Exploring YARP: The Reverse Proxy for .NET Developers
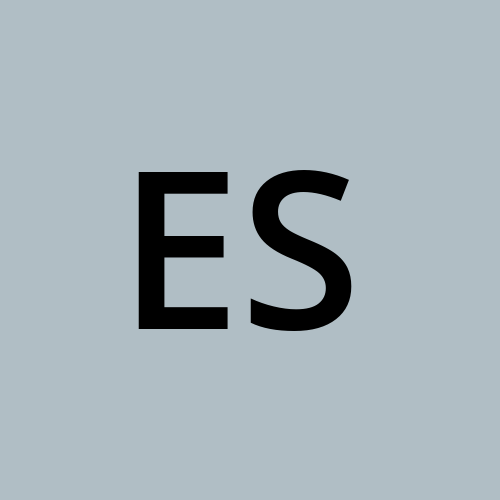
Introduction
In modern web development, as applications scale, the need for efficient routing, load balancing, and reverse proxying becomes crucial. A reverse proxy can be a game-changer, helping to manage traffic, improve performance, and add an extra layer of security to your applications. Enter YARP (Yet Another Reverse Proxy), a powerful and flexible reverse proxy library developed by Microsoft specifically for .NET developers. This article explores what YARP is, why you should consider using it, and how to get started with it in your .NET projects.
What is YARP?
YARP is a highly customizable reverse proxy toolkit for building fast and reliable proxies in .NET. Built on top of ASP.NET Core, it leverages the underlying framework's performance and scalability while providing a flexible way to define routing rules and load balancing strategies.
One of the standout features of YARP is its extensibility. Unlike traditional reverse proxies, YARP is designed to be modified and extended by developers. You can customize its behavior using .NET code, making it a perfect fit for scenarios where out-of-the-box solutions might fall short.
Why Use a Reverse Proxy?
Before diving into YARP, it’s essential to understand why you might want to use a reverse proxy in the first place. A reverse proxy sits between clients and your backend servers, forwarding client requests to the appropriate server. This setup offers several benefits:
Load Balancing: Distribute incoming traffic across multiple backend servers, ensuring no single server is overwhelmed, which leads to better performance and reliability.
Security: By routing all traffic through a reverse proxy, you can hide the identity and internal structure of your backend servers. This adds an additional security layer, as clients interact only with the proxy.
Caching: Reverse proxies can cache responses from your backend servers, reducing the load on those servers and improving response times for clients.
Routing: You can direct traffic to different backend services based on URL patterns, headers, or other criteria, allowing you to manage multiple services or microservices with a single proxy.
SSL Termination: Reverse proxies can handle SSL termination, decrypting incoming requests before forwarding them to backend services, reducing the computational load on your servers.
Getting Started with YARP
Now that we understand the benefits of using a reverse proxy, let’s see how you can implement YARP in your .NET applications.
Installation
To start using YARP in your project, you need to add the YARP NuGet package to your .NET project. You can do this via the .NET CLI or by adding the package directly through your project’s package manager.
dotnet add package Microsoft.ReverseProxy
Basic Configuration
YARP is configured primarily through your appsettings.json
file, where you define routes and clusters. A route in YARP specifies how requests should be matched and routed to a backend cluster. A cluster represents one or more destinations (servers) where the traffic will be forwarded.
Here’s a basic example of a YARP configuration in appsettings.json
:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"ReverseProxy": {
"Routes": {
"users-route": {
"ClusterId": "users-cluster",
"Match": {
"Path": "users-api/{**catch-all}"
},
"Transforms": [
{
"PathPattern": "{**catch-all}"
}
]
}
},
"Clusters": {
"users-cluster": {
"Destinations": {
"destination1": {
"Address": "https://localhost:7247/"
}
}
}
}
}
}
Understanding the Configuration
Let’s break down the configuration:
Routes: Defines how incoming requests should be routed. In the example above, we have a route named
users-route
that matches any request path starting withusers-api/
. TheClusterId
property links this route to a cluster (users-cluster
), which defines the destination servers.Clusters: Specifies one or more destinations (servers) that should receive the requests. The
users-cluster
cluster in the example has a single destination with an address ofhttps://localhost:7247/
.Transforms: YARP supports request transformations, allowing you to modify the request before forwarding it to the backend. In this example, the transform ensures that the matched path is passed along to the destination.
Adding YARP to the Middleware Pipeline
After configuring YARP in appsettings.json
, you need to register it in your Startup.cs
(or Program.cs
in .NET 6 and later) file to make it part of the middleware pipeline.
Here’s how you can do that:
namespace Gateway.Api;
public class Program
{
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddReverseProxy()
.LoadFromConfig(builder.Configuration.GetSection("ReverseProxy"));
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.MapReverseProxy();
app.MapControllers();
app.Run();
}
}
In this setup:
services.AddReverseProxy()
registers the YARP services with the dependency injection container.LoadFromConfig
tells YARP to load the configuration from theappsettings.json
file.app.MapReverseProxy()
enable YARP in the middleware pipeline, allowing it to handle incoming requests.
Advanced Scenarios with YARP
While the basic setup will cover many use cases, YARP's true power lies in its extensibility and advanced features.
Custom Load Balancing: YARP supports custom load-balancing strategies. You can implement your own strategy by extending YARP’s default behavior.
Health Checks: YARP can monitor the health of your destinations and avoid routing traffic to unhealthy servers.
Rate Limiting: You can apply rate limiting to control the flow of traffic to your backend services, protecting them from being overwhelmed.
Custom Middleware: Since YARP is built on ASP.NET Core, you can leverage custom middleware to add logging, authentication, or any other functionality before or after requests are handled by YARP.
Conclusion
YARP is a robust and flexible reverse proxy solution tailored for .NET developers. Whether you need simple routing, complex load balancing, or advanced transformations, YARP provides the tools you need to build a performant and scalable reverse proxy. By leveraging YARP, you can ensure your applications are ready to handle the demands of modern web traffic, all while staying within the familiar .NET ecosystem.
Whether you’re working on a monolithic application that needs to scale or managing a microservices architecture, YARP offers a compelling solution for reverse proxying that integrates seamlessly with your .NET projects.
So, if you haven’t explored YARP yet, now is the time to dive in and see how it can help simplify and enhance your application’s architecture. Happy coding!
Subscribe to my newsletter
Read articles from Ejan Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
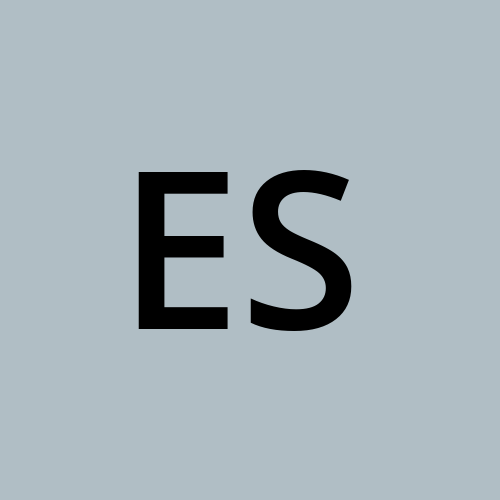