Securing Third-Party Integrations in ASP.NET Core Web APIs: OAuth, OpenID Connect, and API Keys
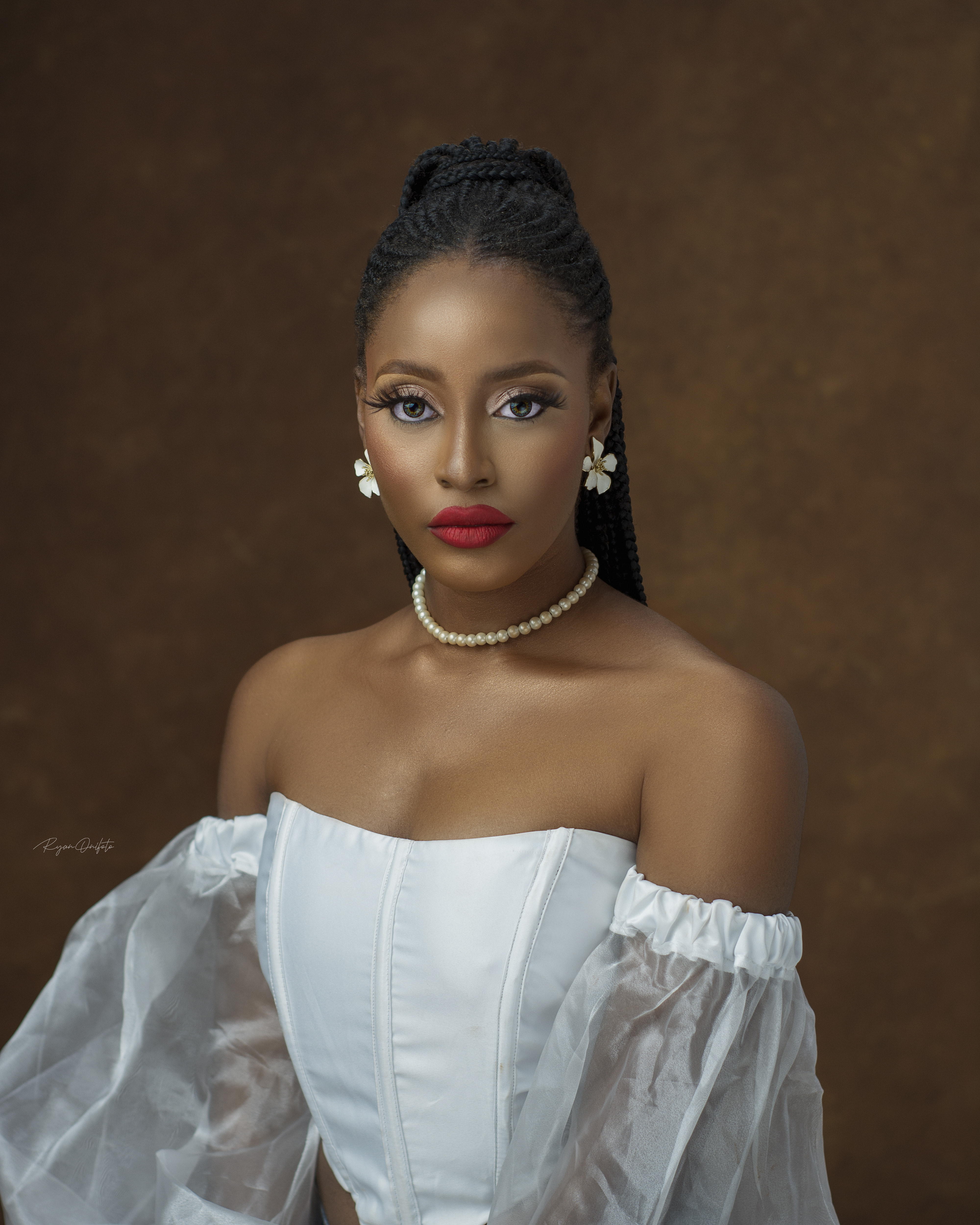
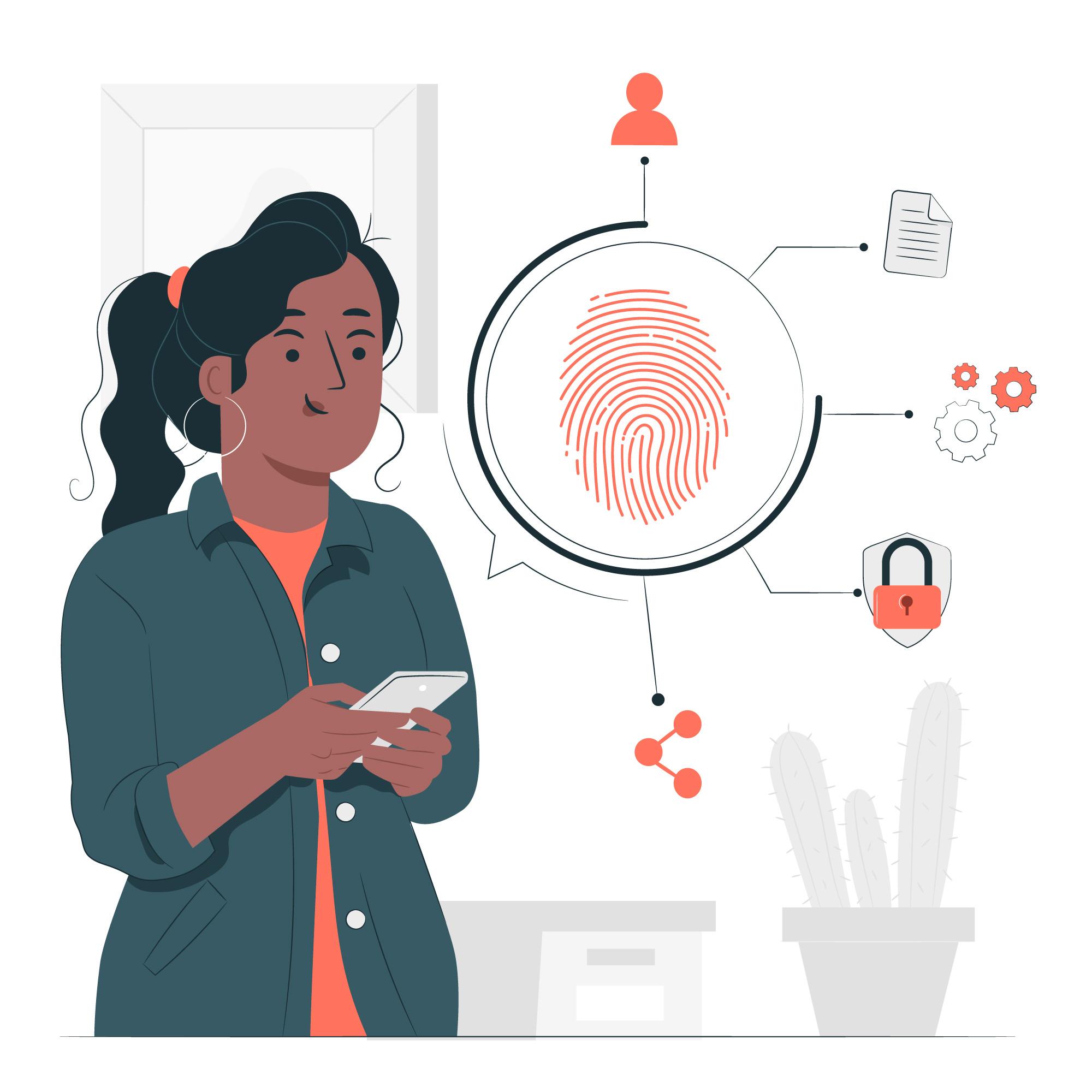
Welcome to the 15th Installation of the Mastering C# Series! We've come a long way, and I'm excited to continue this journey with you. This series has been all about helping beginners master C#, and today's tutorial is no different.
We’re diving into Securing Third-Party Integrations in ASP.NET Core Web APIs. When integrating with third-party services, you're opening your application to external systems, making it crucial to secure these connections to protect your users' data and keep your application safe from potential threats.
Prerequisites
Before diving into this tutorial, it's essential to have some foundational knowledge to make the most of what we'll cover:
Basic Knowledge of ASP.NET Core:
You should understand the basics of ASP.NET Core Web API development, including how to create and configure a simple API.Familiarity with Authentication and Authorization:
A basic understanding of what authentication and authorization are, along with common mechanisms like OAuth, OpenID Connect, and API keys.Experience with RESTful APIs:
Ability to create and consume RESTful APIs, including making requests and handling responses.Development Environment Setup:
Ensure you have Visual Studio or Visual Studio Code with the .NET Core SDK installed.Basic Understanding of JSON and HTTP:
Knowing how to work with JSON data and understanding HTTP methods (GET, POST, PUT, DELETE) will be helpful.(Optional) Familiarity with Postman:
Experience using tools like Postman to test API endpoints.(Optional) Understanding of Dependency Injection:
Basic knowledge of how dependency injection works in ASP.NET Core.
Table of Contents
Introduction to Third-Party Integrations
Integrating with External Authentication Providers
Securing API Endpoints
Best Practices for Managing Secrets and Credentials
Testing and Debugging Secure Integrations
Conclusion
Introduction to Third-Party Integrations
What Are Third-Party Integrations in Web APIs? Third-party integrations enable your web API to interact with other services or applications. For instance, you might allow users to log in with their Google or Facebook accounts, or integrate with a payment gateway like PayPal to handle transactions. These are typical examples of third-party integrations.
Why Is Security Important in Third-Party Integrations? When you connect your application to external services, you're exposing it to potential risks. Ensuring these connections are secure is critical to protect your users' data and prevent unauthorized access. By focusing on security, you maintain the integrity and trustworthiness of your application.
Integrating with External Authentication Providers
Understanding OAuth and OpenID Connect
OAuth:
OAuth is a protocol that allows users to grant websites or applications access to their information on other websites without sharing their passwords. It's like using a "Sign in with Google" button on a website.OpenID Connect:
This protocol builds on OAuth and adds an identity layer. It not only allows users to log in but also securely verifies their identity.
Step-by-Step Guide to Implementing OAuth in ASP.NET Core
Step 1: Set Up a New ASP.NET Core Project
Start by creating a new ASP.NET Core Web API project:
dotnet new webapi -n ThirdPartyIntegrationDemo
Step 2: Install the Required Packages
Use NuGet to install the OAuth and OpenID Connect packages:
dotnet add package Microsoft.AspNetCore.Authentication.OAuth
dotnet add package Microsoft.AspNetCore.Authentication.OpenIdConnect
Step 3: Configure OAuth in Your Project
In your Startup.cs
, configure the OAuth authentication:
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "Google";
})
.AddCookie()
.AddGoogle("Google", options =>
{
options.ClientId = "your-client-id";
options.ClientSecret = "your-client-secret";
});
services.AddControllers();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
Step 4: Create Authentication Endpoints
Set up a controller to handle the OAuth login process:
[Route("[controller]/[action]")]
public class AccountController : Controller
{
[HttpGet]
public IActionResult Login()
{
return Challenge(new AuthenticationProperties { RedirectUri = "/" }, "Google");
}
[HttpGet]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync(CookieAuthenticationDefaults.AuthenticationScheme);
return RedirectToAction("Index", "Home");
}
}
Step 5: Test the Integration
Use a tool like Postman to initiate the OAuth login flow and verify that users can log in using their external accounts.
Implementing OpenID Connect for Secure Authentication
To implement OpenID Connect, follow similar steps but configure it in your Startup.cs
:
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = OpenIdConnectDefaults.AuthenticationScheme;
})
.AddCookie()
.AddOpenIdConnect(options =>
{
options.Authority = "https://login.microsoftonline.com/common";
options.ClientId = "your-client-id";
options.ClientSecret = "your-client-secret";
options.ResponseType = "code";
});
Securing API Endpoints
Securing your API endpoints ensures that only authorized users can access specific data or perform particular actions.
Introduction to API Keys and Access Tokens
API Keys:
An API key is a unique identifier that a user or application provides when making API requests. It's used to track and control how the API is used.Access Tokens:
Access tokens are credentials used to access protected resources. They carry more detailed information and are typically used in OAuth implementations.
How to Generate and Use API Keys in ASP.NET Core
Step 1: Generate API Keys
Generate an API key and store it securely, typically in a database:
public string GenerateApiKey()
{
using (var rng = new RNGCryptoServiceProvider())
{
var byteToken = new byte[32];
rng.GetBytes(byteToken);
return Convert.ToBase64String(byteToken);
}
}
Step 2: Validate API Keys
In your API, validate incoming requests by checking the API key:
public async Task<IActionResult> GetData([FromHeader(Name = "ApiKey")] string apiKey)
{
if (!IsValidApiKey(apiKey))
{
return Unauthorized();
}
return Ok(new { Data = "Here is your data!" });
}
private bool IsValidApiKey(string apiKey)
{
// Logic to check the API key against the stored keys
return true;
}
Validating Access Tokens in Your API Endpoints
Use middleware in ASP.NET Core to validate access tokens automatically:
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.Authority = "https://your-authorization-server.com";
options.Audience = "your-api";
});
Best Practices for Managing Secrets and Credentials
Securely Storing Secrets in ASP.NET Core
Store sensitive information in appsettings.json
or environment variables, not in your code:
{
"ConnectionStrings": {
"DefaultConnection": "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;"
}
}
Using Secret Management Tools
Consider using Azure Key Vault, AWS Secrets Manager, or other secret management tools to handle your sensitive data securely.
Environment-Specific Configurations
Configure different environments with specific settings, ensuring that sensitive data is only available where it’s needed.
Testing and Debugging Secure Integrations
Tools for Testing OAuth and API Keys
Use tools like Postman to test your API endpoints with OAuth tokens or API keys:
Create a new request in Postman.
Add your OAuth token or API key to the request headers.
Send the request and verify the response.
Common Pitfalls and How to Avoid Them
Issues like expired tokens, incorrect API keys, or misconfigurations are common. Always ensure your configurations are correct and that tokens are up-to-date.
Conclusion
Let's quickly recap:
Recap of Key Concepts:
We’ve explored securing your ASP.NET Core Web APIs through OAuth, OpenID Connect, API keys, and access tokens. We also covered best practices for managing secrets and credentials securely.Additional Resources for Further Learning:
Check out the official ASP.NET Core documentation for more on authentication and authorization, as well as tutorials and articles on API security best practices.
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the Mastering C# series: Securing Data Storage in ASP.NET Core Web APIs: Encryption, Key Management, and Data Masking.
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
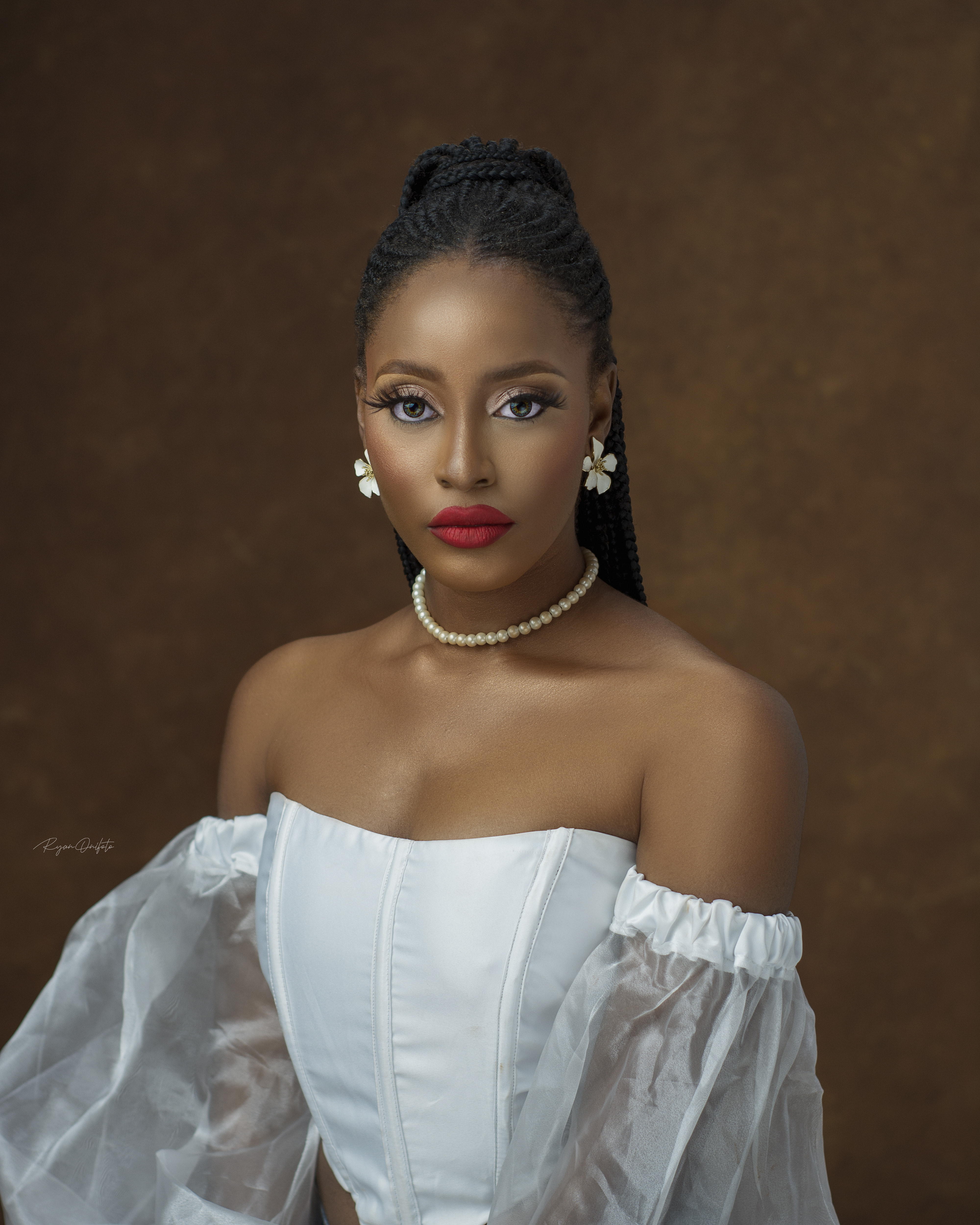