Implementing Firebase Cloud Messaging (FCM) HTTP v1 in PHP/Laravel

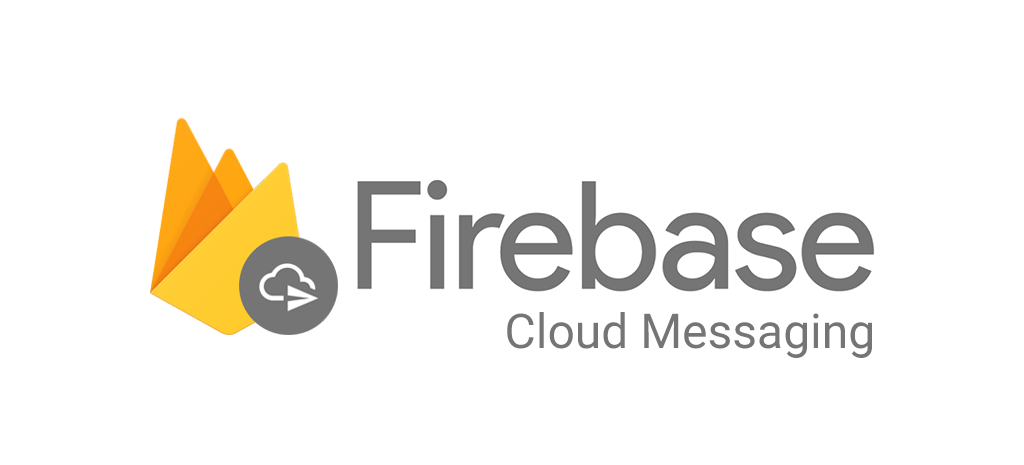
This article provides a practical guide to implementing Firebase Cloud Messaging (FCM) HTTP v1 in PHP or Laravel. With the introduction of the HTTP v1 API, FCM has enhanced security and expanded capabilities. As the legacy FCM API will be deprecated by June 20, 2024, this guide is essential for those looking to implement or migrate to the new HTTP v1 API in PHP.
Prerequisites
Service Account Key: Ensure you have a service account JSON key file for your Firebase project. You can generate this from the Firebase Console under Project Settings > Service Accounts > Generate New Private Key.
Google Client Library: Verify that the
google/apiclient
package is installed. If it's not, you can install it using Composer:composer require google/apiclient
Notification Service
<?php
namespace App\Services;
use App\Models\FcmToken;
use App\Models\Notification;
use Exception;
use Google\Client;
class NotificationService
{
public function store(array $data)
{
try {
Notification::create([
'title' => $data['title'],
'content' => $data['content'],
'user_id' => $data['user_id'],
]);
$message = [
'token' => $data['token'],
'notification' => [
'title' => $data['title'],
'body' => $data['content'],
],
'data' => $data['additional'],
];
$token = $this->getAccessToken(storage_path('YOUR_SERVICE_ACCOUNT_KEY_JSON_PATH'));
$this->sendMessage($token, 'YOUR_PROJECT_ID', $message);
return true;
} catch (\Throwable $th) {
throw $th;
}
}
function getAccessToken($serviceAccountPath)
{
$client = new Client();
$client->setAuthConfig($serviceAccountPath);
$client->addScope('https://www.googleapis.com/auth/firebase.messaging');
$client->useApplicationDefaultCredentials();
$token = $client->fetchAccessTokenWithAssertion();
return $token['access_token'];
}
function sendMessage($accessToken, $projectId, $message = [])
{
$url = 'https://fcm.googleapis.com/v1/projects/' . $projectId . '/messages:send';
$headers = [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json',
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode(['message' => $message]));
$response = curl_exec($ch);
if ($response === false) {
throw new Exception('Curl error: ' . curl_error($ch));
}
curl_close($ch);
return json_decode($response, true);
}
}
The NotificationService
class is responsible for storing notifications in the database and sending them to a user's device using Firebase Cloud Messaging (FCM).
Storing the Notification: The
store
method first creates a new notification record in the database using theNotification
model. It stores thetitle
,content
, anduser_id
fields from the$data
array.Preparing the FCM Message: The method then constructs an
$message
array, which includes the recipient device's FCMtoken
, anotification
an array containing thetitle
andbody
, and any additional data.Getting the Access Token: To send the message through FCM, the method calls
getAccessToken
, passing the path to the Firebase service account JSON file. This method uses Google'sClient
class to authenticate with Firebase, add the necessary scope for Firebase Messaging, and fetch an access token.Sending the FCM Message: With the access token retrieved, the
sendMessage
method is called to send the message. It constructs the API request usingcURL
, sets the required headers (including the authorization token), and sends the message to FCM via a POST request. If the request is successful, the response from FCM is decoded and returned.Error Handling: The entire
store
method is wrapped in atry-catch
block to handle any potential errors that may occur during the notification creation or sending process. If an error occurs, it is re-thrown for further handling.
Subscribe to my newsletter
Read articles from Md Muhaiminul Islam Shihab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Md Muhaiminul Islam Shihab
Md Muhaiminul Islam Shihab
My name is Md Muhaiminul Islam Shihab, I'm a passionate software developer specializing in HTML, CSS, Bootstrap, Tailwind CSS, jQuery, JavaScript, Vue.js, Nuxt.js, React.js, Next.js, React Native, PHP, Laravel, Livewire, Filament PHP, MySQL, and PostgreSQL. With over three years of dedicated expertise, I have worked in various fields, including web design and development, software development, and database design and optimization. I am always excited to explore new tools and technologies.