Building a Simple Language Translator with Python and the GhanaNLP API
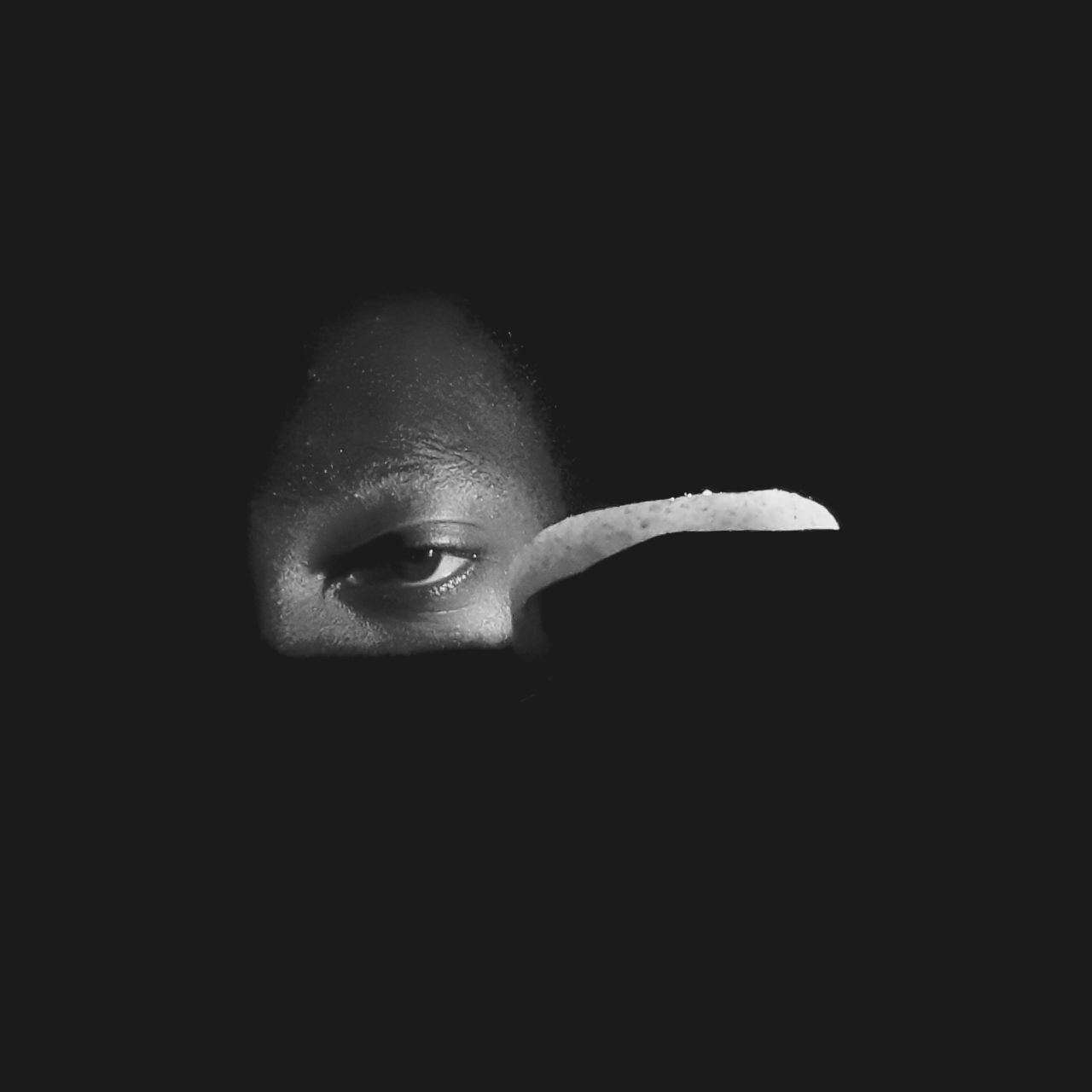
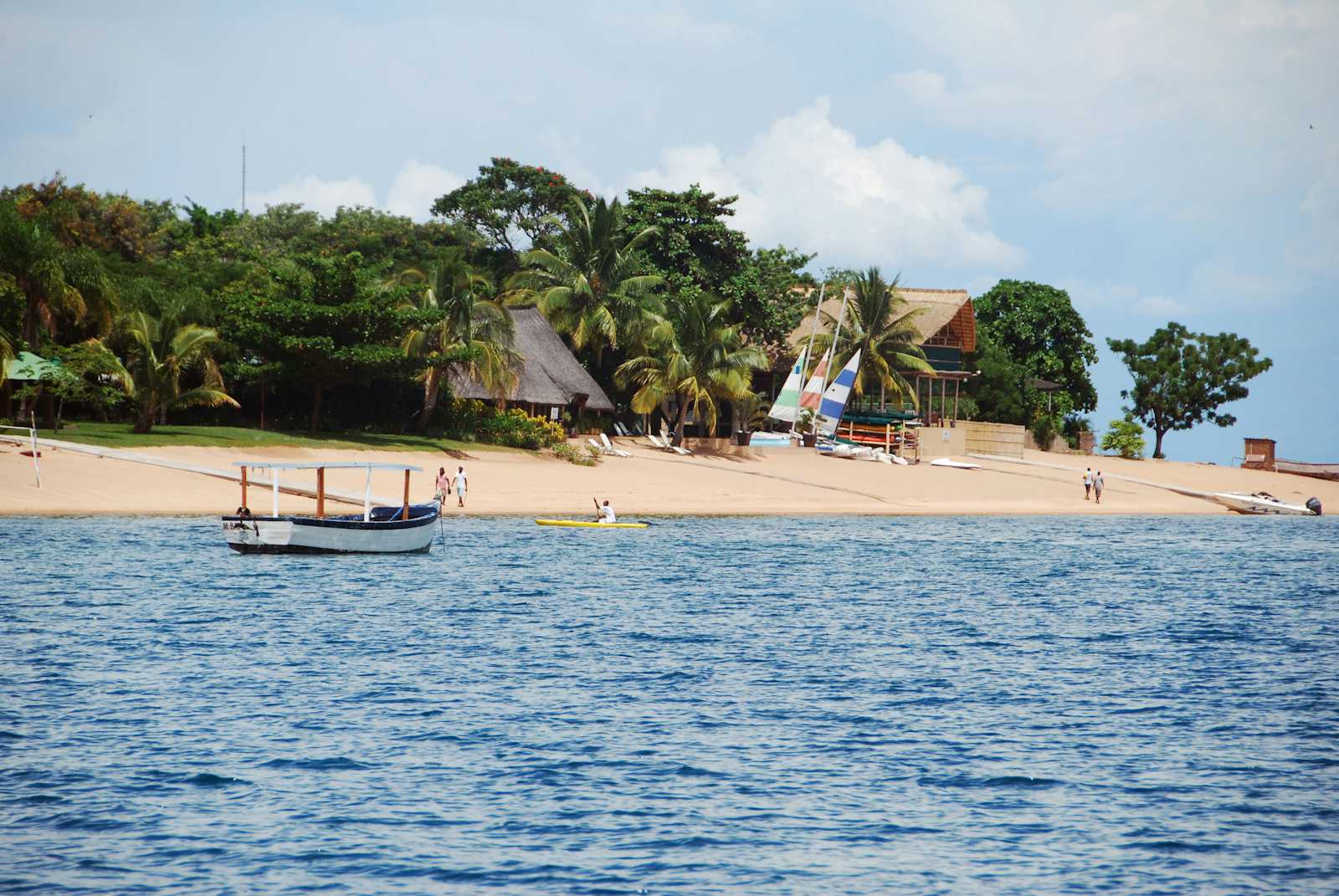
In this blog post, I’ll show you how to build a simple translator using Python to make requests to the GhanaNLP Translation API. This is the first of a series where I’ll be exploring practical ways to integrate some African languages into scalable mini projects.
Why Build This? 🌟
The goal here is to create a simple translator that lets users translate text in real time. For this project, we’ll focus on translating between English and Twi, one of Ghana’s most widely spoken languages.
But don’t worry, the API supports many language pairs, so you can have fun experimenting with others. It's all a learning experience!
What You'll Need!
Before diving into the python script, make sure you have:
Python 3.9+ installed.
Read my introductory blogs to on getting started. In a hurry? Request an API key from GhanaNLP (contact natural.language.proccessing.gh@gmail.com for access).
The
requests
library installed. If you don’t have it yet, you can install it via:pip install requests
How to check for installed libraries? Type in your terminal/command line:
pip list
Setting Up the Translator
This is the python script to perform language translation:
https://github.com/PhidLarkson/ghananlp-translate-python
import requests #the requests import
# a translate function to take the text to be translated and the preferred language
def translate(text, target_language):
url = "https://translation-api.ghananlp.org/v1/translate" # the requests url
headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'Ocp-Apim-Subscription-Key': '******************', # put your API key here
}
data = {
'in': text,
'lang': target_language
}
response = requests.post(url, headers=headers, json=data)
if response.status_code == 200:
return response.json()
else:
return "An error occurred"
# pick a language of your choice
language_pair = "en-tw"
# start
print(f"Welcome to this simple {language_pair.upper()} translator program")
"""
The language pair from the English language to Twi is en-tw.
language.upper() in the print statement capitalises the characters the
language pair variable holds
"""
# while loop
while True:
try:
# message input in English
message = input("Type what you want to translate: ")
translation = translate(message, language_pair)
except Exception as e:
print(f"An error occurred: {e}")
break
print(f"Translation to Twi:{translation}\n")
Walkthrough
Oh hi! I am glad you didn't just copy, paste, and disappear. Awesome! I guess you're ready to dive in and understand what's happening.
How do we start?
Make sure to import the requests library.
The function
translate(text, target_language)
: This takes care of sending the request to the GhanaNLP API and gives back the translated text.The API endpoint is
https://translation-api.ghananlp.org/v1/translate
.The headers include the content type and your API key.
The data payload contains the input text (
in
) and the target language (lang
).
Handling the Response: The response is a JSON object. If the request is successful (status code 200), the translated text is extracted and returned. If not, an error message is shown.
User Input Loop: The program keeps asking the user for input until an error happens or they decide to exit. The text is translated based on the chosen language pair (
en-tw
in this example) and printed to the console.Error Handling: If there’s a problem with the input or the API request, the program handles the error nicely and stops the loop.
Running the Translator
To run the translator:
Replace
'YOUR API KEY'
with the key you got from GhanaNLP API portal.Run the script in your terminal or any Python environment.
Start typing phrases in English, and watch them get translated to Twi!
Example output:
This script is just basic, you can integrate it in several ways: allow users to choose the language pair dynamically (e.g., English to Ga, English to Ewe), implement a GUI using Tkinter or PyQt for a more interactive experience, and add error messages for common issues like unsupported language codes or empty inputs. You think it, you build it.
Whatever you end up working on from here, feel free to send it to me to check out. You can email me at phiddyconcept@gmail.com.
Happy coding!
Subscribe to my newsletter
Read articles from Prince Larbi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
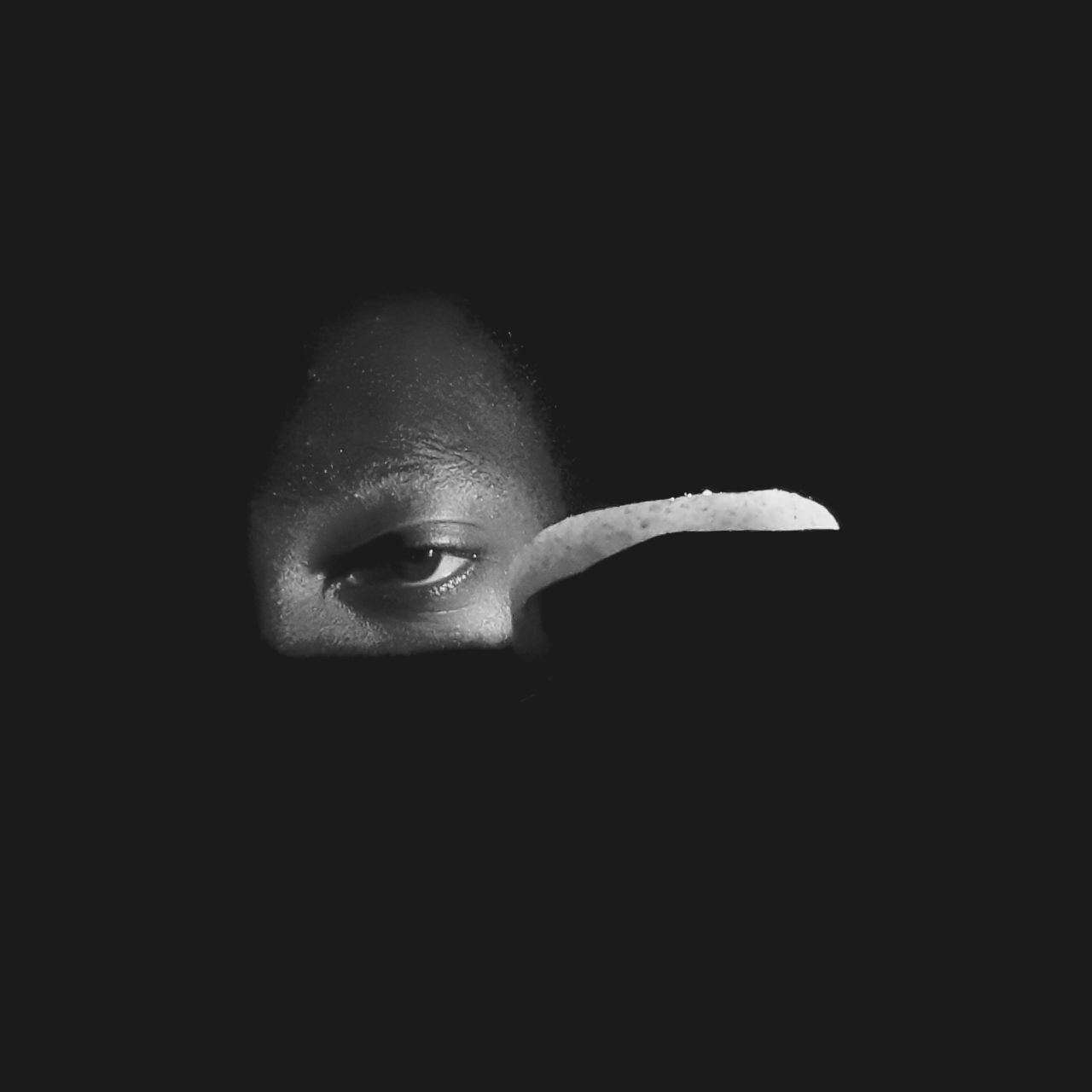
Prince Larbi
Prince Larbi
Enjoy the journey. yoghurt over coffee!! ...any day