Day 5 Task: Advanced Linux Shell Scripting for DevOps Engineers with User Management

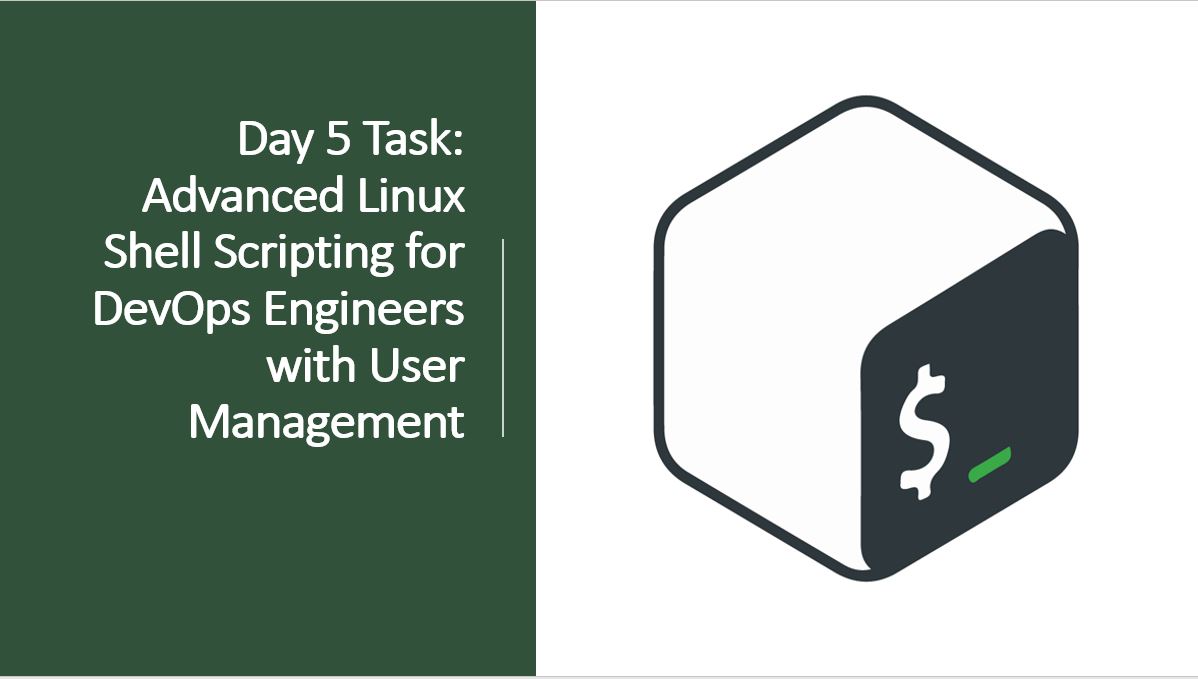
Create Directories Using Shell Script:
Write a bash script
createDirectories.sh
that, when executed with three arguments (directory name, start number of directories, and end number of directories), creates a specified number of directories with a dynamic directory name.Example 1: When executed as
./
createDirectories.sh
day 1 10
, it creates 10 directories asday1 day2 day3 ...
.#!/bin/bash # Variable name as an argument dir_name=$1 start_num=$2 end_num=$3 #for loop for checking the conditions for (( i=${start_num}; i<=${end_num}; i++ )); do # Command for making directories mkdir ${dir_name}${i} done echo "Created Directories" # this if condition check above condition work or not if [ $? -eq 0 ]; then echo "Creation of Directories script ran successfully" else echo "Creation of Directories script did not run successfully" fi
$ ./day5.sh day 1 10
Create a Script to Backup All Your Work:
Backups are an important part of a DevOps Engineer's day-to-day activities.
#!/bin/bash #Taking Inputs source_dir="/home/ubuntu/Scripts/simple_backup.sh" target_dir="/home/ubuntu/Pratice" # Timestamp to monitor the backup timing timestamp=$(date +%Y%m%d%H%M%S) #Giving filename for storing the backup backup_filename="${target_dir}/simple_backup_${timestamp}.tar.gz" # Compress Command tar -Pzcf "${backup_filename}" "${source_dir}" if [ $? -eq 0 ]; then echo "Ran succesfully" else echo "Has some error" fi
As we can see above we created the backup script of our task.
Read About Cron and Crontab to Automate the Backup Script:
What is cron?
Cron is a time-based job scheduler in Unix-like operating systems. It allows you to run scripts or commands automatically at specified intervals. Think of it as a way to schedule tasks to run at regular times without having to manually start them.
What are cron jobs in Linux?
Any task that you schedule through cron is called a cron job. Cron jobs help us automate our routine tasks, whether they're hourly, daily, monthly, or yearly.
How Cron Jobs Work
Cron Daemon: The cron service (daemon) runs in the background and checks the cron schedule for tasks to execute. It wakes up every minute to check if there’s a task that needs to be run.
Cron Table (crontab): Each user has their own crontab file where they can define their own cron jobs. This file lists all the commands or scripts that should run at specific times.
Cron Syntax
A cron job entry consists of five time and date fields followed by the command to execute. Here’s the format:
How to start Crontab -
Edit Crontab: To add or edit cron jobs for your user, use the crontab -e
command. This opens your crontab file in an editor where you can add your jobs.
Here’s what each field represents:
Minute:
0-59
Hour:
0-23
(0 is midnight, 23 is 11 PM)Day of the Month:
1-31
Month:
1-12
Day of the Week:
0-6
(0 or 7 is Sunday, 1 is Monday, etc.)
Here we can see that cron job executed and created the backup of script in Practice folder.
Read About User Management:
A user is an entity in a Linux operating system that can manipulate files and perform several other operations. Each user is assigned an ID that is unique within the system. IDs 0 to 999 are assigned to system users, and local user IDs start from 1000 onwards.
Create 2 users and display their usernames.
#!/bin/bash
username1=$1
username2=$2
function User_management(){
sudo useradd ${username1}
sudo useradd ${username2}
echo "User ${username1} and ${username2} has been created"
echo "Congrats!!!!"
if [ $? -ne 0 ]; then
echo "User hasn't been created"
fi
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.