Starting with Learning React but JS first
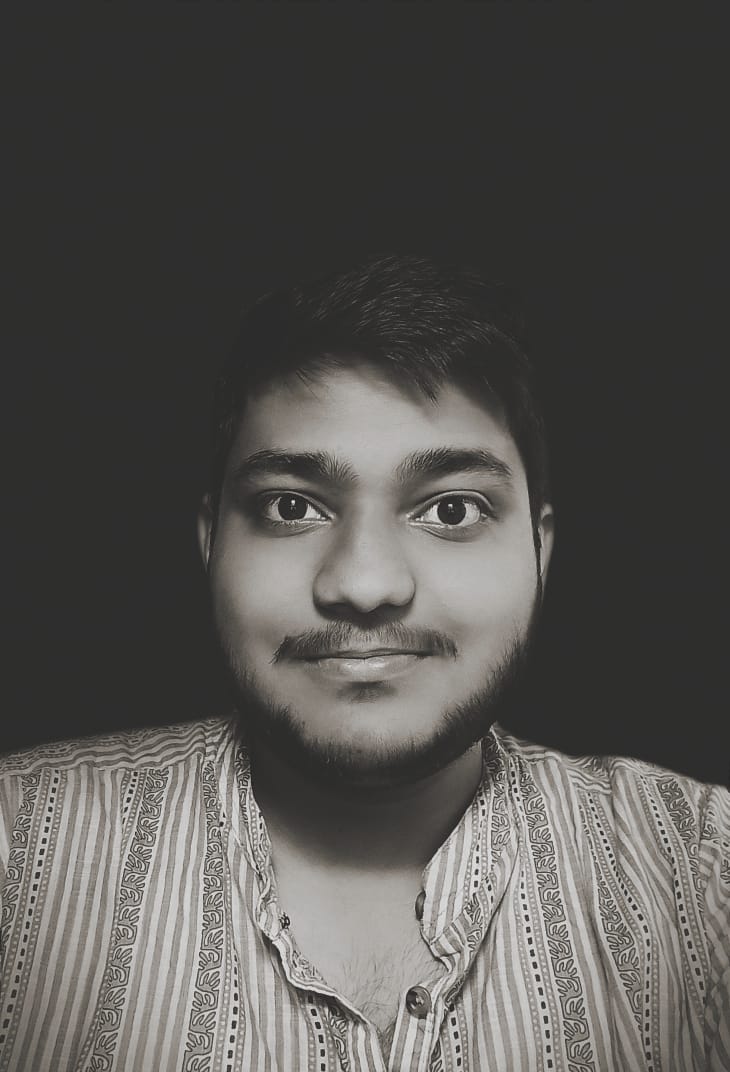
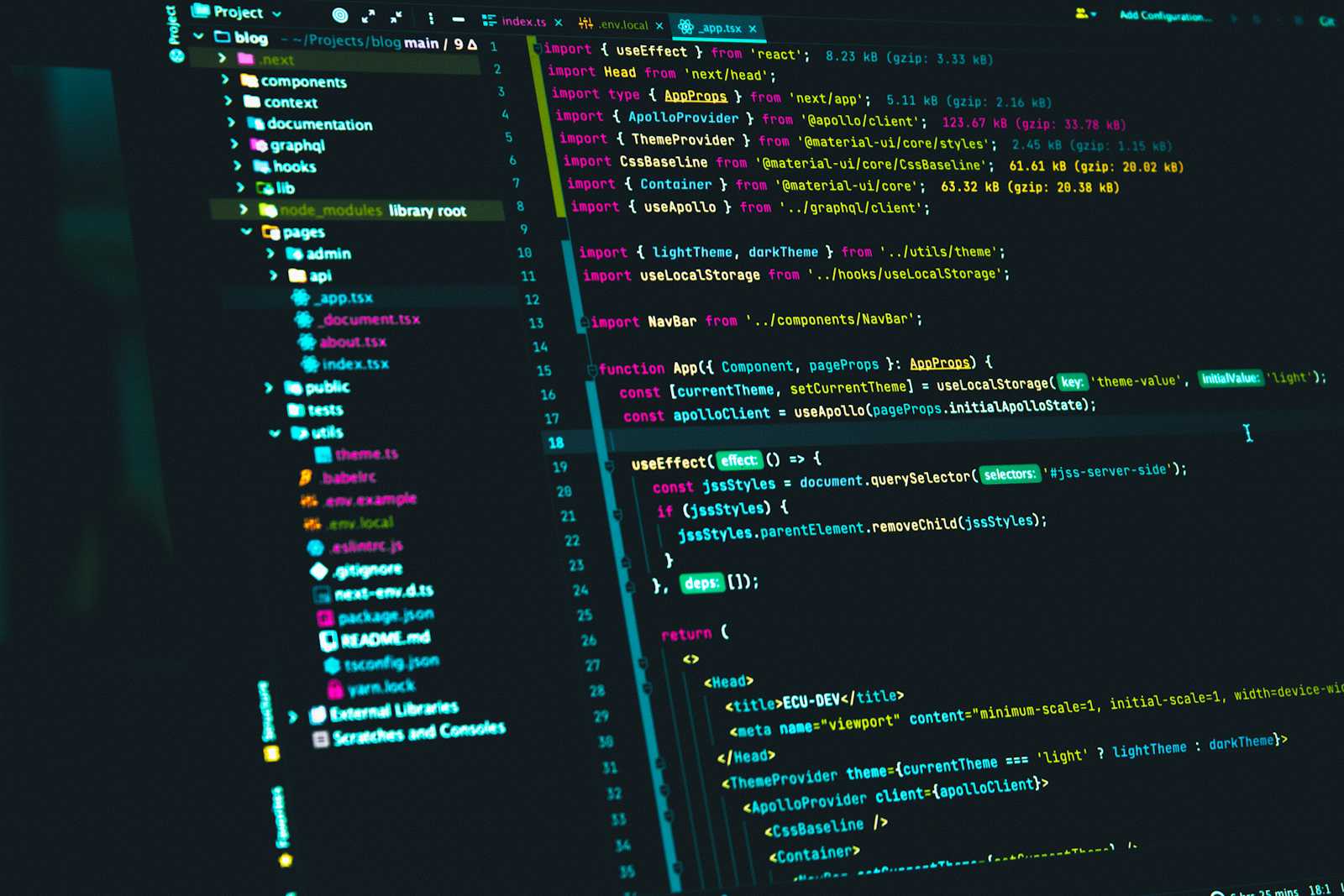
JS brush-up before Starting
Before Starting react, I have to get hold of most important JS concepts used in React such as Promises and Asynchronous JS Functions, Named/Default imports, Arrow functions, Promises and basic event handling and DOM manipulation.
Promises and Asynchronous Functions
Promises represent the completion (or failure) of an asynchronous operation and its resulting value.
They have methods like
.then()
,.catch()
, and.finally()
to handle results or errors.let promise = new Promise((resolve, reject) => { // Asynchronous operation setTimeout(() => resolve('Success'), 1000); }); promise .then(result => console.log(result)) .catch(error => console.error(error));
Asynchronous functions in JavaScript allow for non-blocking operations, meaning the code can execute without waiting for the asynchronous task to complete.
async function fetchData() { let response = await fetch('https://api.example.com/data'); let data = await response.json(); console.log(data); }
They are declared using the
async
keyword and typically involve theawait
keyword to pause execution until a promise resolves. This helps manage tasks like data fetching or timers efficiently.
Named/Default import in JS
In JavaScript ES6 modules, named imports and default imports help in managing and using code from other modules.
Named Imports: Import specific exports from a module by their name. You can import multiple items at once. While importing we have to use same name for the function or JS object.
import { functionA, objectB } from './module';
Default Imports: Import the default export from a module. Each module can have only one default export. we can have any name assigned to this variable in the file importing that function.
import default FunctionA from './module';
Arrow Functions
Arrow functions are a concise way to write functions in JavaScript.
They use the
=>
syntax and are particularly useful for short functions. They also capture thethis
value from their enclosing context, which use in many cases.const add = (a, b) => a + b; // passing parameter a and b then returning answer directly const greet = name => `Hello, ${name}!`; // Or using parameter in the return value // Or for Asynchronous Function Calling using Arrow function const suyash = async (time) => { try { const response = await fetch("https://www.movie.com/moviename"); const movie = await response.json(); console.log(`This is function call for getting ${movie.name} ticket at ${time}`); } catch (error) { console.error('Error fetching movie:', error); } };
Understanding these concepts is crucial as they are used in react and a good understanding is absolute necessary for learning. As learning the wrong topic is far dangerous than learning nothing.
Subscribe to my newsletter
Read articles from Suyash Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
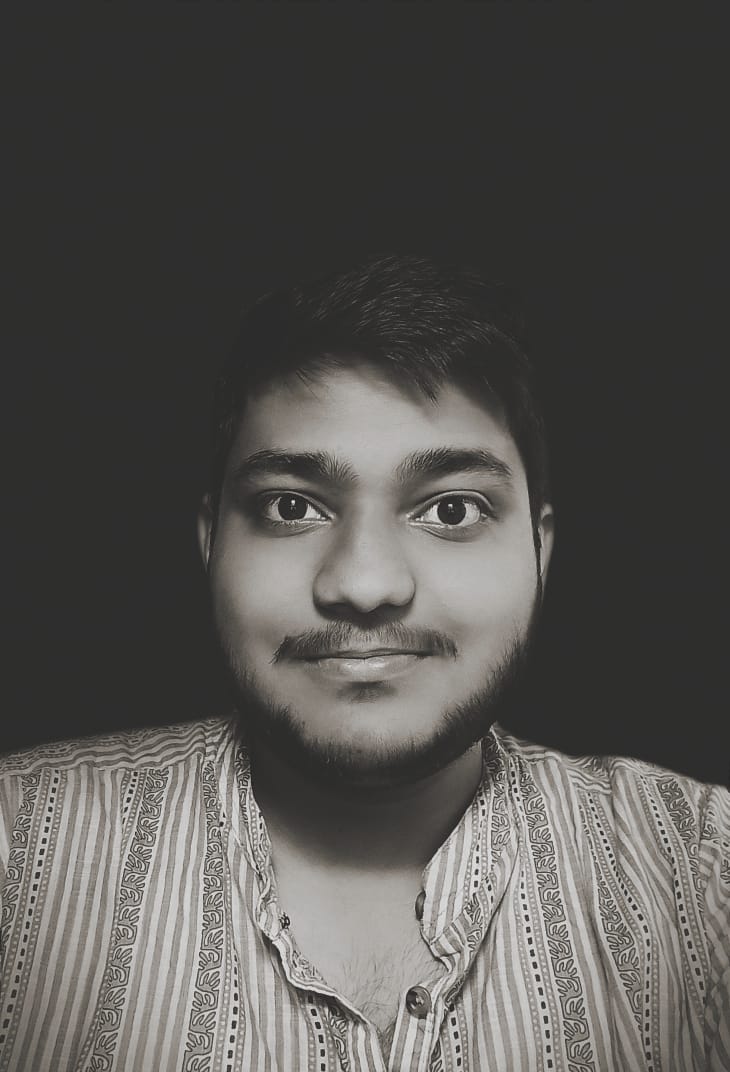
Suyash Pandey
Suyash Pandey
Hi, I am an Aspiring Software Engineer from India, dedicated to making real-life problem Solving Solutions.