Mastering Spring Cloud: A Comprehensive Guide
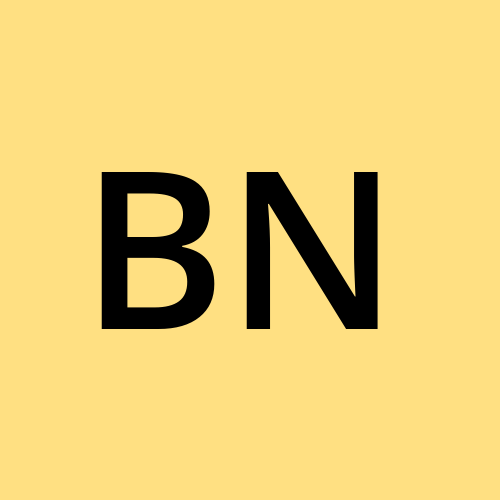
Table of contents
- Introduction to Spring Cloud
- 2. Spring Cloud Config
- Centralised Configuration Management
- Setting Up Spring Cloud Config Server
- Config Client Integration
- Managing Environment-Specific Configurations
- Refreshing Configurations at Runtime
- 3. Service Discovery with Spring Cloud Netflix Eureka
- 4. Spring Cloud Load Balancer
- 5. Spring Cloud Gateway
- 6. Spring Cloud Netflix Zuul (Legacy)
- 7. Spring Cloud Circuit Breaker
- 8. Spring Cloud Sleuth
- 9. Spring Cloud OpenFeign
- 10. Spring Cloud Stream
- 11. Spring Cloud Bus
- 12. Spring Cloud Security
- 13. Spring Cloud Kubernetes
- 14. Spring Cloud Consul/Zookeeper
- 15. Spring Cloud Contract
- 16. Spring Cloud Task
- 17. Spring Cloud Data Flow
- 18. Spring Cloud Deployer
- 19. Spring Cloud Functions
- 20. Spring Cloud Vault
- Overview
- Secure Storage of Secrets
- Integrating with Spring Cloud Config
- Managing Secrets and Credentials Dynamically
- 21. Spring Cloud GCP (Google Cloud Platform)
- 22. Spring Cloud Azure
- 23. Spring Cloud AWS
- 24. Spring Cloud for Microservices Communication
- 25. Distributed Locking with Spring Cloud
- 26. Spring Cloud Deployed on Multiple Cloud Providers
- 28. Spring Cloud Customisation
- 29. Advanced Topics
- 30. Best Practices and Challenges
- Conclusion :

Introduction to Spring Cloud
What is Spring Cloud?
Spring Cloud is a suite of tools for building distributed systems and microservices architectures. It builds on top of the Spring Framework, providing a set of libraries that simplify the development and deployment of cloud-native applications. These tools help developers address common challenges in distributed systems, such as configuration management, service discovery, load balancing, fault tolerance, and distributed tracing.
Spring Cloud integrates with various cloud providers and supports industry-standard technologies, making it easier to build, deploy, and manage microservices in a cloud environment. By abstracting away much of the complexity, Spring Cloud allows developers to focus on writing business logic rather than managing infrastructure.
Why Spring Cloud?
In a microservices architecture, applications are decomposed into smaller, independent services that communicate over a network. While this approach offers benefits such as scalability, flexibility, and resilience, it also introduces challenges related to service coordination, communication, and management.
Spring Cloud addresses these challenges by providing:
Centralised Configuration: Manage configuration for all microservices from a central location.
Service Discovery: Automatically locate services and route requests without hard-coding service addresses.
Load Balancing: Distribute incoming requests across multiple instances of a service.
Circuit Breakers: Gracefully handle failures in the system, preventing cascading failures.
API Gateway: Manage and route client requests to appropriate services.
Distributed Tracing: Monitor and trace requests as they flow through the system, helping in debugging and performance optimisation.
These tools are essential for building reliable, scalable, and maintainable microservices.
Key Components and Projects under Spring Cloud
Spring Cloud is organised into several projects, each focusing on a specific aspect of microservices architecture. Here’s a quick overview of the key components:
Spring Cloud Config: Provides centralized configuration management for distributed systems. It allows you to manage application configuration from a central repository, supporting dynamic updates to configuration properties without restarting services.
Spring Cloud Netflix: Integrates with Netflix’s open-source tools, including Eureka (service discovery), Ribbon (client-side load balancing), Hystrix (circuit breaker), and Zuul (API gateway). These tools are widely used in microservices architectures.
Spring Cloud Gateway: A modern, reactive API gateway that routes requests to appropriate services and handles cross-cutting concerns such as security, logging, and rate limiting.
Spring Cloud Sleuth: Provides distributed tracing, allowing you to track requests as they move through the system, making it easier to diagnose issues and understand system performance.
Spring Cloud Stream: Simplifies the development of event-driven microservices by providing a flexible and extensible messaging abstraction.
Spring Cloud Kubernetes: Provides seamless integration with Kubernetes, enabling Spring Cloud applications to be deployed and managed in a Kubernetes environment.
Spring Cloud Contract: Supports consumer-driven contract testing, ensuring that services can communicate with each other reliably.
2. Spring Cloud Config
Spring Cloud Config is a powerful tool in the Spring ecosystem that helps in managing configuration files centrally across multiple services. This ensures that all services can share configuration data, which can be dynamically updated without restarting the services.
Centralised Configuration Management
What it is: Centralised configuration management refers to storing all the configuration data (like properties or YAML files) for multiple applications in one central place, usually in a repository like Git. This allows different services to retrieve their configuration data from a single location.
Why it's important:
Consistency: All services use the same set of configurations, reducing errors due to configuration mismatches.
Ease of management: Configuration updates can be made in one place, rather than updating files across multiple services.
Version control: When using repositories like Git, configurations are versioned, which allows you to roll back to previous configurations if needed.
Setting Up Spring Cloud Config Server
What it is: The Spring Cloud Config Server is a central hub that manages and distributes configuration properties to client applications.
Steps to set it up:
Create a Spring Boot application: Add dependencies for
spring-cloud-config-server
.Enable the Config Server: Use the
@EnableConfigServer
annotation in your main application class.Configure the server: Point the Config Server to your configuration repository (e.g., a Git repository).
Run the server: Your server is now ready to serve configuration files to client applications.
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
Config Client Integration
What it is: Config clients are the services that need to fetch configuration properties from the Config Server.
Steps to integrate:
Add dependencies: Add
spring-cloud-starter-config
to your Spring Boot project.Configure bootstrap properties: In
bootstrap.yml
orbootstrap.properties
, specify the location of the Config Server.Access configurations: The client will automatically fetch configurations from the server at startup.
# bootstrap.yml
spring:
application:
name: my-service
cloud:
config:
uri: http://localhost:8888
Managing Environment-Specific Configurations
What it is: Sometimes, you have different configurations for different environments (e.g.,
development
,staging
,production
). The Spring Cloud Config server can manage these variations effectively.How to manage:
Naming convention: Create different files in your repository named according to environments like
application-dev.yml
,application-prod.yml
.Automatic selection: Spring Boot will automatically select the correct configuration based on the environment specified in the client’s
bootstrap.yml
.
Refreshing Configurations at Runtime
What it is: In a dynamic system, you might need to update configurations without restarting the application. Spring Cloud Config supports this.
How to enable:
Add
actuator
dependency: Includespring-boot-starter-actuator
in your client project.Enable refresh: Use
@RefreshScope
on beans that need to be refreshed at runtime.Trigger refresh: You can trigger a refresh by calling the
/actuator/refresh
endpoint.
@RefreshScope
@RestController
public class MyController {
@Value("${my.property}")
private String myProperty;
@GetMapping("/property")
public String getProperty() {
return myProperty;
}
}
3. Service Discovery with Spring Cloud Netflix Eureka
Service Discovery is a key aspect in microservices architecture, allowing services to discover each other without hard-coding their network locations.
Introduction to Service Discovery
What it is: Service discovery is the process of automatically detecting services within a network. Instead of hard-coding service addresses, services can dynamically find and communicate with each other.
Why it's important:
Scalability: Services can scale up or down, and the discovery mechanism will keep track of the instances.
Flexibility: Makes it easier to move or change services without updating clients manually.
Fault tolerance: If a service goes down, other services can be directed to the remaining healthy instances.
Setting Up Eureka Server
What it is: Eureka Server acts as a registry where all the services register themselves. It keeps track of all the instances of each service.
Steps to set it up:
Create a Spring Boot application: Add dependencies for
spring-cloud-starter-netflix-eureka-server
.Enable Eureka Server: Use the
@EnableEurekaServer
annotation in your main application class.Run the server: It will now be available for services to register with.
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Configuring Eureka Clients
What it is: Eureka clients are the services that register themselves with the Eureka Server and use it to discover other services.
Steps to configure:
Add dependencies: Include
spring-cloud-starter-netflix-eureka-client
in your project.Configure application properties: Specify the Eureka Server URL in
application.yml
.Enable Eureka client: Spring Boot will automatically register the service with Eureka.
# application.yml
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
Self-registration of Services
What it is: Services register themselves automatically with Eureka Server when they start up, providing their own network location (like IP address and port).
Why it's useful:
Dynamic Registration: Services can start up or shut down without manual intervention in the registry.
Automatic Deregistration: If a service instance fails or is shut down, it’s automatically removed from the registry.
Load Balancing with Ribbon and Eureka
What it is: Ribbon is a client-side load balancer that works with Eureka to distribute requests across multiple service instances.
How it works:
Integration: When a service (like a microservice) makes a request to another service, Ribbon will use Eureka to find available instances.
Load balancing: Ribbon balances the load by distributing the requests among all the available service instances.
@LoadBalanced
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
This integration allows seamless load balancing and service discovery, ensuring high availability and efficient use of resources.
4. Spring Cloud Load Balancer
Spring Cloud Load Balancer is a client-side load balancing library used in Spring Boot applications to distribute requests across multiple instances of a service. Unlike server-side load balancers, which handle the distribution of requests externally, client-side load balancers empower the client to choose which instance of a service to interact with.
Client-Side Load Balancing
What it is: Client-side load balancing is a method where the client application itself is responsible for distributing requests across multiple instances of a service. The client is aware of all the available service instances and selects one for each request, usually based on a predefined algorithm.
Why it's important:
Flexibility: The client can use various strategies to determine the best service instance to handle the request, improving efficiency.
Resilience: If one instance fails, the client can quickly redirect requests to another available instance, improving fault tolerance.
Scalability: As new instances of a service are added or removed, the client-side load balancer dynamically adapts, ensuring balanced traffic distribution.
Example: Imagine you have a service called
PaymentService
running on three different servers. When an order is placed, the client application (like a shopping cart service) needs to contactPaymentService
. Instead of always contacting the same server, the client-side load balancer will choose between the three instances ofPaymentService
, distributing the load evenly.
@LoadBalanced
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
In this example, @LoadBalanced
is applied to a RestTemplate
bean, enabling it to interact with different instances of a service through client-side load balancing.
Configuring Spring Cloud Load Balancer
What it is: Configuring Spring Cloud Load Balancer involves setting up the load-balancing behavior in your Spring Boot application, typically by defining how the client should select service instances.
How to configure:
Add Dependencies: Ensure that the
spring-cloud-starter-loadbalancer
dependency is included in your project.Enable Load Balancing: Annotate your
RestTemplate
orWebClient
bean with@LoadBalanced
to enable load balancing.Custom Load-Balancing Strategy: You can configure the load-balancing strategy by defining a
ServiceInstanceListSupplier
bean or configuring it inapplication.yml
.
@Bean
public ServiceInstanceListSupplier serviceInstanceListSupplier() {
return ServiceInstanceListSupplier.builder()
.withBlockingDiscoveryClient()
.withRoundRobin()
.build();
}
In this configuration, the load balancer uses a round-robin strategy to select instances.
Integration with Eureka and Other Discovery Clients
What it is: Spring Cloud Load Balancer can be integrated with service discovery clients like Eureka, Consul, or Zookeeper, allowing it to automatically discover service instances.
How it works:
Service Registration: Services register themselves with a discovery server like Eureka.
Instance Discovery: The load balancer queries the discovery server to get a list of available instances.
Request Distribution: The load balancer distributes incoming requests among these instances based on the configured strategy.
Example: If your application uses Eureka for service discovery, Spring Cloud Load Balancer will automatically query Eureka to discover the available instances of a service. When a request is made, it selects one of these instances according to the load-balancing algorithm.
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
spring:
cloud:
loadbalancer:
hint:
default: round-robin
This configuration ensures that the load balancer integrates seamlessly with Eureka, using a round-robin strategy to distribute requests.
5. Spring Cloud Gateway
Spring Cloud Gateway is a powerful API Gateway that provides a simple way to route requests, apply filters, and enforce security policies. It serves as a single entry point for all client requests to microservices, handling concerns such as routing, monitoring, security, and more.
API Gateway Patterns
What it is: An API Gateway is an intermediary that sits between clients and backend services, routing requests to the appropriate service while applying cross-cutting concerns like authentication, rate limiting, and logging.
Common patterns:
Routing: The Gateway routes incoming requests to the appropriate service based on the request path, method, or headers.
Aggregation: The Gateway can aggregate responses from multiple services and send a combined response to the client.
Authentication and Authorisation: The Gateway can enforce security policies by authenticating and authorising requests before forwarding them to backend services.
Example: Imagine a client requests user information. The API Gateway routes this request to the
UserService
. If the request also requires order information, the Gateway can aggregate data from bothUserService
andOrderService
and send a combined response.
Setting Up Spring Cloud Gateway
What it is: Setting up Spring Cloud Gateway involves creating a Spring Boot application that routes requests to backend services and applies filters.
Steps to set it up:
Add Dependencies: Include
spring-cloud-starter-gateway
in your project.Define Routes: Configure routes in
application.yml
or Java code to specify how requests should be routed.Run the Gateway: Once set up, the Gateway will start intercepting requests and applying the defined routing logic.
spring:
cloud:
gateway:
routes:
- id: user_route
uri: http://localhost:8081/users
predicates:
- Path=/users/**
In this example, any request matching /users/**
will be routed to http://localhost:8081/users
.
Routing and Filters
What it is: Routing is the process of forwarding client requests to appropriate backend services, while filters allow you to modify requests or responses in transit.
Types of filters:
Pre-filters: Execute before the request is routed to a service, e.g., adding headers, authentication checks.
Post-filters: Execute after the service has responded but before the response is sent back to the client, e.g., logging, modifying the response body.
Example: You can create a pre-filter to log all incoming requests:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("user_route", r -> r.path("/users/**")
.filters(f -> f.addRequestHeader("X-Custom-Header", "CustomValue"))
.uri("http://localhost:8081"))
.build();
}
This example adds a custom header to requests routed to UserService
.
Global Filters and Route-Specific Filters
What it is: Filters in Spring Cloud Gateway can be applied globally (to all routes) or to specific routes.
Global Filters:
Apply to all routes.
Useful for cross-cutting concerns like logging or authentication.
Route-Specific Filters:
Apply only to specific routes.
Useful for route-specific logic like modifying headers or rate limiting.
Example: A global filter that logs every request:
@Bean
public GlobalFilter customGlobalFilter() {
return (exchange, chain) -> {
System.out.println("Global Pre-Filter executed");
return chain.filter(exchange).then(Mono.fromRunnable(() -> {
System.out.println("Global Post-Filter executed");
}));
};
}
Circuit Breaker Integration
What it is: A Circuit Breaker pattern helps to prevent system failures by stopping requests to a failing service, and instead returning a fallback response.
How it works:
If a service fails too often, the Circuit Breaker trips, and requests are no longer forwarded to that service.
The Circuit Breaker can automatically recover after a certain time if the service becomes healthy again.
Example: Integrating Circuit Breaker with a route:
spring:
cloud:
gateway:
routes:
- id: order_route
uri: http://localhost:8082/orders
filters:
- name: CircuitBreaker
args:
name: myCircuitBreaker
fallbackUri: forward:/fallback
In this example, if OrderService
fails, the Gateway will forward the request to a fallback route.
Implementing Rate Limiting in Spring Cloud Gateway
What it is: Rate limiting controls the number of requests a client can make to a service in a given time period, helping to prevent abuse and ensure fair use of resources.
Why it's important:
Security: Protects services from being overwhelmed by too many requests.
Fair Usage: Ensures that no single client consumes all the resources.
Example: Configuring rate limiting in
application.yml
:
spring:
cloud:
gateway:
routes:
- id: rate_limit_route
uri: http://localhost:8083
filters:
- name: RequestRateLimiter
args:
redis-rate-limiter:
replenishRate: 10
burstCapacity: 20
In this configuration, the RequestRateLimiter
filter allows 10 requests per second with a burst capacity of 20.
Configuring Request Throttling and Burst Control
What it is: Request throttling limits the number of requests processed by a service, while burst control handles sudden spikes in traffic by allowing short bursts above the normal rate limit.
How to configure:
Replenish Rate: The steady number of requests allowed per second.
Burst Capacity: The maximum number of requests allowed in a burst.
Example: If your
OrderService
should handle a maximum of 5 requests per second but can tolerate a burst of up to 10 requests, you would configure it like this:
replenishRate: 5
burstCapacity: 10
This means OrderService
can handle up to 10 requests in a burst but will throttle to 5 requests per second afterward.
Integration with Redis for Distributed Rate Limiting
What it is: Redis can be used to manage rate limiting in a distributed environment, ensuring that rate limits are enforced consistently across multiple instances of the Gateway.
How it works:
Redis stores rate limits: When a request is made, the Gateway checks Redis to determine if the rate limit has been exceeded.
Consistency across instances: Redis ensures that all Gateway instances share the same rate limit state, preventing bypassing limits by switching instances.
Example: To integrate Redis with Spring Cloud Gateway for rate limiting:
spring:
redis:
host: localhost
port: 6379
spring:
cloud:
gateway:
routes:
- id: redis_rate_limit_route
uri: http://localhost:8084
filters:
- name: RequestRateLimiter
args:
redis-rate-limiter:
replenishRate: 5
burstCapacity: 10
In this setup, Redis manages the rate limits, ensuring that the configured limits apply consistently across all instances of your API Gateway.
6. Spring Cloud Netflix Zuul (Legacy)
Spring Cloud Netflix Zuul is a legacy gateway solution used for routing requests to different backend services in a microservices architecture. It provides features like dynamic routing, monitoring, resiliency, security, and more. Zuul is often used to implement API Gateway patterns but has been largely replaced by Spring Cloud Gateway in modern Spring Cloud projects.
Overview of Zuul as a Gateway
What it is: Zuul is a reverse proxy and gateway that handles all requests coming from clients and routes them to appropriate backend services. It also allows for additional functionalities like filtering, security checks, and load balancing before forwarding requests.
Why it's important:
Single Entry Point: Zuul acts as a single entry point for all client requests, centralising the routing logic and reducing complexity in client-side code.
Dynamic Routing: Zuul can dynamically route requests to different services based on request parameters, paths, or headers.
Filters: Zuul supports pre-filters and post-filters, allowing you to modify requests before they reach the backend or responses before they are returned to the client.
Example: If you have multiple microservices like
UserService
,OrderService
, andPaymentService
, Zuul can route/users/**
requests toUserService
,/orders/**
requests toOrderService
, and so on.
zuul:
routes:
user-service:
path: /users/**
url: http://localhost:8081
order-service:
path: /orders/**
url: http://localhost:8082
In this configuration, Zuul will route requests to the appropriate backend service based on the URL path.
Basic Routing and Filtering
Routing:
What it is: Zuul's primary function is to route incoming requests to the correct backend service based on the request URL.
How to configure: You define routes in the
application.yml
file, specifying the path to match and the URL of the service to route to.Example: Routing requests based on paths:
zuul: routes: payment-service: path: /payments/** serviceId: payment-service
Here, any request with the path
/payments/**
is routed to thepayment-service
.
Filtering:
What it is: Zuul allows you to define filters that can intercept requests at different points in the request lifecycle (pre-routing, post-routing, etc.).
Types of Filters:
Pre-filters: Execute before routing, useful for authentication or logging.
Route-filters: Handle the actual routing logic.
Post-filters: Execute after routing, useful for modifying responses.
Error-filters: Handle errors during the request lifecycle.
Example: A pre-filter that logs the request method and URL:
@Component public class PreRequestFilter extends ZuulFilter { @Override public String filterType() { return "pre"; } @Override public int filterOrder() { return 1; } @Override public boolean shouldFilter() { return true; } @Override public Object run() { RequestContext ctx = RequestContext.getCurrentContext(); HttpServletRequest request = ctx.getRequest(); System.out.println("Request Method : " + request.getMethod() + " Request URL : " + request.getRequestURL().toString()); return null; } }
This filter logs every incoming request’s method and URL before routing.
Comparison with Spring Cloud Gateway
Zuul vs. Spring Cloud Gateway:
Performance:
Zuul: Zuul 1.x is based on blocking I/O, which can be less efficient for handling a large number of concurrent requests.
Spring Cloud Gateway: Built on top of Spring WebFlux, it uses non-blocking I/O, making it more suitable for modern reactive applications.
Features:
Zuul: Offers basic routing and filtering, but with limited flexibility and scalability.
Spring Cloud Gateway: Provides advanced routing, filtering, rate limiting, Circuit Breaker integration, and more, with better extensibility and support for reactive programming.
Ease of Use:
Zuul: Requires more manual configuration and coding for complex use cases.
Spring Cloud Gateway: Easier to configure with properties or Java code, and more powerful in handling complex routing and filtering scenarios.
Migration Consideration:
- Projects using Zuul should consider migrating to Spring Cloud Gateway to take advantage of better performance, enhanced features, and ongoing support.
7. Spring Cloud Circuit Breaker
Spring Cloud Circuit Breaker is a library that provides Circuit Breaker functionality for microservices, helping them to handle failures gracefully and improve overall resilience. It integrates with popular Circuit Breaker libraries like Resilience4j, Hystrix (deprecated), and Sentinel.
Introduction to Resilience and Fault Tolerance
What it is: Resilience in microservices refers to the system’s ability to handle failures without compromising the overall application. Fault tolerance ensures that the system continues to operate, possibly at a reduced level, even when part of the system fails.
Why it's important:
Preventing cascading failures: When one service fails, it can cause a chain reaction, affecting other services. Circuit Breakers help isolate failures and prevent them from spreading.
Improving user experience: Instead of completely failing, a service can return a default response or a meaningful error message, improving the user experience.
Circuit Breaker Pattern:
Closed State: The service operates normally.
Open State: The Circuit Breaker "opens" after a certain number of failures, stopping requests to the failing service.
Half-Open State: After a cooldown period, the Circuit Breaker allows a few test requests to check if the service has recovered. If successful, it returns to the closed state.
Setting Up Circuit Breaker with Resilience4j
What it is: Resilience4j is a lightweight, easy-to-use library that provides various resilience patterns, including Circuit Breaker, Retry, Rate Limiter, and Bulkhead.
Steps to set it up:
Add Dependencies: Include
spring-cloud-starter-circuitbreaker-resilience4j
in your project.Configure Circuit Breaker: Define Circuit Breaker settings in
application.yml
or Java code.Apply Circuit Breaker: Annotate your service methods with
@CircuitBreaker
to apply the Circuit Breaker pattern.
Example: Applying Circuit Breaker to a service method:
@Service public class PaymentService { @CircuitBreaker(name = "paymentService", fallbackMethod = "fallback") public String processPayment() { // Logic that might fail } public String fallback(Exception e) { return "Payment service is currently unavailable. Please try again later."; } }
In this example, if
processPayment()
fails repeatedly, the Circuit Breaker will open, and the fallback method will be executed.
Fallback Mechanisms
What it is: Fallback mechanisms provide an alternative response or action when the main service fails. This ensures that the user receives a response, even if it’s not the ideal one.
Types of fallbacks:
Static Fallback: Return a predefined static response.
Alternative Service: Redirect the request to another service or a cache.
Graceful Degradation: Offer a reduced level of service or a simplified response.
Example: A fallback method that returns a default message:
public String fallback(Exception e) { return "Service is temporarily unavailable. Please try again later."; }
This fallback method will be triggered if the main service fails, ensuring that the user still receives a response.
Bulkhead and Rate Limiter Patterns
Bulkhead Pattern:
What it is: The Bulkhead pattern isolates different parts of a system to prevent a failure in one part from affecting the whole system. It limits the number of concurrent calls to a particular service or component.
Why it's important: Prevents a flood of requests to one service from overwhelming the entire system, improving stability and resilience.
Example: Configuring a Bulkhead using Resilience4j:
resilience4j.bulkhead: instances: serviceBulkhead: maxConcurrentCalls: 10 maxWaitDuration: 100ms
This configuration limits the number of concurrent calls to
10
, with a maximum wait time of100ms
.
Rate Limiter Pattern:
What it is: The Rate Limiter pattern controls the rate at which requests are allowed to a service, protecting it from being overwhelmed by too many requests.
Why it's important: Helps manage traffic to critical services, ensuring they remain responsive even under heavy load.
Example: Configuring a Rate Limiter using Resilience4j:
resilience4j.ratelimiter: instances: serviceRateLimiter: limitForPeriod: 5 limitRefreshPeriod: 1s timeoutDuration: 500ms
This configuration allows up to
5
requests per second, with a timeout of500ms
.
8. Spring Cloud Sleuth
Spring Cloud Sleuth is a distributed tracing solution designed for microservices architecture. It helps trace the flow of requests as they traverse across multiple microservices, providing visibility into the system's performance and behavior. By integrating with tools like Zipkin and Jaeger, Sleuth enables detailed monitoring and troubleshooting.
Distributed Tracing with Spring Cloud Sleuth
Trace and Span Concepts
Trace: A trace represents the entire journey of a request across different microservices. Every trace has a unique
trace ID
that remains consistent as the request moves from one service to another. This ID is critical for tracking the request's path and understanding its behavior throughout the system.Span: A span is a single unit of work within a trace. Each span has its own
span ID
, and spans can be nested within each other to represent sub-operations within a trace. For instance, a trace could include spans for database access, external API calls, and internal processing within different microservices.Baggage: Baggage consists of key-value pairs that are passed along with the trace. These pairs contain contextual information, such as user ID or transaction ID, which can be used by different services involved in the trace. Baggage ensures that important metadata travels with the request across service boundaries.
Correlating Logs Across Microservices
One of Sleuth's powerful features is its ability to correlate logs from different microservices by adding trace and span IDs to log entries. This makes it easier to troubleshoot issues by following a request's journey through the system.
Example Log Correlation
When Sleuth is integrated with a logging framework like SLF4J, it automatically appends trace and span IDs to each log entry:
2024-08-16 10:15:30.123 INFO [orderservice,2cfa4bcdd3ae47b6,17d4e1231ab1a1f5,1] --- [nio-8080-exec-1] c.e.OrderService : Processing order
orderservice
: The service where the log was generated.2cfa4bcdd3ae47b6
: Thetrace ID
for the request.17d4e1231ab1a1f5
: Thespan ID
for the current operation.1
: The export status, indicating whether the trace data was exported to a tracing system.
With this setup, you can search logs by trace ID to see all related log entries across different services, making it easier to diagnose issues.
Integration with Zipkin or Jaeger
Spring Cloud Sleuth can be integrated with distributed tracing systems like Zipkin and Jaeger, which collect and visualize traces.
Setting Up Zipkin
Add Zipkin Dependency: To integrate Zipkin with Sleuth, include the Zipkin starter in your
pom.xml
:<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-zipkin</artifactId> </dependency>
Configure Zipkin Base URL: Configure the base URL for your Zipkin server in
application.yml
:spring: zipkin: base-url: http://localhost:9411 sleuth: sampler: probability: 1.0
Setting
probability: 1.0
ensures that all requests are sampled and sent to Zipkin. Adjust this value to control the sampling rate.Accessing Zipkin UI: You can access the Zipkin UI at
http://localhost:9411
to view and analyze traces. The UI allows you to filter traces by service name, trace ID, and time range.
Setting Up Jaeger
Add Jaeger Dependency: Similar to Zipkin, you can integrate Jaeger by adding the Zipkin starter dependency.
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-zipkin</artifactId> </dependency>
Configure Jaeger Endpoint: Set the Jaeger endpoint in
application.yml
:spring: sleuth: sampler: probability: 1.0 zipkin: base-url: http://localhost:14268/api/traces
Accessing Jaeger UI: The Jaeger UI is typically accessible at
http://localhost:16686
, where you can search and visualize traces similar to Zipkin.
Customizing Trace and Span Information
Creating Custom Spans
In some cases, you might want to create custom spans to capture specific operations within your application. This can be done using the Tracer
API provided by Sleuth.
Example:
@Autowired
private Tracer tracer;
public void processOrder() {
Span newSpan = tracer.nextSpan().name("processOrder").start();
try (SpanInScope ws = tracer.withSpan(newSpan.start())) {
// Execute business logic
} finally {
newSpan.end();
}
}
Tracer: A Spring-managed bean that allows you to create and manage spans.
SpanInScope: Ensures that the span is active for the duration of the business logic.
Custom Samplers
Sleuth uses samplers to determine which requests should be traced. By default, it traces all requests, but you can customize this behavior.
Example:
@Bean
public Sampler customSampler() {
return Sampler.create(0.5); // 50% of requests will be sampled
}
This configuration samples only 50% of the requests, reducing the amount of trace data collected.
Baggage Propagation
Baggage allows you to pass additional metadata along with the trace, providing more context for the request.
Example:
BaggageField userIdField = BaggageField.create("user-id");
userIdField.updateValue("12345");
System.out.println(userIdField.getValue()); // Outputs "12345"
This example demonstrates how to create a baggage field, set its value, and retrieve it later in the trace.
9. Spring Cloud OpenFeign
Spring Cloud OpenFeign is a declarative HTTP client that simplifies the process of making RESTful calls between microservices. By abstracting away the complexities of HTTP communication, OpenFeign allows you to define service interfaces and automatically generate the underlying client code.
Declarative REST Client with OpenFeign
Feign Clients
Feign clients are interfaces annotated with Feign-specific annotations. Spring automatically generates implementations of these interfaces, which handle the HTTP communication.
Example:
@FeignClient(name = "user-service")
public interface UserClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") Long id);
}
@FeignClient(name = "user-service")
: Specifies the name of the service to communicate with. Spring will look up this service in the service registry (e.g., Eureka) and create a client.@GetMapping("/users/{id}")
: Defines the endpoint and HTTP method for the request.
This interface defines a client that communicates with the user-service
to fetch user data based on the user ID.
Integrating with Eureka for Service Discovery
OpenFeign can integrate with service discovery tools like Eureka to dynamically resolve service URLs, making it easier to manage microservices in a distributed environment.
Example Eureka Configuration:
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
With this setup, Feign uses the service name (user-service
) to discover the actual URL of the service via Eureka, eliminating the need to hardcode service URLs.
Circuit Breaker and Retry Support
Circuit Breaker Integration
Circuit breakers are a crucial part of a resilient microservices architecture. They help protect services from cascading failures by stopping requests to a service that is down or performing poorly.
Example with Resilience4j:
Add Resilience4j Dependency:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-circuitbreaker-resilience4j</artifactId> </dependency>
Configure Circuit Breaker:
resilience4j: circuitbreaker: configs: default: slidingWindowSize: 100 failureRateThreshold: 50
slidingWindowSize
: The number of requests to consider for determining if the circuit breaker should open.failureRateThreshold
: The percentage of failed requests that will trigger the circuit breaker.
Feign Client with Fallback:
@FeignClient(name = "order-service", fallback = OrderClientFallback.class) public interface OrderClient { @GetMapping("/orders/{id}") Order getOrderById(@PathVariable("id") Long id); } @Component public class OrderClientFallback implements OrderClient { @Override public Order getOrderById(Long id) { return new Order(); // Return a default or fallback response } }
In this example, if the order-service
fails, the circuit breaker will trigger, and the OrderClientFallback
class will handle the request, returning a default response.
Retry Mechanism
Feign also supports automatic retries in case of transient failures, improving the resilience of your application.
Example with Spring Retry:
Add Spring Retry Dependency:
<dependency> <groupId>org.springframework.retry</groupId> <artifactId>spring-retry</artifactId> </dependency>
Enable Retry on Feign Client:
feign: client: config: default: retryer: maxAttempts: 3 backoff: 2000 # 2 seconds
maxAttempts
: Number of retry attempts.backoff
: Time delay between retry attempts.
Customizing Feign Clients
Logging Requests and Responses
Feign provides logging capabilities to monitor HTTP requests and responses, which is helpful for debugging.
Example:
@Bean
public Logger.Level feignLoggerLevel() {
return Logger.Level.FULL; // Logs headers, body, and metadata
}
NONE: No logging.
BASIC: Logs only the request method and URL, response status code, and execution time.
HEADERS: Logs the basic information along with request and response headers.
FULL: Logs everything, including headers, request, and response bodies.
Request Interceptors
Request interceptors allow you to modify requests before they are sent. For example, you can add authentication tokens or custom headers.
Example:
@Bean
public RequestInterceptor requestInterceptor() {
return requestTemplate -> {
requestTemplate.header("Authorization", "Bearer " + tokenService.getToken());
};
}
Here, an authorization token is added to every request sent by the Feign client.
Custom Error Handling
Feign allows you to handle HTTP errors using a custom ErrorDecoder
. This is useful for translating HTTP errors into application-specific exceptions.
Example:
@Bean
public ErrorDecoder errorDecoder() {
return new CustomErrorDecoder();
}
public class CustomErrorDecoder implements ErrorDecoder {
@Override
public Exception decode(String methodKey, Response response) {
if (response.status() == 404) {
return new NotFoundException("Resource not found");
}
return new Default().decode(methodKey, response);
}
}
In this example, a 404 error is translated into a NotFoundException
.
10. Spring Cloud Stream
Spring Cloud Stream is a framework for building event-driven microservices, which allows you to connect microservices with messaging systems like Kafka or RabbitMQ. It abstracts the complexities of message-driven microservices, providing a unified and easy-to-use API for message producers and consumers.
Event-Driven Microservices
Event-driven microservices rely on events to communicate between different parts of a system. Instead of making direct synchronous calls, services publish events to a messaging system, and other services consume these events to perform actions or trigger workflows.
Setting up Spring Cloud Stream with Messaging Systems
Adding Dependencies
To get started with Spring Cloud Stream, you need to include the necessary dependencies in your project. Depending on the messaging system you want to use, you'll include the corresponding binder:
For Kafka:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-stream-binder-kafka</artifactId> </dependency>
For RabbitMQ:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-stream-binder-rabbit</artifactId> </dependency>
Configuring the Binder
The binder is the component that connects Spring Cloud Stream to the underlying messaging system. Configuration is typically done in application.yml
or application.properties
.
Example for Kafka:
spring:
cloud:
stream:
bindings:
output:
destination: my-topic
kafka:
binder:
brokers: localhost:9092
Example for RabbitMQ:
spring:
cloud:
stream:
bindings:
output:
destination: my-queue
rabbit:
binder:
hosts: localhost
In these configurations:
bindings
: Defines the channels for input/output communication.destination
: The topic (Kafka) or queue (RabbitMQ) to which the service will send or from which it will receive messages.
Defining and Consuming Messages
Defining Message Channels
Spring Cloud Stream uses the concept of channels to abstract message producers and consumers. Channels are defined using the @Input
and @Output
annotations.
Example:
public interface MyProcessor {
@Input("inputChannel")
SubscribableChannel input();
@Output("outputChannel")
MessageChannel output();
}
@Input("inputChannel")
: Declares an input channel for consuming messages.@Output("outputChannel")
: Declares an output channel for producing messages.
Producing Messages
Messages can be sent to a channel using the MessageChannel
interface.
Example:
@Autowired
private MyProcessor myProcessor;
public void sendMessage(String payload) {
myProcessor.output().send(MessageBuilder.withPayload(payload).build());
}
In this example, a message with the given payload is sent to the outputChannel
.
Consuming Messages
To consume messages, you can use the @StreamListener
annotation.
Example:
@StreamListener("inputChannel")
public void handleMessages(String payload) {
System.out.println("Received: " + payload);
}
This method will be triggered whenever a message is received on the inputChannel
.
Error Handling and Retries
Spring Cloud Stream provides robust error handling mechanisms, including retries, dead-letter queues, and custom error channels.
Retrying Failed Messages
Retries can be configured directly in the binder configuration.
Example:
spring:
cloud:
stream:
bindings:
inputChannel:
consumer:
maxAttempts: 5
backOffInitialInterval: 1000 # 1 second
backOffMaxInterval: 10000 # 10 seconds
In this configuration:
maxAttempts
: Specifies the maximum number of retry attempts.backOffInitialInterval
: The time to wait before the first retry.backOffMaxInterval
: The maximum time to wait between retries.
Using a Dead-Letter Queue
Messages that fail to process after all retry attempts can be sent to a dead-letter queue for further investigation.
Example:
spring:
cloud:
stream:
bindings:
inputChannel:
consumer:
dlqName: my-dead-letter-queue
republishToDlq: true
Here, failed messages are redirected to my-dead-letter-queue
.
Custom Error Channels
You can also define custom error channels to handle failed messages.
Example:
@StreamListener("errorChannel")
public void handleError(Message<?> message) {
System.out.println("Error occurred: " + message);
}
This method handles messages that could not be processed successfully.
Stream Processing with Functional Programming
Spring Cloud Stream also supports the functional programming model, allowing you to define message producers and consumers as Supplier
, Function
, and Consumer
beans.
Example:
@Bean
public Supplier<String> sendMessage() {
return () -> "Hello, World!";
}
@Bean
public Consumer<String> processMessage() {
return message -> System.out.println("Processed: " + message);
}
@Bean
public Function<String, String> transformMessage() {
return message -> message.toUpperCase();
}
Supplier
: Produces messages.Consumer
: Consumes messages.Function
: Transforms messages.
With these beans, Spring Cloud Stream automatically connects them to the appropriate channels based on configuration.
11. Spring Cloud Bus
Overview
Spring Cloud Bus is a framework that allows for the propagation of state changes across multiple instances of a distributed system. It's commonly used to broadcast configuration changes or events across a microservices architecture using a message broker like Kafka or RabbitMQ.
Propagating Configuration Changes Across Microservices
In a microservices architecture, it's often necessary to update configuration properties across multiple services simultaneously. Spring Cloud Bus simplifies this by propagating configuration changes from a central source (like Spring Cloud Config) to all registered microservices.
Integrating Spring Cloud Bus with Spring Cloud Config
Setting Up Spring Cloud Config
To use Spring Cloud Bus for propagating configuration changes, you first need to set up Spring Cloud Config.
Example configuration for the Config Server (application.yml
):
server:
port: 8888
spring:
cloud:
config:
server:
git:
uri: https://github.com/your-repo/config-repo
port: 8888
: The Config Server runs on port 8888.git.uri
: Points to the Git repository containing your configuration files.
Adding Spring Cloud Bus to Your Project
Include the necessary dependencies for Spring Cloud Bus and your chosen messaging system.
For RabbitMQ:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-amqp</artifactId>
</dependency>
For Kafka:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-kafka</artifactId>
</dependency>
Configuring Spring Cloud Bus
Configure Spring Cloud Bus to use your chosen messaging system.
Example for RabbitMQ:
spring:
cloud:
bus:
enabled: true
stream:
bindings:
springCloudBusOutput:
destination: springCloudBusExchange
rabbit:
binder:
environment:
spring:
rabbitmq:
host: localhost
port: 5672
This configuration sets up Spring Cloud Bus to use RabbitMQ with the exchange springCloudBusExchange
.
Broadcasting Events Using Messaging Systems
Spring Cloud Bus can broadcast various events across your microservices, such as configuration changes or custom application events.
Broadcasting Configuration Changes
When a configuration change occurs, you can trigger the propagation of the change using an HTTP request to the /actuator/bus-refresh
endpoint.
Example:
curl -X POST http://localhost:8080/actuator/bus-refresh
This command triggers the bus to broadcast the configuration change across all services that are connected to the Config Server.
Custom Events
You can also create and broadcast custom events using Spring Cloud Bus.
Example:
public class MyCustomEvent extends RemoteApplicationEvent {
public MyCustomEvent(Object source, String originService, String destinationService) {
super(source, originService, destinationService);
}
}
@Component
public class MyEventPublisher {
@Autowired
private ApplicationEventPublisher eventPublisher;
public void publishEvent() {
eventPublisher.publishEvent(new MyCustomEvent(this, "origin-service", "destination-service"));
}
}
MyCustomEvent: A custom event extending
RemoteApplicationEvent
.MyEventPublisher: Publishes the custom event, which is then propagated across the system.
Listening to Events
You can listen to these events using the @EventListener
annotation.
Example:
@EventListener
public void handleCustomEvent(MyCustomEvent event) {
System.out.println("Received custom event: " + event);
}
This method handles custom events broadcast by Spring Cloud Bus.
12. Spring Cloud Security
Spring Cloud Security provides robust security features for microservices architectures, integrating seamlessly with OAuth2 and JWT for authentication and authorisation. It also offers tools to secure API gateways like Spring Cloud Gateway and implements role-based access control to manage user permissions across services.
Securing Microservices with OAuth2
OAuth2 Overview
OAuth2 is a widely used authorisation framework that enables third-party applications to access a user’s resources without exposing their credentials. It is based on access tokens that are issued to clients, allowing them to authenticate and interact with the server.
Implementing OAuth2 in Spring Cloud
To secure microservices with OAuth2, you can leverage Spring Security OAuth2 and Spring Boot's auto-configuration features.
1. Configuring the Authorisation Server
The Authorisation Server issues tokens that clients use to authenticate with resource servers.
Example setup:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-authserver</artifactId>
</dependency>
@EnableAuthorizationServer
public class AuthServerConfig extends AuthorizationServerConfigurerAdapter {
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("client-id")
.secret("{noop}client-secret")
.authorizedGrantTypes("password", "refresh_token")
.scopes("read", "write")
.accessTokenValiditySeconds(3600);
}
}
withClient("client-id")
: Defines the client identifier.secret("client-secret")
: Sets the client secret.authorizedGrantTypes("password", "refresh_token")
: Specifies the grant types the client can use.scopes("read", "write")
: Defines the scopes for the client.
2. Configuring the Resource Server
The Resource Server validates the access tokens and grants access to protected resources.
Example setup:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-resource-server</artifactId>
</dependency>
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.antMatchers("/private/**").authenticated();
}
}
antMatchers("/public/**").permitAll()
: Allows unrestricted access to public endpoints.antMatchers("/private/**").authenticated()
: Secures private endpoints, requiring valid tokens.
JWT Authentication and Authorization
What is JWT?
JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. It is commonly used for securely transmitting information between clients and servers as a JSON object.
Using JWT with OAuth2
JWTs can be used as access tokens in an OAuth2 setup. They are signed to ensure data integrity and can include user claims such as roles and permissions.
1. Adding JWT Dependency
To use JWT with Spring Security, include the following dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-resource-server</artifactId>
</dependency>
2. Configuring JWT
Configure the Resource Server to use JWT tokens:
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.tokenStore(tokenStore());
}
@Bean
public TokenStore tokenStore() {
return new JwtTokenStore(accessTokenConverter());
}
@Bean
public JwtAccessTokenConverter accessTokenConverter() {
JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
converter.setSigningKey("my-secret-key");
return converter;
}
}
JwtTokenStore
: Stores the tokens.JwtAccessTokenConverter
: Converts the JWT into OAuth2 authentication information.
Customizing JWT Claims
You can customise JWT claims to include additional user details, such as roles or permissions.
Example:
public class CustomTokenEnhancer implements TokenEnhancer {
@Override
public OAuth2AccessToken enhance(OAuth2AccessToken accessToken, OAuth2Authentication authentication) {
Map<String, Object> additionalInfo = new HashMap<>();
additionalInfo.put("organization", authentication.getName() + "-organization");
((DefaultOAuth2AccessToken) accessToken).setAdditionalInformation(additionalInfo);
return accessToken;
}
}
Securing Spring Cloud Gateway
Overview
Spring Cloud Gateway can be secured by integrating it with OAuth2 and JWT, ensuring that all incoming requests are authenticated before being routed to the backend services.
Setting Up Security
Add security to Spring Cloud Gateway by configuring it to act as a resource server.
Example configuration:
spring:
security:
oauth2:
resourceserver:
jwt:
issuer-uri: http://auth-server
issuer-uri
: Points to the OAuth2 Authorization Server.
Applying Security Filters
You can apply security filters to specific routes, ensuring that only authorized requests are processed.
Example:
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
http
.authorizeExchange()
.pathMatchers("/public/**").permitAll()
.pathMatchers("/private/**").authenticated()
.and().oauth2ResourceServer().jwt();
return http.build();
}
authorizeExchange()
: Configures access control for different paths..jwt()
: Specifies that JWT tokens will be used for authentication.
Role-Based Access Control (RBAC)
Overview
Role-Based Access Control (RBAC) is a security mechanism that restricts access to resources based on user roles. Each role has specific permissions, and users are assigned roles according to their responsibilities.
Implementing RBAC
You can implement RBAC in Spring Security by assigning roles to users and securing endpoints based on these roles.
Example configuration:
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated();
.hasRole("ADMIN")
: Restricts access to users with the "ADMIN" role..hasRole("USER")
: Restricts access to users with the "USER" role.
Customizing Access Decisions
Spring Security allows you to customize access decisions using expressions.
Example:
http.authorizeRequests()
.antMatchers("/special/**").access("hasRole('ADMIN') and hasIpAddress('192.168.1.0/24')")
.anyRequest().authenticated();
.access("expression")
: Customizes access using SpEL (Spring Expression Language).
13. Spring Cloud Kubernetes
Overview
Spring Cloud Kubernetes integrates Spring Boot applications with Kubernetes, enabling features like service discovery, configuration management, and seamless deployment of Spring Cloud applications in Kubernetes environments. It leverages Kubernetes-native concepts to enhance the scalability and manageability of microservices.
Deploying Spring Cloud Applications in Kubernetes
Containerizing the Application
Before deploying a Spring Cloud application in Kubernetes, you need to containerize it using Docker.
Example Dockerfile:
FROM openjdk:17-jdk-alpine
VOLUME /tmp
ADD target/myapp.jar myapp.jar
ENTRYPOINT ["java","-jar","/myapp.jar"]
FROM openjdk:17-jdk-alpine
: Specifies the base image.ADD target/myapp.jar myapp.jar
: Adds the built JAR file to the container.ENTRYPOINT
: Defines the command to run the application.
Creating Kubernetes Resources
Kubernetes uses resources like Deployment
, Service
, and ConfigMap
to manage applications.
Example Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp-container
image: myapp:latest
ports:
- containerPort: 8080
replicas: 3
: Specifies the number of instances.containers
: Defines the container, including the image and ports.
Example Service:
apiVersion: v1
kind: Service
metadata:
name: myapp-service
spec:
selector:
app: myapp
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: LoadBalancer
type: LoadBalancer
: Exposes the service externally using a load balancer.
Service Discovery and Configuration Management in Kubernetes
Service Discovery
In Kubernetes, service discovery is handled by the DNS service, which allows services to discover each other by name.
Example:
spring:
cloud:
kubernetes:
discovery:
enabled: true
enabled: true
: Enables Kubernetes service discovery.
Spring Cloud applications can now automatically discover other services registered in Kubernetes.
Configuration Management
Kubernetes ConfigMaps and Secrets are used to manage configuration data and sensitive information, respectively.
Example ConfigMap:
apiVersion: v1
kind: ConfigMap
metadata:
name: myapp-config
data:
application.properties: |
spring.datasource.url=jdbc:mysql://db-service/mydb
spring.datasource.username=user
spring.datasource.password=pass
data
: Contains key-value pairs of configuration data.
In the Spring Boot application, you can access this configuration data:
spring:
config:
import: "configserver:kubernetes"
configserver:kubernetes
: Imports configuration data from Kubernetes ConfigMaps.
Kubernetes-Native Features in Spring Cloud
Load Balancing with Kubernetes
Spring Cloud Kubernetes supports load balancing by utilising Kubernetes' native service discovery. Each service in Kubernetes has a stable IP address and DNS name, allowing Spring Cloud to route requests across multiple instances.
Example setup:
spring:
cloud:
loadbalancer:
enabled: true
enabled: true
: Enables load balancing.
Requests to a service will be distributed across its available instances automatically.
Pod Autoscaling
Kubernetes provides Horizontal Pod Autoscaler (HPA) to automatically scale the number of pod replicas based on observed CPU utilisation or other custom metrics.
Example HPA configuration:
apiVersion: autoscaling/v1
kind: HorizontalPodAutoscaler
metadata:
name: myapp-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: myapp
minReplicas: 1
maxReplicas: 10
targetCPUUtilizationPercentage: 50
minReplicas
: Minimum number of replicas.maxReplicas
: Maximum number of replicas.targetCPUUtilisationPercentage
: Target CPU utilisation.
External Configuration with ConfigMaps
Spring Cloud Kubernetes integrates with Kubernetes ConfigMaps to manage externalised configuration. It allows you to change configurations without rebuilding or redeploying applications.
Example configuration:
spring:
cloud:
kubernetes:
config:
sources:
- name: myapp-config
sources
: Specifies the ConfigMap to use.
Changes to the ConfigMap are automatically detected and applied to the running application.
14. Spring Cloud Consul/Zookeeper
Spring Cloud Consul and Spring Cloud Zookeeper are tools used for service discovery and configuration management in microservices architectures. Both offer robust mechanisms for service registration, discovery, and centralized configuration management. While they serve similar purposes, they differ in their underlying architecture and implementation.
Service Discovery and Configuration with Consul/Zookeeper
Consul
Consul is a tool for service discovery, configuration, and health monitoring. It provides features like a key-value store for configuration, a distributed service registry, and health checks for services.
Key Features:
Service Discovery: Allows services to register themselves and discover other services via DNS or HTTP.
Health Checks: Monitors the health of services and removes unhealthy ones from the registry.
Key-Value Store: Stores configuration data that can be accessed by any service.
Service Registration Example:
spring:
cloud:
consul:
discovery:
service-name: my-service
health-check-path: /health
health-check-interval: 10s
service-name
: Registers the service with Consul under this name.health-check-path
: Defines the endpoint for health checks.health-check-interval
: Sets the interval for health checks.
Configuration Management Example:
spring:
cloud:
consul:
config:
enabled: true
data-key: config/application
data-key
: Points to the key in Consul's key-value store that contains configuration data.
Zookeeper
Zookeeper is a distributed coordination service that provides mechanisms for service discovery and configuration management. It is commonly used for managing distributed applications and is known for its strong consistency guarantees.
Key Features:
Service Discovery: Allows services to register themselves and discover other services.
Leader Election: Provides algorithms for electing a leader among distributed services.
Configuration Management: Stores configuration data in a hierarchical structure.
Service Registration Example:
spring:
cloud:
zookeeper:
discovery:
root: /services
instance-id: ${spring.application.name}:${spring.application.instance_id:${random.value}}
service-name: my-service
root
: Defines the root path in Zookeeper where services are registered.instance-id
: Assigns a unique ID to each service instance.
Configuration Management Example:
spring:
cloud:
zookeeper:
config:
root: /config
default-context: application
root
: Specifies the root path for configuration data.
Comparison with Eureka
Eureka is a service discovery tool from Netflix that is part of the Spring Cloud ecosystem. It is often used in conjunction with Spring Boot applications for service discovery.
Consul/Zookeeper vs. Eureka:
Consistency: Zookeeper provides strong consistency, while Eureka offers eventual consistency. Consul offers a balance with its raft-based consensus algorithm.
Health Checks: Consul has built-in health checks; Eureka requires custom implementations.
Configuration Management: Consul and Zookeeper offer centralized configuration management, whereas Eureka focuses primarily on service discovery.
Community Support: Eureka has strong integration with Spring Cloud, while Consul and Zookeeper are more versatile and can be used outside the Spring ecosystem as well.
Integrating with Spring Cloud Applications
Integrating Consul:
Spring Cloud Consul makes it easy to integrate Spring applications with Consul for service discovery and configuration management.
Maven Dependency:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-consul-discovery</artifactId>
</dependency>
Integrating Zookeeper:
Spring Cloud Zookeeper integrates Zookeeper’s service discovery and configuration management features with Spring Cloud applications.
Maven Dependency:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-zookeeper-discovery</artifactId>
</dependency>
Both integrations allow microservices to register themselves with Consul/Zookeeper and to discover other services in the network, making the architecture more resilient and scalable.
15. Spring Cloud Contract
Overview
Spring Cloud Contract is a project that facilitates Consumer-Driven Contract (CDC) testing, which ensures that services in a microservices architecture are compatible with each other. This tool allows developers to define contracts that describe how services should interact, and then automatically generate tests based on those contracts.
Consumer-Driven Contract Testing
Consumer-Driven Contracts are agreements between service consumers and providers. They specify the interactions expected by the consumer, which the provider agrees to uphold. This approach ensures that changes in the provider do not break the consumer’s expectations.
Benefits:
Ensures backward compatibility.
Facilitates collaboration between teams.
Reduces the likelihood of integration issues.
Setting Up Spring Cloud Contract
Adding Dependencies
To get started with Spring Cloud Contract, add the necessary dependencies to your project.
Maven Configuration:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-contract-verifier</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-contract-spec</artifactId>
<version>3.0.0</version>
</dependency>
Writing Contracts
Contracts in Spring Cloud Contract are written in Groovy or YAML and define the expected requests and responses between services.
Example Groovy Contract:
Contract.make {
request {
method 'GET'
url '/api/resource'
headers {
contentType(applicationJson())
}
}
response {
status 200
body([
id: $(regex('[0-9]+')),
name: $(regex('[a-zA-Z]+'))
])
headers {
contentType(applicationJson())
}
}
}
request {}
: Defines the expected HTTP request.response {}
: Defines the expected HTTP response.
Verifying Contracts
Spring Cloud Contract automatically generates tests from the contracts, which can be run to verify that the service provider adheres to the contract.
Generated Test Example:
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.MOCK)
@AutoConfigureMockMvc
@AutoConfigureStubRunner(ids = "com.example:producer:+:stubs:8080")
public class ContractVerifierTest {
@Autowired
private MockMvc mockMvc;
@Test
public void validate_should_return_resource() throws Exception {
mockMvc.perform(get("/api/resource")
.contentType(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(jsonPath("$.id").value(matchesRegex("[0-9]+")))
.andExpect(jsonPath("$.name").value(matchesRegex("[a-zA-Z]+")));
}
}
- @AutoConfigureStubRunner: Automatically downloads the stubs (contracts) and runs them locally to verify the provider's behavior.
Writing and Verifying Contracts
Writing Contracts involves defining interactions between the consumer and provider using Groovy or YAML. The contracts describe the request that the consumer will send and the response that the provider should return.
Verifying Contracts is the process where the provider service tests are generated based on the contracts, ensuring the service behaves as expected. The verification process catches discrepancies early, reducing integration issues.
Integrating with CI/CD Pipelines
Continuous Integration (CI) and Continuous Deployment (CD) pipelines can integrate Spring Cloud Contract to automate contract testing. This ensures that every change in the codebase is validated against the defined contracts, maintaining compatibility between services.
Typical Pipeline Steps:
Build and Test: Code is compiled, and unit tests are run.
Contract Verification: Contracts are verified against the service provider.
Stub Generation: If the provider passes the contract tests, stubs are generated and published.
Consumer Tests: Consumers use the published stubs to test against their expectations.
Example Jenkins Pipeline:
pipeline {
agent any
stages {
stage('Build and Test') {
steps {
sh './gradlew clean build'
}
}
stage('Contract Verification') {
steps {
sh './gradlew contractVerify'
}
}
stage('Publish Stubs') {
steps {
sh './gradlew publishToMavenLocal'
}
}
}
}
contractVerify
: Runs the contract verification process.publishToMavenLocal
: Publishes the generated stubs.
Integrating Spring Cloud Contract into CI/CD pipelines ensures that services are continuously tested for compliance with their contracts, leading to more stable and reliable microservices deployments.
16. Spring Cloud Task
Overview
Spring Cloud Task is a project designed to manage short-lived microservices, often referred to as tasks. Unlike traditional microservices that are long-running and continuously processing requests, tasks in Spring Cloud Task are typically single-use, short-lived, and perform a specific job, like batch processing or data migration. Once the job is completed, the task shuts down.
Managing Short-Lived Microservices
Short-Lived Microservices (Tasks)
Tasks in Spring Cloud Task are designed to execute specific operations that have a defined start and end. They are commonly used for batch processing, data import/export operations, or any other processes that need to be executed periodically or on-demand.
Characteristics of Short-Lived Tasks:
Single-Use: Tasks are initiated, perform their function, and then terminate.
Stateless: Tasks typically do not maintain any state once they are completed.
Autonomous: Each task operates independently of other tasks.
Use Case Example:
- Batch Processing: A task could be designed to process a large batch of data every night, and once the processing is done, the task would stop running.
Creating and Scheduling Tasks
Creating a Task
Creating a task with Spring Cloud Task is straightforward. It can be as simple as annotating a Spring Boot application with @EnableTask
.
Example Task Application:
@SpringBootApplication
@EnableTask
public class MyTaskApplication {
public static void main(String[] args) {
SpringApplication.run(MyTaskApplication.class, args);
}
@Bean
public CommandLineRunner commandLineRunner() {
return args -> {
System.out.println("Task is running...");
// Task logic here
};
}
}
@EnableTask: Enables Spring Cloud Task features.
CommandLineRunner: Contains the logic for the task.
Scheduling Tasks
While Spring Cloud Task focuses on task management, scheduling tasks can be achieved through Spring’s @Scheduled
annotation or by integrating with other scheduling tools like Spring Cloud Data Flow or Kubernetes CronJobs.
Example Scheduled Task:
@SpringBootApplication
@EnableTask
public class ScheduledTaskApplication {
public static void main(String[] args) {
SpringApplication.run(ScheduledTaskApplication.class, args);
}
@Scheduled(fixedRate = 5000)
public void runTask() {
System.out.println("Scheduled task is running...");
// Task logic here
}
}
- @Scheduled: Schedules the task to run at fixed intervals (e.g., every 5 seconds).
Monitoring and Tracing Tasks
Monitoring and tracing are critical for understanding the behavior and performance of tasks, especially when tasks are part of a larger system.
Task Execution Monitoring
Spring Cloud Task provides built-in mechanisms for monitoring the execution of tasks. This includes tracking task start and end times, exit codes, and execution status.
Task Execution Database Schema:
TASK_EXECUTION: Stores information about each task execution, such as start time, end time, and exit code.
TASK_PARAMS: Stores the parameters passed to the task at runtime.
Task Tracing with Spring Cloud Sleuth
Integrating Spring Cloud Sleuth with Spring Cloud Task allows for distributed tracing, which is particularly useful in microservices environments where tasks may interact with other services.
Example Sleuth Integration:
spring:
sleuth:
task:
enabled: true
enabled: true
: Enables tracing for tasks, allowing you to trace the execution flow across microservices and tasks.
17. Spring Cloud Data Flow
Overview
Spring Cloud Data Flow is a microservices-based toolkit for building, deploying, and managing data processing pipelines, which can include both stream and task applications. It provides a unified service for orchestrating and managing data flows, enabling the creation of complex workflows that involve data ingestion, processing, and output.
Orchestrating Data Pipelines
Data Pipelines
A data pipeline is a series of data processing steps. Spring Cloud Data Flow allows developers to define and manage these pipelines using stream and task applications.
Stream Applications: Handle continuous data flows (e.g., real-time data processing). Task Applications: Handle batch or short-lived data processing (e.g., ETL jobs).
Building and Deploying Stream and Task Applications
Stream Applications
Stream applications in Spring Cloud Data Flow are typically built using Spring Cloud Stream. They are designed to handle continuous data flow, making them ideal for real-time data processing.
Example Stream Application:
stream create --name mystream --definition "http | log" --deploy
http: A source application that ingests data from an HTTP endpoint.
log: A sink application that logs the data.
The stream above will capture data from an HTTP endpoint and log it.
Task Applications
Task applications, as mentioned earlier, are designed for batch processing or short-lived tasks. These tasks can be part of a larger data pipeline managed by Spring Cloud Data Flow.
Example Task Application Deployment:
task create --name mytask --definition "timestamp"
task launch mytask
- timestamp: A simple task application that logs the current timestamp.
Monitoring and Scaling Data Flows
Monitoring Data Flows
Spring Cloud Data Flow provides a dashboard and REST APIs for monitoring the status and performance of data pipelines. This includes visualizing the data flow, checking the status of individual components, and monitoring resource usage.
Dashboard Features:
Visual Pipeline Designer: Drag-and-drop interface to design data pipelines.
Real-Time Metrics: Track metrics such as throughput, latency, and error rates.
Scaling Data Flows
Spring Cloud Data Flow supports scaling both stream and task applications. Stream applications can be scaled horizontally by increasing the number of instances handling the data flow, while task applications can be scaled by parallelizing batch processing tasks.
Scaling Stream Applications Example:
stream scale --name mystream --instances 3
- instances: Specifies the number of instances to run for the stream application.
Scaling Task Applications Example:
task launch --name mytask --properties "spring.cloud.task.numberOfPartitions=5"
- numberOfPartitions: Specifies the number of partitions to parallelize the task.
18. Spring Cloud Deployer
Spring Cloud Deployer is a toolkit designed to facilitate the deployment of Spring applications across various platforms. It abstracts the deployment process and provides a consistent interface for deploying applications to different environments such as local servers, Kubernetes, and Cloud Foundry.
Deploying Applications on Various Platforms
Local Deployment
Local Deployment involves running applications on your local machine. Spring Cloud Deployer abstracts this process, allowing you to deploy applications with minimal configuration.
Example:
public class MyLocalDeployerApplication {
public static void main(String[] args) {
SpringApplication.run(MyLocalDeployerApplication.class, args);
}
}
- The application can be run using standard Java commands (e.g.,
java -jar myapp.jar
).
Kubernetes Deployment
Kubernetes Deployment involves deploying Spring applications as containers within a Kubernetes cluster. Spring Cloud Deployer supports this by leveraging Kubernetes APIs and resources.
Configuration Example:
spring:
cloud:
deployer:
k8s:
namespace: default
deployment:
replicas: 3
namespace: Kubernetes namespace for the deployment.
replicas: Number of pod replicas for the deployment.
Deployment Command:
spring-cloud-deployer deploy myapp --platform kubernetes
Cloud Foundry Deployment
Cloud Foundry Deployment involves deploying applications to a Cloud Foundry platform, which is a cloud-native platform-as-a-service (PaaS) offering.
Configuration Example:
spring:
cloud:
deployer:
cloudfoundry:
organization: my-org
space: my-space
organization: Cloud Foundry organization.
space: Cloud Foundry space for deployment.
Deployment Command:
spring-cloud-deployer deploy myapp --platform cloudfoundry
Customising Deployment Strategies
Spring Cloud Deployer allows you to customise deployment strategies to fit specific requirements, such as configuring resource limits, environment variables, or deployment behaviors.
Example Customisation:
Kubernetes Customisation:
spring:
cloud:
deployer:
k8s:
resource:
limits:
cpu: "1"
memory: "1Gi"
env:
- name: SPRING_PROFILES_ACTIVE
value: "prod"
limits: Defines CPU and memory resource limits.
env: Sets environment variables.
Scaling and Monitoring Deployed Applications
Scaling
Spring Cloud Deployer supports scaling applications by adjusting the number of instances or replicas.
Example Command:
spring-cloud-deployer scale myapp --instances 5
- instances: Specifies the number of instances to scale to.
Monitoring
Monitoring involves tracking the health, performance, and logs of deployed applications. Spring Cloud Deployer integrates with various monitoring tools and platforms.
Example Monitoring Integration:
Prometheus: For collecting and querying metrics.
Grafana: For visualizing metrics.
19. Spring Cloud Functions
Overview
Spring Cloud Functions is a project that enables the development and deployment of cloud-agnostic functions. These functions can be deployed to various cloud platforms and executed as serverless applications.
Writing Cloud-Agnostic Functions
Cloud-Agnostic Functions are functions designed to run on any cloud platform without modification. They are typically implemented using Java 8 functional interfaces such as Function
, Consumer
, or Supplier
.
Example Function:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.function.adapter.aws.SpringBootRequestHandler;
import org.springframework.context.annotation.Bean;
import java.util.function.Function;
@SpringBootApplication
public class MyFunctionApplication {
public static void main(String[] args) {
SpringApplication.run(MyFunctionApplication.class, args);
}
@Bean
public Function<String, String> uppercase() {
return value -> value.toUpperCase();
}
}
- Function<String, String>: A function that converts input strings to uppercase.
Deploying and Executing Functions
Functions can be deployed and executed on various serverless platforms, such as AWS Lambda, Azure Functions, or Google Cloud Functions.
AWS Lambda Deployment:
AWS Lambda Deployment Example:
Build Application: Package your function as a JAR file.
Deploy Function: Use AWS CLI or AWS Management Console to create and deploy the Lambda function.
AWS CLI Command:
aws lambda create-function --function-name MyFunction --runtime java11 --role arn:aws:iam::123456789012:role/lambda-role --handler com.example.MyFunctionHandler --zip-file fileb://myfunction.jar
Azure Functions Deployment:
Azure Functions Deployment Example:
Build Application: Package your function as a JAR file.
Deploy Function: Use Azure CLI or Azure Portal to create and deploy the Azure Function.
Azure CLI Command:
az functionapp deployment source config-zip --resource-group myResourceGroup --name myFunctionApp --src myfunction.zip
Integrating with Various Cloud Platforms
Integration Example:
AWS Lambda: Spring Cloud Functions integrates with AWS Lambda, allowing functions to be deployed as Lambda functions.
Azure Functions: Supports deploying functions as Azure Functions and interacting with Azure services.
Google Cloud Functions: Functions can be deployed to Google Cloud Functions, leveraging Google Cloud's serverless environment.
Best Practices for Serverless Spring Cloud Applications
Statelessness: Design functions to be stateless to ensure scalability and reliability.
Idempotency: Ensure that functions can handle repeated invocations without unintended side effects.
Cold Start Optimization: Minimize cold start times by optimizing function initialization.
Monitoring and Logging: Implement robust monitoring and logging to track function performance and troubleshoot issues.
Security: Secure functions by applying appropriate permissions and using secure secrets management.
20. Spring Cloud Vault
Overview
Spring Cloud Vault is a project that provides integration with HashiCorp Vault, a tool for managing secrets and sensitive data. It offers a secure way to store and access secrets, such as API keys, passwords, and configuration data, in Spring applications.
Secure Storage of Secrets
HashiCorp Vault is a tool designed to securely store and manage sensitive information. It provides features such as dynamic secrets, leasing, and revocation.
Vault Secrets Storage Example:
vault kv put secret/myapp db_password=mysecretpassword
secret/myapp: Path in Vault where secrets are stored.
db_password: Key for the secret.
Integrating with Spring Cloud Config
Spring Cloud Vault integrates with Spring Cloud Config to provide centralized configuration management with secure storage.
Configuration Example:
spring:
cloud:
vault:
uri: http://localhost:8200
token: my-vault-token
authentication: token
kv:
enabled: true
default-context: application
profile-separator: '/'
uri: Vault server URI.
token: Authentication token for accessing Vault.
kv: Configures Vault's key-value secrets engine.
Accessing Secrets in Application:
spring:
datasource:
url: ${vault.db_url}
username: ${vault.db_username}
password: ${vault.db_password}
- Secrets are referenced using
${vault.key}
syntax, wherekey
is the path to the secret in Vault.
Managing Secrets and Credentials Dynamically
Dynamic Secrets: Vault can generate secrets dynamically (e.g., database credentials) with a defined lease period.
Example Dynamic Secret Configuration:
# Vault configuration for dynamic database credentials
database secrets engine
database/config/mydb
{
"connection_url": "jdbc:mysql://localhost:3306/mydb",
"username": "admin",
"password": "adminpass"
}
database/roles/myrole
{
"db_name": "mydb",
"creation_statements": "CREATE USER '{{username}}'@'%' IDENTIFIED BY '{{password}}';",
"default_ttl": "1h",
"max_ttl": "24h"
}
creation_statements: Defines how dynamic credentials are created.
default_ttl: Time-to-live for the credentials.
Fetching Dynamic Credentials in Application:
@Autowired
private VaultTemplate vaultTemplate;
public String getDatabasePassword() {
return vaultTemplate.read("database/creds/myrole").getData().get("password");
}
- VaultTemplate: Spring Bean for interacting with Vault.
Secret Rotation and Revocation: Vault supports rotating secrets and revoking them as needed, enhancing security by ensuring that sensitive data is not exposed for extended periods.
21. Spring Cloud GCP (Google Cloud Platform)
Spring Cloud GCP integrates Spring applications with Google Cloud Platform services, enabling seamless interaction with Google’s cloud offerings while leveraging Spring’s features.
Integrating Spring Cloud with GCP Services
Spring Cloud GCP offers a wide range of integrations for Google Cloud services, simplifying the use of these services in Spring applications:
Google Cloud Storage: A scalable object storage service. You can easily integrate it with Spring applications to store and retrieve files.
Example: Uploading a file to Google Cloud Storage
@Service public class GCPStorageService { private final Storage storage; public GCPStorageService(Storage storage) { this.storage = storage; } public void uploadFile(String bucketName, String objectName, byte[] content) { BlobId blobId = BlobId.of(bucketName, objectName); BlobInfo blobInfo = BlobInfo.newBuilder(blobId).build(); storage.create(blobInfo, content); } }
Google Pub/Sub: A messaging service for building event-driven systems. Spring Cloud GCP allows for easy publishing and subscribing to Pub/Sub topics.
Example: Publishing a message to a Pub/Sub topic
@Service public class PubSubPublisher { private final PubSubTemplate pubSubTemplate; public PubSubPublisher(PubSubTemplate pubSubTemplate) { this.pubSubTemplate = pubSubTemplate; } public void publishMessage(String topicName, String message) { pubSubTemplate.publish(topicName, message); } }
Google Cloud SQL: A fully-managed relational database service that supports MySQL, PostgreSQL, and SQL Server. Spring Data JPA can be used with Cloud SQL for seamless database operations.
Google Cloud Firestore: A NoSQL document database, which integrates with Spring Data for scalable and flexible database operations.
Google Cloud Spanner: A globally distributed, horizontally scalable database. Spring Data Spanner provides a Spring Data abstraction over Cloud Spanner, allowing for Spring-friendly interaction.
Service Discovery, Configuration Management, and Messaging with GCP
Service Discovery: GCP doesn’t provide a native service discovery mechanism like Eureka, but Spring Cloud GCP can integrate with Google Kubernetes Engine (GKE) for service discovery and management. You can use Kubernetes service discovery to manage microservices in a GCP environment.
Configuration Management: Spring Cloud GCP integrates with Google Cloud Secret Manager and Google Cloud Config for managing configuration data and secrets across environments.
Example: Accessing secrets from Google Cloud Secret Manager
@Service public class SecretManagerService { private final SecretManagerServiceClient client; public SecretManagerService(SecretManagerServiceClient client) { this.client = client; } public String getSecret(String secretId, String versionId) { SecretVersionName secretVersionName = SecretVersionName.of("project-id", secretId, versionId); AccessSecretVersionResponse response = client.accessSecretVersion(secretVersionName); return response.getPayload().getData().toStringUtf8(); } }
Messaging: GCP Pub/Sub is integrated with Spring Cloud for asynchronous messaging between microservices, enabling scalable and reliable communication.
GCP-Native Features in Spring Cloud
Stackdriver Integration: Spring Cloud GCP integrates with Google Cloud Stackdriver for logging, monitoring, and tracing, enabling you to monitor your Spring applications effectively.
Security: Integration with Google Cloud IAM provides secure access to GCP services, enabling role-based access control and secure service-to-service communication.
Auto Configuration: Spring Cloud GCP provides auto-configuration for various GCP services, reducing boilerplate code and simplifying the integration process.
22. Spring Cloud Azure
Spring Cloud Azure simplifies the integration of Spring applications with Microsoft Azure services, enabling cloud-native development on Azure with Spring's features.
Integrating Spring Cloud with Microsoft Azure Services
Azure Blob Storage: A scalable object storage service for unstructured data, such as images and videos. Spring Cloud Azure integrates seamlessly with Blob Storage.
Example: Uploading a file to Azure Blob Storage
@Service public class AzureBlobService { private final BlobServiceClient blobServiceClient; public AzureBlobService(BlobServiceClient blobServiceClient) { this.blobServiceClient = blobServiceClient; } public void uploadFile(String containerName, String blobName, InputStream data) { BlobContainerClient containerClient = blobServiceClient.getBlobContainerClient(containerName); BlobClient blobClient = containerClient.getBlobClient(blobName); blobClient.upload(data, data.available()); } }
Azure Service Bus: A messaging service for enterprise messaging. Spring Cloud Azure enables easy integration with Service Bus queues and topics.
Example: Sending a message to an Azure Service Bus queue
@Service public class ServiceBusPublisher { private final ServiceBusTemplate serviceBusTemplate; public ServiceBusPublisher(ServiceBusTemplate serviceBusTemplate) { this.serviceBusTemplate = serviceBusTemplate; } public void sendMessage(String queueName, String message) { serviceBusTemplate.sendAsync(queueName, message).block(); } }
Azure Cosmos DB: A globally distributed, multi-model database service. Spring Data Azure Cosmos provides a Spring Data abstraction over Cosmos DB, making it easy to integrate with Spring applications.
Azure SQL Database: A fully-managed relational database service, supported by Spring Data JPA for seamless database operations.
Service Discovery, Configuration Management, and Messaging with Azure
Service Discovery: Spring Cloud Azure can integrate with Azure Kubernetes Service (AKS) and Azure App Services for service discovery. Azure Traffic Manager and Azure Load Balancer can be used for load balancing and routing.
Configuration Management: Azure App Configuration and Azure Key Vault allow for centralised management of application configurations and secrets.
Example: Accessing secrets from Azure Key Vault
@Service public class KeyVaultService { private final SecretClient secretClient; public KeyVaultService(SecretClient secretClient) { this.secretClient = secretClient; } public String getSecret(String secretName) { return secretClient.getSecret(secretName).getValue(); } }
Messaging: Azure Service Bus and Event Hubs are integrated into Spring Cloud Azure, enabling reliable and scalable messaging between microservices.
Azure-Native Features in Spring Cloud
Security: Spring Cloud Azure integrates with Azure Active Directory (AAD) for secure authentication and authorisation, supporting OAuth2, OpenID Connect, and role-based access control.
Monitoring and Logging: Azure Monitor and Application Insights provide comprehensive monitoring, logging, and diagnostics for Spring applications running on Azure.
Auto Configuration: Spring Cloud Azure offers auto-configuration for various Azure services, making it easy to use Azure features in Spring applications.
23. Spring Cloud AWS
Spring Cloud AWS integrates Spring applications with Amazon Web Services (AWS), offering a wide range of tools and frameworks to use AWS services efficiently.
Integrating Spring Cloud with AWS Services
Amazon S3: A scalable object storage service. Spring Cloud AWS simplifies the integration with Amazon S3 for storing and retrieving files.
Example: Uploading a file to Amazon S3
@Service public class S3Service { private final AmazonS3 amazonS3; @Value("${aws.s3.bucket-name}") private String bucketName; public S3Service(AmazonS3 amazonS3) { this.amazonS3 = amazonS3; } public void uploadFile(String key, File file) { amazonS3.putObject(new PutObjectRequest(bucketName, key, file)); } }
Amazon RDS: A fully-managed relational database service that supports MySQL, PostgreSQL, and more. Spring Data JPA can be used to interact with databases on Amazon RDS.
Amazon DynamoDB: A NoSQL database service. Spring Data DynamoDB provides a Spring Data abstraction over DynamoDB, enabling easy integration with Spring applications.
Amazon SNS and SQS: Messaging services for event-driven systems. Spring Cloud AWS provides support for publishing messages to SNS topics and consuming messages from SQS queues.
Example: Sending a message to an SQS queue
@Service public class SQSPublisher { private final QueueMessagingTemplate queueMessagingTemplate; public SQSPublisher(QueueMessagingTemplate queueMessagingTemplate) { this.queueMessagingTemplate = queueMessagingTemplate; } public void sendMessage(String queueName, String message) { queueMessagingTemplate.convertAndSend(queueName, message); } }
Service Discovery, Configuration Management, and Messaging with AWS
Service Discovery: Spring Cloud AWS can use AWS Elastic Load Balancing (ELB) and Amazon Route 53 for service discovery and load balancing. AWS Auto Scaling integrates with Spring Cloud to provide dynamic service scaling based on demand.
Configuration Management: AWS Systems Manager Parameter Store and AWS Secrets Manager can be used for managing configuration properties and secrets across different environments.
Example: Accessing a parameter from AWS Systems Manager Parameter Store
@Service public class ParameterStoreService { private final AWSSimpleSystemsManagement ssm; public ParameterStoreService(AWSSimpleSystemsManagement ssm) { this.ssm = ssm; } public String getParameter(String parameterName) { GetParameterRequest request = new GetParameterRequest().withName(parameterName).withWithDecryption(true); return ssm.getParameter(request).getParameter().getValue(); } }
Messaging: AWS SNS and SQS provide robust messaging capabilities for decoupling microservices. Spring Cloud AWS allows for easy integration with these services.
AWS-Native Features in Spring Cloud
Security: Integration with AWS Identity and Access Management (IAM) allows for secure access control and management of AWS services, ensuring that only authorised services and users have access to resources.
Monitoring: Spring Cloud AWS integrates with AWS CloudWatch for centralized monitoring, logging, and alerting, providing insights into the performance and health of your Spring applications.
Auto Configuration: Spring Cloud AWS offers auto-configuration for various AWS services, reducing the complexity of setup and enabling easy use of AWS features in Spring applications.
24. Spring Cloud for Microservices Communication
Microservices architecture involves multiple services working together to form a cohesive application. Effective communication between these services is crucial for the system's performance, scalability, and reliability.
Synchronous vs. Asynchronous Communication
Synchronous Communication:
Definition: In synchronous communication, the calling service sends a request to another service and waits for a response before continuing its execution.
Use Cases: When the outcome of a service call is immediately needed by the calling service, such as retrieving user details before processing an order.
Examples in Spring Cloud:
REST APIs: Using
RestTemplate
orWebClient
to make HTTP calls between microservices.gRPC: A high-performance RPC (Remote Procedure Call) framework that enables synchronous communication between services.
Example: Using RestTemplate
for synchronous communication
@Service
public class OrderService {
private final RestTemplate restTemplate;
public OrderService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public UserDetails getUserDetails(String userId) {
return restTemplate.getForObject("http://user-service/users/" + userId, UserDetails.class);
}
}
Asynchronous Communication:
Definition: In asynchronous communication, the calling service sends a request or message and immediately continues its execution without waiting for a response.
Use Cases: When tasks can be processed independently, such as sending notifications or processing background jobs.
Examples in Spring Cloud:
Message Brokers: Using RabbitMQ, Kafka, or Google Pub/Sub for message-based communication.
WebSockets: For real-time communication between services or between a server and clients.
Example: Sending a message asynchronously using RabbitMQ
@Service
public class NotificationService {
private final RabbitTemplate rabbitTemplate;
public NotificationService(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void sendNotification(Notification notification) {
rabbitTemplate.convertAndSend("notification.exchange", "notification.routingkey", notification);
}
}
Implementing gRPC with Spring Cloud
gRPC is a high-performance, open-source framework developed by Google that allows services to communicate with each other using Remote Procedure Calls (RPC). It's ideal for situations where you need efficient and robust communication between microservices.
Benefits of gRPC:
Efficiency: gRPC uses HTTP/2, which is more efficient than traditional HTTP, especially for multiplexing streams.
Strongly Typed Contracts: gRPC relies on Protocol Buffers (protobuf) for message serialization, providing a strongly typed contract between services.
Bidirectional Streaming: gRPC supports bidirectional streaming, allowing both client and server to send messages independently over a single connection.
Setting Up gRPC with Spring Cloud:
Define your service contracts using
.proto
files.Generate Java classes from these
.proto
files using the Protocol Buffers compiler.Implement the service logic in your Spring application using the generated classes.
Example: Defining a gRPC service in a .proto
file
syntax = "proto3";
option java_package = "com.example.grpc";
service UserService {
rpc GetUserDetails (UserRequest) returns (UserResponse);
}
message UserRequest {
string userId = 1;
}
message UserResponse {
string name = 1;
string email = 2;
}
Example: Implementing the gRPC service in Spring Boot
@GrpcService
public class UserServiceImpl extends UserServiceGrpc.UserServiceImplBase {
@Override
public void getUserDetails(UserRequest request, StreamObserver<UserResponse> responseObserver) {
UserResponse response = UserResponse.newBuilder()
.setName("John Doe")
.setEmail("john.doe@example.com")
.build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
WebSockets and Message Brokers
WebSockets:
Definition: WebSockets provide full-duplex communication channels over a single TCP connection, enabling real-time data transfer between client and server.
Use Cases: Real-time applications like chat apps, live notifications, and real-time updates in dashboards.
Example: Implementing WebSocket communication in Spring Boot
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/ws").withSockJS();
}
}
Message Brokers:
Definition: Message brokers like RabbitMQ, Kafka, or Google Pub/Sub manage and route messages between microservices, enabling asynchronous communication.
Use Cases: Decoupling services, implementing event-driven architectures, and handling high volumes of messages reliably.
Example: Sending and receiving messages with RabbitMQ in Spring Boot
@RabbitListener(queues = "example.queue")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
25. Distributed Locking with Spring Cloud
In a distributed system, multiple instances of microservices might try to access or modify a shared resource concurrently, leading to race conditions or data inconsistencies. Distributed locking is a mechanism to ensure that only one instance can access a critical section of code at a time.
Implementing Distributed Locking in a Microservices Environment
Why Use Distributed Locking:
To prevent race conditions in a distributed environment where multiple instances of a service might attempt to modify the same resource simultaneously.
To ensure consistency and prevent data corruption in critical sections of code.
Techniques for Distributed Locking:
Using a Centralized Database: Implementing locks using a relational database by inserting lock records and checking them before accessing a resource.
Using Redis: Redis provides distributed lock capabilities through the
SETNX
command and theRedlock
algorithm.Using Zookeeper: Zookeeper provides strong consistency and can be used to implement distributed locks through its ephemeral nodes.
Using Consul: Consul's key-value store can be used for implementing distributed locks by acquiring and releasing locks based on specific keys.
Example: Implementing distributed locking using Redis in Spring Boot
@Service
public class RedisLockService {
private final StringRedisTemplate redisTemplate;
public RedisLockService(StringRedisTemplate redisTemplate) {
this.redisTemplate = redisTemplate;
}
public boolean lock(String lockKey, String lockValue) {
return redisTemplate.opsForValue().setIfAbsent(lockKey, lockValue, 10, TimeUnit.SECONDS);
}
public void unlock(String lockKey, String lockValue) {
String currentValue = redisTemplate.opsForValue().get(lockKey);
if (lockValue.equals(currentValue)) {
redisTemplate.delete(lockKey);
}
}
}
Best Practices for Distributed Locking:
Timeouts: Always set timeouts for locks to prevent deadlocks in case a service crashes or fails to release a lock.
Unique Identifiers: Use unique identifiers (e.g., UUIDs) for lock values to ensure that only the service instance that acquired the lock can release it.
Retry Mechanisms: Implement retry mechanisms in case of lock acquisition failure to avoid overwhelming the system.
26. Spring Cloud Deployed on Multiple Cloud Providers
As organisations adopt multi-cloud strategies, they deploy applications across multiple cloud providers (e.g., AWS, Azure, GCP) to leverage the strengths of each provider, achieve redundancy, and avoid vendor lock-in. Spring Cloud can be used effectively in such multi-cloud environments.
Multi-Cloud Strategies with Spring Cloud
Why Use a Multi-Cloud Strategy:
Redundancy and High Availability: Deploying applications across multiple cloud providers can ensure continued availability even if one provider faces an outage.
Avoiding Vendor Lock-In: By spreading workloads across different providers, organizations can avoid becoming too dependent on a single cloud provider.
Optimizing Costs: Different cloud providers offer competitive pricing for various services, allowing organizations to optimize costs by selecting the most cost-effective options.
Key Considerations in a Multi-Cloud Environment:
Service Discovery: Managing service discovery across multiple clouds can be challenging. Using a global service discovery tool like Consul can help unify service registration and discovery across different environments.
Configuration Management: Ensuring consistent configuration management across multiple cloud providers is crucial. Tools like Spring Cloud Config, in conjunction with cloud-native configuration services (e.g., AWS Systems Manager Parameter Store, Azure App Configuration), can help maintain consistent configurations.
Cross-Cloud Networking: Managing networking between services deployed on different cloud providers requires careful planning. VPNs, direct interconnects, or cloud-native networking solutions may be necessary to ensure secure and efficient communication between services.
Data Consistency: Managing data consistency across multiple clouds can be complex, especially if data needs to be replicated or synchronized between different environments.
Challenges and Best Practices for Multi-Cloud Deployments
Challenges:
Complexity: Managing applications across multiple cloud providers adds complexity in terms of infrastructure management, monitoring, and debugging.
Latency: Cross-cloud communication may introduce latency, impacting the performance of applications that rely on real-time data or interactions.
Security: Ensuring consistent security policies across different cloud environments can be challenging, especially with differing capabilities and configurations.
Best Practices:
Use Abstractions: Spring Cloud provides abstractions for common cloud functionalities, making it easier to switch between different cloud providers without rewriting significant portions of the application code.
Centralized Monitoring and Logging: Use centralized monitoring and logging tools that can aggregate data from multiple cloud providers, providing a unified view of application performance and health.
Automated Deployments: Automate the deployment process using CI/CD pipelines that support multi-cloud environments, ensuring consistent and repeatable deployments across different providers.
Regular Testing: Regularly test failover scenarios and disaster recovery plans to ensure that the application can handle outages or issues with individual cloud providers.
Example: Multi-Cloud Service Discovery with Consul
Consul can be used as a global service discovery tool in a multi-cloud environment. Services deployed on different cloud providers register themselves with a central Consul cluster, allowing them to discover each other across cloud boundaries.
Example: Registering a service with Consul in Spring Boot
@SpringBootApplication
@EnableDiscoveryClient
public class MultiCloudServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MultiCloudServiceApplication.class, args);
}
}
Example: Accessing a service across cloud providers
@RestController
public class GreetingController {
private final RestTemplate restTemplate;
public GreetingController(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@GetMapping("/greet")
public String greet() {
String greetingServiceUrl = "http://greeting-service/greet";
return restTemplate.getForObject(greetingServiceUrl, String.class);
}
}
28. Spring Cloud Customisation
Extending and Customising Spring Cloud Components
Why Customise Spring Cloud?
- While Spring Cloud provides many features out of the box, there are scenarios where you may need to extend or customize components to meet specific business requirements.
Examples of Customisation:
Custom Load Balancing: Implement a load-balancing strategy that fits your application's unique needs, such as weighted load balancing based on service health.
Custom Retry Mechanism: Define custom retry policies for handling transient communication failures between microservices.
Example: Implementing a custom load balancer
@Bean
public ReactorLoadBalancer<ServiceInstance> customLoadBalancer(Environment environment, LoadBalancerClientFactory clientFactory) {
return new CustomLoadBalancer(clientFactory.getLazyProvider("serviceId", ServiceInstanceListSupplier.class), environment);
}
Writing Custom Spring Cloud Filters and Interceptors
Filters and Interceptors:
Filters: In Spring Cloud Gateway, filters modify requests and responses. They can be applied globally or on a per-route basis.
Interceptors: In Spring MVC or WebClient, interceptors intercept HTTP requests and responses, allowing you to add custom logic like logging or authentication.
Use Cases:
Logging: Implement a custom filter to log request and response details for audit purposes.
Security: Create a custom filter to enforce additional security checks before forwarding requests to backend services.
Example: Writing a custom filter in Spring Cloud Gateway
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("custom_route", r -> r.path("/custom/**")
.filters(f -> f.filter(new CustomGatewayFilter()))
.uri("http://example.com"))
.build();
}
Customising Spring Cloud Security and Circuit Breaker Implementations
Security Customisation:
- Customise Spring Security to integrate with custom OAuth2 providers, or add multi-factor authentication.
Example: Custom OAuth2 provider integration
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.withClientDetails(new CustomClientDetailsService());
}
Circuit Breaker Customisation:
Customise circuit breaker behavior, such as defining custom rules for circuit breaking, fallback logic, and resilience strategies.
Extend or replace default implementations provided by Resilience4j or Hystrix to meet your specific needs.
Example: Customising a circuit breaker with Resilience4j
@Bean
public Customizer<Resilience4JCircuitBreakerFactory> customCircuitBreakerFactory() {
return factory -> factory.configure(builder -> builder
.timeLimiterConfig(TimeLimiterConfig.custom().timeoutDuration(Duration.ofSeconds(4)).build())
.circuitBreakerConfig(CircuitBreakerConfig.custom().failureRateThreshold(50).build()), "customCircuitBreaker");
}
29. Advanced Topics
Distributed Caching with Spring Cloud
What is Distributed Caching?
- Distributed caching stores data across multiple servers or nodes, improving application performance by reducing backend load and latency.
Implementing Distributed Caching:
Redis: A popular in-memory data structure store used as a distributed cache in Spring applications.
Hazelcast: An in-memory data grid providing distributed caching, data storage, and computation.
Example: Implementing distributed caching with Redis in Spring Boot
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory connectionFactory) {
RedisCacheConfiguration cacheConfig = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofMinutes(10))
.disableCachingNullValues();
return RedisCacheManager.builder(connectionFactory).cacheDefaults(cacheConfig).build();
}
}
Microservices Patterns (Circuit Breaker, Saga, etc.)
Circuit Breaker Pattern: Prevents cascading failures by stopping the execution of requests that are likely to fail.
Saga Pattern: Manages distributed transactions across multiple services by breaking them into a series of smaller, compensatable transactions.
Example: Implementing a circuit breaker with Resilience4j
@CircuitBreaker(name = "backendA", fallbackMethod = "fallback")
public String performRemoteCall() {
return restTemplate.getForObject("http://remote-service/resource", String.class);
}
public String fallback(Throwable t) {
return "Fallback response due to: " + t.getMessage();
}
Monitoring and Observability with Spring Boot Admin and Micrometer
Spring Boot Admin: A UI for managing and monitoring Spring Boot applications, displaying health, metrics, and logs.
Micrometer: An application metrics facade that integrates with multiple monitoring systems like Prometheus, Graphite, and Datadog.
Example: Setting up Micrometer with Prometheus
management:
endpoints:
web:
exposure:
include: "*"
metrics:
export:
prometheus:
enabled: true
Handling Distributed Transactions
Two-Phase Commit: Ensures all participants in a distributed transaction either commit or roll back changes consistently.
Saga Pattern: Manages distributed transactions by breaking them into smaller transactions with compensating actions.
30. Best Practices and Challenges
Strategies for Deploying Spring Cloud Applications
Containerization: Deploy Spring Cloud applications in containers (e.g., Docker) to ensure consistency across environments and simplify deployment processes.
CI/CD Pipelines: Implement CI/CD pipelines to automate the build, test, and deployment processes, ensuring that code changes are continuously integrated and deployed.
Common Challenges and How to Overcome Them
Configuration Management: Managing environment-specific configurations can be challenging. Use Spring Cloud Config to centralize configuration management and manage different environments effectively.
Service Discovery: In dynamic environments, service discovery can become complex. Spring Cloud Netflix Eureka or Consul can help manage service registration and discovery.
Scaling: Scaling microservices requires careful consideration of resource allocation and load balancing. Use tools like Kubernetes and Spring Cloud LoadBalancer to manage scaling effectively.
Tips for Scaling and Managing Microservices
Use a Service Mesh: Implement a service mesh to manage traffic, security, and observability across microservices, allowing you to scale services independently.
Implement Resilience Patterns: Use resilience patterns like circuit breakers, retries, and bulkheads to ensure that microservices can handle failures gracefully.
Monitoring and Logging: Implement comprehensive monitoring and logging to gain insights into system performance and quickly identify and resolve issues.
Conclusion :
This comprehensive guide to Spring Cloud serves as an essential resource for understanding and implementing microservices architecture. It covers a wide range of topics, from fundamental concepts like configuration management and service discovery to advanced practices such as distributed locking and multi-cloud deployments. By exploring these topics, you will gain a deep understanding of how to build, deploy, and manage resilient, scalable microservices using Spring Cloud, while also staying informed about emerging trends and best practices in the field.
Subscribe to my newsletter
Read articles from Bikash Nishank directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
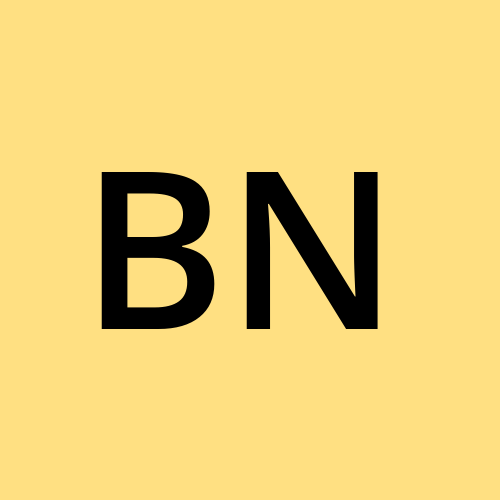