Using Sitecore Webhooks to Publish Items to an Algolia Index
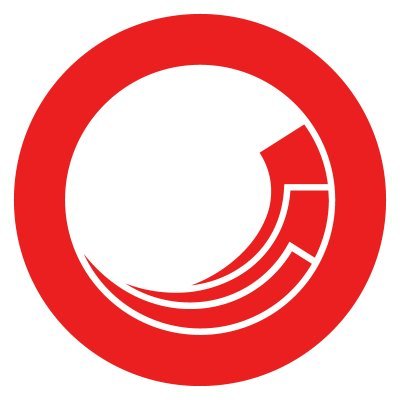
Integrating Algolia, a powerful search and discovery API, with Sitecore can significantly enhance your site's search functionality by delivering fast, relevant results to users. One efficient way to synchronize Sitecore content with an Algolia index is through the use of Sitecore Webhooks. This method automates the process of updating your Algolia index whenever content is published in Sitecore.
What Are Sitecore Webhooks?
Sitecore Webhooks are user-defined HTTP callbacks triggered by specific events within Sitecore. When a particular event occurs, such as content publication, the webhook sends a request to a specified URL with the relevant data. This makes it possible to connect Sitecore with external systems, like Algolia, in real-time.
Setting Up Webhooks in Sitecore
To publish Sitecore items to an Algolia index using webhooks, you'll need to follow these steps:
1. Create a Custom Webhook Handler in Sitecore
Sitecore doesn’t come with a built-in webhook feature for publishing items to third-party services like Algolia. However, you can create a custom webhook handler to listen for the publish event and trigger an HTTP request to Algolia.
Step 1: Create the Webhook Handler
In your Sitecore solution, create a custom event handler that listens for the
item:published
event.This handler will capture the details of the published item, such as the item ID, language, and version.
Step 2: Format the Payload
Prepare the data payload to be sent to Algolia. This should include the item fields that you want to index, such as the title, content, and any other relevant metadata.
Optionally, you can serialize the item into JSON, which will be sent to Algolia.
2. Configure the Webhook to Trigger on Publish
Next, configure the webhook to trigger whenever an item is published in Sitecore.
Step 1: Register the Event Handler
Register your custom webhook handler in the
Sitecore.Events
.config
file or a custom configuration file in theApp_Config
folder.Ensure the handler is set to trigger on the
item:published
event.
Step 2: Define the Webhook Endpoint
In the webhook handler, define the Algolia API endpoint URL.
Use the Algolia API client or a simple HTTP request to send the data to the appropriate Algolia index.
3. Send the Data to Algolia
With the webhook configured, the next step is to send the data to Algolia whenever the event is triggered.
Step 1: Authenticate with Algolia
- Use your Algolia API key to authenticate the HTTP request. Ensure that the API key has the necessary permissions to update the index.
Step 2: Update the Algolia Index
In your webhook handler, make an HTTP POST request to the Algolia index with the serialized Sitecore item data.
Handle the response from Algolia to confirm the item was successfully indexed.
Sample Code for the Webhook Handler
Here’s a simplified example of what the custom webhook handler might look like:
public class PublishToAlgoliaHandler
{
public void OnItemPublished(object sender, EventArgs args)
{
// Get the published item
ItemPublishedEventArgs eventArgs = (ItemPublishedEventArgs)args;
Item item = eventArgs.PublishedItem;
// Prepare the payload
var algoliaData = new
{
objectID = item.ID.ToString(),
title = item["Title"],
content = item["Content"],
url = LinkManager.GetItemUrl(item)
};
// Serialize to JSON
string jsonPayload = JsonConvert.SerializeObject(algoliaData);
// Send to Algolia
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Add("X-Algolia-API-Key", "YourAlgoliaAPIKey");
client.DefaultRequestHeaders.Add("X-Algolia-Application-Id", "YourAlgoliaAppId");
var content = new StringContent(jsonPayload, Encoding.UTF8, "application/json");
var response = client.PostAsync("https://YourAlgoliaAppId.algolia.net/1/indexes/YourIndexName", content).Result;
if (!response.IsSuccessStatusCode)
{
Log.Error("Failed to update Algolia index", this);
}
}
}
}
Testing and Deployment
Test the Webhook: Before deploying to production, thoroughly test the webhook in a development environment. Ensure that items are being correctly published to the Algolia index and that the data is accurate.
Monitor and Log: Implement logging within your webhook handler to track when items are published to Algolia and to capture any errors. This will help you troubleshoot any issues that may arise.
Subscribe to my newsletter
Read articles from Sandeep Bhatia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
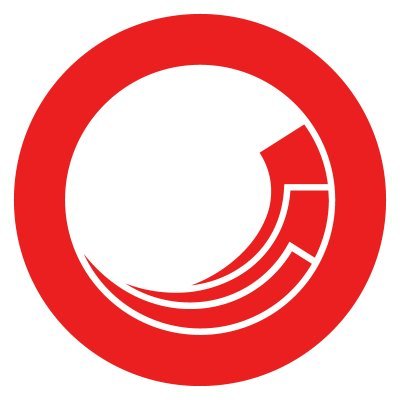
Sandeep Bhatia
Sandeep Bhatia
I am a developer from India.