For Loops vs While Loops in Python
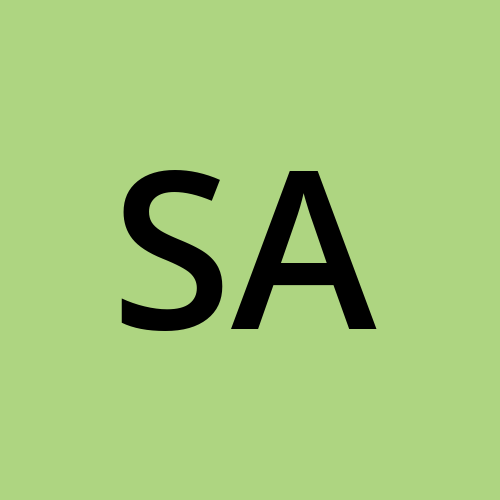
Table of contents
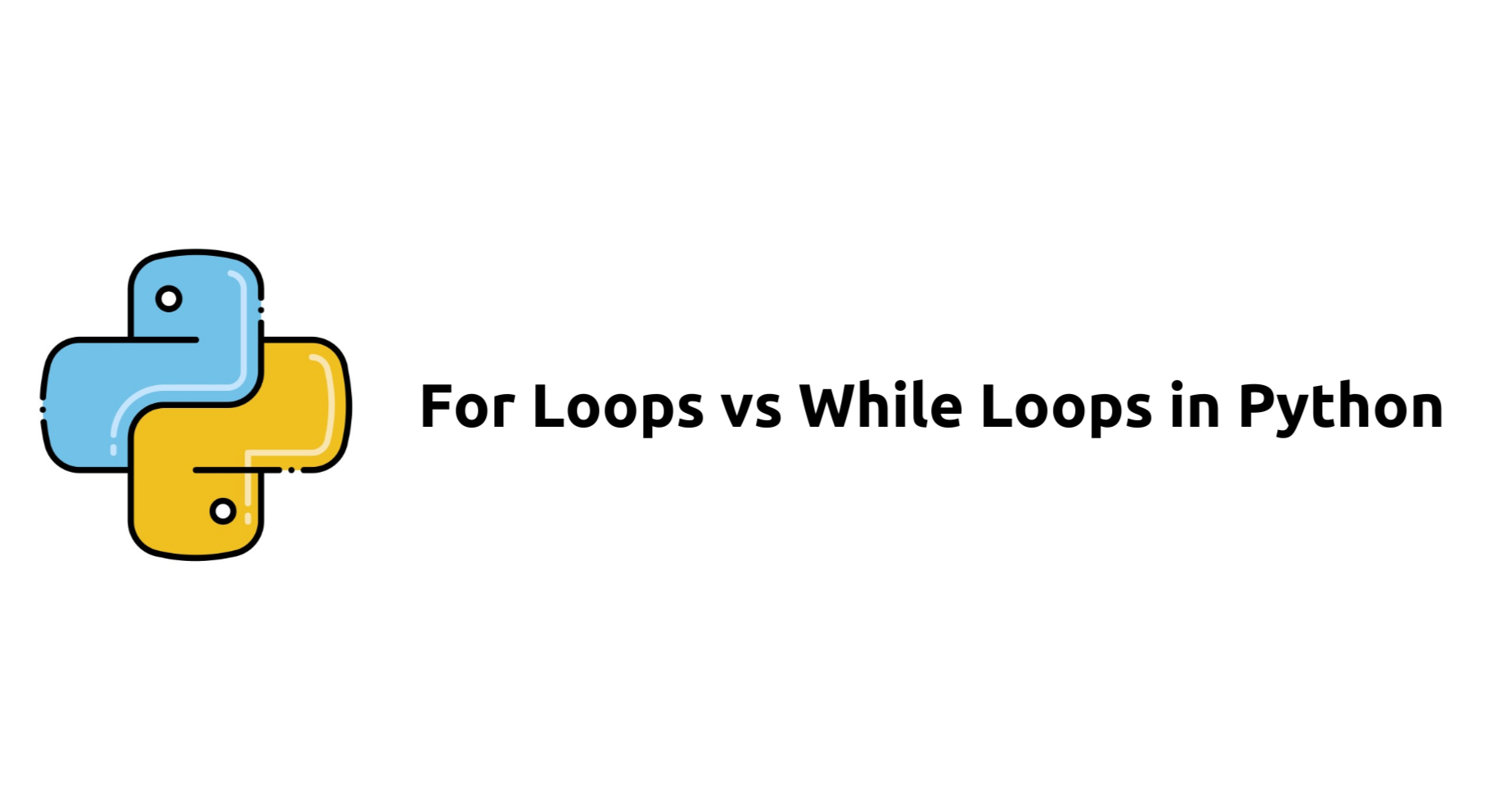
Introduction
In Python, both for loops and while loops are essential control structures used for iterating over sequences and performing repetitive tasks. While they serve similar purposes, they have distinct characteristics and are suited for different scenarios. In this article, we'll delve into the differences between for loops and while loops, discuss their unique features, and outline best practices for using each of them effectively.
Understanding For Loops and While Loops
For Loop:
Definition: A for loop is used to iterate over a sequence (such as a list, tuple, or string) and execute a block of code for each item in the sequence.
Syntax:
for item in sequence: # Execute code block for each item
Example:
for num in range(1, 6): print(num)
While Loop:
Definition: A while loop is used to repeatedly execute a block of code as long as a specified condition evaluates to true.
Syntax:
while condition: # Execute code block as long as condition is true
Example:
num = 1 while num <= 5: print(num) num += 1
Differences Between For Loops and While Loops
Control Flow:
For Loop: The number of iterations in a for loop is determined by the number of elements in the sequence.
While Loop: The number of iterations in a while loop depends on the condition specified, which may vary dynamically.
Initialization and Increment:
For Loop: The iteration variable is automatically initialized and incremented based on the sequence.
While Loop: The iteration variable must be initialized and incremented manually within the loop.
Use Cases:
For Loop: Ideal for iterating over known sequences or collections, such as lists or tuples.
While Loop: Suitable for situations where the number of iterations is not predetermined and depends on changing conditions.
Loop Termination:
For Loop: Terminates automatically when all elements in the sequence have been processed.
While Loop: Requires an explicit condition to terminate the loop, which must be carefully managed to avoid infinite loops.
Best Practices for For Loops and While Loops
For Loops:
Use for loops when iterating over known sequences or collections.
Avoid modifying the sequence being iterated over within the loop to prevent unexpected behavior.
Leverage built-in functions like
enumerate()
orzip()
for enhanced functionality and readability.
While Loops:
Use while loops when the number of iterations is not predetermined and depends on dynamic conditions.
Ensure that the loop condition eventually becomes false to prevent infinite loops and potential system crashes.
Include explicit initialization and incrementation of iteration variables within the loop to control loop behavior effectively.
Conclusion
For loops and while loops are fundamental control structures in Python, each offering unique features and advantages for iterating and performing repetitive tasks. By understanding the differences between for loops and while loops and adhering to best practices for their usage, Python developers and DevOps engineers can optimize their code, enhance readability, and ensure efficient execution of automation scripts. Whether iterating over known sequences with for loops or dynamically managing iterations with while loops, leveraging the appropriate loop construct based on the specific requirements of the task at hand is essential for achieving robust and reliable automation solutions.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
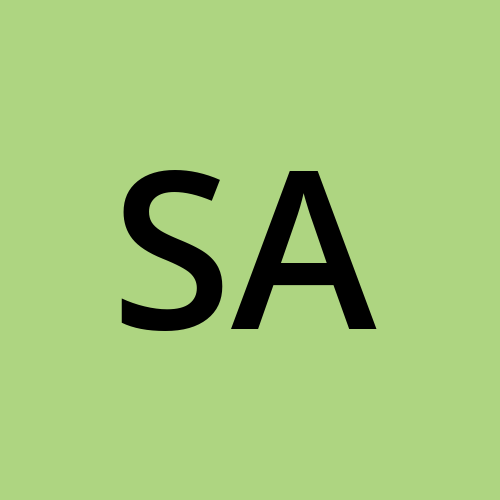
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.