A Guide to Data Types in JavaScript
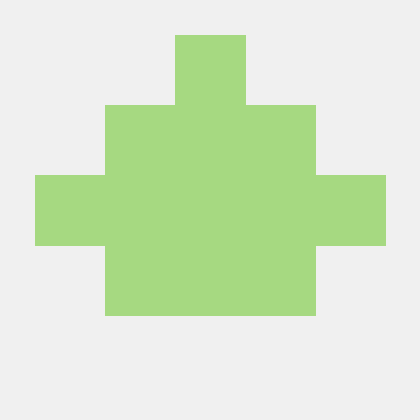
Introduction:
Are you interested in learning about JavaScript for Web development, Mobile App Development, Server-side Development, and more? Well, understanding the datatypes is invaluable so let's begin.
Terms to Know
Variables are containers where data is stored, they can contain data of Primitive (single data about something) or non-Primitive (multiple data about an entity) typing.
Variable Declaration is the process of creating the container, in JavaScript it can look something like this: let Name.
while Variable Assignment is the process of assigning a value to a Declared variable which can look like this: const Age = 18;(Constant) or this: let Name = "Chris";(Dynamic)
What even is A JavaScript Data Type?
A JavaScript Data Type simply categorizes data in the JavaScript Programming Language.
In life, everything is data but not all data can be used the same: a year can be added to your age, but a digit cannot be subtracted from your name; a yes or no question can be answered as either yes or no, a name or number is not expected and so much more, as a result of this, Data Types are created.
JavaScript is Dynamically Typed however which assigns a data type to a variable automatically instead of being forced to state the datatype that the variable must store during declaration unlike other Programming languages like Java, C, C++, C#, etc.
Types of Data Types
There are 7 Primitive Data Types in JavaScript, they are:
Numbers: These are just as you think, Digits both negative and positive digits, Numbers contain Integers (Whole Numbers), Floats (Decimal Numbers), NaN(Not a Number), and Infinity, all JavaScript numbers are stored as floating Numbers, and they can be written with or without decimals.
The memory limit for Numbers is 9,007,199,254,740,991 bits.
E.g:
let userAge = 28; let accountBalance = Infinity; let numberOfEggs = -Infinity; let numberOfBicycles = NaN; let weightOfPap = 200.25;
BigInt: It is a New Data Type used to store numbers (positive or negative) that exceed the 9,007,199,254,740,991 bits limit. Similar to numbers, it is stored in a 64-bit floating-point format.
Strings: These are a string of characters; they are identified using Single and Double Quotation marks.
let userAddress = 'M.I.B Plaza'; let userEmail = "yvonnaderogba18@gmail.com" console.log(typeof(userAddress))//This prints out the datatype of the variable in the console console.log(typeof(userEmail))Boolean:
Boolean: The Boolean type has only two values: true and false, with true meaning yes and false meaning no.
It is very useful in creating authentication processes.
console.log(true||true) console.log(true||false) console.log(false||true) console.log(false||false) //AND console.log(true&&true) console.log(false&&true) console.log(true&&false) console.log(false&&false)
Undefined: Undefined is the datatype of a variable that has only been declared but not assigned to any value
let gender; console.log(typeof(gender))
Null: Null is a datatype for a variable assigned to the value 'Null'; in this case, even if the variable is declared with the 'let' keyword, the variable cannot be assigned any other value in the program.
let age = null; console.log(typeof(age))
Object and Symbols:
The object data type is the first Non-Primitive Data Type in JavaScript,
it is a class that contains all the data structures/Component data types.
It holds multiple values of a particular entity; as an example, a class can be a classroom of students with each student being an object that has a name, age, gender, religion, etc.
The typeof method reveals the below array as an Object.
To learn more about Data Structures read Data Structures in JavaScript – With Code Examples (freecodecamp.org)
let fruits=["Papaya", "Mango", "Apple", "Watermelon", "Strawberry"] console.log(fruits) console.log(typeof(fruits))
The symbol type is used to create unique identifiers for objects. It is mentioned here for completeness, but it will be expanded on later.
In Summary; JavaScript has 7 Primitive Data Types: number, BigInt, Boolean, undefined, null, string, symbol, and 1 complex data type: object.
Subscribe to my newsletter
Read articles from Yvonne Aderogba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
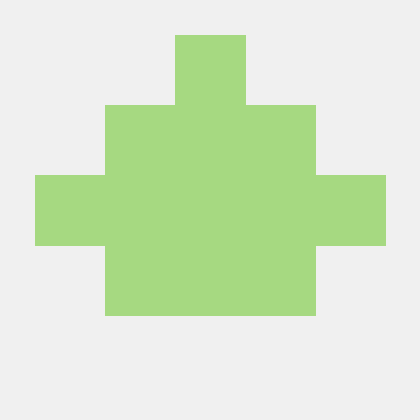