Advanced Techniques for Implementing Modals in Web Applications
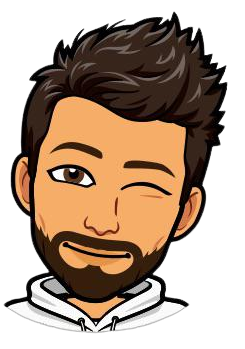
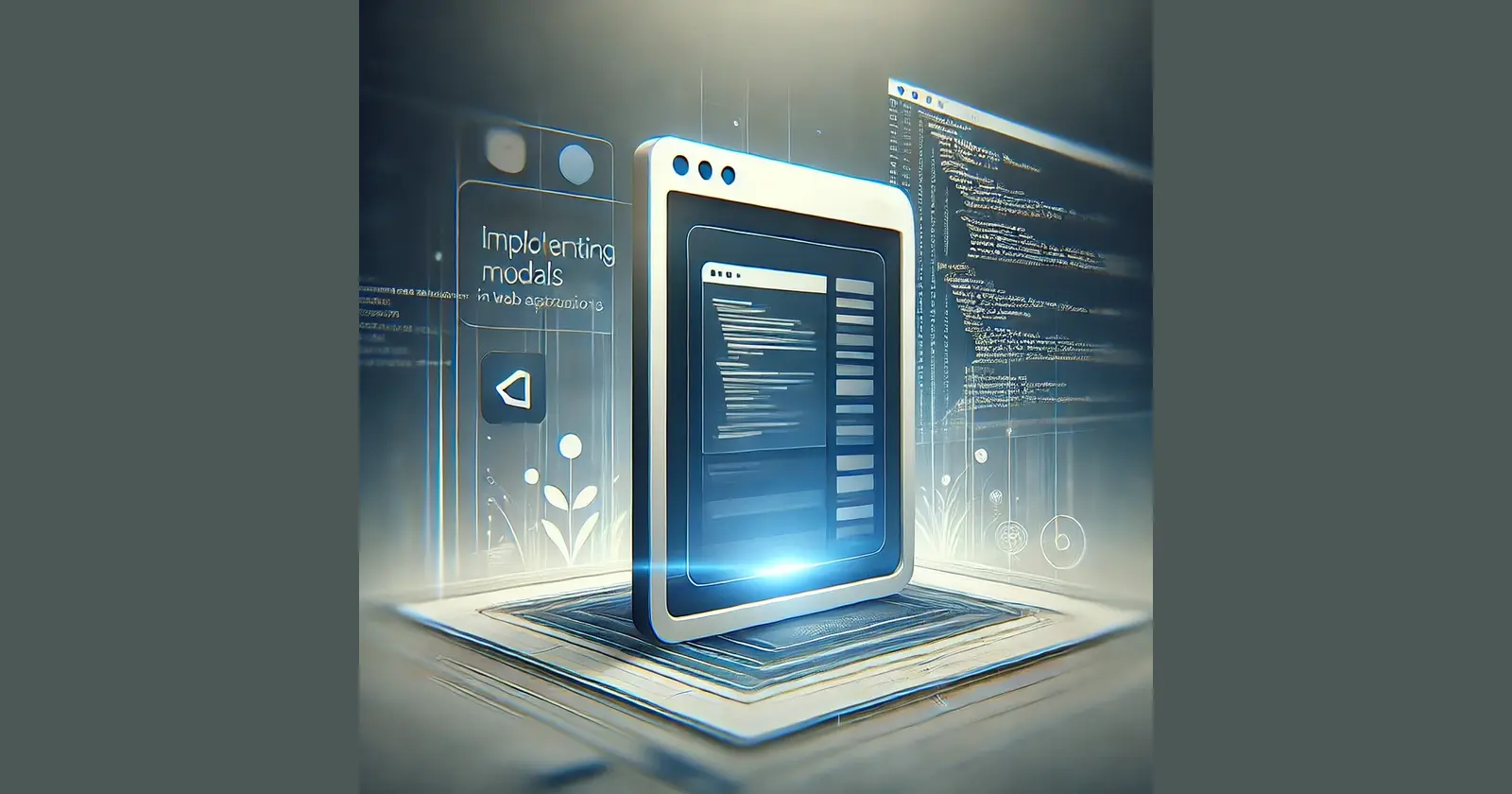
Modals are a fundamental UI component in web applications, providing an interactive way to present content, forms, or actions without navigating away from the current page. While adding a simple modal might seem straightforward, implementing them in a scalable and maintainable way—especially in large applications—requires a more sophisticated approach.
In this article, we’ll explore three advanced methods for implementing modals, each designed to handle different use cases and provide maximum flexibility. By the end of this guide, you’ll know how to implement modals like a pro, ensuring your code is clean, decoupled, and ready for production-level challenges.
Method 1: Conditional Rendering with Component State
The Basics
The most straightforward way to implement a modal is by creating a modal component and conditionally rendering it based on a state variable. This method is widely used and works well for simple cases.
Implementation
import React, { useState } from 'react';
const SimpleModal = ({ isOpen, onClose }) => {
if (!isOpen) return null;
return (
<div className="modal-overlay">
<div className="modal-content">
<button onClick={onClose}>Close</button>
<p>This is a simple modal.</p>
</div>
</div>
);
};
const ParentComponent = () => {
const [isModalOpen, setIsModalOpen] = useState(false);
return (
<div>
<button onClick={() => setIsModalOpen(true)}>Open Modal</button>
<SimpleModal isOpen={isModalOpen} onClose={() => setIsModalOpen(false)} />
</div>
);
};
Pros:
Simple and easy to implement.
No external dependencies.
Cons:
Tight coupling: The modal is directly tied to the parent component's state, making it less reusable.
Limited flexibility: If you need to open the modal from another part of the application, you have to pass the state down through props or use context, which can become cumbersome.
Method 2: URL-Based Modals with Query Parameters
The Basics
A more advanced approach involves using query parameters to control whether a modal is open. This method decouples the modal’s state from the component that triggers it, making it easier to manage and more flexible across the application.
Implementation
import React, { useEffect, useState } from 'react';
import { useRouter } from 'next/router';
const QueryParamModal = () => {
const router = useRouter();
const [isOpen, setIsOpen] = useState(false);
useEffect(() => {
setIsOpen(router.query.modal === 'true');
}, [router.query]);
const closeModal = () => {
router.push(router.pathname); // Remove modal query param
};
if (!isOpen) return null;
return (
<div className="modal-overlay">
<div className="modal-content">
<button onClick={closeModal}>Close</button>
<p>This modal is controlled by a query parameter.</p>
</div>
</div>
);
};
const ParentComponent = () => {
const router = useRouter();
const openModal = () => {
router.push({ query: { modal: 'true' } });
};
return (
<div>
<button onClick={openModal}>Open Modal</button>
<QueryParamModal />
</div>
);
};
Pros:
Decoupled state: The modal's visibility is controlled by the URL, making it accessible from any component in the app.
Bookmarkable and shareable: Since the modal state is in the URL, you can bookmark or share links that open the modal directly.
Cons:
Component always present: The modal component is always rendered at the root level, even if it’s not visible.
URL management: You need to manage the query parameters carefully to avoid conflicts.
Method 3: Centralized State Management with a Modal Map
The Basics
For large-scale applications, a centralized state management approach is often necessary. In this method, you maintain a store that tracks the current modal type and any associated data. This allows you to trigger modals from anywhere in the application without directly manipulating component states or URLs.
Implementation
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { setModalType } from '../store/modalActions';
const MODAL_COMPONENTS = {
'EXAMPLE_MODAL': ExampleModal,
'ANOTHER_MODAL': AnotherModal,
};
const GlobalModal = () => {
const dispatch = useDispatch();
const { modalType, modalProps } = useSelector(state => state.modal);
if (!modalType) return null;
const ModalComponent = MODAL_COMPONENTS[modalType];
return (
<div className="modal-overlay">
<ModalComponent {...modalProps} onClose={() => dispatch(setModalType(null))} />
</div>
);
};
const ParentComponent = () => {
const dispatch = useDispatch();
const openModal = (type) => {
dispatch(setModalType(type, { someProp: 'value' }));
};
return (
<div>
<button onClick={() => openModal('EXAMPLE_MODAL')}>Open Example Modal</button>
<button onClick={() => openModal('ANOTHER_MODAL')}>Open Another Modal</button>
<GlobalModal />
</div>
);
};
Pros:
Highly decoupled: The modal logic is entirely separated from the triggering components, making it easy to manage.
Scalable: This approach scales well as the number of modals increases, especially in large applications.
Flexible: You can add, remove, or update modals with minimal changes to the existing code.
Cons:
Initial setup: Setting up centralized state management and creating a modal map requires more initial effort.
Redux dependency: This example uses Redux, which adds a dependency to your project, though you could use other state management tools as well.
Conclusion
Implementing modals in a web application might seem trivial at first glance, but as your application grows, it’s crucial to adopt more sophisticated approaches. Whether you choose to go with conditional rendering, URL-based modals, or a centralized state management system, the key is to select the method that best fits your application’s needs.
In this article, we’ve covered:
Basic Conditional Rendering: Quick to implement but limited in scalability.
URL-Based Modals: Decoupled and flexible, but requires careful URL management.
Centralized State Management: Ideal for large applications with complex modal requirements.
By mastering these techniques, you can implement modals in a way that’s not only professional but also scalable and maintainable, ensuring that your application is ready for industry-level challenges.
Subscribe to my newsletter
Read articles from Shubham Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
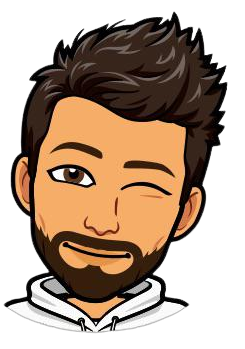
Shubham Jain
Shubham Jain
I work as an SDE-1 in frontend role at a healthcare startup. I am always curious to learn new technologies and share my learnings. I love development and love to code.