MongoDB Aggregation Pipeline Explained: Best Practices and Use Cases
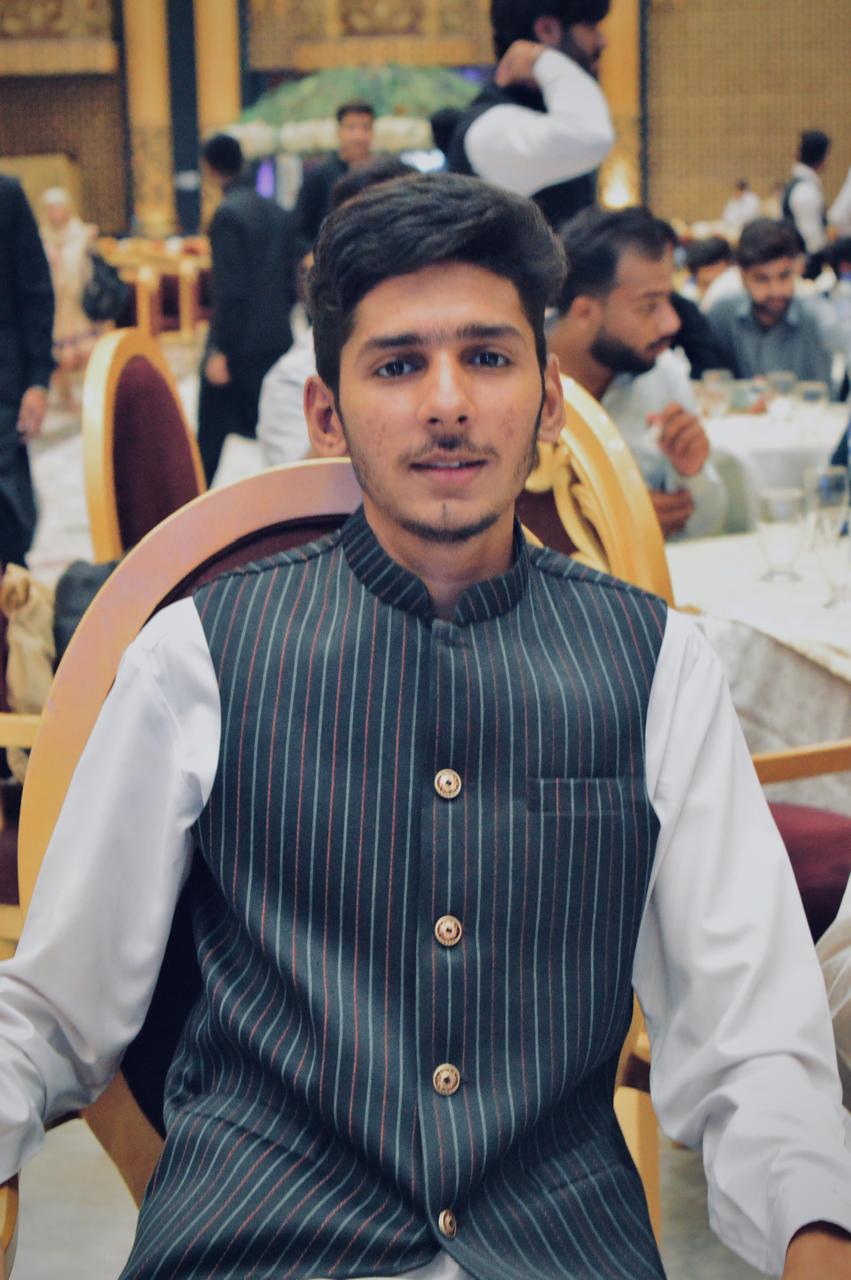
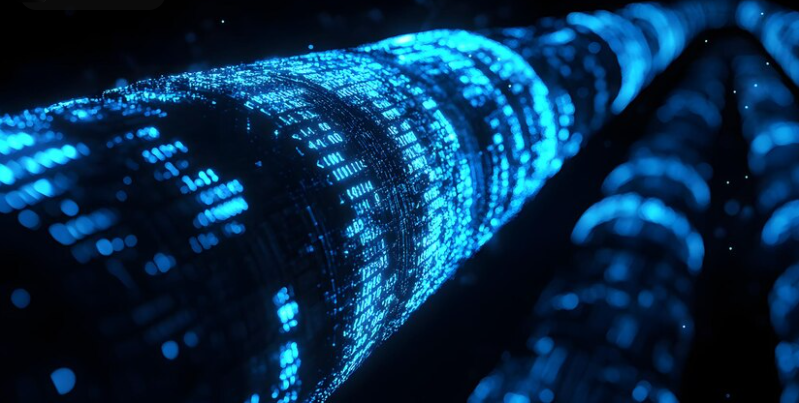
MongoDB Aggregation Pipeline Explained
MongoDB’s aggregation pipeline is a powerful framework used to perform complex data processing tasks. It enables you to transform and analyze data within MongoDB collections by applying a series of operations, or "stages," to the documents in a collection. Each stage in the pipeline processes the documents and passes the results to the next stage, allowing for multi-step data transformations and aggregations.
Key Components of Aggregation Pipeline
Pipeline Stages:
$match: Filters documents based on specified criteria. Similar to querying with
find()
, but applied within the pipeline to narrow down the data set before further processing.$group: Groups documents by a specified field and performs aggregate operations like sum, average, count, etc., on grouped data.
$sort: Orders documents based on specified fields, which is useful for organizing data before or after aggregation.
$project: Reshapes each document by including or excluding fields, or creating new computed fields.
$lookup: Performs a left outer join with another collection, allowing you to combine documents from multiple collections.
$unwind: Deconstructs an array field from the documents, creating separate documents for each element in the array.
$limit: Limits the number of documents passed to the next stage in the pipeline.
$skip: Skips a specified number of documents, useful for pagination.
Use Cases for MongoDB Aggregation Pipeline
Data Aggregation and Reporting:
Example: Calculate total sales, average order value, or sales by region.
Use Case: E-commerce platforms often need to generate sales reports, analyze customer spending patterns, and identify high-performing products.
Data Transformation:
Example: Reshape data for reporting or visualization.
Use Case: Transform data from a raw format into a format suitable for charts and graphs in business intelligence dashboards.
Real-Time Analytics:
Example: Analyze user activity logs to provide real-time insights.
Use Case: Monitor and analyze user behavior on a website to make real-time adjustments or provide immediate feedback.
Data Enrichment:
Example: Combine data from multiple collections to provide more comprehensive results.
Use Case: Use
$lookup
to join user profiles with their activity logs to generate detailed user reports.
Data Filtering and Cleaning:
Example: Remove duplicate records or filter out irrelevant data.
Use Case: Clean and preprocess data before performing deeper analysis or generating reports.
Complex Aggregations:
Example: Perform multi-stage aggregations that involve grouping, sorting, and filtering data.
Use Case: Complex analytics where you need to calculate metrics across different dimensions and time periods.
Let's dive into some code examples.
import mongoose, { Schema } from "mongoose";
const subscriptionSchema= new mongoose.Schema(
{
subscriber:{
tyoe: Schema.Types.ObjectId,
ref:'User',
},
channel:{
tyoe: Schema.Types.ObjectId,
ref:'User',
}
}
,{timestamps : true}
);
export const SubScription = mongoose.model("Subscription",subscriptionSchema);
This is simple MongoDB Scheme Model
const channel = await User.aggregate([
{
$match:{username: username.toLowerCase()}
},
{
$lookup:{
from : "subscriptions",
localField : "_id",
foreignField:"channel",
as:"subscribers"
}
},
{
$lookup:{
from : "subscriptions",
localField : "_id",
foreignField:"subscriber",
as:"SubscribedTo"
}
},
{
$addFields:{
subscriberCout:{
$size:"$subscribers"
},
subscribedToCount:{
$size:"$SubscribedTo"
},
isSubscribed:{
$cond:{
if:{$in:[req.user._id,"$subscribers.subscriber"]},
then:true,
else:false
}
}
}
},
{
$project:{ //give Project to send particular data
fullname:1,
username:1,
subscriberCout:1,
subscribedToCount:1,
isSubscribed:1,
avatar:1,
coverImage:1,
email:1,
}
}
])
Start with your model name and use the Aggregate function.
Aggregation Pipeline Stages:
$match:
{ $match: { username: username.toLowerCase() } }
Purpose: Filters the documents in the
User
collection to find the user whoseusername
matches the providedusername
, converted to lowercase.Result: This stage isolates the specific user document(s) that will be processed in the subsequent stages.
$lookup (First Instance):
{ $lookup: { from: "subscriptions", localField: "_id", foreignField: "channel", as: "subscribers" } }
Purpose: Performs a left outer join with the
subscriptions
collection. It matches theUser
document’s_id
with thechannel
field in thesubscriptions
collection.Result: Adds a new field called
subscribers
to the user document, containing an array of subscription documents where the user is a channel.
$lookup (Second Instance):
{ $lookup: { from: "subscriptions", localField: "_id", foreignField: "subscriber", as: "SubscribedTo" } }
Purpose: Performs another left outer join with the
subscriptions
collection. This time, it matches theUser
document’s_id
with thesubscriber
field in thesubscriptions
collection.Result: Adds a new field called
SubscribedTo
to the user document, containing an array of subscription documents where the user is a subscriber.
$addFields:
{ $addFields: { subscriberCount: { $size: "$subscribers" }, subscribedToCount: { $size: "$SubscribedTo" }, isSubscribed: { $cond: { if: { $in: [req.user._id, "$subscribers.subscriber"] }, then: true, else: false } } } }
Purpose: Adds new fields to the user document:
subscriberCount
: Counts the number of subscribers by calculating the size of thesubscribers
array.subscribedToCount
: Counts the number of channels the user is subscribed to by calculating the size of theSubscribedTo
array.isSubscribed
: Determines if the current user (fromreq.user._id
) is subscribed to the user’s channel. If the user’s ID is found in thesubscribers.subscriber
array,isSubscribed
is set totrue
; otherwise, it is set tofalse
.
$project:
{ $project: { fullname: 1, username: 1, subscriberCount: 1, subscribedToCount: 1, isSubscribed: 1, avatar: 1, coverImage: 1, email: 1 } }
Purpose: Specifies which fields to include in the output documents.
Result: The resulting documents will include the fields
fullname
,username
,subscriberCount
,subscribedToCount
,isSubscribed
,avatar
,coverImage
, andemail
, while excluding any other fields.
What the Code is Doing
Finds a Specific User: It filters the
User
collection to find a user byusername
.Gathers Subscription Data:
Retrieves the list of subscribers for the user (i.e., users who have subscribed to this user’s channel).
Retrieves the list of channels the user is subscribed to (i.e., channels this user has subscribed to).
Calculates and Adds Metrics:
Calculates how many users are subscribed to this user’s channel (
subscriberCount
).Calculates how many channels this user is subscribed to (
subscribedToCount
).Checks if the current user is among the subscribers of this user’s channel (
isSubscribed
).
Projects Relevant Data:
- Outputs a document with the user’s basic information and the calculated metrics.
This pipeline provides a comprehensive view of a user’s subscription statistics and relationship status, enriching the user document with relevant subscription information.
Subscribe to my newsletter
Read articles from Abdullah ata directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
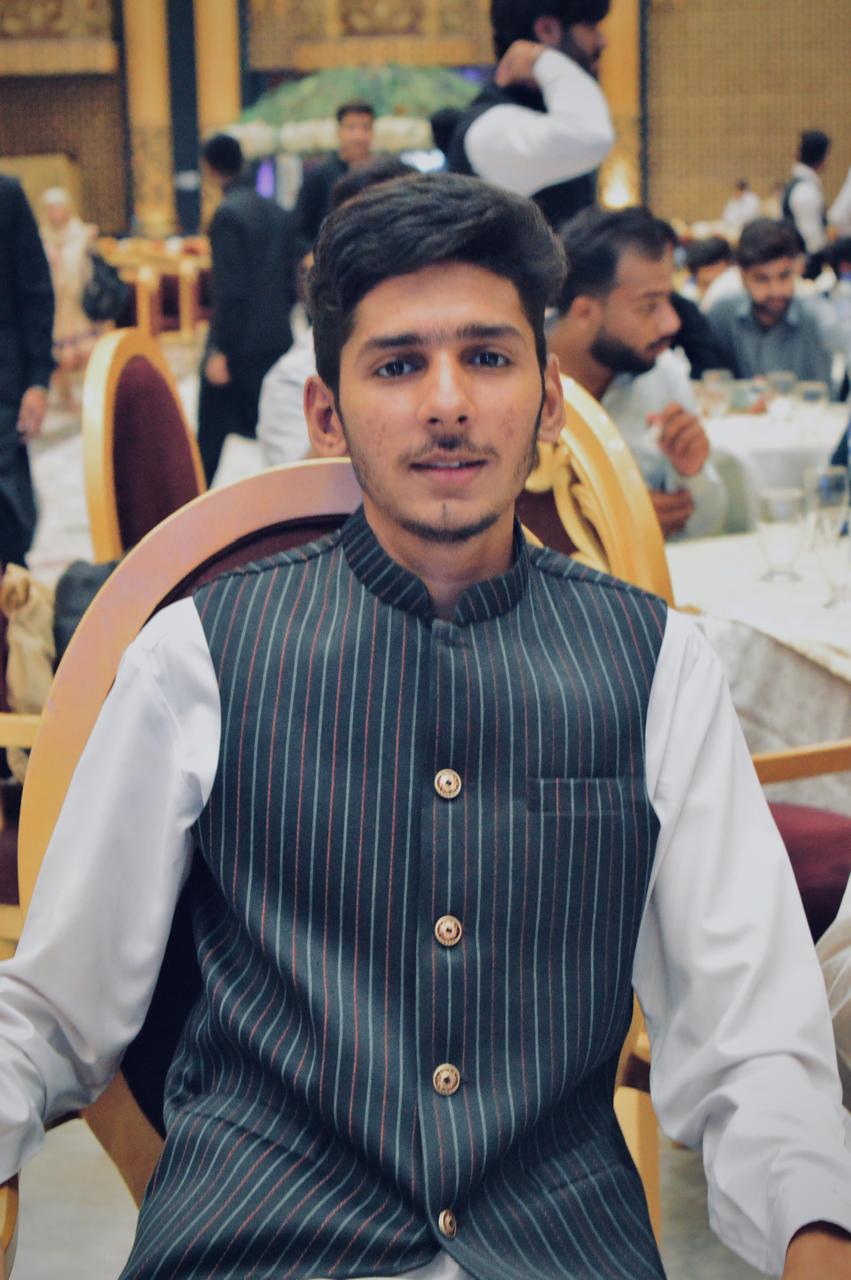
Abdullah ata
Abdullah ata
👋 Hi there! I’m a passionate developer from Lahore, Punjab, Pakistan, Hand in the MERN stack. With a knack for creating dynamic and responsive web applications, I love turning ideas into reality through code.