work in Python. See you then!
Subscribe to my newsletter
Read articles from JMN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
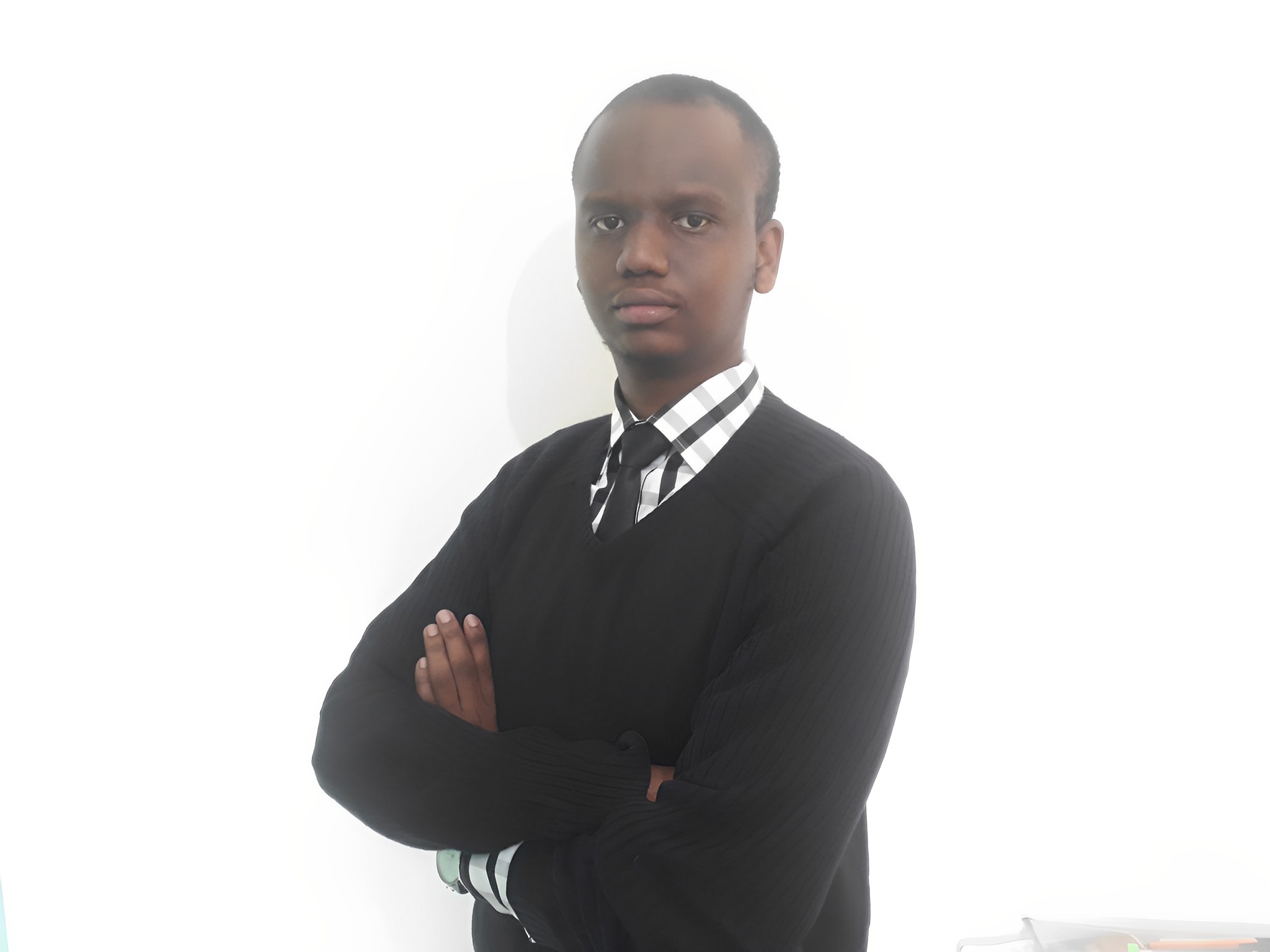
JMN
JMN
I'm JMN (Jaffery Mwangi Ndisho), and I'm thrilled to share my passion for data science and programming with you. With a background in business and IT, I've always been fascinated by the power of algorithms and their ability to transform data into insights. Through this blog, I hope to share my learning journey and practical knowledge with you, as well as explore new techniques and applications in the field. Join me on this journey and let's discover the world of data science and programming together!